Step by Step Guide to Building RAG-based LLM Applications with Examples
Step by Step Guide to Building RAG-based LLM Applications with Examples
Step by Step Guide to Building RAG-based LLM Applications with Examples
Rehan Asif
Nov 27, 2024




If you've been exploring the world of large language models (LLMs), you've likely encountered their impressive capabilities and notable limitations. One of the significant challenges with LLMs is their tendency to produce hallucinations or inaccurate information, especially when generating responses without sufficient contextual grounding.
This is where Retrieval Augmented Generation (RAG) comes in. For a great RAG LLM example, RAG enhances LLMs by integrating them with external databases, allowing the model to retrieve relevant information and use it to generate more accurate and contextually appropriate responses. This technique not only improves the quality of the generated content but also significantly reduces the risk of hallucinations, making LLM applications more reliable and effective.
In this guide, you'll learn how to build your own RAG-based LLM application from scratch. We'll start with a clear definition of what RAG is and why it's essential for addressing some of the common issues associated with LLMs. For a practical Rag LLM example, we'll walk you through preparing your database, processing the necessary data, and implementing the RAG application. By the end of this guide, you'll have a solid understanding of integrating RAG into your LLM applications, enhancing their performance and reliability. Now, let's dive into understanding RAG and how it tackles the hallucination problem in LLMs.
Understanding RAG
Retrieval Augmented Generation (RAG) is a powerful technique designed to address some limitations of large language models (LLMs). By integrating external knowledge sources, RAG enhances the accuracy and contextual relevance of the generated responses. Let's explain how RAG works and why it's a valuable addition to LLM applications.
How RAG Tackles the Hallucination Problem
One of the biggest issues with LLMs is their tendency to produce hallucinations or responses that seem plausible but are factually incorrect. RAG addresses this by pulling in relevant information from external databases before generating a response. This means the model can access accurate and up-to-date information, significantly reducing the likelihood of hallucinations.
Learn more about LLM hallucinations in this article.
Example:
Consider a query about the capital of New Jersey. Based on its training data, an LLM might generate a wrong answer. However, with RAG, the model retrieves information from a reliable source, ensuring the response is accurate.
from transformers import pipeline, RagTokenizer, RagRetriever, RagTokenForGeneration
# Initialize tokenizer and retriever
tokenizer = RagTokenizer.from_pretrained("facebook/rag-token-base")
retriever = RagRetriever.from_pretrained("facebook/rag-token-base")
# Initialize model
model = RagTokenForGeneration.from_pretrained("facebook/rag-token-base", retriever=retriever)
# Input query
query = "What is the capital of New Jersey?"
# Generate response
input_ids = tokenizer(query, return_tensors="pt").input_ids
outputs = model.generate(input_ids=input_ids)
answer = tokenizer.decode(outputs[0], skip_special_tokens=True)
print(answer)
The Architectural Overview of RAG
RAG combines the strengths of generative models with the precision of retrieval systems. Here's a high-level overview of its architecture:
Retrieval Component: This part of the system searches external databases for relevant information based on the input query. The retrieved documents or data chunks are then fed into the generative model.
Generative Component: Using the retrieved information, the generative model creates a response that is both accurate and contextually appropriate.
Diagram of RAG Architecture:
Practical Benefits of RAG
Improved Accuracy: By integrating external data sources, RAG ensures responses are grounded in factual information.
Enhanced Context: RAG provides additional context to LLMs, making responses more relevant and informative.
Versatility: RAG can be applied to various applications, from customer support chatbots to content generation.
Key Components of RAG
Database Preparation: Ensuring that the external knowledge source is comprehensive and regularly updated.
Data Processing: Efficiently extracting, chunking, and embedding data for quick retrieval.
Integration: Seamlessly combining the retrieval and generative components to work in harmony.
By understanding the foundational principles of RAG, you're now equipped to explore its implementation in your LLM applications. To give you a clear RAG LLM example, we'll next dive into the specifics of preparing your database for RAG.
Preparing the Database for RAG
Setting up a robust database is crucial for the success of your Retrieval Augmented Generation (RAG) application. The database is the backbone, providing the external knowledge needed to generate accurate and contextually relevant responses. Let's break down the steps involved in preparing your database for RAG.
Loading Data into a Local Directory
The first step in preparing your database is to load your data into a local directory. This data can come from various sources such as text files, PDFs, or structured data files like CSVs. Here’s a simple example of how to load text data into a directory.
Example:
import os
# Define the directory path
data_dir = 'rag_data'
# Create the directory if it doesn't exist
if not os.path.exists(data_dir):
os.makedirs(data_dir)
# Sample data
documents = [
"New Jersey's capital is Trenton.",
"Python is a versatile programming language."
]
# Save each document as a separate text file
for i, doc in enumerate(documents):
with open(os.path.join(data_dir, f'doc_{i}.txt'), 'w') as f:
f.write(doc)
Creating Scalable Datasets
Once your data is loaded, the next step is to create scalable datasets that can be efficiently processed. This involves structuring your data in a way that allows for quick retrieval and minimal processing time.
Steps:
Chunking Data: Break down large documents into smaller, manageable chunks.
Embedding Data: Convert text chunks into numerical vectors using pre-trained models.
Example:
from transformers import AutoTokenizer, AutoModel
# Load a pre-trained tokenizer and model
tokenizer = AutoTokenizer.from_pretrained('bert-base-uncased')
model = AutoModel.from_pretrained('bert-base-uncased')
# Function to chunk text
def chunk_text(text, chunk_size=512):
tokens = tokenizer.tokenize(text)
chunks = [tokens[i:i + chunk_size] for i in range(0, len(tokens), chunk_size)]
return [' '.join(chunk) for chunk in chunks]
# Function to embed text
def embed_text(text):
inputs = tokenizer(text, return_tensors='pt')
outputs = model(**inputs)
return outputs.last_hidden_state.mean(dim=1).detach().numpy()
# Example usage
chunks = chunk_text(documents[0])
embeddings = [embed_text(chunk) for chunk in chunks]
Indexing Chunks for Rapid Retrieval
Indexing your data chunks is vital for quick retrieval during query processing. You can use various indexing techniques, such as inverted indices or vector databases, to achieve this.
Example:
from sklearn.neighbors import NearestNeighbors
import numpy as np
# Create an index of embeddings
index = NearestNeighbors(n_neighbors=1, algorithm='ball_tree')
index.fit(np.vstack(embeddings))
# Save the index
import joblib
joblib.dump(index, 'rag_index.pkl')
Diagram of the Data Preparation Process
By ensuring your database is well-prepared and efficiently indexed, you set a solid foundation for your RAG application.
Next, we’ll examine the data processing for RAG, which includes extracting, chunking, and embedding data sections.
Processing Data for RAG
Once you have your data loaded and organized, the next step is to process it for use in your Retrieval Augmented Generation (RAG) application. This involves extracting relevant information, chunking it into manageable pieces, and embedding these chunks for efficient retrieval. Let's dive into these processes in detail to illustrate with a RAG LLM example.
Extracting Data
Extracting data means pulling relevant information from your sources. Depending on your data format, this might involve parsing text files, scraping web content, or querying databases.
Example:
import os
# Directory containing the data
data_dir = 'rag_data'
# Function to read text files from the directory
def read_files(directory):
documents = []
for filename in os.listdir(directory):
if filename.endswith('.txt'):
with open(os.path.join(directory, filename), 'r') as f:
documents.append(f.read())
return documents
# Read data
documents = read_files(data_dir)
print(documents)
Chunking Data
Chunking involves breaking down large documents into smaller, more manageable pieces. This is important for both efficiency and accuracy, as smaller chunks are easier to process and retrieve.
Example:
def chunk_text(text, chunk_size=512):
tokens = tokenizer.tokenize(text)
chunks = [tokens[i:i + chunk_size] for i in range(0, len(tokens), chunk_size)]
return [' '.join(chunk) for chunk in chunks]
# Chunk all documents
chunked_documents = [chunk_text(doc) for doc in documents]
print(chunked_documents)
Embedding Data
Embedding converts text chunks into numerical vectors that can be used for efficient retrieval. Using a pre-trained model, you can transform each chunk into a vector representation.
Example:
from transformers import AutoTokenizer, AutoModel
import torch
# Load a pre-trained tokenizer and model
tokenizer = AutoTokenizer.from_pretrained('bert-base-uncased')
model = AutoModel.from_pretrained('bert-base-uncased')
# Function to embed text
def embed_text(text):
inputs = tokenizer(text, return_tensors='pt', truncation=True, padding=True)
outputs = model(**inputs)
return outputs.last_hidden_state.mean(dim=1).detach().numpy()
# Embed all chunks
embeddings = [embed_text(chunk) for doc in chunked_documents for chunk in doc]
print(embeddings)
Indexing Chunks
Indexing the embedded chunks allows for quick retrieval during the query process. Various indexing techniques can be used, such as using k-nearest neighbors for efficient lookups.
Example:
from sklearn.neighbors import NearestNeighbors
import numpy as np
# Flatten the list of embeddings for indexing
flat_embeddings = np.vstack(embeddings)
# Create an index
index = NearestNeighbors(n_neighbors=5, algorithm='ball_tree')
index.fit(flat_embeddings)
# Save the index for later use
import joblib
joblib.dump(index, 'rag_index.pkl')
Diagram of Data Processing Workflow:
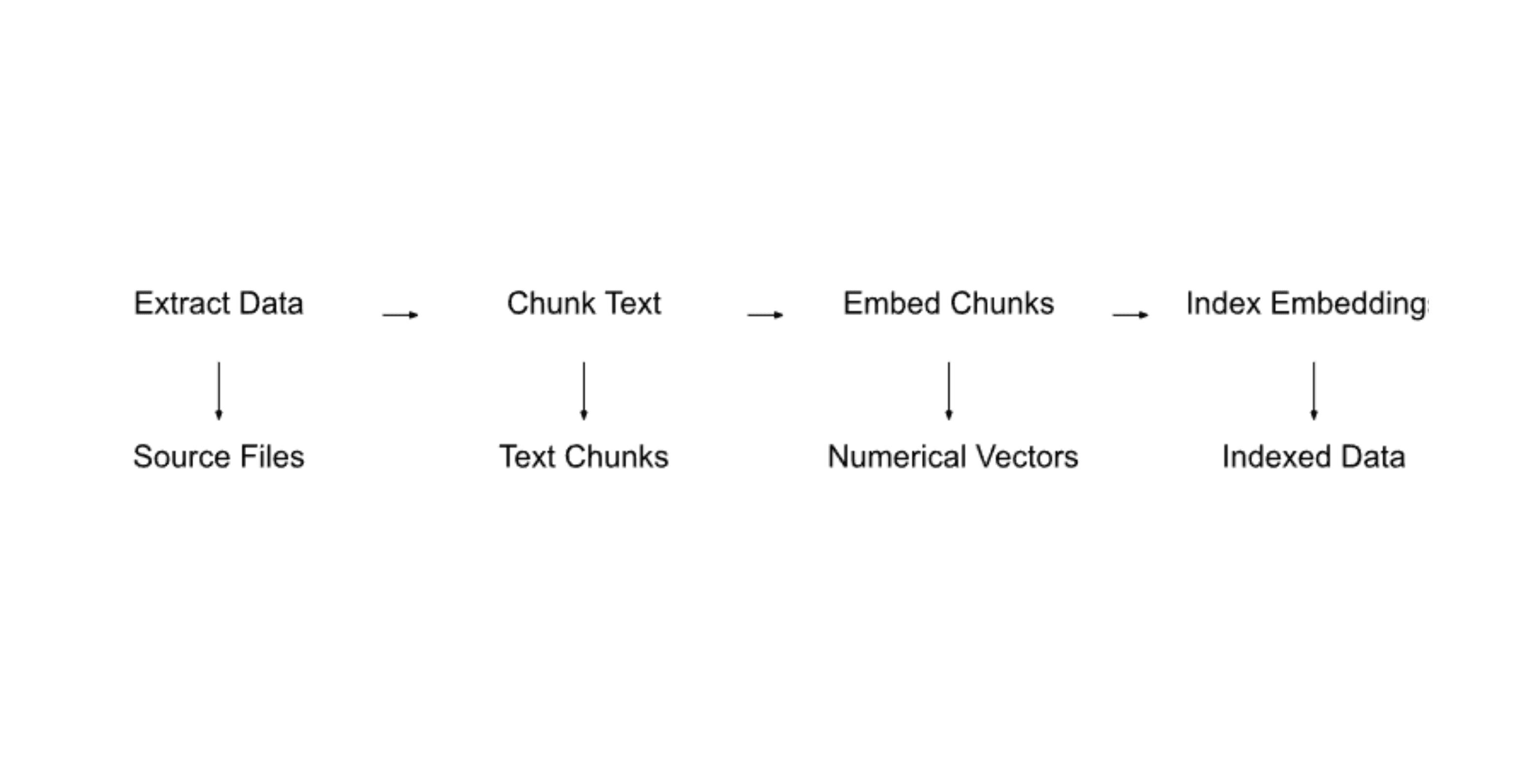
By efficiently processing your data through extraction, chunking, embedding, and indexing, you set the stage for building a powerful RAG application. Next, we will focus on implementing the RAG application, including query retrieval and response generation.
