RAG vs Fine-Tuning: Choosing the Best AI Learning Technique
RAG vs Fine-Tuning: Choosing the Best AI Learning Technique
RAG vs Fine-Tuning: Choosing the Best AI Learning Technique
Jigar Gupta
Dec 27, 2024
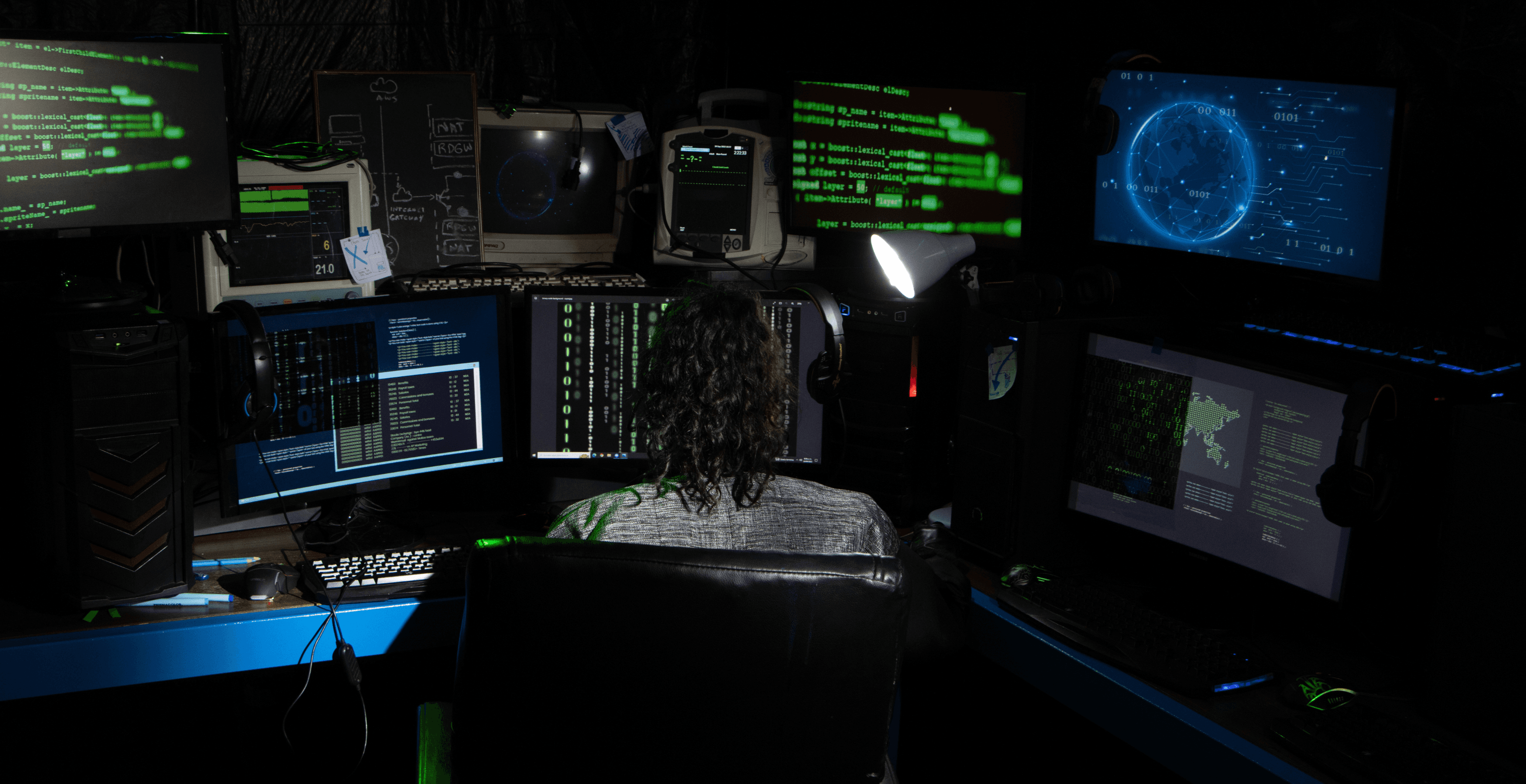
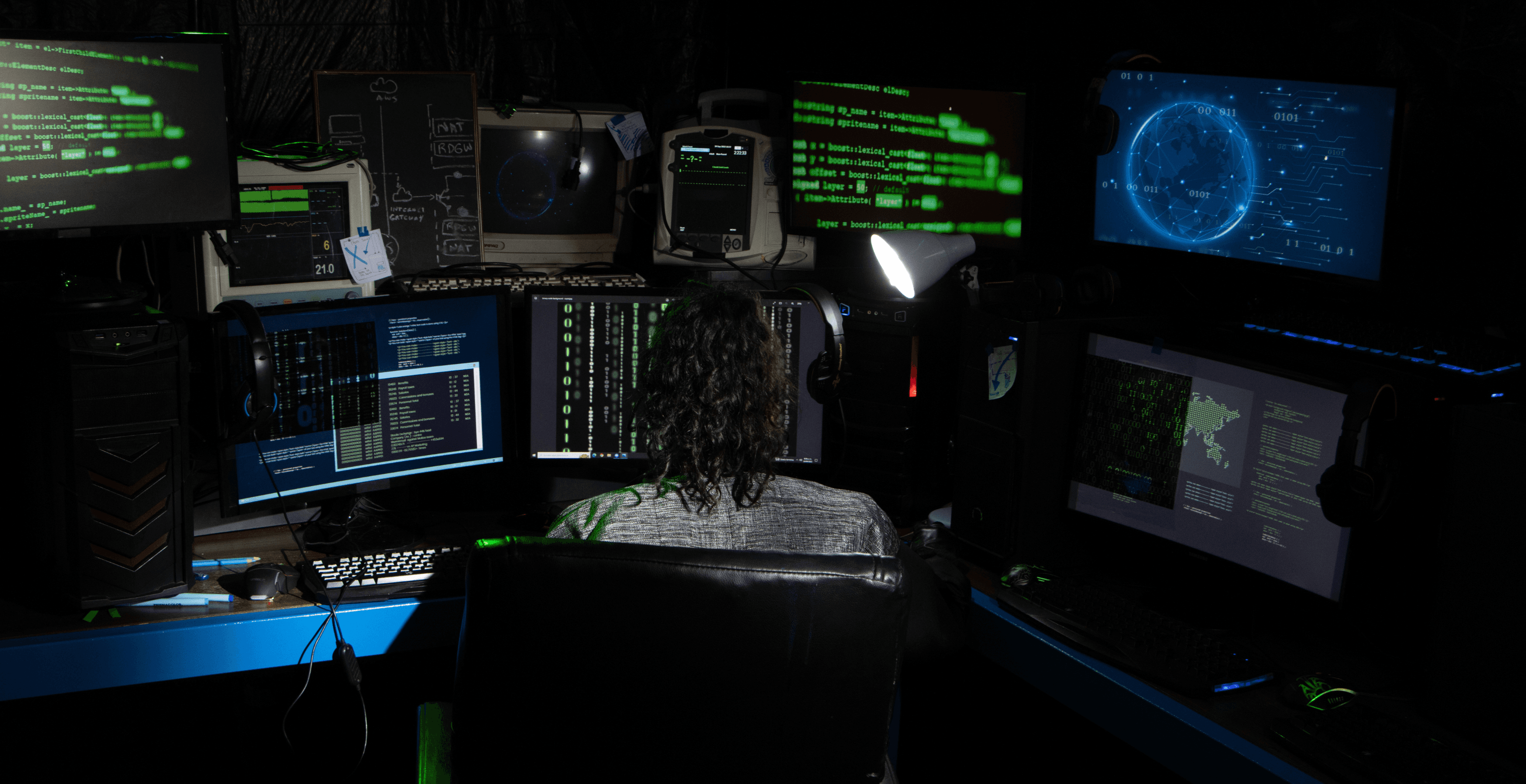
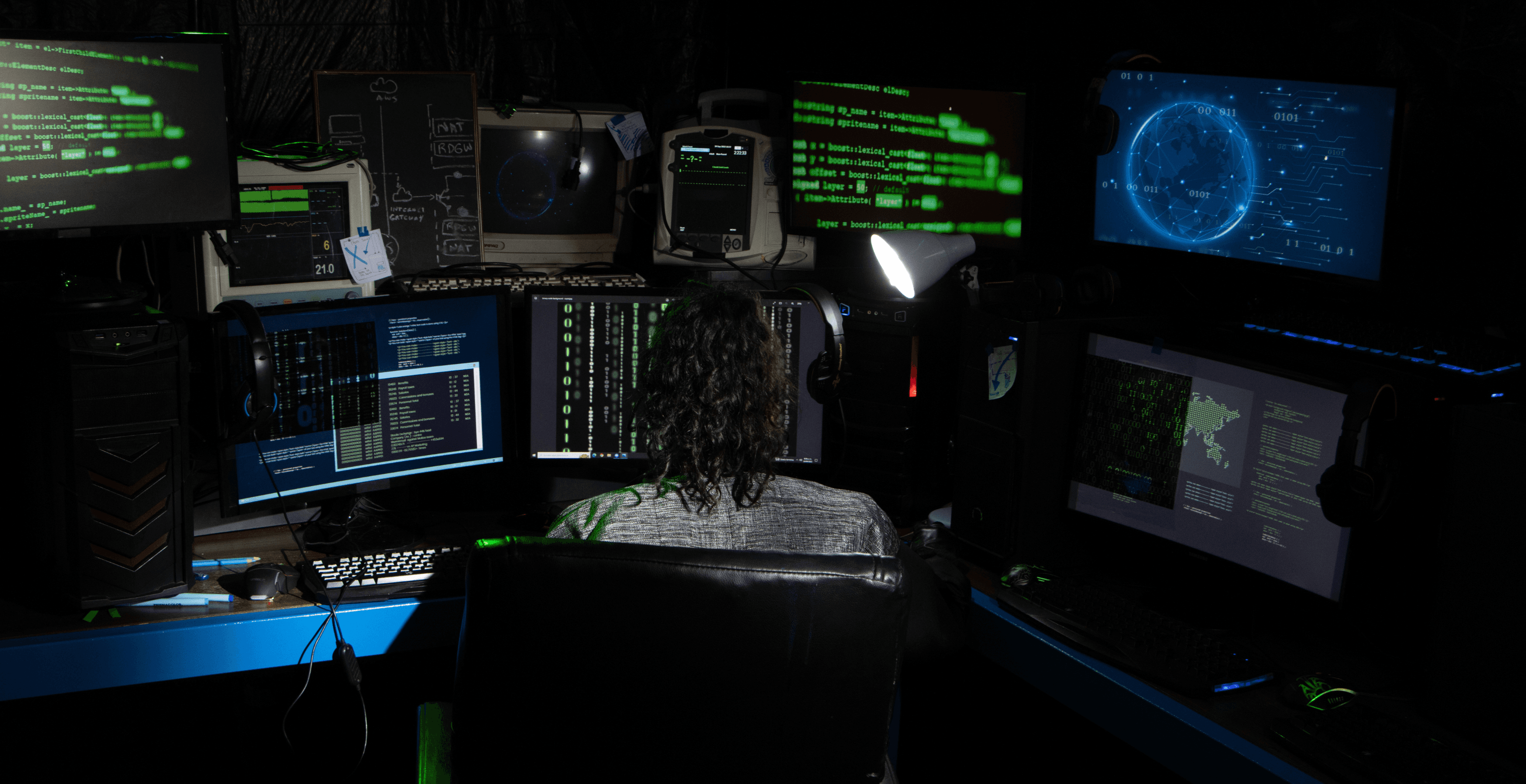
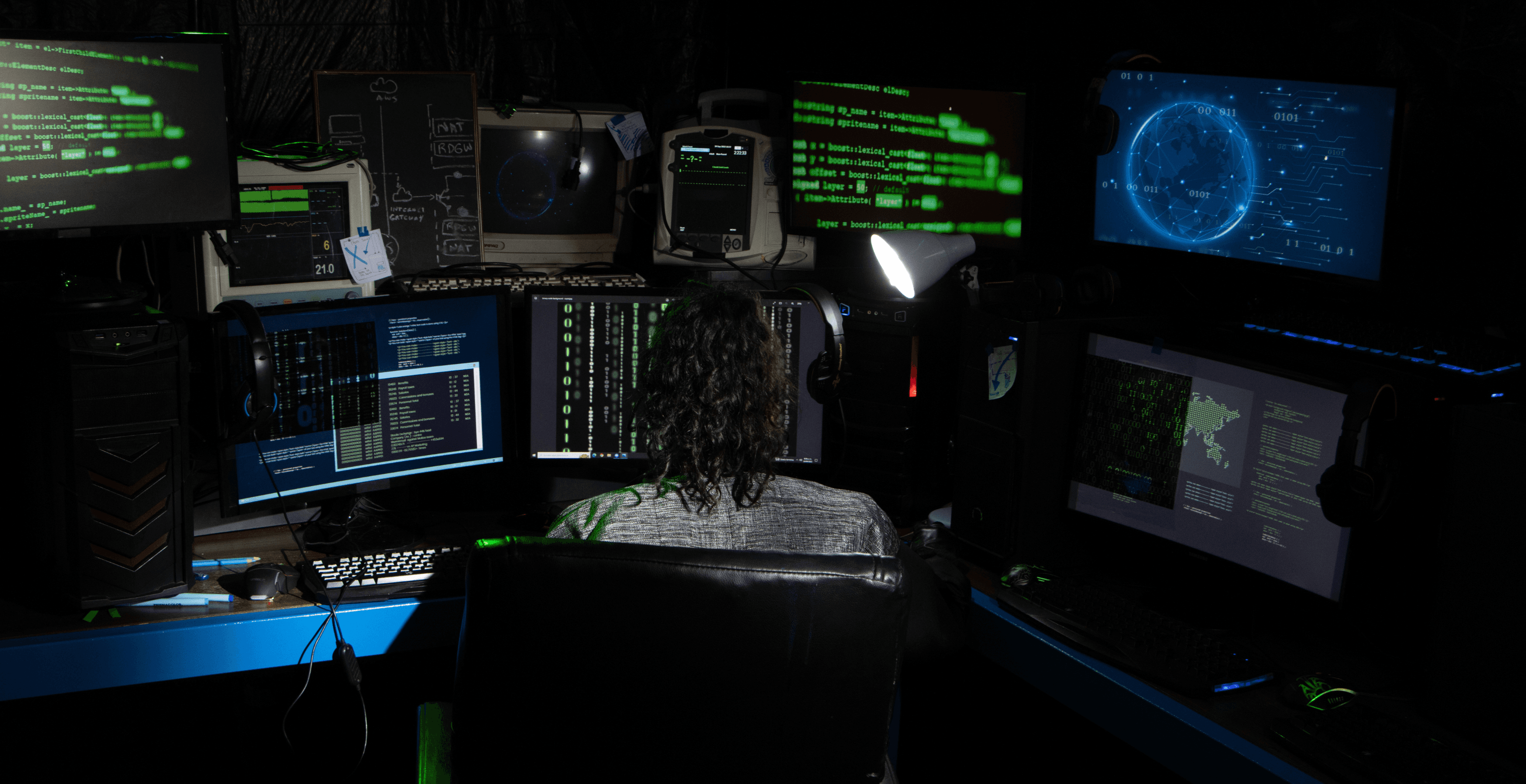
In the rapidly evolving world of AI, choosing the right learning technique can make all the difference in the performance and scalability of your models. Two of the most powerful methods in this space are Retrieval-Augmented Generation (RAG) and fine-tuning. But with each technique offering distinct advantages, how do you decide which is best for your application?
Understanding the core principles and how they impact your AI system is crucial in making an informed decision. The choice between these methods not only affects the accuracy and efficiency of your AI but also determines how scalable and adaptable your solutions will be in real-world scenarios.
Let’s dive deeper into each approach to see how they stack up against one another.
Understanding RAG (Retrieval-Augmented Generation)
Retrieval-Augmented Generation (RAG) is a powerful AI technique that combines the strengths of retrieval-based methods with generative models. Essentially, RAG enables models to enhance their responses by fetching relevant information from external data sources before generating an output. This approach not only boosts accuracy but also helps in reducing hallucinations, where the model might otherwise produce plausible but incorrect information.
How RAG Works
RAG operates by breaking down the generation process into two main steps: retrieval and generation. First, the model queries an external database or knowledge source to retrieve the most relevant pieces of information. Next, this retrieved data is integrated into the model’s generative process, helping it produce a response that is both contextually relevant and factually accurate.
Here’s a simplified example in Python using a pseudo RAG implementation:
def retrieve_information(query):
# Simulate retrieving relevant data from an external source
external_data = {
"query1": "Relevant information 1",
"query2": "Relevant information 2"
}
return external_data.get(query, "Default information")
def generate_response(query):
# Retrieve data using the RAG method
retrieved_data = retrieve_information(query)
# Integrate retrieved data into the response
response = f"Based on your query, here is what I found: {retrieved_data}"
return response
# Example usage
query = "query1"
response = generate_response(query)
print(response)
In this example, the retrieve_information function simulates fetching data from an external source, which then integrates into the response generated by the generate_response function.