Learn more about the LLM parameters.
Building the RAG Application
Now that your data is processed and ready, it's time to build the Retrieval Augmented Generation (RAG) application. This involves setting up the system for query retrieval, generating responses using the embedded data, and optimizing the application for performance. Let's break down the steps to build your RAG application.
Implementing Query Retrieval
The first step in building your RAG application is implementing the query retrieval system. This system will handle user queries and retrieve relevant data chunks from the indexed database.
Example:
# Function to retrieve relevant data chunks for a query
def retrieve_chunks(query, index, tokenizer, model):
query_embedding = embed_text(query)
distances, indices = index.kneighbors(query_embedding)
return indices[0]
# Example query
query = "What is the capital of New Jersey?"
retrieved_indices = retrieve_chunks(query, index, tokenizer, model)
print(retrieved_indices)
Generating Responses
Once the relevant data chunks are retrieved, the next step is to generate a response using the LLM. The retrieved data provides context, helping the model to produce accurate and relevant answers.
Example:
from transformers import pipeline
# Load a pre-trained generative model
generator = pipeline('text-generation', model='gpt-3.5-turbo')
# Function to generate a response using the retrieved chunks
def generate_response(query, retrieved_indices, data_dir):
context = []
for idx in retrieved_indices:
with open(os.path.join(data_dir, f'doc_{idx}.txt'), 'r') as f:
context.append(f.read())
context = ' '.join(context)
response = generator(f"Context: {context} Query: {query}", max_length=100)
return response[0]['generated_text']
# Generate a response
response = generate_response(query, retrieved_indices, data_dir)
print(response)
Configurations and Optimizations
To ensure your RAG application runs efficiently, you need to configure and optimize various system aspects. This includes tuning the retrieval process and the response generation model.
Example:
# Configurations for query retrieval
NUM_NEIGHBORS = 5 # Number of nearest neighbors to retrieve
# Optimizations for response generation
GENERATION_MAX_LENGTH = 150 # Maximum length of generated responses
# Function to optimize retrieval and generation
def optimized_generate_response(query, index, tokenizer, model, generator, data_dir):
query_embedding = embed_text(query)
distances, indices = index.kneighbors(query_embedding, n_neighbors=NUM_NEIGHBORS)
context = []
for idx in indices[0]:
with open(os.path.join(data_dir, f'doc_{idx}.txt'), 'r') as f:
context.append(f.read())
context = ' '.join(context)
response = generator(f"Context: {context} Query: {query}", max_length=GENERATION_MAX_LENGTH)
return response[0]['generated_text']
# Generate an optimized response
optimized_response = optimized_generate_response(query, index, tokenizer, model, generator, data_dir)
print(optimized_response)
Diagram of the RAG Application Workflow:
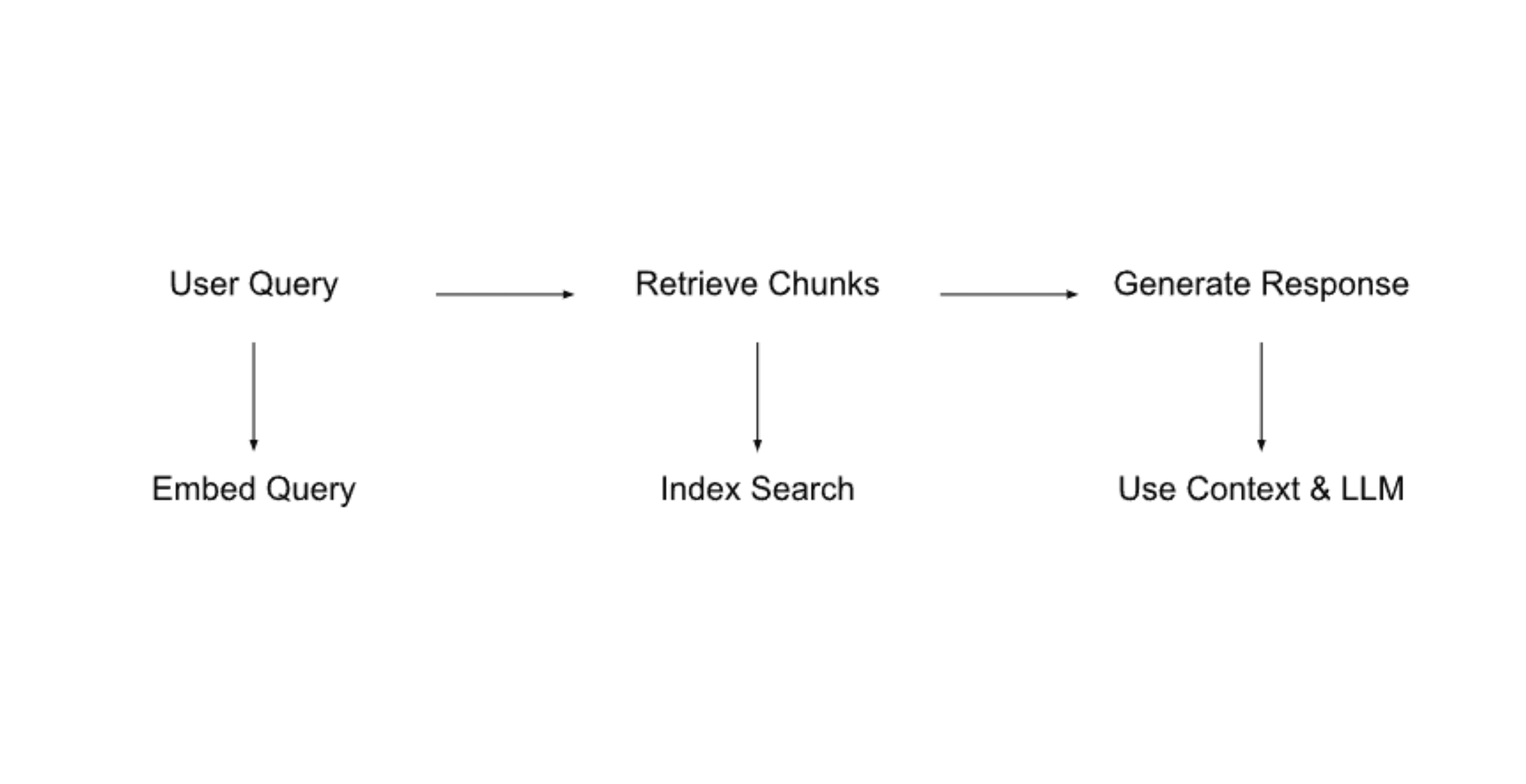
By carefully implementing query retrieval, generating responses with context, and optimizing your configurations, you create a robust RAG application.
To learn more, visit this webinar on building RAG applications and ensuring safe and reliable GenAI.
Next, we will look at how to implement and test the RAG application to ensure it functions correctly.
Implementing and Testing the RAG Application
With your RAG application built, the next crucial step is to implement it and ensure it works correctly. This involves setting up the application for querying, running tests to validate its performance, and fine-tuning as necessary. To illustrate with a RAG LLM example, let's go through these steps in detail.
Setting Up the RAG Application for Querying
The initial implementation step is to set up the RAG application so it can handle queries effectively. This involves integrating all components and ensuring they work together seamlessly.
Example:
# Function to set up the RAG application
def setup_rag_application(data_dir, tokenizer, model, generator, index):
def query_rag(query):
query_embedding = embed_text(query)
distances, indices = index.kneighbors(query_embedding, n_neighbors=5)
context = []
for idx in indices[0]:
with open(os.path.join(data_dir, f'doc_{idx}.txt'), 'r') as f:
context.append(f.read())
context = ' '.join(context)
response = generator(f"Context: {context} Query: {query}", max_length=150)
return response[0]['generated_text']
return query_rag
# Initialize the RAG application
query_rag = setup_rag_application(data_dir, tokenizer, model, generator, index)
Examples and Validation
Testing your RAG application with different queries is essential to validate its performance. By running several tests, you can ensure the system retrieves relevant information and generates accurate responses.
Example Query Test:
# Test the RAG application with a sample query
sample_query = "What is the capital of New Jersey?"
response = query_rag(sample_query)
print(f"Query: {sample_query}\nResponse: {response}")
Validation with Multiple Queries
To thoroughly test the application, use a set of diverse queries and evaluate the responses.
Example:
# List of sample queries
queries = [
"Who wrote 'Pride and Prejudice'?",
"What is the boiling point of water?",
"Define machine learning."
]
# Validate responses
for query in queries:
response = query_rag(query)
print(f"Query: {query}\nResponse: {response}\n")
Performance Metrics:

To quantify the effectiveness of your RAG application, consider using performance metrics such as accuracy, response time, and user satisfaction.
Example of Performance Metrics:
Diagram of Implementation and Testing Workflow:
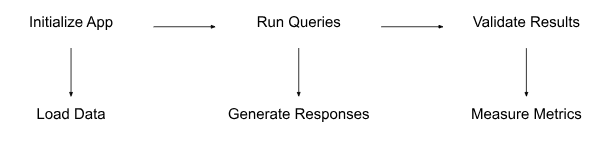
Raga AI’s testing platform is a comprehensive tool that automates the process to ensure you don’t have any data, model, or operational issues. Check out how it is the future of AI testing.
By systematically setting up, implementing, and validating your RAG application, you ensure it performs effectively and meets user needs. Next, we will explore optimizing and evaluating the RAG implementation to enhance performance.
Optimizing and Evaluating the RAG Implementation
Once your RAG application is up and running, the next step is to optimize its performance and evaluate its effectiveness. This ensures that the application not only works but works well. Using a RAG LLM example, optimization and evaluation involve tweaking configurations, conducting thorough assessments, and refining the system based on feedback. Let's explore these processes in detail.
Exploring Different Configurations
Experimenting with different configurations can significantly impact the performance of your RAG application. Adjusting parameters like chunk size, embedding model, and query retrieval settings can enhance efficiency and accuracy.
Example:
# Adjust configurations
CHUNK_SIZE = 256 # Smaller chunks for more precise retrieval
NUM_NEIGHBORS = 3 # Fewer neighbors for faster response
# Function to adjust configurations
def configure_rag(chunk_size, num_neighbors):
# Update chunking and retrieval settings
def chunk_text(text, chunk_size=chunk_size):
tokens = tokenizer.tokenize(text)
chunks = [tokens[i:i + chunk_size] for i in range(0, len(tokens), chunk_size)]
return [' '.join(chunk) for chunk in chunks]
def query_rag(query):
query_embedding = embed_text(query)
distances, indices = index.kneighbors(query_embedding, n_neighbors=num_neighbors)
context = []
for idx in indices[0]:
with open(os.path.join(data_dir, f'doc_{idx}.txt'), 'r') as f:
context.append(f.read())
context = ' '.join(context)
response = generator(f"Context: {context} Query: {query}", max_length=150)
return response[0]['generated_text']
return query_rag
# Apply new configurations
query_rag = configure_rag(CHUNK_SIZE, NUM_NEIGHBORS)
Conducting Evaluations
Systematic evaluations are crucial for understanding how well your RAG application performs. Evaluations can be conducted for both individual components and the entire system.
Example:
# List of sample queries for evaluation
queries = [
"What is the capital of New Jersey?",
"Who wrote 'To Kill a Mockingbird'?",
"Explain the theory of relativity."
]
# Evaluate responses
responses = [query_rag(query) for query in queries]
for query, response in zip(queries, responses):
print(f"Query: {query}\nResponse: {response}\n")
Methods to Quantitatively Assess Generative Tasks
To ensure your RAG application is functioning optimally, assess its performance using quantitative metrics. Common metrics include precision, recall, F1 score, and response time.
Example of Performance Metrics:

Diagram of Optimization and Evaluation Workflow:
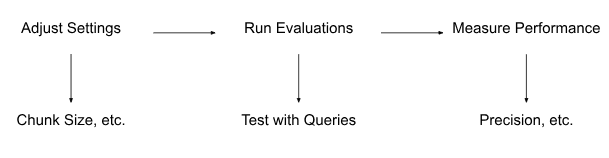
By methodically optimizing configurations and conducting detailed evaluations, you can significantly enhance the performance of your RAG application. Using a RAG LLM example, this approach ensures that it meets the required standards for efficiency and accuracy. Next, we will explore strategies for addressing the cold start problem, which is crucial for maintaining high performance from the start.
Addressing the Cold Start Problem
The cold start problem can be a significant hurdle when implementing a RAG application. It refers to the challenge of getting your model to perform well with little or no initial data. This issue can affect both the quality of responses and the overall user experience. Using a RAG LLM example, here’s how you can address this problem effectively.
Generating Synthetic Q&A Pairs
One of the most effective ways to combat the cold start problem is by generating synthetic Q&A pairs. This involves creating artificial data that can help train your model and improve its initial performance.
Example:
# Function to generate synthetic Q&A pairs
def generate_synthetic_pairs(num_pairs):
synthetic_pairs = []
for _ in range(num_pairs):
question = f"Sample question {_}?"
answer = f"Sample answer for question {_}."
synthetic_pairs.append((question, answer))
return synthetic_pairs
# Generate synthetic pairs
synthetic_data = generate_synthetic_pairs(100)
print(synthetic_data[:5])
Using Pre-trained Models
Leveraging pre-trained models can also help mitigate the cold start problem. These models have already been trained on large datasets and can provide a strong foundation for your application.
Example:
from transformers import AutoModelForQuestionAnswering, AutoTokenizer
# Load a pre-trained model and tokenizer
tokenizer = AutoTokenizer.from_pretrained('bert-large-uncased-whole-word-masking-finetuned-squad')
model = AutoModelForQuestionAnswering.from_pretrained('bert-large-uncased-whole-word-masking-finetuned-squad')
# Function to use pre-trained model for answering questions
def answer_question(question, context):
inputs = tokenizer.encode_plus(question, context, add_special_tokens=True, return_tensors='pt')
answer_start_scores, answer_end_scores = model(**inputs)
answer_start = torch.argmax(answer_start_scores)
answer_end = torch.argmax(answer_end_scores) + 1
answer = tokenizer.convert_tokens_to_string(tokenizer.convert_ids_to_tokens(inputs['input_ids'][0][answer_start:answer_end]))
return answer
# Example usage
context = "New Jersey's capital is Trenton."
question = "What is the capital of New Jersey?"
answer = answer_question(question, context)
print(answer)
Pre-training with Domain-Specific Data
Another effective strategy is to pre-train your model with domain-specific data. This approach ensures that the model is familiar with the specific type of queries it will encounter, thereby improving its performance from the start.
Example:
from transformers import TextDataset, DataCollatorForLanguageModeling, Trainer, TrainingArguments
# Function to load domain-specific data for pre-training
def load_dataset(file_path):
return TextDataset(tokenizer=tokenizer, file_path=file_path, block_size=128)
# Load the dataset
dataset = load_dataset('domain_specific_data.txt')
# Data collator
data_collator = DataCollatorForLanguageModeling(tokenizer=tokenizer, mlm=True)
# Training arguments
training_args = TrainingArguments(
output_dir='./results',
overwrite_output_dir=True,
num_train_epochs=3,
per_device_train_batch_size=8,
save_steps=10_000,
save_total_limit=2,
)
# Trainer
trainer = Trainer(
model=model,
args=training_args,
data_collator=data_collator,
train_dataset=dataset,
)
# Train the model
trainer.train()
Diagram of Cold Start Mitigation Strategies:
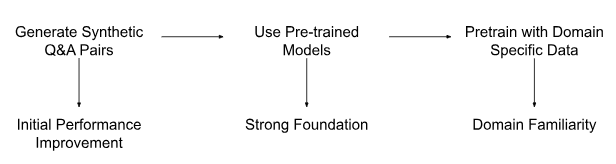
Employing these strategies can effectively address the cold start problem and ensure your RAG application performs well right from the beginning.
Read this case study on enhancing reliability with guardrails.
Next, we will explore how to experiment with different configurations and fine-tune the system for optimal performance.
Experimentation and Improvement
After addressing the cold start problem, the next step is to refine and enhance your RAG application. Using a RAG LLM example, experimentation and continuous improvement are vital to optimizing performance and ensuring the application meets your needs. This involves testing various configurations, models, and techniques to find the best setup. Let's dive into these processes.
Testing Different Chunk Sizes
The size of the text chunks used in your RAG application can significantly impact performance. Experimenting with different chunk sizes helps find the optimal balance between retrieval accuracy and processing efficiency.
Example:
# Function to experiment with different chunk sizes
def test_chunk_sizes(text, sizes=[128, 256, 512]):
results = {}
for size in sizes:
chunks = chunk_text(text, chunk_size=size)
embeddings = [embed_text(chunk) for chunk in chunks]
results[size] = embeddings
return results
# Sample text for chunking
text = "New Jersey's capital is Trenton. Python is a versatile programming language."
# Test different chunk sizes
chunk_results = test_chunk_sizes(text)
for size, embeddings in chunk_results.items():
print(f"Chunk Size: {size}, Number of Chunks: {len(embeddings)}")
Experimenting with Embedding Models
Different embedding models can produce varying results in terms of accuracy and performance. Testing multiple models helps identify which one works best for your specific use case.
Example:
from transformers import AutoModel
# Function to test different embedding models
def test_embedding_models(text, models=['bert-base-uncased', 'roberta-base']):
results = {}
for model_name in models:
tokenizer = AutoTokenizer.from_pretrained(model_name)
model = AutoModel.from_pretrained(model_name)
inputs = tokenizer(text, return_tensors='pt', truncation=True, padding=True)
outputs = model(**inputs)
embeddings = outputs.last_hidden_state.mean(dim=1).detach().numpy()
results[model_name] = embeddings
return results
# Test text for embeddings
test_text = "Machine learning is a branch of artificial intelligence."
# Test different embedding models
embedding_results = test_embedding_models(test_text)
for model_name, embedding in embedding_results.items():
print(f"Model: {model_name}, Embedding Shape: {embedding.shape}")
Fine-Tuning for Better Context Representation
Fine-tuning your models with domain-specific data can significantly improve their ability to represent context accurately. This process involves training the model on data that is similar to what it will encounter in actual use.
Example:
from transformers import Trainer, TrainingArguments
# Function to fine-tune a model
def fine_tune_model(model_name, dataset_path):
tokenizer = AutoTokenizer.from_pretrained(model_name)
model = AutoModel.from_pretrained(model_name)
# Load dataset
dataset = load_dataset(dataset_path)
# Data collator
data_collator = DataCollatorForLanguageModeling(tokenizer=tokenizer, mlm=True)
# Training arguments
training_args = TrainingArguments(
output_dir='./results',
num_train_epochs=3,
per_device_train_batch_size=8,
save_steps=10_000,
save_total_limit=2,
)
# Trainer
trainer = Trainer(
model=model,
args=training_args,
data_collator=data_collator,
train_dataset=dataset,
)
# Train the model
trainer.train()
return model
# Fine-tune the model with domain-specific data
fine_tuned_model = fine_tune_model('bert-base-uncased', 'domain_specific_data.txt')
Diagram of Experimentation and Improvement Workflow:
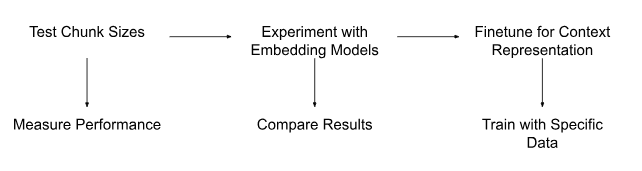
By experimenting with different configurations and models and fine-tuning your system, you can continuously improve the performance of your RAG application. This iterative process ensures that the application remains effective and efficient. Next, we will discuss examples of RAG LLM techniques for serving and scaling your RAG application to handle real-world demands.
Application Serving, Scaling, and Continuous Improvement
Building a robust RAG application is only the beginning. To make it truly useful, you must serve it efficiently, scale it to meet demand, and continuously improve it based on user feedback and performance metrics. Let’s delve into these aspects.
Serving the RAG Application
Serving your RAG application means making it accessible for users to query. This typically involves setting up an API endpoint that handles incoming requests and returns generated responses.
Example:
from flask import Flask, request, jsonify
app = Flask(__name__)
# Load the model and other necessary components
query_rag = setup_rag_application(data_dir, tokenizer, model, generator, index)
@app.route('/query', methods=['POST'])
def query():
data = request.get_json()
query_text = data['query']
response = query_rag(query_text)
return jsonify({'response': response})
if __name__ == '__main__':
app.run(debug=True)
Scaling the RAG Application
To handle multiple queries simultaneously and ensure fast response times, you need to scale your application. Using a RAG LLM example, this involves deploying your application on a robust infrastructure that can manage high traffic and distribute load effectively.
Example:
Horizontal Scaling: Deploy multiple instances of your application and use a load balancer to distribute traffic.
Vertical Scaling: Increase the computational resources (CPU, RAM) of your existing server to handle more requests.
Techniques for Scaling:
Containerization: Use Docker to containerize your application, making it easy to deploy and scale across different environments.
Example:
# Dockerfile for RAG application
FROM python:3.8-slim
WORKDIR /app
COPY requirements.txt requirements.txt
RUN pip install -r requirements.txt
COPY . .
CMD ["python", "app.py"]
Orchestration: Use Kubernetes to manage and scale your containerized application.
Example:
apiVersion: apps/v1
kind: Deployment
metadata:
name: rag-deployment
spec:
replicas: 3
selector:
matchLabels:
app: rag-app
template:
metadata:
labels:
app: rag-app
spec:
containers:
- name: rag-container
image: rag-image
ports:
- containerPort: 5000
Continuous Improvement
To ensure your application remains effective, continuous improvement is crucial. This involves regularly updating your models, refining data, and incorporating user feedback.
Strategies for Continuous Improvement:
Monitor Performance: Regularly track key metrics such as response time, accuracy, and user satisfaction.
Example of Monitoring Metrics:

Feedback Loop: Implement a feedback mechanism where users can rate responses and provide comments. Use this feedback to improve your models and data.
Regular Updates: Schedule regular updates for your models and data to incorporate the latest information and improvements.
Diagram of Application Serving and Scaling Workflow:
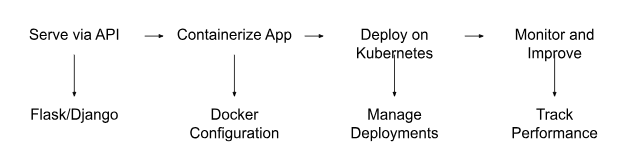
By efficiently serving, scaling, and continuously improving your RAG application, you ensure it remains reliable, responsive, and effective. Next, we will summarize the entire process and discuss the future prospects of integrating RAG into LLM applications.
Conclusion
Building a Retrieval Augmented Generation (RAG) application is a comprehensive process that involves several crucial steps. Using a RAG LLM example, you'll start by understanding the basics of RAG, preparing your database, processing data, building, implementing, and continuously improving your application. Each step plays a vital role in ensuring the success of your project. By following the detailed instructions provided, you can create a robust RAG application that delivers accurate and contextually relevant responses, enhancing the overall user experience.
Raga AI offers a state-of-the-art platform designed to simplify the development and deployment of AI applications. With features such as automated issue detection, diagnosis, and resolution, Raga AI ensures your applications are reliable and high-performing. If you're looking to enhance your AI projects with the latest in RAG technology, consider exploring Raga AI's comprehensive solutions. Start your journey with Raga AI today and take your AI applications to the next level.
If you've been exploring the world of large language models (LLMs), you've likely encountered their impressive capabilities and notable limitations. One of the significant challenges with LLMs is their tendency to produce hallucinations or inaccurate information, especially when generating responses without sufficient contextual grounding.
This is where Retrieval Augmented Generation (RAG) comes in. For a great RAG LLM example, RAG enhances LLMs by integrating them with external databases, allowing the model to retrieve relevant information and use it to generate more accurate and contextually appropriate responses. This technique not only improves the quality of the generated content but also significantly reduces the risk of hallucinations, making LLM applications more reliable and effective.
In this guide, you'll learn how to build your own RAG-based LLM application from scratch. We'll start with a clear definition of what RAG is and why it's essential for addressing some of the common issues associated with LLMs. For a practical Rag LLM example, we'll walk you through preparing your database, processing the necessary data, and implementing the RAG application. By the end of this guide, you'll have a solid understanding of integrating RAG into your LLM applications, enhancing their performance and reliability. Now, let's dive into understanding RAG and how it tackles the hallucination problem in LLMs.
Understanding RAG
Retrieval Augmented Generation (RAG) is a powerful technique designed to address some limitations of large language models (LLMs). By integrating external knowledge sources, RAG enhances the accuracy and contextual relevance of the generated responses. Let's explain how RAG works and why it's a valuable addition to LLM applications.
How RAG Tackles the Hallucination Problem
One of the biggest issues with LLMs is their tendency to produce hallucinations or responses that seem plausible but are factually incorrect. RAG addresses this by pulling in relevant information from external databases before generating a response. This means the model can access accurate and up-to-date information, significantly reducing the likelihood of hallucinations.
Learn more about LLM hallucinations in this article.
Example:
Consider a query about the capital of New Jersey. Based on its training data, an LLM might generate a wrong answer. However, with RAG, the model retrieves information from a reliable source, ensuring the response is accurate.
from transformers import pipeline, RagTokenizer, RagRetriever, RagTokenForGeneration
# Initialize tokenizer and retriever
tokenizer = RagTokenizer.from_pretrained("facebook/rag-token-base")
retriever = RagRetriever.from_pretrained("facebook/rag-token-base")
# Initialize model
model = RagTokenForGeneration.from_pretrained("facebook/rag-token-base", retriever=retriever)
# Input query
query = "What is the capital of New Jersey?"