Methods of RAG: Passive and Active
There are two primary methods of RAG: passive and active.
Passive RAG:
Passive RAG relies on existing data repositories, using them to retrieve information as needed during the generation process. The key characteristic of this method is that it does not modify or update the data source in real time.
For a query, the model pulls relevant information from a static database or knowledge base that has been pre-compiled. The retrieved data then integrates into the response generated by the model. Some of its advantages are:
Stability: Since the data source remains unchanged, passive RAG offers a consistent and stable reference for information retrieval, which is beneficial in scenarios where consistency is critical.
Simplicity: With no need for continuous updates, passive RAG is easier to implement and manage, making it suitable for applications where the data does not frequently change.
Efficiency: By relying on pre-existing, static data, passive RAG can be more efficient in terms of computational resources, as it does not require the overhead of updating or refining the data.
Active RAG:
Active RAG involves a more dynamic approach, where the data source is continuously updated and refined based on new inputs and information. This ensures that the model has access to the most current and relevant data.
As the model receives new inputs or queries, it not only retrieves information but also updates the data repository with new data, refining the knowledge base over time. This ongoing refinement allows the model to adapt to changes and incorporate fresh information into its responses. Some advantages are:
Adaptability: Active RAG is highly adaptable, making it ideal for environments where information is constantly changing. The model can adjust to new data, trends, or discoveries in real time, ensuring that the responses are always up-to-date.
Relevance: By continuously updating the data source, active RAG ensures that the information retrieved is as relevant as possible, which is particularly valuable in fields like medical research, legal analysis, or customer support.
Scalability: Active RAG can scale effectively with growing and evolving datasets, allowing the model to handle increasingly complex queries with accurate and timely information.
Each method of RAG has its own strengths, and the choice between passive and active RAG depends on the specific needs of the application—whether you require the consistency of static data or the adaptability to dynamic changes.
Internal Process: Query, Retrieve, Integrate, Generate
The summary of the internal workings of RAG in four key steps:
Query: The model formulates a query based on the user’s input.
Retrieve: It then retrieves relevant data from an external source.
Integrate: The retrieved information integrates into the model’s existing knowledge.
Generate: Finally, the model generates a response that combines the retrieved data with its internal processing capabilities.
This process allows RAG to produce outputs that are not only generated by the model but are also enriched with precise, real-world data, making it particularly effective for complex applications like legal research or technical support.
Interested in learning more about how to build RAG applications? Explore how to build RAG applications, ensuring safe and reliable genAI.
Next, we’ll explore how fine-tuning works, detailing the steps involved and the specific scenarios where this technique excels.
How Fine-Tuning Works
Fine-tuning is a critical technique in the AI learning process, allowing you to adapt pre-trained models to specific tasks by adjusting their parameters based on task-specific data. Unlike RAG, which relies on external data sources during generation, fine-tuning hones the internal parameters of a model, making it more specialized and accurate for a given task. Understanding the differences between RAG and fine-tuning is essential for selecting the best approach for your AI projects.
Steps Involved in Fine-Tuning
The fine-tuning process involves several steps that refine the model’s ability to perform a specific task. Here’s a breakdown of these steps:
Pre-train: Start with a pre-trained model on a large dataset. This model already understands a wide range of general knowledge, which forms the foundation for fine-tuning.
Task-Specific Data Preparation: Gather and prepare the data that is relevant to the specific task you want the model to perform. This step is crucial as the quality and relevance of this data will directly impact the effectiveness of the fine-tuning process.
Reprocess: Reprocess the pre-trained model’s layers to accommodate the new, task-specific data. It involves resetting certain weights and biases to ensure the model can learn the nuances of the new task.
Adjust Layers: Modify the model’s architecture, if necessary, to better suit the task. It could involve adding or removing layers or changing the activation functions to optimize performance.
Configure Model: Set up the model’s parameters, such as learning rate and batch size, to ensure optimal training. These settings play a crucial role in how quickly and effectively the model learns from the new data.
Train: Train the model on the task-specific data, allowing it to learn and adapt. This step typically involves multiple iterations, where the model’s parameters are adjusted based on the data it processes.
Evaluate: After training, evaluate the model’s performance on a validation set to ensure it has learned the task effectively. This step helps identify any issues or areas where further fine-tuning may be needed.
Iterate: Fine-tuning is often an iterative process. Based on the evaluation results, you may need to adjust the data, reconfigure the model, or train further to achieve the desired performance.
When comparing RAG and fine-tuning, it’s clear that fine-tuning requires less reliance on external data during inference but demands careful preparation and iteration to achieve optimal results.
Here’s a simplified code example to illustrate the fine-tuning process:
from transformers import Trainer, TrainingArguments
# Load pre-trained model
model = AutoModelForSequenceClassification.from_pretrained("bert-base-uncased")
# Load task-specific dataset
train_dataset = load_dataset("my_dataset", split="train")
# Define training arguments
training_args = TrainingArguments(
output_dir="./results",
evaluation_strategy="epoch",
learning_rate=2e-5,
per_device_train_batch_size=16,
num_train_epochs=3
)
# Initialize Trainer
trainer = Trainer(
model=model,
args=training_args,
train_dataset=train_dataset,
eval_dataset=val_dataset
)
# Train the model
trainer.train()
This code snippet fine-tunes a pre-trained model using task-specific data, highlighting how the process is more about adjusting and refining an existing model rather than relying on external information retrieval.
Understanding the fine-tuning process and how it contrasts with RAG is key to choosing the right technique for your AI projects. Fine-tuning offers a more focused approach, ideal for scenarios where specialized, high-accuracy models are required.
Want to know more about specific steps involved in optimizing AI models? Explore how Raga AI fine-tunes AI models for specific tasks with precision and efficiency.
Next, we’ll explore the advantages and limitations of RAG, giving you a clearer picture of when to use each technique.
Advantages and Limitations of RAG
Retrieval-Augmented Generation (RAG) is a distinctive approach in AI that merges retrieval-based methods with generative models. This technique offers several benefits but also comes with certain challenges. Understanding these can help you decide when to choose RAG over fine-tuning your AI projects.
Advantages of RAG
Improved Accuracy: RAG enhances accuracy by pulling in relevant, real-time information from external sources. This integration allows the model to generate responses that are not only contextually appropriate but also factually correct.
Reduced Hallucinations: One of RAG's key strengths is its ability to minimize hallucinations, where a model might otherwise produce convincing but incorrect information. By grounding responses in external data, RAG ensures that the information provided is reliable and based on actual data.
Adaptability to New Data: RAG is highly adaptable to new information because it retrieves data in real time. Unlike fine-tuning, which requires retraining the model with new data, RAG can incorporate fresh data on the fly, making it ideal for environments where information changes frequently.
Cost-Effectiveness: Since RAG utilizes existing data sources, it often requires less training data and fewer computational resources. It makes RAG a more cost-effective choice for projects that need to scale and access large volumes of information without the expense of retraining models frequently.
Limitations of RAG
Higher Latency: A significant drawback of RAG is the potential for increased latency. Because the model needs to fetch information from external sources before generating a response, this process can be slower compared to fine-tuned models that rely solely on internal data. It can be a concern in applications where speed is crucial.
Complex Architecture Requirements: Implementing RAG can require a more complex setup, especially when dealing with large-scale retrieval systems. Managing external databases and ensuring efficient data retrieval adds layers of complexity, making RAG more challenging to implement compared to fine-tuning.
When comparing RAG to fine-tuning, RAG excels in accuracy, adaptability, and cost-efficiency, particularly in scenarios where up-to-date information is essential. However, it’s important to consider the trade-offs, such as potential latency issues and the need for a more complex architecture, which might not be suitable for every application.
To learn more about the benefits of using RAG in AI systems, learn how Raga AI enhances AI performance by integrating retrieval-based techniques.
Next, we’ll explore the advantages and limitations of fine-tuning, giving you a deeper understanding of when each method is most appropriate.
Advantages and Limitations of Fine-Tuning
Fine-tuning is a powerful method in AI that allows you to refine a pre-trained model for specific tasks by adjusting its parameters. This approach is particularly effective in scenarios where precise, task-specific performance is required. However, like any technique, fine-tuning comes with its own set of benefits and drawbacks.
Advantages of Fine-Tuning
Less Training Data Required: Fine-tuning is efficient because it builds on an already pre-trained model. It means you need less task-specific data to achieve high accuracy, making it a practical choice when data is limited.
Improved Accuracy: Fine-tuning can significantly enhance the accuracy of a model for a specific task. By fine-tuning the model on a smaller, focused dataset, you can achieve a high level of precision that is well-suited to the particular needs of the application.
Increased Robustness: Through fine-tuning, the model becomes more robust in handling the nuances of the tailored task. It allows the model to perform better on specific, narrow tasks where general pre-trained models might struggle.
Limitations of Fine-Tuning
Potential for Forgetting: One of the main challenges of fine-tuning is the risk of the model "forgetting" the broader knowledge it gained during pre-training. It can occur when the model becomes too specialized in the new task, losing its ability to generalize.
Dependence on Training Data: The effectiveness of fine-tuning depends on the quality and relevance of the task-specific data. If the data is biased or incomplete, the model’s performance will suffer, leading to less reliable outcomes.
Lack of External Knowledge: Unlike RAG, which can pull in real-time information from external sources, fine-tuning relies solely on internal data. This can limit the model's ability to handle new or evolving information, making it less adaptable to changes.
When comparing RAG vs. fine-tuning, fine-tuning shines in tasks that require a deep focus on specific data, providing high accuracy and robustness with less training data. However, it also requires careful consideration of potential drawbacks like forgetting, data dependency, and the absence of external knowledge sources.
To learn more about the process of refining AI models for specific tasks, discover how Raga AI fine-tunes models to achieve high accuracy and reliability.
Next, we’ll dive into a direct comparison of RAG and fine-tuning, examining the factors that influence the choice between these two powerful AI techniques.
Comparison Factors: RAG vs Fine-Tuning
When deciding between Retrieval-Augmented Generation (RAG) and fine-tuning for your AI projects, it’s essential to consider several key factors. Each technique has its strengths and weaknesses, and understanding these can help you choose the best approach based on your specific needs.
External Data Access and Integration
RAG excels in scenarios where real-time access to external data is crucial. By retrieving information from databases or knowledge sources during the generation process, RAG ensures that the model’s responses are both accurate and up-to-date. In contrast, fine-tuning does not access external data during inference; it relies solely on its training data. This makes RAG the better choice when you need the model to handle dynamic or evolving information.
Model Behavior Adjustment
With fine-tuning, you can precisely adjust the behavior of your model by retraining it on task-specific data. This allows for a high degree of customization, making fine-tuning ideal for applications that require the model to perform specific, narrow tasks with great accuracy. RAG, on the other hand, adjusts behavior dynamically during inference by integrating retrieved data, offering flexibility without needing to retrain the model.
Hallucination Suppression
One of the significant advantages of RAG is its ability to suppress hallucinations by grounding responses in external data. This makes RAG particularly useful in applications where accuracy is critical, such as legal or medical fields. Fine-tuning, while capable of improving accuracy, can sometimes lead to overfitting, where the model might produce confident but incorrect outputs if not carefully managed.
Availability of Labeled Training Data
Fine-tuning requires a well-curated, labeled dataset for training. The quality of this data directly impacts the model’s performance. If such data is scarce or costly to obtain, RAG might be a more practical option since it can work with existing external sources, reducing the dependency on large, labeled datasets.
Handling Dynamic vs Static Data
RAG is highly effective in environments where data is dynamic and constantly changing. It allows the model to incorporate the latest information during inference, making it adaptable to new trends or facts. Fine-tuning is better suited for static data scenarios where the task does not require continuous updates, as it involves retraining the model with new data to reflect changes.
Transparency and Interpretability
When it comes to transparency, fine-tuning often provides clearer insights into how a model arrives at its decisions, as the adjustments are made directly within the model’s parameters. RAG, while powerful, can sometimes be less transparent due to the dynamic nature of data retrieval, making it harder to trace the exact source of information used in a response.
Computational Requirements and Scalability
RAG can be more computationally intensive, especially when dealing with large external databases, leading to higher resource consumption and potentially longer response times. Fine-tuning generally has lower runtime requirements since the model does not need to query external data during inference, making it more scalable for high-volume tasks.
Speed and Latency
In terms of speed, fine-tuning often outperforms RAG because it doesn’t involve the additional step of retrieving external data. This makes fine-tuning a better option for applications where low latency is critical. RAG, while potentially slower, offers the advantage of generating more informed and accurate responses by incorporating real-time data.
In summary, the choice between RAG vs. fine-tuning depends on the specific requirements of your project. RAG offers dynamic data integration and reduces hallucinations, making it ideal for complex, information-rich environments. Fine-tuning provides precise behavior adjustment and faster response times, suited for tasks where accuracy and speed are paramount.
To learn more about the differences between various AI model training techniques, explore how Raga AI evaluates and compares AI models to optimize performance and reliability.
Next, we’ll explore real-world use cases for RAG, demonstrating how this technique can be applied to enhance AI applications in various fields.
Use Cases for RAG
Retrieval-Augmented Generation (RAG) is particularly effective in scenarios where integrating real-time, external data into AI-generated responses is crucial. This approach allows AI systems to produce more accurate, contextually relevant, and up-to-date information. Here are some key use cases where RAG shines:
Chatbots and AI Technical Support
RAG is highly beneficial in developing advanced chatbots and AI-driven technical support systems. By retrieving the latest information from knowledge bases or databases, these systems can provide users with precise, relevant answers. For example, in technical support, RAG can pull in real-time troubleshooting steps from a database, ensuring that users receive the most accurate and helpful guidance possible.
Language Translation and Education Tools
In the field of language translation and educational tools, RAG can enhance the learning experience by integrating up-to-date language nuances or educational content into the generated responses. This is particularly useful in applications where the AI needs to stay current with evolving language trends or educational standards, providing learners with the most relevant information.
Medical Research and Diagnosis Augmentation
RAG can play a critical role in medical research and diagnosis, where access to the latest studies, clinical trials, or patient data is essential. By retrieving and integrating the most recent medical information, RAG-powered AI systems can assist healthcare professionals in making informed decisions, improving diagnosis accuracy, and suggesting up-to-date treatment options.
Legal Research and Review Tasks
In legal research, where precision and up-to-date information are paramount, RAG enables AI systems to retrieve relevant case law, statutes, and legal opinions from extensive databases. This capability allows legal professionals to conduct thorough research quickly, ensuring that they are referencing the most current and applicable legal precedents in their work.
Looking to apply RAG in your projects? Discover how Raga AI utilizes retrieval-based techniques to enhance the accuracy and reliability of AI systems.
Next, we’ll explore the use cases for fine-tuning, showing applications of this technique in different scenarios where deep task-specific learning is required.
Use Cases for Fine-Tuning
Fine-tuning is a versatile technique that excels in scenarios where AI models need to be adapted to perform specific tasks with high accuracy. By refining a pre-trained model using task-specific data, fine-tuning ensures that the AI is nuanced to the task at hand. Here are some key use cases where fine-tuning is particularly effective:
Sentiment Analysis
In sentiment analysis, where understanding the emotional tone of the text is critical, fine-tuning allows you to train the AI models on specific datasets that reflect the sentiment patterns relevant to the application. For example, a model fine-tuned on customer reviews can accurately determine whether a review is positive, negative, or neutral, helping businesses understand customer feedback more precisely.
Named-Entity Recognition (NER)
Named-entity recognition involves identifying and classifying key entities within a text, such as names, dates, or locations. Fine-tuning is essential in this area because it enables the AI model to learn the specific entity types that are important for the task. Whether it’s for legal document analysis or extracting key information from medical records, fine-tuning ensures that the model can recognize and categorize entities with high accuracy.
Personalized Content Recommendation
In personalized content recommendation systems, fine-tuning helps tailor the AI’s recommendations to individual user preferences. By training the model on data that reflects user behavior and preferences, fine-tuning ensures that the content suggested is highly relevant and engaging. This approach is widely used in streaming services, e-commerce platforms, and social media, where personalized recommendations are crucial for user satisfaction.
Specific Domain Summarization
Fine-tuning is also highly effective in domain-specific summarization tasks, where the goal is to generate concise summaries of lengthy documents within a particular field. For example, a model fine-tuned on legal documents can summarize complex contracts, while a model fine-tuned on scientific papers can distill key findings into a summary. This specialization allows the AI to produce summaries that are not only accurate but also aligned with the specific requirements of the domain.
Ready to see how fine-tuning can enhance your AI applications? Learn how Raga AI fine-tunes models to achieve high accuracy and reliability for specific tasks.