# Generate response
input_ids = tokenizer(query, return_tensors="pt").input_ids
outputs = model.generate(input_ids=input_ids)
answer = tokenizer.decode(outputs[0], skip_special_tokens=True)
print(answer)
The Architectural Overview of RAG
RAG combines the strengths of generative models with the precision of retrieval systems. Here's a high-level overview of its architecture:
Retrieval Component: This part of the system searches external databases for relevant information based on the input query. The retrieved documents or data chunks are then fed into the generative model.
Generative Component: Using the retrieved information, the generative model creates a response that is both accurate and contextually appropriate.
Diagram of RAG Architecture:
Practical Benefits of RAG
Improved Accuracy: By integrating external data sources, RAG ensures responses are grounded in factual information.
Enhanced Context: RAG provides additional context to LLMs, making responses more relevant and informative.
Versatility: RAG can be applied to various applications, from customer support chatbots to content generation.
Key Components of RAG
Database Preparation: Ensuring that the external knowledge source is comprehensive and regularly updated.
Data Processing: Efficiently extracting, chunking, and embedding data for quick retrieval.
Integration: Seamlessly combining the retrieval and generative components to work in harmony.
By understanding the foundational principles of RAG, you're now equipped to explore its implementation in your LLM applications. To give you a clear RAG LLM example, we'll next dive into the specifics of preparing your database for RAG.
Preparing the Database for RAG
Setting up a robust database is crucial for the success of your Retrieval Augmented Generation (RAG) application. The database is the backbone, providing the external knowledge needed to generate accurate and contextually relevant responses. Let's break down the steps involved in preparing your database for RAG.
Loading Data into a Local Directory
The first step in preparing your database is to load your data into a local directory. This data can come from various sources such as text files, PDFs, or structured data files like CSVs. Here’s a simple example of how to load text data into a directory.
Example:
import os
# Define the directory path
data_dir = 'rag_data'
# Create the directory if it doesn't exist
if not os.path.exists(data_dir):
os.makedirs(data_dir)
# Sample data
documents = [
"New Jersey's capital is Trenton.",
"Python is a versatile programming language."
]
# Save each document as a separate text file
for i, doc in enumerate(documents):
with open(os.path.join(data_dir, f'doc_{i}.txt'), 'w') as f:
f.write(doc)
Creating Scalable Datasets
Once your data is loaded, the next step is to create scalable datasets that can be efficiently processed. This involves structuring your data in a way that allows for quick retrieval and minimal processing time.
Steps:
Chunking Data: Break down large documents into smaller, manageable chunks.
Embedding Data: Convert text chunks into numerical vectors using pre-trained models.
Example:
from transformers import AutoTokenizer, AutoModel
# Load a pre-trained tokenizer and model
tokenizer = AutoTokenizer.from_pretrained('bert-base-uncased')
model = AutoModel.from_pretrained('bert-base-uncased')
# Function to chunk text
def chunk_text(text, chunk_size=512):
tokens = tokenizer.tokenize(text)
chunks = [tokens[i:i + chunk_size] for i in range(0, len(tokens), chunk_size)]
return [' '.join(chunk) for chunk in chunks]
# Function to embed text
def embed_text(text):
inputs = tokenizer(text, return_tensors='pt')
outputs = model(**inputs)
return outputs.last_hidden_state.mean(dim=1).detach().numpy()
# Example usage
chunks = chunk_text(documents[0])
embeddings = [embed_text(chunk) for chunk in chunks]
Indexing Chunks for Rapid Retrieval
Indexing your data chunks is vital for quick retrieval during query processing. You can use various indexing techniques, such as inverted indices or vector databases, to achieve this.
Example:
from sklearn.neighbors import NearestNeighbors
import numpy as np
# Create an index of embeddings
index = NearestNeighbors(n_neighbors=1, algorithm='ball_tree')
index.fit(np.vstack(embeddings))
# Save the index
import joblib
joblib.dump(index, 'rag_index.pkl')
Diagram of the Data Preparation Process
By ensuring your database is well-prepared and efficiently indexed, you set a solid foundation for your RAG application.
Next, we’ll examine the data processing for RAG, which includes extracting, chunking, and embedding data sections.
Processing Data for RAG
Once you have your data loaded and organized, the next step is to process it for use in your Retrieval Augmented Generation (RAG) application. This involves extracting relevant information, chunking it into manageable pieces, and embedding these chunks for efficient retrieval. Let's dive into these processes in detail to illustrate with a RAG LLM example.
Extracting Data
Extracting data means pulling relevant information from your sources. Depending on your data format, this might involve parsing text files, scraping web content, or querying databases.
Example:
import os
# Directory containing the data
data_dir = 'rag_data'
# Function to read text files from the directory
def read_files(directory):
documents = []
for filename in os.listdir(directory):
if filename.endswith('.txt'):
with open(os.path.join(directory, filename), 'r') as f:
documents.append(f.read())
return documents
# Read data
documents = read_files(data_dir)
print(documents)
Chunking Data
Chunking involves breaking down large documents into smaller, more manageable pieces. This is important for both efficiency and accuracy, as smaller chunks are easier to process and retrieve.
Example:
def chunk_text(text, chunk_size=512):
tokens = tokenizer.tokenize(text)
chunks = [tokens[i:i + chunk_size] for i in range(0, len(tokens), chunk_size)]
return [' '.join(chunk) for chunk in chunks]
# Chunk all documents
chunked_documents = [chunk_text(doc) for doc in documents]
print(chunked_documents)
Embedding Data
Embedding converts text chunks into numerical vectors that can be used for efficient retrieval. Using a pre-trained model, you can transform each chunk into a vector representation.
Example:
from transformers import AutoTokenizer, AutoModel
import torch
# Load a pre-trained tokenizer and model
tokenizer = AutoTokenizer.from_pretrained('bert-base-uncased')
model = AutoModel.from_pretrained('bert-base-uncased')
# Function to embed text
def embed_text(text):
inputs = tokenizer(text, return_tensors='pt', truncation=True, padding=True)
outputs = model(**inputs)
return outputs.last_hidden_state.mean(dim=1).detach().numpy()
# Embed all chunks
embeddings = [embed_text(chunk) for doc in chunked_documents for chunk in doc]
print(embeddings)
Indexing Chunks
Indexing the embedded chunks allows for quick retrieval during the query process. Various indexing techniques can be used, such as using k-nearest neighbors for efficient lookups.
Example:
from sklearn.neighbors import NearestNeighbors
import numpy as np
# Flatten the list of embeddings for indexing
flat_embeddings = np.vstack(embeddings)
# Create an index
index = NearestNeighbors(n_neighbors=5, algorithm='ball_tree')
index.fit(flat_embeddings)
# Save the index for later use
import joblib
joblib.dump(index, 'rag_index.pkl')
Diagram of Data Processing Workflow:
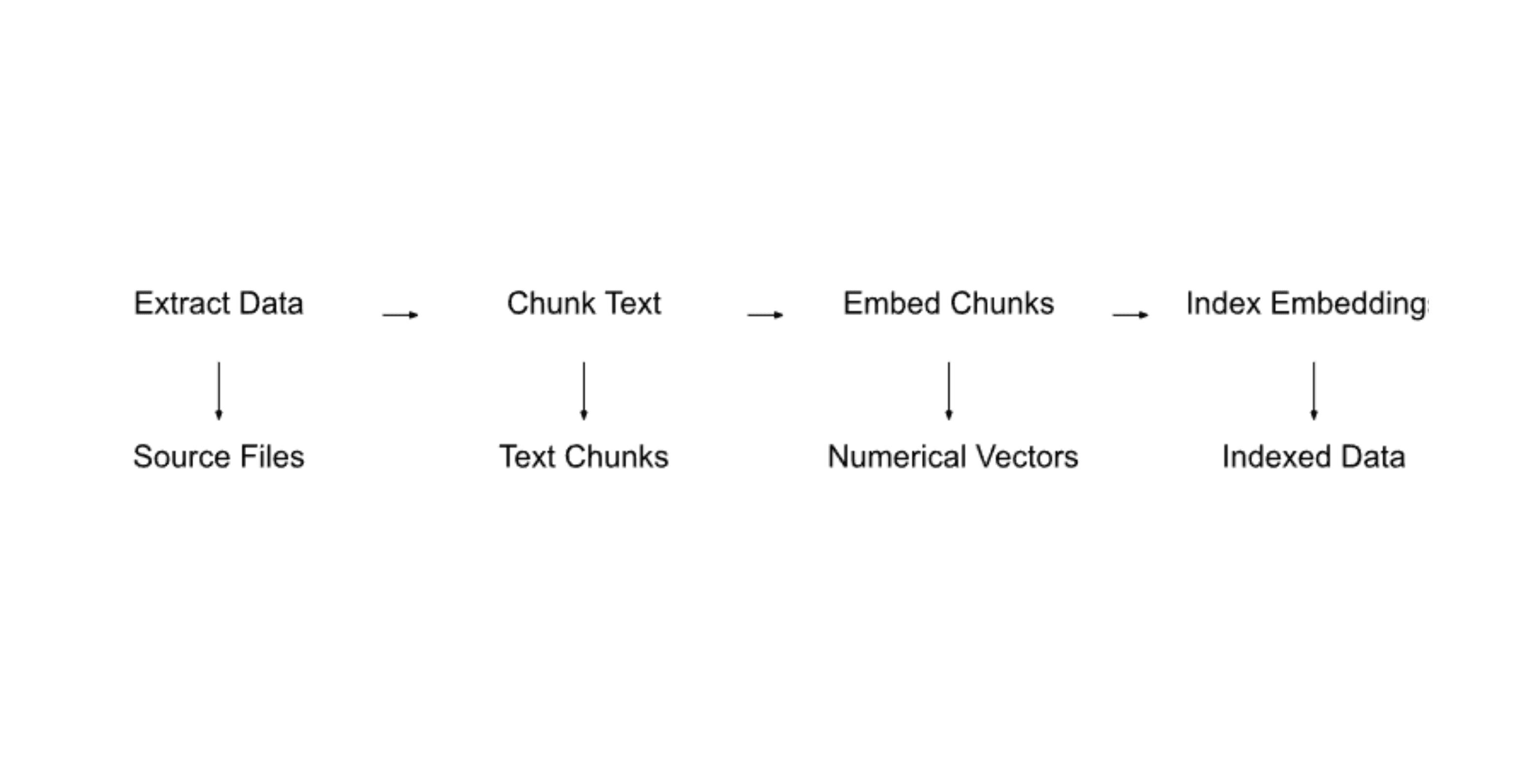
By efficiently processing your data through extraction, chunking, embedding, and indexing, you set the stage for building a powerful RAG application. Next, we will focus on implementing the RAG application, including query retrieval and response generation.
Learn more about the LLM parameters.
Building the RAG Application
Now that your data is processed and ready, it's time to build the Retrieval Augmented Generation (RAG) application. This involves setting up the system for query retrieval, generating responses using the embedded data, and optimizing the application for performance. Let's break down the steps to build your RAG application.
Implementing Query Retrieval
The first step in building your RAG application is implementing the query retrieval system. This system will handle user queries and retrieve relevant data chunks from the indexed database.
Example:
# Function to retrieve relevant data chunks for a query
def retrieve_chunks(query, index, tokenizer, model):
query_embedding = embed_text(query)
distances, indices = index.kneighbors(query_embedding)
return indices[0]
# Example query
query = "What is the capital of New Jersey?"
retrieved_indices = retrieve_chunks(query, index, tokenizer, model)
print(retrieved_indices)
Generating Responses
Once the relevant data chunks are retrieved, the next step is to generate a response using the LLM. The retrieved data provides context, helping the model to produce accurate and relevant answers.
Example:
from transformers import pipeline
# Load a pre-trained generative model
generator = pipeline('text-generation', model='gpt-3.5-turbo')
# Function to generate a response using the retrieved chunks
def generate_response(query, retrieved_indices, data_dir):
context = []
for idx in retrieved_indices:
with open(os.path.join(data_dir, f'doc_{idx}.txt'), 'r') as f:
context.append(f.read())
context = ' '.join(context)
response = generator(f"Context: {context} Query: {query}", max_length=100)
return response[0]['generated_text']
# Generate a response
response = generate_response(query, retrieved_indices, data_dir)
print(response)
Configurations and Optimizations
To ensure your RAG application runs efficiently, you need to configure and optimize various system aspects. This includes tuning the retrieval process and the response generation model.
Example:
# Configurations for query retrieval
NUM_NEIGHBORS = 5 # Number of nearest neighbors to retrieve
# Optimizations for response generation
GENERATION_MAX_LENGTH = 150 # Maximum length of generated responses
# Function to optimize retrieval and generation
def optimized_generate_response(query, index, tokenizer, model, generator, data_dir):
query_embedding = embed_text(query)
distances, indices = index.kneighbors(query_embedding, n_neighbors=NUM_NEIGHBORS)
context = []
for idx in indices[0]:
with open(os.path.join(data_dir, f'doc_{idx}.txt'), 'r') as f:
context.append(f.read())
context = ' '.join(context)
response = generator(f"Context: {context} Query: {query}", max_length=GENERATION_MAX_LENGTH)
return response[0]['generated_text']
# Generate an optimized response
optimized_response = optimized_generate_response(query, index, tokenizer, model, generator, data_dir)
print(optimized_response)
Diagram of the RAG Application Workflow:
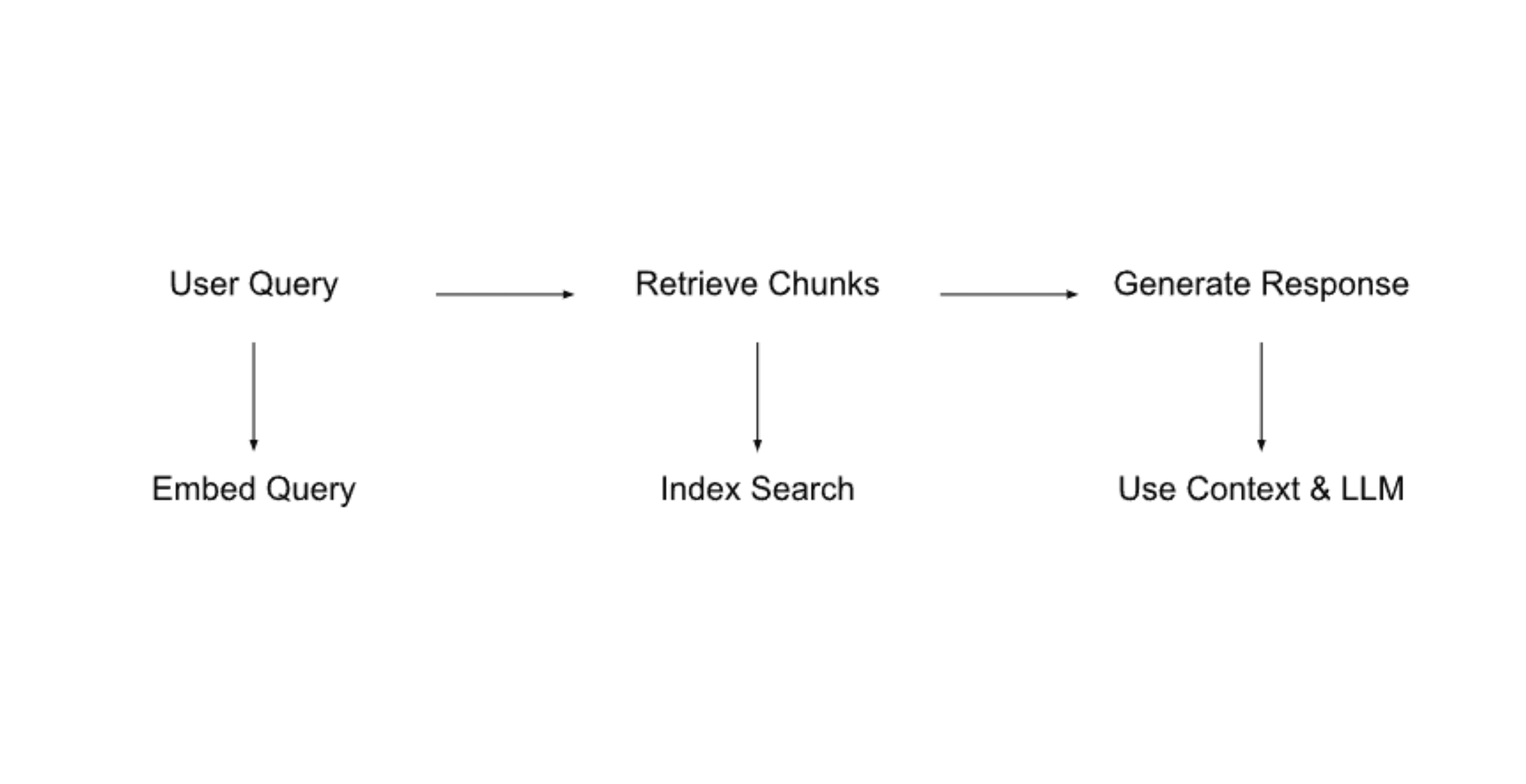
By carefully implementing query retrieval, generating responses with context, and optimizing your configurations, you create a robust RAG application.
To learn more, visit this webinar on building RAG applications and ensuring safe and reliable GenAI.
Next, we will look at how to implement and test the RAG application to ensure it functions correctly.
Implementing and Testing the RAG Application
With your RAG application built, the next crucial step is to implement it and ensure it works correctly. This involves setting up the application for querying, running tests to validate its performance, and fine-tuning as necessary. To illustrate with a RAG LLM example, let's go through these steps in detail.
Setting Up the RAG Application for Querying
The initial implementation step is to set up the RAG application so it can handle queries effectively. This involves integrating all components and ensuring they work together seamlessly.
Example:
# Function to set up the RAG application
def setup_rag_application(data_dir, tokenizer, model, generator, index):
def query_rag(query):
query_embedding = embed_text(query)
distances, indices = index.kneighbors(query_embedding, n_neighbors=5)
context = []
for idx in indices[0]:
with open(os.path.join(data_dir, f'doc_{idx}.txt'), 'r') as f:
context.append(f.read())
context = ' '.join(context)
response = generator(f"Context: {context} Query: {query}", max_length=150)
return response[0]['generated_text']
return query_rag
# Initialize the RAG application
query_rag = setup_rag_application(data_dir, tokenizer, model, generator, index)
Examples and Validation
Testing your RAG application with different queries is essential to validate its performance. By running several tests, you can ensure the system retrieves relevant information and generates accurate responses.
Example Query Test:
# Test the RAG application with a sample query
sample_query = "What is the capital of New Jersey?"
response = query_rag(sample_query)
print(f"Query: {sample_query}\nResponse: {response}")
Validation with Multiple Queries
To thoroughly test the application, use a set of diverse queries and evaluate the responses.
Example:
# List of sample queries
queries = [
"Who wrote 'Pride and Prejudice'?",
"What is the boiling point of water?",
"Define machine learning."
]
# Validate responses
for query in queries:
response = query_rag(query)
print(f"Query: {query}\nResponse: {response}\n")
Performance Metrics:

To quantify the effectiveness of your RAG application, consider using performance metrics such as accuracy, response time, and user satisfaction.
Example of Performance Metrics:
Diagram of Implementation and Testing Workflow:
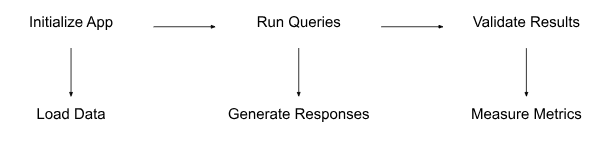
Raga AI’s testing platform is a comprehensive tool that automates the process to ensure you don’t have any data, model, or operational issues. Check out how it is the future of AI testing.
By systematically setting up, implementing, and validating your RAG application, you ensure it performs effectively and meets user needs. Next, we will explore optimizing and evaluating the RAG implementation to enhance performance.
Optimizing and Evaluating the RAG Implementation
Once your RAG application is up and running, the next step is to optimize its performance and evaluate its effectiveness. This ensures that the application not only works but works well. Using a RAG LLM example, optimization and evaluation involve tweaking configurations, conducting thorough assessments, and refining the system based on feedback. Let's explore these processes in detail.
Exploring Different Configurations
Experimenting with different configurations can significantly impact the performance of your RAG application. Adjusting parameters like chunk size, embedding model, and query retrieval settings can enhance efficiency and accuracy.
Example:
# Adjust configurations
CHUNK_SIZE = 256 # Smaller chunks for more precise retrieval
NUM_NEIGHBORS = 3 # Fewer neighbors for faster response
# Function to adjust configurations
def configure_rag(chunk_size, num_neighbors):
# Update chunking and retrieval settings
def chunk_text(text, chunk_size=chunk_size):
tokens = tokenizer.tokenize(text)
chunks = [tokens[i:i + chunk_size] for i in range(0, len(tokens), chunk_size)]
return [' '.join(chunk) for chunk in chunks]
def query_rag(query):
query_embedding = embed_text(query)
distances, indices = index.kneighbors(query_embedding, n_neighbors=num_neighbors)
context = []
for idx in indices[0]:
with open(os.path.join(data_dir, f'doc_{idx}.txt'), 'r') as f:
context.append(f.read())
context = ' '.join(context)
response = generator(f"Context: {context} Query: {query}", max_length=150)
return response[0]['generated_text']
return query_rag
# Apply new configurations
query_rag = configure_rag(CHUNK_SIZE, NUM_NEIGHBORS)
Conducting Evaluations
Systematic evaluations are crucial for understanding how well your RAG application performs. Evaluations can be conducted for both individual components and the entire system.
Example:
# List of sample queries for evaluation
queries = [
"What is the capital of New Jersey?",
"Who wrote 'To Kill a Mockingbird'?",
"Explain the theory of relativity."
]
# Evaluate responses
responses = [query_rag(query) for query in queries]
for query, response in zip(queries, responses):
print(f"Query: {query}\nResponse: {response}\n")
Methods to Quantitatively Assess Generative Tasks
To ensure your RAG application is functioning optimally, assess its performance using quantitative metrics. Common metrics include precision, recall, F1 score, and response time.
Example of Performance Metrics:

Diagram of Optimization and Evaluation Workflow:
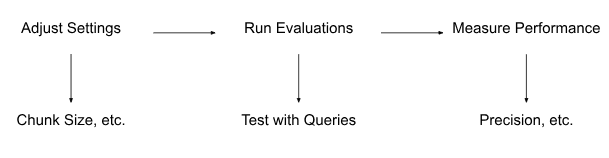
By methodically optimizing configurations and conducting detailed evaluations, you can significantly enhance the performance of your RAG application. Using a RAG LLM example, this approach ensures that it meets the required standards for efficiency and accuracy. Next, we will explore strategies for addressing the cold start problem, which is crucial for maintaining high performance from the start.
Addressing the Cold Start Problem
The cold start problem can be a significant hurdle when implementing a RAG application. It refers to the challenge of getting your model to perform well with little or no initial data. This issue can affect both the quality of responses and the overall user experience. Using a RAG LLM example, here’s how you can address this problem effectively.
Generating Synthetic Q&A Pairs
One of the most effective ways to combat the cold start problem is by generating synthetic Q&A pairs. This involves creating artificial data that can help train your model and improve its initial performance.
Example:
# Function to generate synthetic Q&A pairs
def generate_synthetic_pairs(num_pairs):
synthetic_pairs = []
for _ in range(num_pairs):
question = f"Sample question {_}?"
answer = f"Sample answer for question {_}."
synthetic_pairs.append((question, answer))
return synthetic_pairs
# Generate synthetic pairs
synthetic_data = generate_synthetic_pairs(100)
print(synthetic_data[:5])
Using Pre-trained Models
Leveraging pre-trained models can also help mitigate the cold start problem. These models have already been trained on large datasets and can provide a strong foundation for your application.
Example:
from transformers import AutoModelForQuestionAnswering, AutoTokenizer
# Load a pre-trained model and tokenizer
tokenizer = AutoTokenizer.from_pretrained('bert-large-uncased-whole-word-masking-finetuned-squad')
model = AutoModelForQuestionAnswering.from_pretrained('bert-large-uncased-whole-word-masking-finetuned-squad')
# Function to use pre-trained model for answering questions
def answer_question(question, context):
inputs = tokenizer.encode_plus(question, context, add_special_tokens=True, return_tensors='pt')
answer_start_scores, answer_end_scores = model(**inputs)
answer_start = torch.argmax(answer_start_scores)
answer_end = torch.argmax(answer_end_scores) + 1
answer = tokenizer.convert_tokens_to_string(tokenizer.convert_ids_to_tokens(inputs['input_ids'][0][answer_start:answer_end]))
return answer
# Example usage
context = "New Jersey's capital is Trenton."
question = "What is the capital of New Jersey?"
answer = answer_question(question, context)
print(answer)
Pre-training with Domain-Specific Data
Another effective strategy is to pre-train your model with domain-specific data. This approach ensures that the model is familiar with the specific type of queries it will encounter, thereby improving its performance from the start.
Example:
from transformers import TextDataset, DataCollatorForLanguageModeling, Trainer, TrainingArguments
# Function to load domain-specific data for pre-training
def load_dataset(file_path):
return TextDataset(tokenizer=tokenizer, file_path=file_path, block_size=128)
# Load the dataset
dataset = load_dataset('domain_specific_data.txt')
# Data collator
data_collator = DataCollatorForLanguageModeling(tokenizer=tokenizer, mlm=True)
# Training arguments
training_args = TrainingArguments(
output_dir='./results',
overwrite_output_dir=True,
num_train_epochs=3,
per_device_train_batch_size=8,
save_steps=10_000,
save_total_limit=2,
)
# Trainer
trainer = Trainer(
model=model,
args=training_args,
data_collator=data_collator,
train_dataset=dataset,
)
# Train the model
trainer.train()
Diagram of Cold Start Mitigation Strategies:
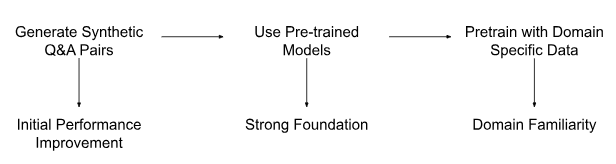
Employing these strategies can effectively address the cold start problem and ensure your RAG application performs well right from the beginning.