Next, we’ll explore how combining RAG and fine-tuning can utilize the strengths of both techniques, offering even more powerful AI solutions for complex applications.
Combining RAG and Fine-Tuning
While Retrieval-Augmented Generation (RAG) and fine-tuning each offer distinct advantages, combining these two techniques can reveal even greater potential in AI applications. By leveraging the strengths of both methods, you can create AI systems that are not only highly accurate but also adaptable and resource-efficient, making them ideal for complex, real-world scenarios.
Hybrid Approaches for Both Techniques
Combining RAG and fine-tuning allows you to harness the dynamic data integration of RAG alongside the specialized accuracy of fine-tuning. For instance, a model can be fine-tuned on task-specific data to master a particular domain, ensuring it performs with high precision. At the same time, you can integrate RAG to pull in real-time data during inference, allowing the model to adapt to new information or trends that weren’t available during training. This hybrid approach ensures that your AI model remains both deeply knowledgeable and highly responsive to changes.
Example: Customer Support Automation Using Both Methods
Consider a customer support chatbot that needs to handle a wide range of inquiries, from frequently asked questions to complex technical issues. By fine-tuning the model on a dataset of common customer queries, the chatbot can provide accurate, task-specific responses. However, when a new or unusual question arises, RAG can be employed to retrieve the latest information from a knowledge base or support database, ensuring the chatbot delivers a relevant and up-to-date answer. This combination of fine-tuning for accuracy and RAG for adaptability creates a robust customer support system capable of handling both routine and novel queries effectively.
Curious about how to implement a hybrid AI approach? Discover how Raga AI combines advanced techniques to create powerful, adaptable AI systems.
Looking ahead, we’ll explore future trends and recommendations, including the potential for hybrid models, advancements in hardware, and practical advice for choosing the best AI techniques for your needs.
Future Trends and Recommendations
As AI technology continues to evolve, you can expect the integration of techniques like Retrieval-Augmented Generation (RAG) and fine-tuning to become more sophisticated. The future holds exciting possibilities for hybrid models that blend the strengths of both approaches, creating AI systems that are not only highly accurate but also incredibly adaptable to new data and changing environments.
Potential for Hybrid Models
The ongoing development of hybrid models that combine RAG and fine-tuning is likely to reshape how AI performs across industries. These models will benefit from the precision of fine-tuning for specific tasks while using RAG to integrate real-time information, ensuring that AI systems can respond dynamically to new challenges. As the demand for more versatile and intelligent AI solutions grows, hybrid models will play a critical role in meeting these needs.
Hardware Advancements
Advancements in AI hardware can also reduce the computational limitations that currently restrict the use of complex models. With the development of more powerful processors and optimized algorithms, running sophisticated hybrid models will become more feasible even in resource-constrained environments. This will open up new opportunities for deploying advanced AI systems in areas like healthcare, finance, and education, where computational efficiency is crucial.
Increasing Data Improving Methods
As more data becomes available, both RAG and fine-tuning techniques will continue to improve in effectiveness. The ability to access vast amounts of data in real time will enhance the accuracy and relevance of AI models, making them more capable of delivering actionable insights. Organizations should focus on building robust data pipelines that feed their AI systems with high-quality, diverse datasets to maximize the potential of these techniques.
Practical Recommendations
When deciding between RAG and fine-tuning—or whether to combine them—it’s important to consider the specific needs of your project. For tasks that require real-time data integration and adaptability, RAG is an excellent choice. Fine-tuning, on the other hand, is ideal for scenarios where precise, task-specific accuracy is critical. In many cases, a hybrid approach may offer the best of both worlds, providing a balance between accuracy and adaptability.
At Raga AI, we are at the forefront of these exciting developments. Our expertise in combining RAG, fine-tuning, and other advanced techniques enables us to create AI systems that are not only accurate but also adaptable and efficient. Whether you’re looking to enhance your existing AI capabilities or explore new possibilities, Raga AI provides the tools and insights you need to stay ahead in the rapidly evolving AI market. Ready to transform your AI systems? Try Raga AI today!
In the rapidly evolving world of AI, choosing the right learning technique can make all the difference in the performance and scalability of your models. Two of the most powerful methods in this space are Retrieval-Augmented Generation (RAG) and fine-tuning. But with each technique offering distinct advantages, how do you decide which is best for your application?
Understanding the core principles and how they impact your AI system is crucial in making an informed decision. The choice between these methods not only affects the accuracy and efficiency of your AI but also determines how scalable and adaptable your solutions will be in real-world scenarios.
Let’s dive deeper into each approach to see how they stack up against one another.
Understanding RAG (Retrieval-Augmented Generation)
Retrieval-Augmented Generation (RAG) is a powerful AI technique that combines the strengths of retrieval-based methods with generative models. Essentially, RAG enables models to enhance their responses by fetching relevant information from external data sources before generating an output. This approach not only boosts accuracy but also helps in reducing hallucinations, where the model might otherwise produce plausible but incorrect information.
How RAG Works
RAG operates by breaking down the generation process into two main steps: retrieval and generation. First, the model queries an external database or knowledge source to retrieve the most relevant pieces of information. Next, this retrieved data is integrated into the model’s generative process, helping it produce a response that is both contextually relevant and factually accurate.
Here’s a simplified example in Python using a pseudo RAG implementation:
def retrieve_information(query):
# Simulate retrieving relevant data from an external source
external_data = {
"query1": "Relevant information 1",
"query2": "Relevant information 2"
}
return external_data.get(query, "Default information")
def generate_response(query):
# Retrieve data using the RAG method
retrieved_data = retrieve_information(query)
# Integrate retrieved data into the response
response = f"Based on your query, here is what I found: {retrieved_data}"
return response
# Example usage
query = "query1"
response = generate_response(query)
print(response)
In this example, the retrieve_information function simulates fetching data from an external source, which then integrates into the response generated by the generate_response function.
Methods of RAG: Passive and Active
There are two primary methods of RAG: passive and active.
Passive RAG:
Passive RAG relies on existing data repositories, using them to retrieve information as needed during the generation process. The key characteristic of this method is that it does not modify or update the data source in real time.
For a query, the model pulls relevant information from a static database or knowledge base that has been pre-compiled. The retrieved data then integrates into the response generated by the model. Some of its advantages are:
Stability: Since the data source remains unchanged, passive RAG offers a consistent and stable reference for information retrieval, which is beneficial in scenarios where consistency is critical.
Simplicity: With no need for continuous updates, passive RAG is easier to implement and manage, making it suitable for applications where the data does not frequently change.
Efficiency: By relying on pre-existing, static data, passive RAG can be more efficient in terms of computational resources, as it does not require the overhead of updating or refining the data.
Active RAG:
Active RAG involves a more dynamic approach, where the data source is continuously updated and refined based on new inputs and information. This ensures that the model has access to the most current and relevant data.
As the model receives new inputs or queries, it not only retrieves information but also updates the data repository with new data, refining the knowledge base over time. This ongoing refinement allows the model to adapt to changes and incorporate fresh information into its responses. Some advantages are:
Adaptability: Active RAG is highly adaptable, making it ideal for environments where information is constantly changing. The model can adjust to new data, trends, or discoveries in real time, ensuring that the responses are always up-to-date.
Relevance: By continuously updating the data source, active RAG ensures that the information retrieved is as relevant as possible, which is particularly valuable in fields like medical research, legal analysis, or customer support.
Scalability: Active RAG can scale effectively with growing and evolving datasets, allowing the model to handle increasingly complex queries with accurate and timely information.
Each method of RAG has its own strengths, and the choice between passive and active RAG depends on the specific needs of the application—whether you require the consistency of static data or the adaptability to dynamic changes.
Internal Process: Query, Retrieve, Integrate, Generate
The summary of the internal workings of RAG in four key steps:
Query: The model formulates a query based on the user’s input.
Retrieve: It then retrieves relevant data from an external source.
Integrate: The retrieved information integrates into the model’s existing knowledge.
Generate: Finally, the model generates a response that combines the retrieved data with its internal processing capabilities.
This process allows RAG to produce outputs that are not only generated by the model but are also enriched with precise, real-world data, making it particularly effective for complex applications like legal research or technical support.
Interested in learning more about how to build RAG applications? Explore how to build RAG applications, ensuring safe and reliable genAI.
Next, we’ll explore how fine-tuning works, detailing the steps involved and the specific scenarios where this technique excels.
How Fine-Tuning Works
Fine-tuning is a critical technique in the AI learning process, allowing you to adapt pre-trained models to specific tasks by adjusting their parameters based on task-specific data. Unlike RAG, which relies on external data sources during generation, fine-tuning hones the internal parameters of a model, making it more specialized and accurate for a given task. Understanding the differences between RAG and fine-tuning is essential for selecting the best approach for your AI projects.
Steps Involved in Fine-Tuning
The fine-tuning process involves several steps that refine the model’s ability to perform a specific task. Here’s a breakdown of these steps:
Pre-train: Start with a pre-trained model on a large dataset. This model already understands a wide range of general knowledge, which forms the foundation for fine-tuning.
Task-Specific Data Preparation: Gather and prepare the data that is relevant to the specific task you want the model to perform. This step is crucial as the quality and relevance of this data will directly impact the effectiveness of the fine-tuning process.
Reprocess: Reprocess the pre-trained model’s layers to accommodate the new, task-specific data. It involves resetting certain weights and biases to ensure the model can learn the nuances of the new task.
Adjust Layers: Modify the model’s architecture, if necessary, to better suit the task. It could involve adding or removing layers or changing the activation functions to optimize performance.
Configure Model: Set up the model’s parameters, such as learning rate and batch size, to ensure optimal training. These settings play a crucial role in how quickly and effectively the model learns from the new data.
Train: Train the model on the task-specific data, allowing it to learn and adapt. This step typically involves multiple iterations, where the model’s parameters are adjusted based on the data it processes.
Evaluate: After training, evaluate the model’s performance on a validation set to ensure it has learned the task effectively. This step helps identify any issues or areas where further fine-tuning may be needed.
Iterate: Fine-tuning is often an iterative process. Based on the evaluation results, you may need to adjust the data, reconfigure the model, or train further to achieve the desired performance.
When comparing RAG and fine-tuning, it’s clear that fine-tuning requires less reliance on external data during inference but demands careful preparation and iteration to achieve optimal results.
Here’s a simplified code example to illustrate the fine-tuning process:
from transformers import Trainer, TrainingArguments
# Load pre-trained model
model = AutoModelForSequenceClassification.from_pretrained("bert-base-uncased")
# Load task-specific dataset
train_dataset = load_dataset("my_dataset", split="train")
# Define training arguments
training_args = TrainingArguments(
output_dir="./results",
evaluation_strategy="epoch",
learning_rate=2e-5,
per_device_train_batch_size=16,
num_train_epochs=3
)
# Initialize Trainer
trainer = Trainer(
model=model,
args=training_args,
train_dataset=train_dataset,
eval_dataset=val_dataset
)
# Train the model
trainer.train()
This code snippet fine-tunes a pre-trained model using task-specific data, highlighting how the process is more about adjusting and refining an existing model rather than relying on external information retrieval.
Understanding the fine-tuning process and how it contrasts with RAG is key to choosing the right technique for your AI projects. Fine-tuning offers a more focused approach, ideal for scenarios where specialized, high-accuracy models are required.
Want to know more about specific steps involved in optimizing AI models? Explore how Raga AI fine-tunes AI models for specific tasks with precision and efficiency.
Next, we’ll explore the advantages and limitations of RAG, giving you a clearer picture of when to use each technique.
Advantages and Limitations of RAG
Retrieval-Augmented Generation (RAG) is a distinctive approach in AI that merges retrieval-based methods with generative models. This technique offers several benefits but also comes with certain challenges. Understanding these can help you decide when to choose RAG over fine-tuning your AI projects.
Advantages of RAG
Improved Accuracy: RAG enhances accuracy by pulling in relevant, real-time information from external sources. This integration allows the model to generate responses that are not only contextually appropriate but also factually correct.
Reduced Hallucinations: One of RAG's key strengths is its ability to minimize hallucinations, where a model might otherwise produce convincing but incorrect information. By grounding responses in external data, RAG ensures that the information provided is reliable and based on actual data.
Adaptability to New Data: RAG is highly adaptable to new information because it retrieves data in real time. Unlike fine-tuning, which requires retraining the model with new data, RAG can incorporate fresh data on the fly, making it ideal for environments where information changes frequently.
Cost-Effectiveness: Since RAG utilizes existing data sources, it often requires less training data and fewer computational resources. It makes RAG a more cost-effective choice for projects that need to scale and access large volumes of information without the expense of retraining models frequently.
Limitations of RAG
Higher Latency: A significant drawback of RAG is the potential for increased latency. Because the model needs to fetch information from external sources before generating a response, this process can be slower compared to fine-tuned models that rely solely on internal data. It can be a concern in applications where speed is crucial.
Complex Architecture Requirements: Implementing RAG can require a more complex setup, especially when dealing with large-scale retrieval systems. Managing external databases and ensuring efficient data retrieval adds layers of complexity, making RAG more challenging to implement compared to fine-tuning.
When comparing RAG to fine-tuning, RAG excels in accuracy, adaptability, and cost-efficiency, particularly in scenarios where up-to-date information is essential. However, it’s important to consider the trade-offs, such as potential latency issues and the need for a more complex architecture, which might not be suitable for every application.
To learn more about the benefits of using RAG in AI systems, learn how Raga AI enhances AI performance by integrating retrieval-based techniques.
Next, we’ll explore the advantages and limitations of fine-tuning, giving you a deeper understanding of when each method is most appropriate.
Advantages and Limitations of Fine-Tuning
Fine-tuning is a powerful method in AI that allows you to refine a pre-trained model for specific tasks by adjusting its parameters. This approach is particularly effective in scenarios where precise, task-specific performance is required. However, like any technique, fine-tuning comes with its own set of benefits and drawbacks.
Advantages of Fine-Tuning
Less Training Data Required: Fine-tuning is efficient because it builds on an already pre-trained model. It means you need less task-specific data to achieve high accuracy, making it a practical choice when data is limited.
Improved Accuracy: Fine-tuning can significantly enhance the accuracy of a model for a specific task. By fine-tuning the model on a smaller, focused dataset, you can achieve a high level of precision that is well-suited to the particular needs of the application.
Increased Robustness: Through fine-tuning, the model becomes more robust in handling the nuances of the tailored task. It allows the model to perform better on specific, narrow tasks where general pre-trained models might struggle.
Limitations of Fine-Tuning
Potential for Forgetting: One of the main challenges of fine-tuning is the risk of the model "forgetting" the broader knowledge it gained during pre-training. It can occur when the model becomes too specialized in the new task, losing its ability to generalize.
Dependence on Training Data: The effectiveness of fine-tuning depends on the quality and relevance of the task-specific data. If the data is biased or incomplete, the model’s performance will suffer, leading to less reliable outcomes.
Lack of External Knowledge: Unlike RAG, which can pull in real-time information from external sources, fine-tuning relies solely on internal data. This can limit the model's ability to handle new or evolving information, making it less adaptable to changes.
When comparing RAG vs. fine-tuning, fine-tuning shines in tasks that require a deep focus on specific data, providing high accuracy and robustness with less training data. However, it also requires careful consideration of potential drawbacks like forgetting, data dependency, and the absence of external knowledge sources.
To learn more about the process of refining AI models for specific tasks, discover how Raga AI fine-tunes models to achieve high accuracy and reliability.
Next, we’ll dive into a direct comparison of RAG and fine-tuning, examining the factors that influence the choice between these two powerful AI techniques.
Comparison Factors: RAG vs Fine-Tuning
When deciding between Retrieval-Augmented Generation (RAG) and fine-tuning for your AI projects, it’s essential to consider several key factors. Each technique has its strengths and weaknesses, and understanding these can help you choose the best approach based on your specific needs.
External Data Access and Integration
RAG excels in scenarios where real-time access to external data is crucial. By retrieving information from databases or knowledge sources during the generation process, RAG ensures that the model’s responses are both accurate and up-to-date. In contrast, fine-tuning does not access external data during inference; it relies solely on its training data. This makes RAG the better choice when you need the model to handle dynamic or evolving information.
Model Behavior Adjustment
With fine-tuning, you can precisely adjust the behavior of your model by retraining it on task-specific data. This allows for a high degree of customization, making fine-tuning ideal for applications that require the model to perform specific, narrow tasks with great accuracy. RAG, on the other hand, adjusts behavior dynamically during inference by integrating retrieved data, offering flexibility without needing to retrain the model.
Hallucination Suppression
One of the significant advantages of RAG is its ability to suppress hallucinations by grounding responses in external data. This makes RAG particularly useful in applications where accuracy is critical, such as legal or medical fields. Fine-tuning, while capable of improving accuracy, can sometimes lead to overfitting, where the model might produce confident but incorrect outputs if not carefully managed.
Availability of Labeled Training Data
Fine-tuning requires a well-curated, labeled dataset for training. The quality of this data directly impacts the model’s performance. If such data is scarce or costly to obtain, RAG might be a more practical option since it can work with existing external sources, reducing the dependency on large, labeled datasets.
Handling Dynamic vs Static Data
RAG is highly effective in environments where data is dynamic and constantly changing. It allows the model to incorporate the latest information during inference, making it adaptable to new trends or facts. Fine-tuning is better suited for static data scenarios where the task does not require continuous updates, as it involves retraining the model with new data to reflect changes.
Transparency and Interpretability
When it comes to transparency, fine-tuning often provides clearer insights into how a model arrives at its decisions, as the adjustments are made directly within the model’s parameters. RAG, while powerful, can sometimes be less transparent due to the dynamic nature of data retrieval, making it harder to trace the exact source of information used in a response.
Computational Requirements and Scalability
RAG can be more computationally intensive, especially when dealing with large external databases, leading to higher resource consumption and potentially longer response times. Fine-tuning generally has lower runtime requirements since the model does not need to query external data during inference, making it more scalable for high-volume tasks.
Speed and Latency
In terms of speed, fine-tuning often outperforms RAG because it doesn’t involve the additional step of retrieving external data. This makes fine-tuning a better option for applications where low latency is critical. RAG, while potentially slower, offers the advantage of generating more informed and accurate responses by incorporating real-time data.
In summary, the choice between RAG vs. fine-tuning depends on the specific requirements of your project. RAG offers dynamic data integration and reduces hallucinations, making it ideal for complex, information-rich environments. Fine-tuning provides precise behavior adjustment and faster response times, suited for tasks where accuracy and speed are paramount.
To learn more about the differences between various AI model training techniques, explore how Raga AI evaluates and compares AI models to optimize performance and reliability.
Next, we’ll explore real-world use cases for RAG, demonstrating how this technique can be applied to enhance AI applications in various fields.
Use Cases for RAG
Retrieval-Augmented Generation (RAG) is particularly effective in scenarios where integrating real-time, external data into AI-generated responses is crucial. This approach allows AI systems to produce more accurate, contextually relevant, and up-to-date information. Here are some key use cases where RAG shines:
Chatbots and AI Technical Support
RAG is highly beneficial in developing advanced chatbots and AI-driven technical support systems. By retrieving the latest information from knowledge bases or databases, these systems can provide users with precise, relevant answers. For example, in technical support, RAG can pull in real-time troubleshooting steps from a database, ensuring that users receive the most accurate and helpful guidance possible.
Language Translation and Education Tools
In the field of language translation and educational tools, RAG can enhance the learning experience by integrating up-to-date language nuances or educational content into the generated responses. This is particularly useful in applications where the AI needs to stay current with evolving language trends or educational standards, providing learners with the most relevant information.
Medical Research and Diagnosis Augmentation
RAG can play a critical role in medical research and diagnosis, where access to the latest studies, clinical trials, or patient data is essential. By retrieving and integrating the most recent medical information, RAG-powered AI systems can assist healthcare professionals in making informed decisions, improving diagnosis accuracy, and suggesting up-to-date treatment options.
Legal Research and Review Tasks
In legal research, where precision and up-to-date information are paramount, RAG enables AI systems to retrieve relevant case law, statutes, and legal opinions from extensive databases. This capability allows legal professionals to conduct thorough research quickly, ensuring that they are referencing the most current and applicable legal precedents in their work.
Looking to apply RAG in your projects? Discover how Raga AI utilizes retrieval-based techniques to enhance the accuracy and reliability of AI systems.
Next, we’ll explore the use cases for fine-tuning, showing applications of this technique in different scenarios where deep task-specific learning is required.
Use Cases for Fine-Tuning
Fine-tuning is a versatile technique that excels in scenarios where AI models need to be adapted to perform specific tasks with high accuracy. By refining a pre-trained model using task-specific data, fine-tuning ensures that the AI is nuanced to the task at hand. Here are some key use cases where fine-tuning is particularly effective:
Sentiment Analysis
In sentiment analysis, where understanding the emotional tone of the text is critical, fine-tuning allows you to train the AI models on specific datasets that reflect the sentiment patterns relevant to the application. For example, a model fine-tuned on customer reviews can accurately determine whether a review is positive, negative, or neutral, helping businesses understand customer feedback more precisely.
Named-Entity Recognition (NER)
Named-entity recognition involves identifying and classifying key entities within a text, such as names, dates, or locations. Fine-tuning is essential in this area because it enables the AI model to learn the specific entity types that are important for the task. Whether it’s for legal document analysis or extracting key information from medical records, fine-tuning ensures that the model can recognize and categorize entities with high accuracy.
Personalized Content Recommendation
In personalized content recommendation systems, fine-tuning helps tailor the AI’s recommendations to individual user preferences. By training the model on data that reflects user behavior and preferences, fine-tuning ensures that the content suggested is highly relevant and engaging. This approach is widely used in streaming services, e-commerce platforms, and social media, where personalized recommendations are crucial for user satisfaction.
Specific Domain Summarization
Fine-tuning is also highly effective in domain-specific summarization tasks, where the goal is to generate concise summaries of lengthy documents within a particular field. For example, a model fine-tuned on legal documents can summarize complex contracts, while a model fine-tuned on scientific papers can distill key findings into a summary. This specialization allows the AI to produce summaries that are not only accurate but also aligned with the specific requirements of the domain.
Ready to see how fine-tuning can enhance your AI applications? Learn how Raga AI fine-tunes models to achieve high accuracy and reliability for specific tasks.
Next, we’ll explore how combining RAG and fine-tuning can utilize the strengths of both techniques, offering even more powerful AI solutions for complex applications.
Combining RAG and Fine-Tuning
While Retrieval-Augmented Generation (RAG) and fine-tuning each offer distinct advantages, combining these two techniques can reveal even greater potential in AI applications. By leveraging the strengths of both methods, you can create AI systems that are not only highly accurate but also adaptable and resource-efficient, making them ideal for complex, real-world scenarios.
Hybrid Approaches for Both Techniques
Combining RAG and fine-tuning allows you to harness the dynamic data integration of RAG alongside the specialized accuracy of fine-tuning. For instance, a model can be fine-tuned on task-specific data to master a particular domain, ensuring it performs with high precision. At the same time, you can integrate RAG to pull in real-time data during inference, allowing the model to adapt to new information or trends that weren’t available during training. This hybrid approach ensures that your AI model remains both deeply knowledgeable and highly responsive to changes.
Example: Customer Support Automation Using Both Methods
Consider a customer support chatbot that needs to handle a wide range of inquiries, from frequently asked questions to complex technical issues. By fine-tuning the model on a dataset of common customer queries, the chatbot can provide accurate, task-specific responses. However, when a new or unusual question arises, RAG can be employed to retrieve the latest information from a knowledge base or support database, ensuring the chatbot delivers a relevant and up-to-date answer. This combination of fine-tuning for accuracy and RAG for adaptability creates a robust customer support system capable of handling both routine and novel queries effectively.
Curious about how to implement a hybrid AI approach? Discover how Raga AI combines advanced techniques to create powerful, adaptable AI systems.
Looking ahead, we’ll explore future trends and recommendations, including the potential for hybrid models, advancements in hardware, and practical advice for choosing the best AI techniques for your needs.
Future Trends and Recommendations
As AI technology continues to evolve, you can expect the integration of techniques like Retrieval-Augmented Generation (RAG) and fine-tuning to become more sophisticated. The future holds exciting possibilities for hybrid models that blend the strengths of both approaches, creating AI systems that are not only highly accurate but also incredibly adaptable to new data and changing environments.
Potential for Hybrid Models
The ongoing development of hybrid models that combine RAG and fine-tuning is likely to reshape how AI performs across industries. These models will benefit from the precision of fine-tuning for specific tasks while using RAG to integrate real-time information, ensuring that AI systems can respond dynamically to new challenges. As the demand for more versatile and intelligent AI solutions grows, hybrid models will play a critical role in meeting these needs.
Hardware Advancements
Advancements in AI hardware can also reduce the computational limitations that currently restrict the use of complex models. With the development of more powerful processors and optimized algorithms, running sophisticated hybrid models will become more feasible even in resource-constrained environments. This will open up new opportunities for deploying advanced AI systems in areas like healthcare, finance, and education, where computational efficiency is crucial.
Increasing Data Improving Methods
As more data becomes available, both RAG and fine-tuning techniques will continue to improve in effectiveness. The ability to access vast amounts of data in real time will enhance the accuracy and relevance of AI models, making them more capable of delivering actionable insights. Organizations should focus on building robust data pipelines that feed their AI systems with high-quality, diverse datasets to maximize the potential of these techniques.
Practical Recommendations
When deciding between RAG and fine-tuning—or whether to combine them—it’s important to consider the specific needs of your project. For tasks that require real-time data integration and adaptability, RAG is an excellent choice. Fine-tuning, on the other hand, is ideal for scenarios where precise, task-specific accuracy is critical. In many cases, a hybrid approach may offer the best of both worlds, providing a balance between accuracy and adaptability.
At Raga AI, we are at the forefront of these exciting developments. Our expertise in combining RAG, fine-tuning, and other advanced techniques enables us to create AI systems that are not only accurate but also adaptable and efficient. Whether you’re looking to enhance your existing AI capabilities or explore new possibilities, Raga AI provides the tools and insights you need to stay ahead in the rapidly evolving AI market. Ready to transform your AI systems? Try Raga AI today!
In the rapidly evolving world of AI, choosing the right learning technique can make all the difference in the performance and scalability of your models. Two of the most powerful methods in this space are Retrieval-Augmented Generation (RAG) and fine-tuning. But with each technique offering distinct advantages, how do you decide which is best for your application?
Understanding the core principles and how they impact your AI system is crucial in making an informed decision. The choice between these methods not only affects the accuracy and efficiency of your AI but also determines how scalable and adaptable your solutions will be in real-world scenarios.
Let’s dive deeper into each approach to see how they stack up against one another.
Understanding RAG (Retrieval-Augmented Generation)
Retrieval-Augmented Generation (RAG) is a powerful AI technique that combines the strengths of retrieval-based methods with generative models. Essentially, RAG enables models to enhance their responses by fetching relevant information from external data sources before generating an output. This approach not only boosts accuracy but also helps in reducing hallucinations, where the model might otherwise produce plausible but incorrect information.
How RAG Works
RAG operates by breaking down the generation process into two main steps: retrieval and generation. First, the model queries an external database or knowledge source to retrieve the most relevant pieces of information. Next, this retrieved data is integrated into the model’s generative process, helping it produce a response that is both contextually relevant and factually accurate.
Here’s a simplified example in Python using a pseudo RAG implementation:
def retrieve_information(query):
# Simulate retrieving relevant data from an external source
external_data = {
"query1": "Relevant information 1",
"query2": "Relevant information 2"
}
return external_data.get(query, "Default information")
def generate_response(query):
# Retrieve data using the RAG method
retrieved_data = retrieve_information(query)
# Integrate retrieved data into the response
response = f"Based on your query, here is what I found: {retrieved_data}"
return response
# Example usage
query = "query1"
response = generate_response(query)
print(response)
In this example, the retrieve_information function simulates fetching data from an external source, which then integrates into the response generated by the generate_response function.
Methods of RAG: Passive and Active
There are two primary methods of RAG: passive and active.
Passive RAG:
Passive RAG relies on existing data repositories, using them to retrieve information as needed during the generation process. The key characteristic of this method is that it does not modify or update the data source in real time.
For a query, the model pulls relevant information from a static database or knowledge base that has been pre-compiled. The retrieved data then integrates into the response generated by the model. Some of its advantages are:
Stability: Since the data source remains unchanged, passive RAG offers a consistent and stable reference for information retrieval, which is beneficial in scenarios where consistency is critical.
Simplicity: With no need for continuous updates, passive RAG is easier to implement and manage, making it suitable for applications where the data does not frequently change.
Efficiency: By relying on pre-existing, static data, passive RAG can be more efficient in terms of computational resources, as it does not require the overhead of updating or refining the data.
Active RAG:
Active RAG involves a more dynamic approach, where the data source is continuously updated and refined based on new inputs and information. This ensures that the model has access to the most current and relevant data.
As the model receives new inputs or queries, it not only retrieves information but also updates the data repository with new data, refining the knowledge base over time. This ongoing refinement allows the model to adapt to changes and incorporate fresh information into its responses. Some advantages are:
Adaptability: Active RAG is highly adaptable, making it ideal for environments where information is constantly changing. The model can adjust to new data, trends, or discoveries in real time, ensuring that the responses are always up-to-date.
Relevance: By continuously updating the data source, active RAG ensures that the information retrieved is as relevant as possible, which is particularly valuable in fields like medical research, legal analysis, or customer support.
Scalability: Active RAG can scale effectively with growing and evolving datasets, allowing the model to handle increasingly complex queries with accurate and timely information.
Each method of RAG has its own strengths, and the choice between passive and active RAG depends on the specific needs of the application—whether you require the consistency of static data or the adaptability to dynamic changes.
Internal Process: Query, Retrieve, Integrate, Generate
The summary of the internal workings of RAG in four key steps:
Query: The model formulates a query based on the user’s input.
Retrieve: It then retrieves relevant data from an external source.
Integrate: The retrieved information integrates into the model’s existing knowledge.
Generate: Finally, the model generates a response that combines the retrieved data with its internal processing capabilities.
This process allows RAG to produce outputs that are not only generated by the model but are also enriched with precise, real-world data, making it particularly effective for complex applications like legal research or technical support.
Interested in learning more about how to build RAG applications? Explore how to build RAG applications, ensuring safe and reliable genAI.
Next, we’ll explore how fine-tuning works, detailing the steps involved and the specific scenarios where this technique excels.
How Fine-Tuning Works
Fine-tuning is a critical technique in the AI learning process, allowing you to adapt pre-trained models to specific tasks by adjusting their parameters based on task-specific data. Unlike RAG, which relies on external data sources during generation, fine-tuning hones the internal parameters of a model, making it more specialized and accurate for a given task. Understanding the differences between RAG and fine-tuning is essential for selecting the best approach for your AI projects.
Steps Involved in Fine-Tuning
The fine-tuning process involves several steps that refine the model’s ability to perform a specific task. Here’s a breakdown of these steps:
Pre-train: Start with a pre-trained model on a large dataset. This model already understands a wide range of general knowledge, which forms the foundation for fine-tuning.
Task-Specific Data Preparation: Gather and prepare the data that is relevant to the specific task you want the model to perform. This step is crucial as the quality and relevance of this data will directly impact the effectiveness of the fine-tuning process.
Reprocess: Reprocess the pre-trained model’s layers to accommodate the new, task-specific data. It involves resetting certain weights and biases to ensure the model can learn the nuances of the new task.
Adjust Layers: Modify the model’s architecture, if necessary, to better suit the task. It could involve adding or removing layers or changing the activation functions to optimize performance.
Configure Model: Set up the model’s parameters, such as learning rate and batch size, to ensure optimal training. These settings play a crucial role in how quickly and effectively the model learns from the new data.
Train: Train the model on the task-specific data, allowing it to learn and adapt. This step typically involves multiple iterations, where the model’s parameters are adjusted based on the data it processes.
Evaluate: After training, evaluate the model’s performance on a validation set to ensure it has learned the task effectively. This step helps identify any issues or areas where further fine-tuning may be needed.
Iterate: Fine-tuning is often an iterative process. Based on the evaluation results, you may need to adjust the data, reconfigure the model, or train further to achieve the desired performance.
When comparing RAG and fine-tuning, it’s clear that fine-tuning requires less reliance on external data during inference but demands careful preparation and iteration to achieve optimal results.
Here’s a simplified code example to illustrate the fine-tuning process:
from transformers import Trainer, TrainingArguments
# Load pre-trained model
model = AutoModelForSequenceClassification.from_pretrained("bert-base-uncased")
# Load task-specific dataset
train_dataset = load_dataset("my_dataset", split="train")
# Define training arguments
training_args = TrainingArguments(
output_dir="./results",
evaluation_strategy="epoch",
learning_rate=2e-5,
per_device_train_batch_size=16,
num_train_epochs=3
)
# Initialize Trainer
trainer = Trainer(
model=model,
args=training_args,
train_dataset=train_dataset,
eval_dataset=val_dataset
)
# Train the model
trainer.train()
This code snippet fine-tunes a pre-trained model using task-specific data, highlighting how the process is more about adjusting and refining an existing model rather than relying on external information retrieval.
Understanding the fine-tuning process and how it contrasts with RAG is key to choosing the right technique for your AI projects. Fine-tuning offers a more focused approach, ideal for scenarios where specialized, high-accuracy models are required.
Want to know more about specific steps involved in optimizing AI models? Explore how Raga AI fine-tunes AI models for specific tasks with precision and efficiency.
Next, we’ll explore the advantages and limitations of RAG, giving you a clearer picture of when to use each technique.