Read this case study on enhancing reliability with guardrails.
Next, we will explore how to experiment with different configurations and fine-tune the system for optimal performance.
Experimentation and Improvement
After addressing the cold start problem, the next step is to refine and enhance your RAG application. Using a RAG LLM example, experimentation and continuous improvement are vital to optimizing performance and ensuring the application meets your needs. This involves testing various configurations, models, and techniques to find the best setup. Let's dive into these processes.
Testing Different Chunk Sizes
The size of the text chunks used in your RAG application can significantly impact performance. Experimenting with different chunk sizes helps find the optimal balance between retrieval accuracy and processing efficiency.
Example:
# Function to experiment with different chunk sizes
def test_chunk_sizes(text, sizes=[128, 256, 512]):
results = {}
for size in sizes:
chunks = chunk_text(text, chunk_size=size)
embeddings = [embed_text(chunk) for chunk in chunks]
results[size] = embeddings
return results
# Sample text for chunking
text = "New Jersey's capital is Trenton. Python is a versatile programming language."
# Test different chunk sizes
chunk_results = test_chunk_sizes(text)
for size, embeddings in chunk_results.items():
print(f"Chunk Size: {size}, Number of Chunks: {len(embeddings)}")
Experimenting with Embedding Models
Different embedding models can produce varying results in terms of accuracy and performance. Testing multiple models helps identify which one works best for your specific use case.
Example:
from transformers import AutoModel
# Function to test different embedding models
def test_embedding_models(text, models=['bert-base-uncased', 'roberta-base']):
results = {}
for model_name in models:
tokenizer = AutoTokenizer.from_pretrained(model_name)
model = AutoModel.from_pretrained(model_name)
inputs = tokenizer(text, return_tensors='pt', truncation=True, padding=True)
outputs = model(**inputs)
embeddings = outputs.last_hidden_state.mean(dim=1).detach().numpy()
results[model_name] = embeddings
return results
# Test text for embeddings
test_text = "Machine learning is a branch of artificial intelligence."
# Test different embedding models
embedding_results = test_embedding_models(test_text)
for model_name, embedding in embedding_results.items():
print(f"Model: {model_name}, Embedding Shape: {embedding.shape}")
Fine-Tuning for Better Context Representation
Fine-tuning your models with domain-specific data can significantly improve their ability to represent context accurately. This process involves training the model on data that is similar to what it will encounter in actual use.
Example:
from transformers import Trainer, TrainingArguments
# Function to fine-tune a model
def fine_tune_model(model_name, dataset_path):
tokenizer = AutoTokenizer.from_pretrained(model_name)
model = AutoModel.from_pretrained(model_name)
# Load dataset
dataset = load_dataset(dataset_path)
# Data collator
data_collator = DataCollatorForLanguageModeling(tokenizer=tokenizer, mlm=True)
# Training arguments
training_args = TrainingArguments(
output_dir='./results',
num_train_epochs=3,
per_device_train_batch_size=8,
save_steps=10_000,
save_total_limit=2,
)
# Trainer
trainer = Trainer(
model=model,
args=training_args,
data_collator=data_collator,
train_dataset=dataset,
)
# Train the model
trainer.train()
return model
# Fine-tune the model with domain-specific data
fine_tuned_model = fine_tune_model('bert-base-uncased', 'domain_specific_data.txt')
Diagram of Experimentation and Improvement Workflow:
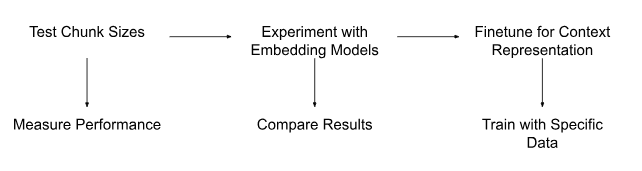
By experimenting with different configurations and models and fine-tuning your system, you can continuously improve the performance of your RAG application. This iterative process ensures that the application remains effective and efficient. Next, we will discuss examples of RAG LLM techniques for serving and scaling your RAG application to handle real-world demands.
Application Serving, Scaling, and Continuous Improvement
Building a robust RAG application is only the beginning. To make it truly useful, you must serve it efficiently, scale it to meet demand, and continuously improve it based on user feedback and performance metrics. Let’s delve into these aspects.
Serving the RAG Application
Serving your RAG application means making it accessible for users to query. This typically involves setting up an API endpoint that handles incoming requests and returns generated responses.
Example:
from flask import Flask, request, jsonify
app = Flask(__name__)
# Load the model and other necessary components
query_rag = setup_rag_application(data_dir, tokenizer, model, generator, index)
@app.route('/query', methods=['POST'])
def query():
data = request.get_json()
query_text = data['query']
response = query_rag(query_text)
return jsonify({'response': response})
if __name__ == '__main__':
app.run(debug=True)
Scaling the RAG Application
To handle multiple queries simultaneously and ensure fast response times, you need to scale your application. Using a RAG LLM example, this involves deploying your application on a robust infrastructure that can manage high traffic and distribute load effectively.
Example:
Horizontal Scaling: Deploy multiple instances of your application and use a load balancer to distribute traffic.
Vertical Scaling: Increase the computational resources (CPU, RAM) of your existing server to handle more requests.
Techniques for Scaling:
Containerization: Use Docker to containerize your application, making it easy to deploy and scale across different environments.
Example:
# Dockerfile for RAG application
FROM python:3.8-slim
WORKDIR /app
COPY requirements.txt requirements.txt
RUN pip install -r requirements.txt
COPY . .
CMD ["python", "app.py"]
Orchestration: Use Kubernetes to manage and scale your containerized application.
Example:
apiVersion: apps/v1
kind: Deployment
metadata:
name: rag-deployment
spec:
replicas: 3
selector:
matchLabels:
app: rag-app
template:
metadata:
labels:
app: rag-app
spec:
containers:
- name: rag-container
image: rag-image
ports:
- containerPort: 5000
Continuous Improvement
To ensure your application remains effective, continuous improvement is crucial. This involves regularly updating your models, refining data, and incorporating user feedback.
Strategies for Continuous Improvement:
Monitor Performance: Regularly track key metrics such as response time, accuracy, and user satisfaction.
Example of Monitoring Metrics:

Feedback Loop: Implement a feedback mechanism where users can rate responses and provide comments. Use this feedback to improve your models and data.
Regular Updates: Schedule regular updates for your models and data to incorporate the latest information and improvements.
Diagram of Application Serving and Scaling Workflow:
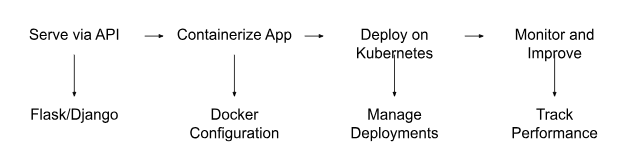
By efficiently serving, scaling, and continuously improving your RAG application, you ensure it remains reliable, responsive, and effective. Next, we will summarize the entire process and discuss the future prospects of integrating RAG into LLM applications.
Conclusion
Building a Retrieval Augmented Generation (RAG) application is a comprehensive process that involves several crucial steps. Using a RAG LLM example, you'll start by understanding the basics of RAG, preparing your database, processing data, building, implementing, and continuously improving your application. Each step plays a vital role in ensuring the success of your project. By following the detailed instructions provided, you can create a robust RAG application that delivers accurate and contextually relevant responses, enhancing the overall user experience.
Raga AI offers a state-of-the-art platform designed to simplify the development and deployment of AI applications. With features such as automated issue detection, diagnosis, and resolution, Raga AI ensures your applications are reliable and high-performing. If you're looking to enhance your AI projects with the latest in RAG technology, consider exploring Raga AI's comprehensive solutions. Start your journey with Raga AI today and take your AI applications to the next level.
If you've been exploring the world of large language models (LLMs), you've likely encountered their impressive capabilities and notable limitations. One of the significant challenges with LLMs is their tendency to produce hallucinations or inaccurate information, especially when generating responses without sufficient contextual grounding.
This is where Retrieval Augmented Generation (RAG) comes in. For a great RAG LLM example, RAG enhances LLMs by integrating them with external databases, allowing the model to retrieve relevant information and use it to generate more accurate and contextually appropriate responses. This technique not only improves the quality of the generated content but also significantly reduces the risk of hallucinations, making LLM applications more reliable and effective.
In this guide, you'll learn how to build your own RAG-based LLM application from scratch. We'll start with a clear definition of what RAG is and why it's essential for addressing some of the common issues associated with LLMs. For a practical Rag LLM example, we'll walk you through preparing your database, processing the necessary data, and implementing the RAG application. By the end of this guide, you'll have a solid understanding of integrating RAG into your LLM applications, enhancing their performance and reliability. Now, let's dive into understanding RAG and how it tackles the hallucination problem in LLMs.
Understanding RAG
Retrieval Augmented Generation (RAG) is a powerful technique designed to address some limitations of large language models (LLMs). By integrating external knowledge sources, RAG enhances the accuracy and contextual relevance of the generated responses. Let's explain how RAG works and why it's a valuable addition to LLM applications.
How RAG Tackles the Hallucination Problem
One of the biggest issues with LLMs is their tendency to produce hallucinations or responses that seem plausible but are factually incorrect. RAG addresses this by pulling in relevant information from external databases before generating a response. This means the model can access accurate and up-to-date information, significantly reducing the likelihood of hallucinations.
Learn more about LLM hallucinations in this article.
Example:
Consider a query about the capital of New Jersey. Based on its training data, an LLM might generate a wrong answer. However, with RAG, the model retrieves information from a reliable source, ensuring the response is accurate.
from transformers import pipeline, RagTokenizer, RagRetriever, RagTokenForGeneration
# Initialize tokenizer and retriever
tokenizer = RagTokenizer.from_pretrained("facebook/rag-token-base")
retriever = RagRetriever.from_pretrained("facebook/rag-token-base")
# Initialize model
model = RagTokenForGeneration.from_pretrained("facebook/rag-token-base", retriever=retriever)
# Input query
query = "What is the capital of New Jersey?"
# Generate response
input_ids = tokenizer(query, return_tensors="pt").input_ids
outputs = model.generate(input_ids=input_ids)
answer = tokenizer.decode(outputs[0], skip_special_tokens=True)
print(answer)
The Architectural Overview of RAG
RAG combines the strengths of generative models with the precision of retrieval systems. Here's a high-level overview of its architecture:
Retrieval Component: This part of the system searches external databases for relevant information based on the input query. The retrieved documents or data chunks are then fed into the generative model.
Generative Component: Using the retrieved information, the generative model creates a response that is both accurate and contextually appropriate.
Diagram of RAG Architecture:
Practical Benefits of RAG
Improved Accuracy: By integrating external data sources, RAG ensures responses are grounded in factual information.
Enhanced Context: RAG provides additional context to LLMs, making responses more relevant and informative.
Versatility: RAG can be applied to various applications, from customer support chatbots to content generation.
Key Components of RAG
Database Preparation: Ensuring that the external knowledge source is comprehensive and regularly updated.
Data Processing: Efficiently extracting, chunking, and embedding data for quick retrieval.
Integration: Seamlessly combining the retrieval and generative components to work in harmony.
By understanding the foundational principles of RAG, you're now equipped to explore its implementation in your LLM applications. To give you a clear RAG LLM example, we'll next dive into the specifics of preparing your database for RAG.
Preparing the Database for RAG
Setting up a robust database is crucial for the success of your Retrieval Augmented Generation (RAG) application. The database is the backbone, providing the external knowledge needed to generate accurate and contextually relevant responses. Let's break down the steps involved in preparing your database for RAG.
Loading Data into a Local Directory
The first step in preparing your database is to load your data into a local directory. This data can come from various sources such as text files, PDFs, or structured data files like CSVs. Here’s a simple example of how to load text data into a directory.
Example:
import os
# Define the directory path
data_dir = 'rag_data'
# Create the directory if it doesn't exist
if not os.path.exists(data_dir):
os.makedirs(data_dir)
# Sample data
documents = [
"New Jersey's capital is Trenton.",
"Python is a versatile programming language."
]
# Save each document as a separate text file
for i, doc in enumerate(documents):
with open(os.path.join(data_dir, f'doc_{i}.txt'), 'w') as f:
f.write(doc)
Creating Scalable Datasets
Once your data is loaded, the next step is to create scalable datasets that can be efficiently processed. This involves structuring your data in a way that allows for quick retrieval and minimal processing time.
Steps:
Chunking Data: Break down large documents into smaller, manageable chunks.
Embedding Data: Convert text chunks into numerical vectors using pre-trained models.