Advantages and Limitations of RAG
Retrieval-Augmented Generation (RAG) is a distinctive approach in AI that merges retrieval-based methods with generative models. This technique offers several benefits but also comes with certain challenges. Understanding these can help you decide when to choose RAG over fine-tuning your AI projects.
Advantages of RAG
Improved Accuracy: RAG enhances accuracy by pulling in relevant, real-time information from external sources. This integration allows the model to generate responses that are not only contextually appropriate but also factually correct.
Reduced Hallucinations: One of RAG's key strengths is its ability to minimize hallucinations, where a model might otherwise produce convincing but incorrect information. By grounding responses in external data, RAG ensures that the information provided is reliable and based on actual data.
Adaptability to New Data: RAG is highly adaptable to new information because it retrieves data in real time. Unlike fine-tuning, which requires retraining the model with new data, RAG can incorporate fresh data on the fly, making it ideal for environments where information changes frequently.
Cost-Effectiveness: Since RAG utilizes existing data sources, it often requires less training data and fewer computational resources. It makes RAG a more cost-effective choice for projects that need to scale and access large volumes of information without the expense of retraining models frequently.
Limitations of RAG
Higher Latency: A significant drawback of RAG is the potential for increased latency. Because the model needs to fetch information from external sources before generating a response, this process can be slower compared to fine-tuned models that rely solely on internal data. It can be a concern in applications where speed is crucial.
Complex Architecture Requirements: Implementing RAG can require a more complex setup, especially when dealing with large-scale retrieval systems. Managing external databases and ensuring efficient data retrieval adds layers of complexity, making RAG more challenging to implement compared to fine-tuning.
When comparing RAG to fine-tuning, RAG excels in accuracy, adaptability, and cost-efficiency, particularly in scenarios where up-to-date information is essential. However, it’s important to consider the trade-offs, such as potential latency issues and the need for a more complex architecture, which might not be suitable for every application.
To learn more about the benefits of using RAG in AI systems, learn how Raga AI enhances AI performance by integrating retrieval-based techniques.
Next, we’ll explore the advantages and limitations of fine-tuning, giving you a deeper understanding of when each method is most appropriate.
Advantages and Limitations of Fine-Tuning
Fine-tuning is a powerful method in AI that allows you to refine a pre-trained model for specific tasks by adjusting its parameters. This approach is particularly effective in scenarios where precise, task-specific performance is required. However, like any technique, fine-tuning comes with its own set of benefits and drawbacks.
Advantages of Fine-Tuning
Less Training Data Required: Fine-tuning is efficient because it builds on an already pre-trained model. It means you need less task-specific data to achieve high accuracy, making it a practical choice when data is limited.
Improved Accuracy: Fine-tuning can significantly enhance the accuracy of a model for a specific task. By fine-tuning the model on a smaller, focused dataset, you can achieve a high level of precision that is well-suited to the particular needs of the application.
Increased Robustness: Through fine-tuning, the model becomes more robust in handling the nuances of the tailored task. It allows the model to perform better on specific, narrow tasks where general pre-trained models might struggle.
Limitations of Fine-Tuning
Potential for Forgetting: One of the main challenges of fine-tuning is the risk of the model "forgetting" the broader knowledge it gained during pre-training. It can occur when the model becomes too specialized in the new task, losing its ability to generalize.
Dependence on Training Data: The effectiveness of fine-tuning depends on the quality and relevance of the task-specific data. If the data is biased or incomplete, the model’s performance will suffer, leading to less reliable outcomes.
Lack of External Knowledge: Unlike RAG, which can pull in real-time information from external sources, fine-tuning relies solely on internal data. This can limit the model's ability to handle new or evolving information, making it less adaptable to changes.
When comparing RAG vs. fine-tuning, fine-tuning shines in tasks that require a deep focus on specific data, providing high accuracy and robustness with less training data. However, it also requires careful consideration of potential drawbacks like forgetting, data dependency, and the absence of external knowledge sources.
To learn more about the process of refining AI models for specific tasks, discover how Raga AI fine-tunes models to achieve high accuracy and reliability.
Next, we’ll dive into a direct comparison of RAG and fine-tuning, examining the factors that influence the choice between these two powerful AI techniques.
Comparison Factors: RAG vs Fine-Tuning
When deciding between Retrieval-Augmented Generation (RAG) and fine-tuning for your AI projects, it’s essential to consider several key factors. Each technique has its strengths and weaknesses, and understanding these can help you choose the best approach based on your specific needs.
External Data Access and Integration
RAG excels in scenarios where real-time access to external data is crucial. By retrieving information from databases or knowledge sources during the generation process, RAG ensures that the model’s responses are both accurate and up-to-date. In contrast, fine-tuning does not access external data during inference; it relies solely on its training data. This makes RAG the better choice when you need the model to handle dynamic or evolving information.
Model Behavior Adjustment
With fine-tuning, you can precisely adjust the behavior of your model by retraining it on task-specific data. This allows for a high degree of customization, making fine-tuning ideal for applications that require the model to perform specific, narrow tasks with great accuracy. RAG, on the other hand, adjusts behavior dynamically during inference by integrating retrieved data, offering flexibility without needing to retrain the model.
Hallucination Suppression
One of the significant advantages of RAG is its ability to suppress hallucinations by grounding responses in external data. This makes RAG particularly useful in applications where accuracy is critical, such as legal or medical fields. Fine-tuning, while capable of improving accuracy, can sometimes lead to overfitting, where the model might produce confident but incorrect outputs if not carefully managed.
Availability of Labeled Training Data
Fine-tuning requires a well-curated, labeled dataset for training. The quality of this data directly impacts the model’s performance. If such data is scarce or costly to obtain, RAG might be a more practical option since it can work with existing external sources, reducing the dependency on large, labeled datasets.
Handling Dynamic vs Static Data
RAG is highly effective in environments where data is dynamic and constantly changing. It allows the model to incorporate the latest information during inference, making it adaptable to new trends or facts. Fine-tuning is better suited for static data scenarios where the task does not require continuous updates, as it involves retraining the model with new data to reflect changes.
Transparency and Interpretability
When it comes to transparency, fine-tuning often provides clearer insights into how a model arrives at its decisions, as the adjustments are made directly within the model’s parameters. RAG, while powerful, can sometimes be less transparent due to the dynamic nature of data retrieval, making it harder to trace the exact source of information used in a response.
Computational Requirements and Scalability
RAG can be more computationally intensive, especially when dealing with large external databases, leading to higher resource consumption and potentially longer response times. Fine-tuning generally has lower runtime requirements since the model does not need to query external data during inference, making it more scalable for high-volume tasks.
Speed and Latency
In terms of speed, fine-tuning often outperforms RAG because it doesn’t involve the additional step of retrieving external data. This makes fine-tuning a better option for applications where low latency is critical. RAG, while potentially slower, offers the advantage of generating more informed and accurate responses by incorporating real-time data.
In summary, the choice between RAG vs. fine-tuning depends on the specific requirements of your project. RAG offers dynamic data integration and reduces hallucinations, making it ideal for complex, information-rich environments. Fine-tuning provides precise behavior adjustment and faster response times, suited for tasks where accuracy and speed are paramount.
To learn more about the differences between various AI model training techniques, explore how Raga AI evaluates and compares AI models to optimize performance and reliability.
Next, we’ll explore real-world use cases for RAG, demonstrating how this technique can be applied to enhance AI applications in various fields.
Use Cases for RAG
Retrieval-Augmented Generation (RAG) is particularly effective in scenarios where integrating real-time, external data into AI-generated responses is crucial. This approach allows AI systems to produce more accurate, contextually relevant, and up-to-date information. Here are some key use cases where RAG shines:
Chatbots and AI Technical Support
RAG is highly beneficial in developing advanced chatbots and AI-driven technical support systems. By retrieving the latest information from knowledge bases or databases, these systems can provide users with precise, relevant answers. For example, in technical support, RAG can pull in real-time troubleshooting steps from a database, ensuring that users receive the most accurate and helpful guidance possible.
Language Translation and Education Tools
In the field of language translation and educational tools, RAG can enhance the learning experience by integrating up-to-date language nuances or educational content into the generated responses. This is particularly useful in applications where the AI needs to stay current with evolving language trends or educational standards, providing learners with the most relevant information.
Medical Research and Diagnosis Augmentation
RAG can play a critical role in medical research and diagnosis, where access to the latest studies, clinical trials, or patient data is essential. By retrieving and integrating the most recent medical information, RAG-powered AI systems can assist healthcare professionals in making informed decisions, improving diagnosis accuracy, and suggesting up-to-date treatment options.
Legal Research and Review Tasks
In legal research, where precision and up-to-date information are paramount, RAG enables AI systems to retrieve relevant case law, statutes, and legal opinions from extensive databases. This capability allows legal professionals to conduct thorough research quickly, ensuring that they are referencing the most current and applicable legal precedents in their work.
Looking to apply RAG in your projects? Discover how Raga AI utilizes retrieval-based techniques to enhance the accuracy and reliability of AI systems.
Next, we’ll explore the use cases for fine-tuning, showing applications of this technique in different scenarios where deep task-specific learning is required.
Use Cases for Fine-Tuning
Fine-tuning is a versatile technique that excels in scenarios where AI models need to be adapted to perform specific tasks with high accuracy. By refining a pre-trained model using task-specific data, fine-tuning ensures that the AI is nuanced to the task at hand. Here are some key use cases where fine-tuning is particularly effective:
Sentiment Analysis
In sentiment analysis, where understanding the emotional tone of the text is critical, fine-tuning allows you to train the AI models on specific datasets that reflect the sentiment patterns relevant to the application. For example, a model fine-tuned on customer reviews can accurately determine whether a review is positive, negative, or neutral, helping businesses understand customer feedback more precisely.
Named-Entity Recognition (NER)
Named-entity recognition involves identifying and classifying key entities within a text, such as names, dates, or locations. Fine-tuning is essential in this area because it enables the AI model to learn the specific entity types that are important for the task. Whether it’s for legal document analysis or extracting key information from medical records, fine-tuning ensures that the model can recognize and categorize entities with high accuracy.
Personalized Content Recommendation
In personalized content recommendation systems, fine-tuning helps tailor the AI’s recommendations to individual user preferences. By training the model on data that reflects user behavior and preferences, fine-tuning ensures that the content suggested is highly relevant and engaging. This approach is widely used in streaming services, e-commerce platforms, and social media, where personalized recommendations are crucial for user satisfaction.
Specific Domain Summarization
Fine-tuning is also highly effective in domain-specific summarization tasks, where the goal is to generate concise summaries of lengthy documents within a particular field. For example, a model fine-tuned on legal documents can summarize complex contracts, while a model fine-tuned on scientific papers can distill key findings into a summary. This specialization allows the AI to produce summaries that are not only accurate but also aligned with the specific requirements of the domain.
Ready to see how fine-tuning can enhance your AI applications? Learn how Raga AI fine-tunes models to achieve high accuracy and reliability for specific tasks.
Next, we’ll explore how combining RAG and fine-tuning can utilize the strengths of both techniques, offering even more powerful AI solutions for complex applications.
Combining RAG and Fine-Tuning
While Retrieval-Augmented Generation (RAG) and fine-tuning each offer distinct advantages, combining these two techniques can reveal even greater potential in AI applications. By leveraging the strengths of both methods, you can create AI systems that are not only highly accurate but also adaptable and resource-efficient, making them ideal for complex, real-world scenarios.
Hybrid Approaches for Both Techniques
Combining RAG and fine-tuning allows you to harness the dynamic data integration of RAG alongside the specialized accuracy of fine-tuning. For instance, a model can be fine-tuned on task-specific data to master a particular domain, ensuring it performs with high precision. At the same time, you can integrate RAG to pull in real-time data during inference, allowing the model to adapt to new information or trends that weren’t available during training. This hybrid approach ensures that your AI model remains both deeply knowledgeable and highly responsive to changes.
Example: Customer Support Automation Using Both Methods
Consider a customer support chatbot that needs to handle a wide range of inquiries, from frequently asked questions to complex technical issues. By fine-tuning the model on a dataset of common customer queries, the chatbot can provide accurate, task-specific responses. However, when a new or unusual question arises, RAG can be employed to retrieve the latest information from a knowledge base or support database, ensuring the chatbot delivers a relevant and up-to-date answer. This combination of fine-tuning for accuracy and RAG for adaptability creates a robust customer support system capable of handling both routine and novel queries effectively.
Curious about how to implement a hybrid AI approach? Discover how Raga AI combines advanced techniques to create powerful, adaptable AI systems.
Looking ahead, we’ll explore future trends and recommendations, including the potential for hybrid models, advancements in hardware, and practical advice for choosing the best AI techniques for your needs.
Future Trends and Recommendations
As AI technology continues to evolve, you can expect the integration of techniques like Retrieval-Augmented Generation (RAG) and fine-tuning to become more sophisticated. The future holds exciting possibilities for hybrid models that blend the strengths of both approaches, creating AI systems that are not only highly accurate but also incredibly adaptable to new data and changing environments.
Potential for Hybrid Models
The ongoing development of hybrid models that combine RAG and fine-tuning is likely to reshape how AI performs across industries. These models will benefit from the precision of fine-tuning for specific tasks while using RAG to integrate real-time information, ensuring that AI systems can respond dynamically to new challenges. As the demand for more versatile and intelligent AI solutions grows, hybrid models will play a critical role in meeting these needs.
Hardware Advancements
Advancements in AI hardware can also reduce the computational limitations that currently restrict the use of complex models. With the development of more powerful processors and optimized algorithms, running sophisticated hybrid models will become more feasible even in resource-constrained environments. This will open up new opportunities for deploying advanced AI systems in areas like healthcare, finance, and education, where computational efficiency is crucial.
Increasing Data Improving Methods
As more data becomes available, both RAG and fine-tuning techniques will continue to improve in effectiveness. The ability to access vast amounts of data in real time will enhance the accuracy and relevance of AI models, making them more capable of delivering actionable insights. Organizations should focus on building robust data pipelines that feed their AI systems with high-quality, diverse datasets to maximize the potential of these techniques.
Practical Recommendations
When deciding between RAG and fine-tuning—or whether to combine them—it’s important to consider the specific needs of your project. For tasks that require real-time data integration and adaptability, RAG is an excellent choice. Fine-tuning, on the other hand, is ideal for scenarios where precise, task-specific accuracy is critical. In many cases, a hybrid approach may offer the best of both worlds, providing a balance between accuracy and adaptability.
At Raga AI, we are at the forefront of these exciting developments. Our expertise in combining RAG, fine-tuning, and other advanced techniques enables us to create AI systems that are not only accurate but also adaptable and efficient. Whether you’re looking to enhance your existing AI capabilities or explore new possibilities, Raga AI provides the tools and insights you need to stay ahead in the rapidly evolving AI market. Ready to transform your AI systems? Try Raga AI today!
In the rapidly evolving world of AI, choosing the right learning technique can make all the difference in the performance and scalability of your models. Two of the most powerful methods in this space are Retrieval-Augmented Generation (RAG) and fine-tuning. But with each technique offering distinct advantages, how do you decide which is best for your application?
Understanding the core principles and how they impact your AI system is crucial in making an informed decision. The choice between these methods not only affects the accuracy and efficiency of your AI but also determines how scalable and adaptable your solutions will be in real-world scenarios.
Let’s dive deeper into each approach to see how they stack up against one another.
Understanding RAG (Retrieval-Augmented Generation)
Retrieval-Augmented Generation (RAG) is a powerful AI technique that combines the strengths of retrieval-based methods with generative models. Essentially, RAG enables models to enhance their responses by fetching relevant information from external data sources before generating an output. This approach not only boosts accuracy but also helps in reducing hallucinations, where the model might otherwise produce plausible but incorrect information.
How RAG Works
RAG operates by breaking down the generation process into two main steps: retrieval and generation. First, the model queries an external database or knowledge source to retrieve the most relevant pieces of information. Next, this retrieved data is integrated into the model’s generative process, helping it produce a response that is both contextually relevant and factually accurate.
Here’s a simplified example in Python using a pseudo RAG implementation:
def retrieve_information(query):
# Simulate retrieving relevant data from an external source
external_data = {
"query1": "Relevant information 1",
"query2": "Relevant information 2"
}
return external_data.get(query, "Default information")
def generate_response(query):
# Retrieve data using the RAG method
retrieved_data = retrieve_information(query)
# Integrate retrieved data into the response
response = f"Based on your query, here is what I found: {retrieved_data}"
return response
# Example usage
query = "query1"
response = generate_response(query)
print(response)
In this example, the retrieve_information function simulates fetching data from an external source, which then integrates into the response generated by the generate_response function.
Methods of RAG: Passive and Active
There are two primary methods of RAG: passive and active.
Passive RAG:
Passive RAG relies on existing data repositories, using them to retrieve information as needed during the generation process. The key characteristic of this method is that it does not modify or update the data source in real time.