Example:
from transformers import AutoTokenizer, AutoModel
# Load a pre-trained tokenizer and model
tokenizer = AutoTokenizer.from_pretrained('bert-base-uncased')
model = AutoModel.from_pretrained('bert-base-uncased')
# Function to chunk text
def chunk_text(text, chunk_size=512):
tokens = tokenizer.tokenize(text)
chunks = [tokens[i:i + chunk_size] for i in range(0, len(tokens), chunk_size)]
return [' '.join(chunk) for chunk in chunks]
# Function to embed text
def embed_text(text):
inputs = tokenizer(text, return_tensors='pt')
outputs = model(**inputs)
return outputs.last_hidden_state.mean(dim=1).detach().numpy()
# Example usage
chunks = chunk_text(documents[0])
embeddings = [embed_text(chunk) for chunk in chunks]
Indexing Chunks for Rapid Retrieval
Indexing your data chunks is vital for quick retrieval during query processing. You can use various indexing techniques, such as inverted indices or vector databases, to achieve this.
Example:
from sklearn.neighbors import NearestNeighbors
import numpy as np
# Create an index of embeddings
index = NearestNeighbors(n_neighbors=1, algorithm='ball_tree')
index.fit(np.vstack(embeddings))
# Save the index
import joblib
joblib.dump(index, 'rag_index.pkl')
Diagram of the Data Preparation Process
By ensuring your database is well-prepared and efficiently indexed, you set a solid foundation for your RAG application.
Next, we’ll examine the data processing for RAG, which includes extracting, chunking, and embedding data sections.
Processing Data for RAG
Once you have your data loaded and organized, the next step is to process it for use in your Retrieval Augmented Generation (RAG) application. This involves extracting relevant information, chunking it into manageable pieces, and embedding these chunks for efficient retrieval. Let's dive into these processes in detail to illustrate with a RAG LLM example.
Extracting Data
Extracting data means pulling relevant information from your sources. Depending on your data format, this might involve parsing text files, scraping web content, or querying databases.
Example:
import os
# Directory containing the data
data_dir = 'rag_data'
# Function to read text files from the directory
def read_files(directory):
documents = []
for filename in os.listdir(directory):
if filename.endswith('.txt'):
with open(os.path.join(directory, filename), 'r') as f:
documents.append(f.read())
return documents
# Read data
documents = read_files(data_dir)
print(documents)
Chunking Data
Chunking involves breaking down large documents into smaller, more manageable pieces. This is important for both efficiency and accuracy, as smaller chunks are easier to process and retrieve.
Example:
def chunk_text(text, chunk_size=512):
tokens = tokenizer.tokenize(text)
chunks = [tokens[i:i + chunk_size] for i in range(0, len(tokens), chunk_size)]
return [' '.join(chunk) for chunk in chunks]
# Chunk all documents
chunked_documents = [chunk_text(doc) for doc in documents]
print(chunked_documents)
Embedding Data
Embedding converts text chunks into numerical vectors that can be used for efficient retrieval. Using a pre-trained model, you can transform each chunk into a vector representation.
Example:
from transformers import AutoTokenizer, AutoModel
import torch
# Load a pre-trained tokenizer and model
tokenizer = AutoTokenizer.from_pretrained('bert-base-uncased')
model = AutoModel.from_pretrained('bert-base-uncased')
# Function to embed text
def embed_text(text):
inputs = tokenizer(text, return_tensors='pt', truncation=True, padding=True)
outputs = model(**inputs)
return outputs.last_hidden_state.mean(dim=1).detach().numpy()
# Embed all chunks
embeddings = [embed_text(chunk) for doc in chunked_documents for chunk in doc]
print(embeddings)
Indexing Chunks
Indexing the embedded chunks allows for quick retrieval during the query process. Various indexing techniques can be used, such as using k-nearest neighbors for efficient lookups.
Example:
from sklearn.neighbors import NearestNeighbors
import numpy as np
# Flatten the list of embeddings for indexing
flat_embeddings = np.vstack(embeddings)
# Create an index
index = NearestNeighbors(n_neighbors=5, algorithm='ball_tree')
index.fit(flat_embeddings)
# Save the index for later use
import joblib
joblib.dump(index, 'rag_index.pkl')
Diagram of Data Processing Workflow:
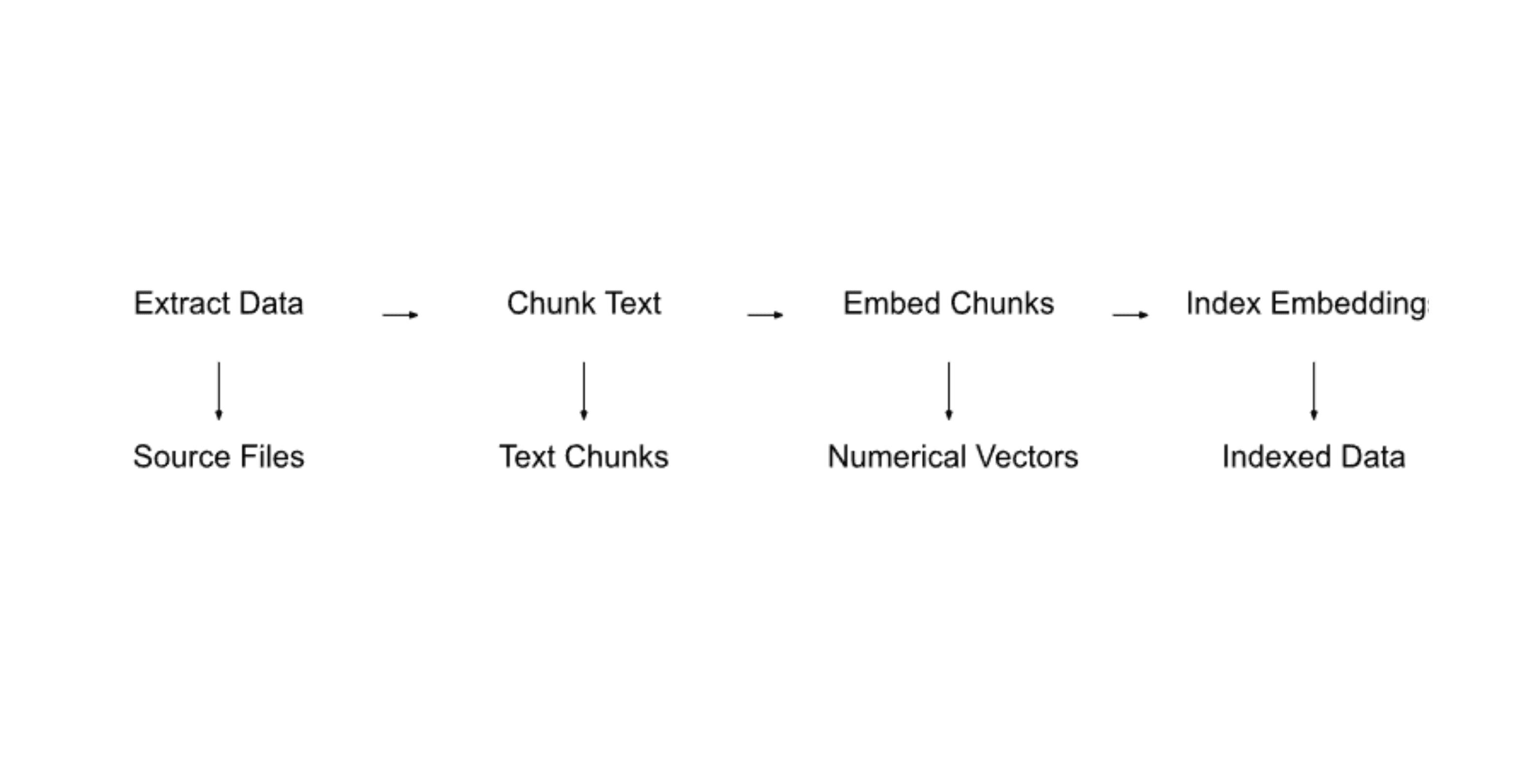
By efficiently processing your data through extraction, chunking, embedding, and indexing, you set the stage for building a powerful RAG application. Next, we will focus on implementing the RAG application, including query retrieval and response generation.
Learn more about the LLM parameters.
Building the RAG Application
Now that your data is processed and ready, it's time to build the Retrieval Augmented Generation (RAG) application. This involves setting up the system for query retrieval, generating responses using the embedded data, and optimizing the application for performance. Let's break down the steps to build your RAG application.
Implementing Query Retrieval
The first step in building your RAG application is implementing the query retrieval system. This system will handle user queries and retrieve relevant data chunks from the indexed database.
Example:
# Function to retrieve relevant data chunks for a query
def retrieve_chunks(query, index, tokenizer, model):
query_embedding = embed_text(query)
distances, indices = index.kneighbors(query_embedding)
return indices[0]
# Example query
query = "What is the capital of New Jersey?"
retrieved_indices = retrieve_chunks(query, index, tokenizer, model)
print(retrieved_indices)
Generating Responses
Once the relevant data chunks are retrieved, the next step is to generate a response using the LLM. The retrieved data provides context, helping the model to produce accurate and relevant answers.
Example:
from transformers import pipeline
# Load a pre-trained generative model
generator = pipeline('text-generation', model='gpt-3.5-turbo')
# Function to generate a response using the retrieved chunks
def generate_response(query, retrieved_indices, data_dir):
context = []
for idx in retrieved_indices:
with open(os.path.join(data_dir, f'doc_{idx}.txt'), 'r') as f:
context.append(f.read())
context = ' '.join(context)
response = generator(f"Context: {context} Query: {query}", max_length=100)
return response[0]['generated_text']
# Generate a response
response = generate_response(query, retrieved_indices, data_dir)
print(response)
Configurations and Optimizations
To ensure your RAG application runs efficiently, you need to configure and optimize various system aspects. This includes tuning the retrieval process and the response generation model.
Example:
# Configurations for query retrieval
NUM_NEIGHBORS = 5 # Number of nearest neighbors to retrieve
# Optimizations for response generation
GENERATION_MAX_LENGTH = 150 # Maximum length of generated responses
# Function to optimize retrieval and generation
def optimized_generate_response(query, index, tokenizer, model, generator, data_dir):
query_embedding = embed_text(query)
distances, indices = index.kneighbors(query_embedding, n_neighbors=NUM_NEIGHBORS)
context = []
for idx in indices[0]:
with open(os.path.join(data_dir, f'doc_{idx}.txt'), 'r') as f:
context.append(f.read())
context = ' '.join(context)
response = generator(f"Context: {context} Query: {query}", max_length=GENERATION_MAX_LENGTH)
return response[0]['generated_text']
# Generate an optimized response
optimized_response = optimized_generate_response(query, index, tokenizer, model, generator, data_dir)
print(optimized_response)
Diagram of the RAG Application Workflow:
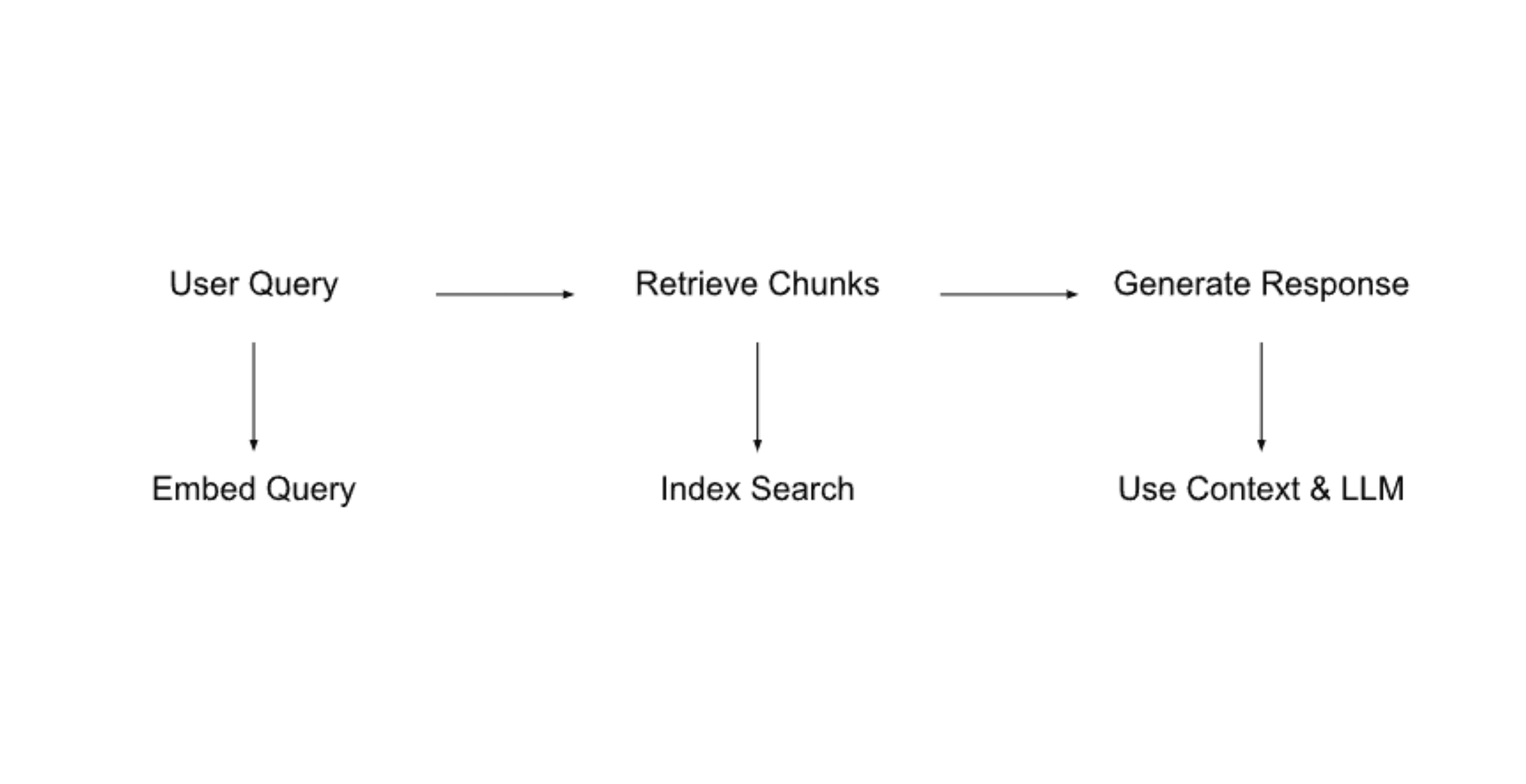
By carefully implementing query retrieval, generating responses with context, and optimizing your configurations, you create a robust RAG application.