For a query, the model pulls relevant information from a static database or knowledge base that has been pre-compiled. The retrieved data then integrates into the response generated by the model. Some of its advantages are:
Stability: Since the data source remains unchanged, passive RAG offers a consistent and stable reference for information retrieval, which is beneficial in scenarios where consistency is critical.
Simplicity: With no need for continuous updates, passive RAG is easier to implement and manage, making it suitable for applications where the data does not frequently change.
Efficiency: By relying on pre-existing, static data, passive RAG can be more efficient in terms of computational resources, as it does not require the overhead of updating or refining the data.
Active RAG:
Active RAG involves a more dynamic approach, where the data source is continuously updated and refined based on new inputs and information. This ensures that the model has access to the most current and relevant data.
As the model receives new inputs or queries, it not only retrieves information but also updates the data repository with new data, refining the knowledge base over time. This ongoing refinement allows the model to adapt to changes and incorporate fresh information into its responses. Some advantages are:
Adaptability: Active RAG is highly adaptable, making it ideal for environments where information is constantly changing. The model can adjust to new data, trends, or discoveries in real time, ensuring that the responses are always up-to-date.
Relevance: By continuously updating the data source, active RAG ensures that the information retrieved is as relevant as possible, which is particularly valuable in fields like medical research, legal analysis, or customer support.
Scalability: Active RAG can scale effectively with growing and evolving datasets, allowing the model to handle increasingly complex queries with accurate and timely information.
Each method of RAG has its own strengths, and the choice between passive and active RAG depends on the specific needs of the application—whether you require the consistency of static data or the adaptability to dynamic changes.
Internal Process: Query, Retrieve, Integrate, Generate
The summary of the internal workings of RAG in four key steps:
Query: The model formulates a query based on the user’s input.
Retrieve: It then retrieves relevant data from an external source.
Integrate: The retrieved information integrates into the model’s existing knowledge.
Generate: Finally, the model generates a response that combines the retrieved data with its internal processing capabilities.
This process allows RAG to produce outputs that are not only generated by the model but are also enriched with precise, real-world data, making it particularly effective for complex applications like legal research or technical support.
Interested in learning more about how to build RAG applications? Explore how to build RAG applications, ensuring safe and reliable genAI.
Next, we’ll explore how fine-tuning works, detailing the steps involved and the specific scenarios where this technique excels.
How Fine-Tuning Works
Fine-tuning is a critical technique in the AI learning process, allowing you to adapt pre-trained models to specific tasks by adjusting their parameters based on task-specific data. Unlike RAG, which relies on external data sources during generation, fine-tuning hones the internal parameters of a model, making it more specialized and accurate for a given task. Understanding the differences between RAG and fine-tuning is essential for selecting the best approach for your AI projects.
Steps Involved in Fine-Tuning
The fine-tuning process involves several steps that refine the model’s ability to perform a specific task. Here’s a breakdown of these steps:
Pre-train: Start with a pre-trained model on a large dataset. This model already understands a wide range of general knowledge, which forms the foundation for fine-tuning.
Task-Specific Data Preparation: Gather and prepare the data that is relevant to the specific task you want the model to perform. This step is crucial as the quality and relevance of this data will directly impact the effectiveness of the fine-tuning process.
Reprocess: Reprocess the pre-trained model’s layers to accommodate the new, task-specific data. It involves resetting certain weights and biases to ensure the model can learn the nuances of the new task.
Adjust Layers: Modify the model’s architecture, if necessary, to better suit the task. It could involve adding or removing layers or changing the activation functions to optimize performance.
Configure Model: Set up the model’s parameters, such as learning rate and batch size, to ensure optimal training. These settings play a crucial role in how quickly and effectively the model learns from the new data.
Train: Train the model on the task-specific data, allowing it to learn and adapt. This step typically involves multiple iterations, where the model’s parameters are adjusted based on the data it processes.
Evaluate: After training, evaluate the model’s performance on a validation set to ensure it has learned the task effectively. This step helps identify any issues or areas where further fine-tuning may be needed.
Iterate: Fine-tuning is often an iterative process. Based on the evaluation results, you may need to adjust the data, reconfigure the model, or train further to achieve the desired performance.
When comparing RAG and fine-tuning, it’s clear that fine-tuning requires less reliance on external data during inference but demands careful preparation and iteration to achieve optimal results.
Here’s a simplified code example to illustrate the fine-tuning process:
from transformers import Trainer, TrainingArguments
# Load pre-trained model
model = AutoModelForSequenceClassification.from_pretrained("bert-base-uncased")
# Load task-specific dataset
train_dataset = load_dataset("my_dataset", split="train")
# Define training arguments
training_args = TrainingArguments(
output_dir="./results",
evaluation_strategy="epoch",
learning_rate=2e-5,
per_device_train_batch_size=16,
num_train_epochs=3
)
# Initialize Trainer
trainer = Trainer(
model=model,
args=training_args,
train_dataset=train_dataset,
eval_dataset=val_dataset
)
# Train the model
trainer.train()
This code snippet fine-tunes a pre-trained model using task-specific data, highlighting how the process is more about adjusting and refining an existing model rather than relying on external information retrieval.
Understanding the fine-tuning process and how it contrasts with RAG is key to choosing the right technique for your AI projects. Fine-tuning offers a more focused approach, ideal for scenarios where specialized, high-accuracy models are required.
Want to know more about specific steps involved in optimizing AI models? Explore how Raga AI fine-tunes AI models for specific tasks with precision and efficiency.
Next, we’ll explore the advantages and limitations of RAG, giving you a clearer picture of when to use each technique.
Advantages and Limitations of RAG
Retrieval-Augmented Generation (RAG) is a distinctive approach in AI that merges retrieval-based methods with generative models. This technique offers several benefits but also comes with certain challenges. Understanding these can help you decide when to choose RAG over fine-tuning your AI projects.
Advantages of RAG
Improved Accuracy: RAG enhances accuracy by pulling in relevant, real-time information from external sources. This integration allows the model to generate responses that are not only contextually appropriate but also factually correct.
Reduced Hallucinations: One of RAG's key strengths is its ability to minimize hallucinations, where a model might otherwise produce convincing but incorrect information. By grounding responses in external data, RAG ensures that the information provided is reliable and based on actual data.
Adaptability to New Data: RAG is highly adaptable to new information because it retrieves data in real time. Unlike fine-tuning, which requires retraining the model with new data, RAG can incorporate fresh data on the fly, making it ideal for environments where information changes frequently.
Cost-Effectiveness: Since RAG utilizes existing data sources, it often requires less training data and fewer computational resources. It makes RAG a more cost-effective choice for projects that need to scale and access large volumes of information without the expense of retraining models frequently.
Limitations of RAG
Higher Latency: A significant drawback of RAG is the potential for increased latency. Because the model needs to fetch information from external sources before generating a response, this process can be slower compared to fine-tuned models that rely solely on internal data. It can be a concern in applications where speed is crucial.
Complex Architecture Requirements: Implementing RAG can require a more complex setup, especially when dealing with large-scale retrieval systems. Managing external databases and ensuring efficient data retrieval adds layers of complexity, making RAG more challenging to implement compared to fine-tuning.
When comparing RAG to fine-tuning, RAG excels in accuracy, adaptability, and cost-efficiency, particularly in scenarios where up-to-date information is essential. However, it’s important to consider the trade-offs, such as potential latency issues and the need for a more complex architecture, which might not be suitable for every application.
To learn more about the benefits of using RAG in AI systems, learn how Raga AI enhances AI performance by integrating retrieval-based techniques.
Next, we’ll explore the advantages and limitations of fine-tuning, giving you a deeper understanding of when each method is most appropriate.
Advantages and Limitations of Fine-Tuning
Fine-tuning is a powerful method in AI that allows you to refine a pre-trained model for specific tasks by adjusting its parameters. This approach is particularly effective in scenarios where precise, task-specific performance is required. However, like any technique, fine-tuning comes with its own set of benefits and drawbacks.
Advantages of Fine-Tuning
Less Training Data Required: Fine-tuning is efficient because it builds on an already pre-trained model. It means you need less task-specific data to achieve high accuracy, making it a practical choice when data is limited.
Improved Accuracy: Fine-tuning can significantly enhance the accuracy of a model for a specific task. By fine-tuning the model on a smaller, focused dataset, you can achieve a high level of precision that is well-suited to the particular needs of the application.
Increased Robustness: Through fine-tuning, the model becomes more robust in handling the nuances of the tailored task. It allows the model to perform better on specific, narrow tasks where general pre-trained models might struggle.
Limitations of Fine-Tuning
Potential for Forgetting: One of the main challenges of fine-tuning is the risk of the model "forgetting" the broader knowledge it gained during pre-training. It can occur when the model becomes too specialized in the new task, losing its ability to generalize.
Dependence on Training Data: The effectiveness of fine-tuning depends on the quality and relevance of the task-specific data. If the data is biased or incomplete, the model’s performance will suffer, leading to less reliable outcomes.
Lack of External Knowledge: Unlike RAG, which can pull in real-time information from external sources, fine-tuning relies solely on internal data. This can limit the model's ability to handle new or evolving information, making it less adaptable to changes.
When comparing RAG vs. fine-tuning, fine-tuning shines in tasks that require a deep focus on specific data, providing high accuracy and robustness with less training data. However, it also requires careful consideration of potential drawbacks like forgetting, data dependency, and the absence of external knowledge sources.
To learn more about the process of refining AI models for specific tasks, discover how Raga AI fine-tunes models to achieve high accuracy and reliability.
Next, we’ll dive into a direct comparison of RAG and fine-tuning, examining the factors that influence the choice between these two powerful AI techniques.
Comparison Factors: RAG vs Fine-Tuning
When deciding between Retrieval-Augmented Generation (RAG) and fine-tuning for your AI projects, it’s essential to consider several key factors. Each technique has its strengths and weaknesses, and understanding these can help you choose the best approach based on your specific needs.
External Data Access and Integration
RAG excels in scenarios where real-time access to external data is crucial. By retrieving information from databases or knowledge sources during the generation process, RAG ensures that the model’s responses are both accurate and up-to-date. In contrast, fine-tuning does not access external data during inference; it relies solely on its training data. This makes RAG the better choice when you need the model to handle dynamic or evolving information.
Model Behavior Adjustment
With fine-tuning, you can precisely adjust the behavior of your model by retraining it on task-specific data. This allows for a high degree of customization, making fine-tuning ideal for applications that require the model to perform specific, narrow tasks with great accuracy. RAG, on the other hand, adjusts behavior dynamically during inference by integrating retrieved data, offering flexibility without needing to retrain the model.
Hallucination Suppression
One of the significant advantages of RAG is its ability to suppress hallucinations by grounding responses in external data. This makes RAG particularly useful in applications where accuracy is critical, such as legal or medical fields. Fine-tuning, while capable of improving accuracy, can sometimes lead to overfitting, where the model might produce confident but incorrect outputs if not carefully managed.
Availability of Labeled Training Data
Fine-tuning requires a well-curated, labeled dataset for training. The quality of this data directly impacts the model’s performance. If such data is scarce or costly to obtain, RAG might be a more practical option since it can work with existing external sources, reducing the dependency on large, labeled datasets.
Handling Dynamic vs Static Data
RAG is highly effective in environments where data is dynamic and constantly changing. It allows the model to incorporate the latest information during inference, making it adaptable to new trends or facts. Fine-tuning is better suited for static data scenarios where the task does not require continuous updates, as it involves retraining the model with new data to reflect changes.
Transparency and Interpretability
When it comes to transparency, fine-tuning often provides clearer insights into how a model arrives at its decisions, as the adjustments are made directly within the model’s parameters. RAG, while powerful, can sometimes be less transparent due to the dynamic nature of data retrieval, making it harder to trace the exact source of information used in a response.
Computational Requirements and Scalability
RAG can be more computationally intensive, especially when dealing with large external databases, leading to higher resource consumption and potentially longer response times. Fine-tuning generally has lower runtime requirements since the model does not need to query external data during inference, making it more scalable for high-volume tasks.
Speed and Latency
In terms of speed, fine-tuning often outperforms RAG because it doesn’t involve the additional step of retrieving external data. This makes fine-tuning a better option for applications where low latency is critical. RAG, while potentially slower, offers the advantage of generating more informed and accurate responses by incorporating real-time data.
In summary, the choice between RAG vs. fine-tuning depends on the specific requirements of your project. RAG offers dynamic data integration and reduces hallucinations, making it ideal for complex, information-rich environments. Fine-tuning provides precise behavior adjustment and faster response times, suited for tasks where accuracy and speed are paramount.
To learn more about the differences between various AI model training techniques, explore how Raga AI evaluates and compares AI models to optimize performance and reliability.
Next, we’ll explore real-world use cases for RAG, demonstrating how this technique can be applied to enhance AI applications in various fields.
Use Cases for RAG
Retrieval-Augmented Generation (RAG) is particularly effective in scenarios where integrating real-time, external data into AI-generated responses is crucial. This approach allows AI systems to produce more accurate, contextually relevant, and up-to-date information. Here are some key use cases where RAG shines:
Chatbots and AI Technical Support
RAG is highly beneficial in developing advanced chatbots and AI-driven technical support systems. By retrieving the latest information from knowledge bases or databases, these systems can provide users with precise, relevant answers. For example, in technical support, RAG can pull in real-time troubleshooting steps from a database, ensuring that users receive the most accurate and helpful guidance possible.
Language Translation and Education Tools
In the field of language translation and educational tools, RAG can enhance the learning experience by integrating up-to-date language nuances or educational content into the generated responses. This is particularly useful in applications where the AI needs to stay current with evolving language trends or educational standards, providing learners with the most relevant information.
Medical Research and Diagnosis Augmentation
RAG can play a critical role in medical research and diagnosis, where access to the latest studies, clinical trials, or patient data is essential. By retrieving and integrating the most recent medical information, RAG-powered AI systems can assist healthcare professionals in making informed decisions, improving diagnosis accuracy, and suggesting up-to-date treatment options.
Legal Research and Review Tasks
In legal research, where precision and up-to-date information are paramount, RAG enables AI systems to retrieve relevant case law, statutes, and legal opinions from extensive databases. This capability allows legal professionals to conduct thorough research quickly, ensuring that they are referencing the most current and applicable legal precedents in their work.
Looking to apply RAG in your projects? Discover how Raga AI utilizes retrieval-based techniques to enhance the accuracy and reliability of AI systems.
Next, we’ll explore the use cases for fine-tuning, showing applications of this technique in different scenarios where deep task-specific learning is required.
Use Cases for Fine-Tuning
Fine-tuning is a versatile technique that excels in scenarios where AI models need to be adapted to perform specific tasks with high accuracy. By refining a pre-trained model using task-specific data, fine-tuning ensures that the AI is nuanced to the task at hand. Here are some key use cases where fine-tuning is particularly effective:
Sentiment Analysis
In sentiment analysis, where understanding the emotional tone of the text is critical, fine-tuning allows you to train the AI models on specific datasets that reflect the sentiment patterns relevant to the application. For example, a model fine-tuned on customer reviews can accurately determine whether a review is positive, negative, or neutral, helping businesses understand customer feedback more precisely.
Named-Entity Recognition (NER)
Named-entity recognition involves identifying and classifying key entities within a text, such as names, dates, or locations. Fine-tuning is essential in this area because it enables the AI model to learn the specific entity types that are important for the task. Whether it’s for legal document analysis or extracting key information from medical records, fine-tuning ensures that the model can recognize and categorize entities with high accuracy.
Personalized Content Recommendation
In personalized content recommendation systems, fine-tuning helps tailor the AI’s recommendations to individual user preferences. By training the model on data that reflects user behavior and preferences, fine-tuning ensures that the content suggested is highly relevant and engaging. This approach is widely used in streaming services, e-commerce platforms, and social media, where personalized recommendations are crucial for user satisfaction.
Specific Domain Summarization
Fine-tuning is also highly effective in domain-specific summarization tasks, where the goal is to generate concise summaries of lengthy documents within a particular field. For example, a model fine-tuned on legal documents can summarize complex contracts, while a model fine-tuned on scientific papers can distill key findings into a summary. This specialization allows the AI to produce summaries that are not only accurate but also aligned with the specific requirements of the domain.
Ready to see how fine-tuning can enhance your AI applications? Learn how Raga AI fine-tunes models to achieve high accuracy and reliability for specific tasks.
Next, we’ll explore how combining RAG and fine-tuning can utilize the strengths of both techniques, offering even more powerful AI solutions for complex applications.
Combining RAG and Fine-Tuning
While Retrieval-Augmented Generation (RAG) and fine-tuning each offer distinct advantages, combining these two techniques can reveal even greater potential in AI applications. By leveraging the strengths of both methods, you can create AI systems that are not only highly accurate but also adaptable and resource-efficient, making them ideal for complex, real-world scenarios.
Hybrid Approaches for Both Techniques
Combining RAG and fine-tuning allows you to harness the dynamic data integration of RAG alongside the specialized accuracy of fine-tuning. For instance, a model can be fine-tuned on task-specific data to master a particular domain, ensuring it performs with high precision. At the same time, you can integrate RAG to pull in real-time data during inference, allowing the model to adapt to new information or trends that weren’t available during training. This hybrid approach ensures that your AI model remains both deeply knowledgeable and highly responsive to changes.
Example: Customer Support Automation Using Both Methods
Consider a customer support chatbot that needs to handle a wide range of inquiries, from frequently asked questions to complex technical issues. By fine-tuning the model on a dataset of common customer queries, the chatbot can provide accurate, task-specific responses. However, when a new or unusual question arises, RAG can be employed to retrieve the latest information from a knowledge base or support database, ensuring the chatbot delivers a relevant and up-to-date answer. This combination of fine-tuning for accuracy and RAG for adaptability creates a robust customer support system capable of handling both routine and novel queries effectively.
Curious about how to implement a hybrid AI approach? Discover how Raga AI combines advanced techniques to create powerful, adaptable AI systems.
Looking ahead, we’ll explore future trends and recommendations, including the potential for hybrid models, advancements in hardware, and practical advice for choosing the best AI techniques for your needs.
Future Trends and Recommendations
As AI technology continues to evolve, you can expect the integration of techniques like Retrieval-Augmented Generation (RAG) and fine-tuning to become more sophisticated. The future holds exciting possibilities for hybrid models that blend the strengths of both approaches, creating AI systems that are not only highly accurate but also incredibly adaptable to new data and changing environments.
Potential for Hybrid Models
The ongoing development of hybrid models that combine RAG and fine-tuning is likely to reshape how AI performs across industries. These models will benefit from the precision of fine-tuning for specific tasks while using RAG to integrate real-time information, ensuring that AI systems can respond dynamically to new challenges. As the demand for more versatile and intelligent AI solutions grows, hybrid models will play a critical role in meeting these needs.
Hardware Advancements
Advancements in AI hardware can also reduce the computational limitations that currently restrict the use of complex models. With the development of more powerful processors and optimized algorithms, running sophisticated hybrid models will become more feasible even in resource-constrained environments. This will open up new opportunities for deploying advanced AI systems in areas like healthcare, finance, and education, where computational efficiency is crucial.
Increasing Data Improving Methods
As more data becomes available, both RAG and fine-tuning techniques will continue to improve in effectiveness. The ability to access vast amounts of data in real time will enhance the accuracy and relevance of AI models, making them more capable of delivering actionable insights. Organizations should focus on building robust data pipelines that feed their AI systems with high-quality, diverse datasets to maximize the potential of these techniques.
Practical Recommendations
When deciding between RAG and fine-tuning—or whether to combine them—it’s important to consider the specific needs of your project. For tasks that require real-time data integration and adaptability, RAG is an excellent choice. Fine-tuning, on the other hand, is ideal for scenarios where precise, task-specific accuracy is critical. In many cases, a hybrid approach may offer the best of both worlds, providing a balance between accuracy and adaptability.
At Raga AI, we are at the forefront of these exciting developments. Our expertise in combining RAG, fine-tuning, and other advanced techniques enables us to create AI systems that are not only accurate but also adaptable and efficient. Whether you’re looking to enhance your existing AI capabilities or explore new possibilities, Raga AI provides the tools and insights you need to stay ahead in the rapidly evolving AI market. Ready to transform your AI systems? Try Raga AI today!
In the rapidly evolving world of AI, choosing the right learning technique can make all the difference in the performance and scalability of your models. Two of the most powerful methods in this space are Retrieval-Augmented Generation (RAG) and fine-tuning. But with each technique offering distinct advantages, how do you decide which is best for your application?
Understanding the core principles and how they impact your AI system is crucial in making an informed decision. The choice between these methods not only affects the accuracy and efficiency of your AI but also determines how scalable and adaptable your solutions will be in real-world scenarios.
Let’s dive deeper into each approach to see how they stack up against one another.
Understanding RAG (Retrieval-Augmented Generation)
Retrieval-Augmented Generation (RAG) is a powerful AI technique that combines the strengths of retrieval-based methods with generative models. Essentially, RAG enables models to enhance their responses by fetching relevant information from external data sources before generating an output. This approach not only boosts accuracy but also helps in reducing hallucinations, where the model might otherwise produce plausible but incorrect information.
How RAG Works
RAG operates by breaking down the generation process into two main steps: retrieval and generation. First, the model queries an external database or knowledge source to retrieve the most relevant pieces of information. Next, this retrieved data is integrated into the model’s generative process, helping it produce a response that is both contextually relevant and factually accurate.
Here’s a simplified example in Python using a pseudo RAG implementation:
def retrieve_information(query):
# Simulate retrieving relevant data from an external source
external_data = {
"query1": "Relevant information 1",
"query2": "Relevant information 2"
}
return external_data.get(query, "Default information")
def generate_response(query):
# Retrieve data using the RAG method
retrieved_data = retrieve_information(query)
# Integrate retrieved data into the response
response = f"Based on your query, here is what I found: {retrieved_data}"
return response
# Example usage
query = "query1"
response = generate_response(query)
print(response)
In this example, the retrieve_information function simulates fetching data from an external source, which then integrates into the response generated by the generate_response function.
Methods of RAG: Passive and Active
There are two primary methods of RAG: passive and active.
Passive RAG:
Passive RAG relies on existing data repositories, using them to retrieve information as needed during the generation process. The key characteristic of this method is that it does not modify or update the data source in real time.
For a query, the model pulls relevant information from a static database or knowledge base that has been pre-compiled. The retrieved data then integrates into the response generated by the model. Some of its advantages are:
Stability: Since the data source remains unchanged, passive RAG offers a consistent and stable reference for information retrieval, which is beneficial in scenarios where consistency is critical.
Simplicity: With no need for continuous updates, passive RAG is easier to implement and manage, making it suitable for applications where the data does not frequently change.
Efficiency: By relying on pre-existing, static data, passive RAG can be more efficient in terms of computational resources, as it does not require the overhead of updating or refining the data.
Active RAG:
Active RAG involves a more dynamic approach, where the data source is continuously updated and refined based on new inputs and information. This ensures that the model has access to the most current and relevant data.
As the model receives new inputs or queries, it not only retrieves information but also updates the data repository with new data, refining the knowledge base over time. This ongoing refinement allows the model to adapt to changes and incorporate fresh information into its responses. Some advantages are:
Adaptability: Active RAG is highly adaptable, making it ideal for environments where information is constantly changing. The model can adjust to new data, trends, or discoveries in real time, ensuring that the responses are always up-to-date.
Relevance: By continuously updating the data source, active RAG ensures that the information retrieved is as relevant as possible, which is particularly valuable in fields like medical research, legal analysis, or customer support.
Scalability: Active RAG can scale effectively with growing and evolving datasets, allowing the model to handle increasingly complex queries with accurate and timely information.
Each method of RAG has its own strengths, and the choice between passive and active RAG depends on the specific needs of the application—whether you require the consistency of static data or the adaptability to dynamic changes.
Internal Process: Query, Retrieve, Integrate, Generate
The summary of the internal workings of RAG in four key steps:
Query: The model formulates a query based on the user’s input.
Retrieve: It then retrieves relevant data from an external source.
Integrate: The retrieved information integrates into the model’s existing knowledge.
Generate: Finally, the model generates a response that combines the retrieved data with its internal processing capabilities.
This process allows RAG to produce outputs that are not only generated by the model but are also enriched with precise, real-world data, making it particularly effective for complex applications like legal research or technical support.
Interested in learning more about how to build RAG applications? Explore how to build RAG applications, ensuring safe and reliable genAI.
Next, we’ll explore how fine-tuning works, detailing the steps involved and the specific scenarios where this technique excels.
How Fine-Tuning Works
Fine-tuning is a critical technique in the AI learning process, allowing you to adapt pre-trained models to specific tasks by adjusting their parameters based on task-specific data. Unlike RAG, which relies on external data sources during generation, fine-tuning hones the internal parameters of a model, making it more specialized and accurate for a given task. Understanding the differences between RAG and fine-tuning is essential for selecting the best approach for your AI projects.
Steps Involved in Fine-Tuning
The fine-tuning process involves several steps that refine the model’s ability to perform a specific task. Here’s a breakdown of these steps:
Pre-train: Start with a pre-trained model on a large dataset. This model already understands a wide range of general knowledge, which forms the foundation for fine-tuning.
Task-Specific Data Preparation: Gather and prepare the data that is relevant to the specific task you want the model to perform. This step is crucial as the quality and relevance of this data will directly impact the effectiveness of the fine-tuning process.
Reprocess: Reprocess the pre-trained model’s layers to accommodate the new, task-specific data. It involves resetting certain weights and biases to ensure the model can learn the nuances of the new task.
Adjust Layers: Modify the model’s architecture, if necessary, to better suit the task. It could involve adding or removing layers or changing the activation functions to optimize performance.
Configure Model: Set up the model’s parameters, such as learning rate and batch size, to ensure optimal training. These settings play a crucial role in how quickly and effectively the model learns from the new data.
Train: Train the model on the task-specific data, allowing it to learn and adapt. This step typically involves multiple iterations, where the model’s parameters are adjusted based on the data it processes.
Evaluate: After training, evaluate the model’s performance on a validation set to ensure it has learned the task effectively. This step helps identify any issues or areas where further fine-tuning may be needed.
Iterate: Fine-tuning is often an iterative process. Based on the evaluation results, you may need to adjust the data, reconfigure the model, or train further to achieve the desired performance.
When comparing RAG and fine-tuning, it’s clear that fine-tuning requires less reliance on external data during inference but demands careful preparation and iteration to achieve optimal results.
Here’s a simplified code example to illustrate the fine-tuning process:
from transformers import Trainer, TrainingArguments
# Load pre-trained model
model = AutoModelForSequenceClassification.from_pretrained("bert-base-uncased")
# Load task-specific dataset
train_dataset = load_dataset("my_dataset", split="train")
# Define training arguments
training_args = TrainingArguments(
output_dir="./results",
evaluation_strategy="epoch",
learning_rate=2e-5,
per_device_train_batch_size=16,
num_train_epochs=3
)
# Initialize Trainer
trainer = Trainer(
model=model,
args=training_args,
train_dataset=train_dataset,
eval_dataset=val_dataset
)
# Train the model
trainer.train()
This code snippet fine-tunes a pre-trained model using task-specific data, highlighting how the process is more about adjusting and refining an existing model rather than relying on external information retrieval.
Understanding the fine-tuning process and how it contrasts with RAG is key to choosing the right technique for your AI projects. Fine-tuning offers a more focused approach, ideal for scenarios where specialized, high-accuracy models are required.
Want to know more about specific steps involved in optimizing AI models? Explore how Raga AI fine-tunes AI models for specific tasks with precision and efficiency.
Next, we’ll explore the advantages and limitations of RAG, giving you a clearer picture of when to use each technique.
Advantages and Limitations of RAG
Retrieval-Augmented Generation (RAG) is a distinctive approach in AI that merges retrieval-based methods with generative models. This technique offers several benefits but also comes with certain challenges. Understanding these can help you decide when to choose RAG over fine-tuning your AI projects.
Advantages of RAG
Improved Accuracy: RAG enhances accuracy by pulling in relevant, real-time information from external sources. This integration allows the model to generate responses that are not only contextually appropriate but also factually correct.
Reduced Hallucinations: One of RAG's key strengths is its ability to minimize hallucinations, where a model might otherwise produce convincing but incorrect information. By grounding responses in external data, RAG ensures that the information provided is reliable and based on actual data.
Adaptability to New Data: RAG is highly adaptable to new information because it retrieves data in real time. Unlike fine-tuning, which requires retraining the model with new data, RAG can incorporate fresh data on the fly, making it ideal for environments where information changes frequently.
Cost-Effectiveness: Since RAG utilizes existing data sources, it often requires less training data and fewer computational resources. It makes RAG a more cost-effective choice for projects that need to scale and access large volumes of information without the expense of retraining models frequently.
Limitations of RAG
Higher Latency: A significant drawback of RAG is the potential for increased latency. Because the model needs to fetch information from external sources before generating a response, this process can be slower compared to fine-tuned models that rely solely on internal data. It can be a concern in applications where speed is crucial.
Complex Architecture Requirements: Implementing RAG can require a more complex setup, especially when dealing with large-scale retrieval systems. Managing external databases and ensuring efficient data retrieval adds layers of complexity, making RAG more challenging to implement compared to fine-tuning.
When comparing RAG to fine-tuning, RAG excels in accuracy, adaptability, and cost-efficiency, particularly in scenarios where up-to-date information is essential. However, it’s important to consider the trade-offs, such as potential latency issues and the need for a more complex architecture, which might not be suitable for every application.
To learn more about the benefits of using RAG in AI systems, learn how Raga AI enhances AI performance by integrating retrieval-based techniques.
Next, we’ll explore the advantages and limitations of fine-tuning, giving you a deeper understanding of when each method is most appropriate.
Advantages and Limitations of Fine-Tuning
Fine-tuning is a powerful method in AI that allows you to refine a pre-trained model for specific tasks by adjusting its parameters. This approach is particularly effective in scenarios where precise, task-specific performance is required. However, like any technique, fine-tuning comes with its own set of benefits and drawbacks.
Advantages of Fine-Tuning
Less Training Data Required: Fine-tuning is efficient because it builds on an already pre-trained model. It means you need less task-specific data to achieve high accuracy, making it a practical choice when data is limited.
Improved Accuracy: Fine-tuning can significantly enhance the accuracy of a model for a specific task. By fine-tuning the model on a smaller, focused dataset, you can achieve a high level of precision that is well-suited to the particular needs of the application.
Increased Robustness: Through fine-tuning, the model becomes more robust in handling the nuances of the tailored task. It allows the model to perform better on specific, narrow tasks where general pre-trained models might struggle.
Limitations of Fine-Tuning
Potential for Forgetting: One of the main challenges of fine-tuning is the risk of the model "forgetting" the broader knowledge it gained during pre-training. It can occur when the model becomes too specialized in the new task, losing its ability to generalize.
Dependence on Training Data: The effectiveness of fine-tuning depends on the quality and relevance of the task-specific data. If the data is biased or incomplete, the model’s performance will suffer, leading to less reliable outcomes.
Lack of External Knowledge: Unlike RAG, which can pull in real-time information from external sources, fine-tuning relies solely on internal data. This can limit the model's ability to handle new or evolving information, making it less adaptable to changes.
When comparing RAG vs. fine-tuning, fine-tuning shines in tasks that require a deep focus on specific data, providing high accuracy and robustness with less training data. However, it also requires careful consideration of potential drawbacks like forgetting, data dependency, and the absence of external knowledge sources.
To learn more about the process of refining AI models for specific tasks, discover how Raga AI fine-tunes models to achieve high accuracy and reliability.
Next, we’ll dive into a direct comparison of RAG and fine-tuning, examining the factors that influence the choice between these two powerful AI techniques.
Comparison Factors: RAG vs Fine-Tuning
When deciding between Retrieval-Augmented Generation (RAG) and fine-tuning for your AI projects, it’s essential to consider several key factors. Each technique has its strengths and weaknesses, and understanding these can help you choose the best approach based on your specific needs.
External Data Access and Integration
RAG excels in scenarios where real-time access to external data is crucial. By retrieving information from databases or knowledge sources during the generation process, RAG ensures that the model’s responses are both accurate and up-to-date. In contrast, fine-tuning does not access external data during inference; it relies solely on its training data. This makes RAG the better choice when you need the model to handle dynamic or evolving information.
Model Behavior Adjustment
With fine-tuning, you can precisely adjust the behavior of your model by retraining it on task-specific data. This allows for a high degree of customization, making fine-tuning ideal for applications that require the model to perform specific, narrow tasks with great accuracy. RAG, on the other hand, adjusts behavior dynamically during inference by integrating retrieved data, offering flexibility without needing to retrain the model.
Hallucination Suppression
One of the significant advantages of RAG is its ability to suppress hallucinations by grounding responses in external data. This makes RAG particularly useful in applications where accuracy is critical, such as legal or medical fields. Fine-tuning, while capable of improving accuracy, can sometimes lead to overfitting, where the model might produce confident but incorrect outputs if not carefully managed.
Availability of Labeled Training Data
Fine-tuning requires a well-curated, labeled dataset for training. The quality of this data directly impacts the model’s performance. If such data is scarce or costly to obtain, RAG might be a more practical option since it can work with existing external sources, reducing the dependency on large, labeled datasets.
Handling Dynamic vs Static Data
RAG is highly effective in environments where data is dynamic and constantly changing. It allows the model to incorporate the latest information during inference, making it adaptable to new trends or facts. Fine-tuning is better suited for static data scenarios where the task does not require continuous updates, as it involves retraining the model with new data to reflect changes.
Transparency and Interpretability
When it comes to transparency, fine-tuning often provides clearer insights into how a model arrives at its decisions, as the adjustments are made directly within the model’s parameters. RAG, while powerful, can sometimes be less transparent due to the dynamic nature of data retrieval, making it harder to trace the exact source of information used in a response.
Computational Requirements and Scalability
RAG can be more computationally intensive, especially when dealing with large external databases, leading to higher resource consumption and potentially longer response times. Fine-tuning generally has lower runtime requirements since the model does not need to query external data during inference, making it more scalable for high-volume tasks.
Speed and Latency
In terms of speed, fine-tuning often outperforms RAG because it doesn’t involve the additional step of retrieving external data. This makes fine-tuning a better option for applications where low latency is critical. RAG, while potentially slower, offers the advantage of generating more informed and accurate responses by incorporating real-time data.
In summary, the choice between RAG vs. fine-tuning depends on the specific requirements of your project. RAG offers dynamic data integration and reduces hallucinations, making it ideal for complex, information-rich environments. Fine-tuning provides precise behavior adjustment and faster response times, suited for tasks where accuracy and speed are paramount.
To learn more about the differences between various AI model training techniques, explore how Raga AI evaluates and compares AI models to optimize performance and reliability.
Next, we’ll explore real-world use cases for RAG, demonstrating how this technique can be applied to enhance AI applications in various fields.
Use Cases for RAG
Retrieval-Augmented Generation (RAG) is particularly effective in scenarios where integrating real-time, external data into AI-generated responses is crucial. This approach allows AI systems to produce more accurate, contextually relevant, and up-to-date information. Here are some key use cases where RAG shines:
Chatbots and AI Technical Support
RAG is highly beneficial in developing advanced chatbots and AI-driven technical support systems. By retrieving the latest information from knowledge bases or databases, these systems can provide users with precise, relevant answers. For example, in technical support, RAG can pull in real-time troubleshooting steps from a database, ensuring that users receive the most accurate and helpful guidance possible.
Language Translation and Education Tools
In the field of language translation and educational tools, RAG can enhance the learning experience by integrating up-to-date language nuances or educational content into the generated responses. This is particularly useful in applications where the AI needs to stay current with evolving language trends or educational standards, providing learners with the most relevant information.
Medical Research and Diagnosis Augmentation
RAG can play a critical role in medical research and diagnosis, where access to the latest studies, clinical trials, or patient data is essential. By retrieving and integrating the most recent medical information, RAG-powered AI systems can assist healthcare professionals in making informed decisions, improving diagnosis accuracy, and suggesting up-to-date treatment options.
Legal Research and Review Tasks
In legal research, where precision and up-to-date information are paramount, RAG enables AI systems to retrieve relevant case law, statutes, and legal opinions from extensive databases. This capability allows legal professionals to conduct thorough research quickly, ensuring that they are referencing the most current and applicable legal precedents in their work.
Looking to apply RAG in your projects? Discover how Raga AI utilizes retrieval-based techniques to enhance the accuracy and reliability of AI systems.
Next, we’ll explore the use cases for fine-tuning, showing applications of this technique in different scenarios where deep task-specific learning is required.
Use Cases for Fine-Tuning
Fine-tuning is a versatile technique that excels in scenarios where AI models need to be adapted to perform specific tasks with high accuracy. By refining a pre-trained model using task-specific data, fine-tuning ensures that the AI is nuanced to the task at hand. Here are some key use cases where fine-tuning is particularly effective:
Sentiment Analysis
In sentiment analysis, where understanding the emotional tone of the text is critical, fine-tuning allows you to train the AI models on specific datasets that reflect the sentiment patterns relevant to the application. For example, a model fine-tuned on customer reviews can accurately determine whether a review is positive, negative, or neutral, helping businesses understand customer feedback more precisely.
Named-Entity Recognition (NER)
Named-entity recognition involves identifying and classifying key entities within a text, such as names, dates, or locations. Fine-tuning is essential in this area because it enables the AI model to learn the specific entity types that are important for the task. Whether it’s for legal document analysis or extracting key information from medical records, fine-tuning ensures that the model can recognize and categorize entities with high accuracy.
Personalized Content Recommendation
In personalized content recommendation systems, fine-tuning helps tailor the AI’s recommendations to individual user preferences. By training the model on data that reflects user behavior and preferences, fine-tuning ensures that the content suggested is highly relevant and engaging. This approach is widely used in streaming services, e-commerce platforms, and social media, where personalized recommendations are crucial for user satisfaction.
Specific Domain Summarization
Fine-tuning is also highly effective in domain-specific summarization tasks, where the goal is to generate concise summaries of lengthy documents within a particular field. For example, a model fine-tuned on legal documents can summarize complex contracts, while a model fine-tuned on scientific papers can distill key findings into a summary. This specialization allows the AI to produce summaries that are not only accurate but also aligned with the specific requirements of the domain.
Ready to see how fine-tuning can enhance your AI applications? Learn how Raga AI fine-tunes models to achieve high accuracy and reliability for specific tasks.
Next, we’ll explore how combining RAG and fine-tuning can utilize the strengths of both techniques, offering even more powerful AI solutions for complex applications.
Combining RAG and Fine-Tuning
While Retrieval-Augmented Generation (RAG) and fine-tuning each offer distinct advantages, combining these two techniques can reveal even greater potential in AI applications. By leveraging the strengths of both methods, you can create AI systems that are not only highly accurate but also adaptable and resource-efficient, making them ideal for complex, real-world scenarios.
Hybrid Approaches for Both Techniques
Combining RAG and fine-tuning allows you to harness the dynamic data integration of RAG alongside the specialized accuracy of fine-tuning. For instance, a model can be fine-tuned on task-specific data to master a particular domain, ensuring it performs with high precision. At the same time, you can integrate RAG to pull in real-time data during inference, allowing the model to adapt to new information or trends that weren’t available during training. This hybrid approach ensures that your AI model remains both deeply knowledgeable and highly responsive to changes.
Example: Customer Support Automation Using Both Methods
Consider a customer support chatbot that needs to handle a wide range of inquiries, from frequently asked questions to complex technical issues. By fine-tuning the model on a dataset of common customer queries, the chatbot can provide accurate, task-specific responses. However, when a new or unusual question arises, RAG can be employed to retrieve the latest information from a knowledge base or support database, ensuring the chatbot delivers a relevant and up-to-date answer. This combination of fine-tuning for accuracy and RAG for adaptability creates a robust customer support system capable of handling both routine and novel queries effectively.
Curious about how to implement a hybrid AI approach? Discover how Raga AI combines advanced techniques to create powerful, adaptable AI systems.
Looking ahead, we’ll explore future trends and recommendations, including the potential for hybrid models, advancements in hardware, and practical advice for choosing the best AI techniques for your needs.
Future Trends and Recommendations
As AI technology continues to evolve, you can expect the integration of techniques like Retrieval-Augmented Generation (RAG) and fine-tuning to become more sophisticated. The future holds exciting possibilities for hybrid models that blend the strengths of both approaches, creating AI systems that are not only highly accurate but also incredibly adaptable to new data and changing environments.
Potential for Hybrid Models
The ongoing development of hybrid models that combine RAG and fine-tuning is likely to reshape how AI performs across industries. These models will benefit from the precision of fine-tuning for specific tasks while using RAG to integrate real-time information, ensuring that AI systems can respond dynamically to new challenges. As the demand for more versatile and intelligent AI solutions grows, hybrid models will play a critical role in meeting these needs.
Hardware Advancements
Advancements in AI hardware can also reduce the computational limitations that currently restrict the use of complex models. With the development of more powerful processors and optimized algorithms, running sophisticated hybrid models will become more feasible even in resource-constrained environments. This will open up new opportunities for deploying advanced AI systems in areas like healthcare, finance, and education, where computational efficiency is crucial.
Increasing Data Improving Methods
As more data becomes available, both RAG and fine-tuning techniques will continue to improve in effectiveness. The ability to access vast amounts of data in real time will enhance the accuracy and relevance of AI models, making them more capable of delivering actionable insights. Organizations should focus on building robust data pipelines that feed their AI systems with high-quality, diverse datasets to maximize the potential of these techniques.
Practical Recommendations
When deciding between RAG and fine-tuning—or whether to combine them—it’s important to consider the specific needs of your project. For tasks that require real-time data integration and adaptability, RAG is an excellent choice. Fine-tuning, on the other hand, is ideal for scenarios where precise, task-specific accuracy is critical. In many cases, a hybrid approach may offer the best of both worlds, providing a balance between accuracy and adaptability.
At Raga AI, we are at the forefront of these exciting developments. Our expertise in combining RAG, fine-tuning, and other advanced techniques enables us to create AI systems that are not only accurate but also adaptable and efficient. Whether you’re looking to enhance your existing AI capabilities or explore new possibilities, Raga AI provides the tools and insights you need to stay ahead in the rapidly evolving AI market. Ready to transform your AI systems? Try Raga AI today!
Subscribe to our newsletter to never miss an update
Other articles


Agents Talk, Models Think: A2A + MCP for Enterprise Agentic AI

Riya Parikh, Nitai Agarwal
Apr 27, 2025
Read the article
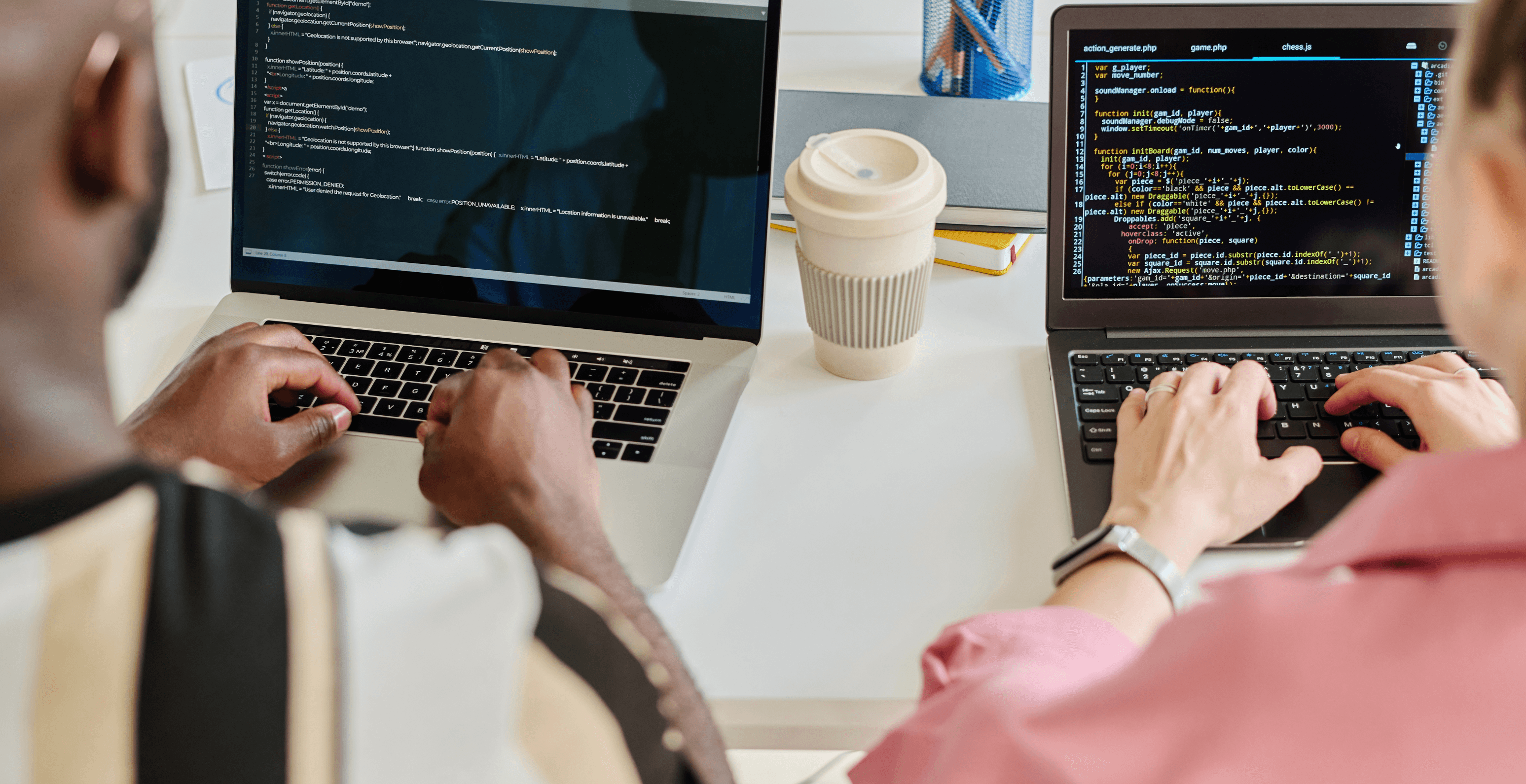
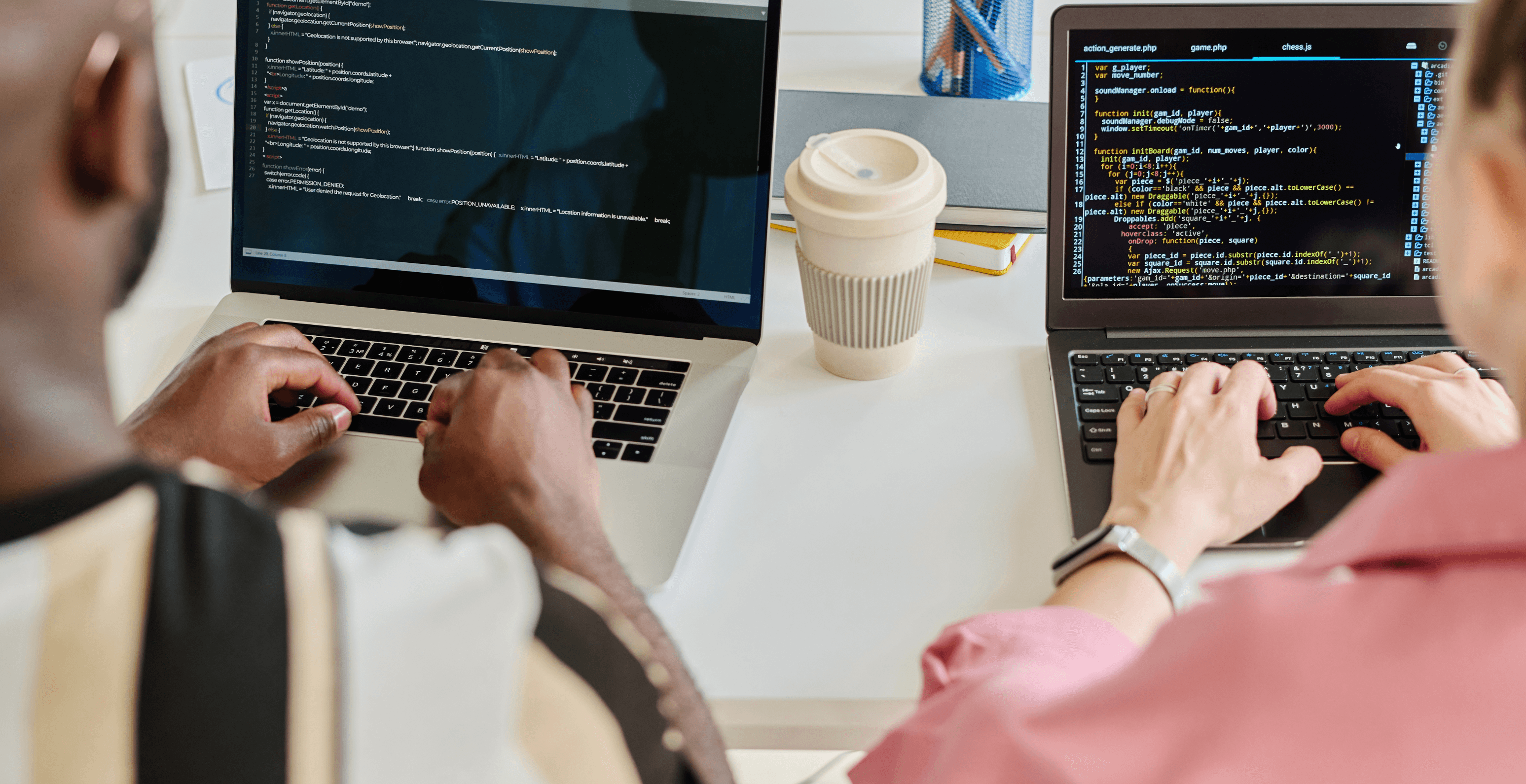
Understanding NeMo Guardrails: A Toolkit for LLM Security

Rehan Asif
Dec 24, 2024
Read the article


Understanding Differences in Large vs Small Language Models (LLM vs SLM)

Rehan Asif
Dec 21, 2024
Read the article