To learn more, visit this webinar on building RAG applications and ensuring safe and reliable GenAI.
Next, we will look at how to implement and test the RAG application to ensure it functions correctly.
Implementing and Testing the RAG Application
With your RAG application built, the next crucial step is to implement it and ensure it works correctly. This involves setting up the application for querying, running tests to validate its performance, and fine-tuning as necessary. To illustrate with a RAG LLM example, let's go through these steps in detail.
Setting Up the RAG Application for Querying
The initial implementation step is to set up the RAG application so it can handle queries effectively. This involves integrating all components and ensuring they work together seamlessly.
Example:
# Function to set up the RAG application
def setup_rag_application(data_dir, tokenizer, model, generator, index):
def query_rag(query):
query_embedding = embed_text(query)
distances, indices = index.kneighbors(query_embedding, n_neighbors=5)
context = []
for idx in indices[0]:
with open(os.path.join(data_dir, f'doc_{idx}.txt'), 'r') as f:
context.append(f.read())
context = ' '.join(context)
response = generator(f"Context: {context} Query: {query}", max_length=150)
return response[0]['generated_text']
return query_rag
# Initialize the RAG application
query_rag = setup_rag_application(data_dir, tokenizer, model, generator, index)
Examples and Validation
Testing your RAG application with different queries is essential to validate its performance. By running several tests, you can ensure the system retrieves relevant information and generates accurate responses.
Example Query Test:
# Test the RAG application with a sample query
sample_query = "What is the capital of New Jersey?"
response = query_rag(sample_query)
print(f"Query: {sample_query}\nResponse: {response}")
Validation with Multiple Queries
To thoroughly test the application, use a set of diverse queries and evaluate the responses.
Example:
# List of sample queries
queries = [
"Who wrote 'Pride and Prejudice'?",
"What is the boiling point of water?",
"Define machine learning."
]
# Validate responses
for query in queries:
response = query_rag(query)
print(f"Query: {query}\nResponse: {response}\n")
Performance Metrics:

To quantify the effectiveness of your RAG application, consider using performance metrics such as accuracy, response time, and user satisfaction.
Example of Performance Metrics:
Diagram of Implementation and Testing Workflow:
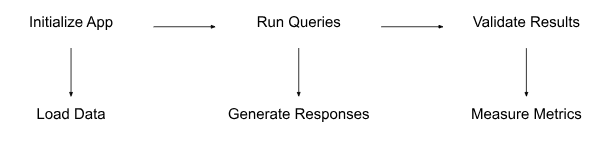
Raga AI’s testing platform is a comprehensive tool that automates the process to ensure you don’t have any data, model, or operational issues. Check out how it is the future of AI testing.
By systematically setting up, implementing, and validating your RAG application, you ensure it performs effectively and meets user needs. Next, we will explore optimizing and evaluating the RAG implementation to enhance performance.
Optimizing and Evaluating the RAG Implementation
Once your RAG application is up and running, the next step is to optimize its performance and evaluate its effectiveness. This ensures that the application not only works but works well. Using a RAG LLM example, optimization and evaluation involve tweaking configurations, conducting thorough assessments, and refining the system based on feedback. Let's explore these processes in detail.
Exploring Different Configurations
Experimenting with different configurations can significantly impact the performance of your RAG application. Adjusting parameters like chunk size, embedding model, and query retrieval settings can enhance efficiency and accuracy.
Example:
# Adjust configurations
CHUNK_SIZE = 256 # Smaller chunks for more precise retrieval
NUM_NEIGHBORS = 3 # Fewer neighbors for faster response
# Function to adjust configurations
def configure_rag(chunk_size, num_neighbors):
# Update chunking and retrieval settings
def chunk_text(text, chunk_size=chunk_size):
tokens = tokenizer.tokenize(text)
chunks = [tokens[i:i + chunk_size] for i in range(0, len(tokens), chunk_size)]
return [' '.join(chunk) for chunk in chunks]
def query_rag(query):
query_embedding = embed_text(query)
distances, indices = index.kneighbors(query_embedding, n_neighbors=num_neighbors)
context = []
for idx in indices[0]:
with open(os.path.join(data_dir, f'doc_{idx}.txt'), 'r') as f:
context.append(f.read())
context = ' '.join(context)
response = generator(f"Context: {context} Query: {query}", max_length=150)
return response[0]['generated_text']
return query_rag
# Apply new configurations
query_rag = configure_rag(CHUNK_SIZE, NUM_NEIGHBORS)
Conducting Evaluations
Systematic evaluations are crucial for understanding how well your RAG application performs. Evaluations can be conducted for both individual components and the entire system.
Example:
# List of sample queries for evaluation
queries = [
"What is the capital of New Jersey?",
"Who wrote 'To Kill a Mockingbird'?",
"Explain the theory of relativity."
]
# Evaluate responses
responses = [query_rag(query) for query in queries]
for query, response in zip(queries, responses):
print(f"Query: {query}\nResponse: {response}\n")
Methods to Quantitatively Assess Generative Tasks
To ensure your RAG application is functioning optimally, assess its performance using quantitative metrics. Common metrics include precision, recall, F1 score, and response time.
Example of Performance Metrics:

Diagram of Optimization and Evaluation Workflow:
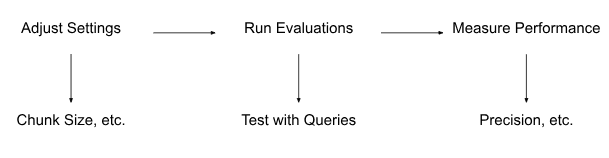
By methodically optimizing configurations and conducting detailed evaluations, you can significantly enhance the performance of your RAG application. Using a RAG LLM example, this approach ensures that it meets the required standards for efficiency and accuracy. Next, we will explore strategies for addressing the cold start problem, which is crucial for maintaining high performance from the start.
Addressing the Cold Start Problem
The cold start problem can be a significant hurdle when implementing a RAG application. It refers to the challenge of getting your model to perform well with little or no initial data. This issue can affect both the quality of responses and the overall user experience. Using a RAG LLM example, here’s how you can address this problem effectively.
Generating Synthetic Q&A Pairs
One of the most effective ways to combat the cold start problem is by generating synthetic Q&A pairs. This involves creating artificial data that can help train your model and improve its initial performance.
Example:
# Function to generate synthetic Q&A pairs
def generate_synthetic_pairs(num_pairs):
synthetic_pairs = []
for _ in range(num_pairs):
question = f"Sample question {_}?"
answer = f"Sample answer for question {_}."
synthetic_pairs.append((question, answer))
return synthetic_pairs
# Generate synthetic pairs
synthetic_data = generate_synthetic_pairs(100)
print(synthetic_data[:5])
Using Pre-trained Models
Leveraging pre-trained models can also help mitigate the cold start problem. These models have already been trained on large datasets and can provide a strong foundation for your application.
Example:
from transformers import AutoModelForQuestionAnswering, AutoTokenizer
# Load a pre-trained model and tokenizer
tokenizer = AutoTokenizer.from_pretrained('bert-large-uncased-whole-word-masking-finetuned-squad')
model = AutoModelForQuestionAnswering.from_pretrained('bert-large-uncased-whole-word-masking-finetuned-squad')
# Function to use pre-trained model for answering questions
def answer_question(question, context):
inputs = tokenizer.encode_plus(question, context, add_special_tokens=True, return_tensors='pt')
answer_start_scores, answer_end_scores = model(**inputs)
answer_start = torch.argmax(answer_start_scores)
answer_end = torch.argmax(answer_end_scores) + 1
answer = tokenizer.convert_tokens_to_string(tokenizer.convert_ids_to_tokens(inputs['input_ids'][0][answer_start:answer_end]))
return answer
# Example usage
context = "New Jersey's capital is Trenton."
question = "What is the capital of New Jersey?"
answer = answer_question(question, context)
print(answer)
Pre-training with Domain-Specific Data
Another effective strategy is to pre-train your model with domain-specific data. This approach ensures that the model is familiar with the specific type of queries it will encounter, thereby improving its performance from the start.
Example:
from transformers import TextDataset, DataCollatorForLanguageModeling, Trainer, TrainingArguments
# Function to load domain-specific data for pre-training
def load_dataset(file_path):
return TextDataset(tokenizer=tokenizer, file_path=file_path, block_size=128)
# Load the dataset
dataset = load_dataset('domain_specific_data.txt')
# Data collator
data_collator = DataCollatorForLanguageModeling(tokenizer=tokenizer, mlm=True)
# Training arguments
training_args = TrainingArguments(
output_dir='./results',
overwrite_output_dir=True,
num_train_epochs=3,
per_device_train_batch_size=8,
save_steps=10_000,
save_total_limit=2,
)
# Trainer
trainer = Trainer(
model=model,
args=training_args,
data_collator=data_collator,
train_dataset=dataset,
)
# Train the model
trainer.train()
Diagram of Cold Start Mitigation Strategies:
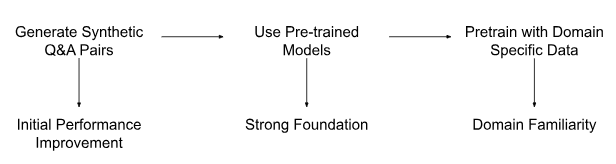
Employing these strategies can effectively address the cold start problem and ensure your RAG application performs well right from the beginning.
Read this case study on enhancing reliability with guardrails.
Next, we will explore how to experiment with different configurations and fine-tune the system for optimal performance.
Experimentation and Improvement
After addressing the cold start problem, the next step is to refine and enhance your RAG application. Using a RAG LLM example, experimentation and continuous improvement are vital to optimizing performance and ensuring the application meets your needs. This involves testing various configurations, models, and techniques to find the best setup. Let's dive into these processes.
Testing Different Chunk Sizes
The size of the text chunks used in your RAG application can significantly impact performance. Experimenting with different chunk sizes helps find the optimal balance between retrieval accuracy and processing efficiency.
Example:
# Function to experiment with different chunk sizes
def test_chunk_sizes(text, sizes=[128, 256, 512]):
results = {}
for size in sizes:
chunks = chunk_text(text, chunk_size=size)
embeddings = [embed_text(chunk) for chunk in chunks]
results[size] = embeddings
return results
# Sample text for chunking
text = "New Jersey's capital is Trenton. Python is a versatile programming language."
# Test different chunk sizes
chunk_results = test_chunk_sizes(text)
for size, embeddings in chunk_results.items():
print(f"Chunk Size: {size}, Number of Chunks: {len(embeddings)}")
Experimenting with Embedding Models
Different embedding models can produce varying results in terms of accuracy and performance. Testing multiple models helps identify which one works best for your specific use case.
Example:
from transformers import AutoModel
# Function to test different embedding models
def test_embedding_models(text, models=['bert-base-uncased', 'roberta-base']):
results = {}
for model_name in models:
tokenizer = AutoTokenizer.from_pretrained(model_name)
model = AutoModel.from_pretrained(model_name)
inputs = tokenizer(text, return_tensors='pt', truncation=True, padding=True)
outputs = model(**inputs)
embeddings = outputs.last_hidden_state.mean(dim=1).detach().numpy()
results[model_name] = embeddings
return results
# Test text for embeddings
test_text = "Machine learning is a branch of artificial intelligence."
# Test different embedding models
embedding_results = test_embedding_models(test_text)
for model_name, embedding in embedding_results.items():
print(f"Model: {model_name}, Embedding Shape: {embedding.shape}")
Fine-Tuning for Better Context Representation
Fine-tuning your models with domain-specific data can significantly improve their ability to represent context accurately. This process involves training the model on data that is similar to what it will encounter in actual use.
Example:
from transformers import Trainer, TrainingArguments
# Function to fine-tune a model
def fine_tune_model(model_name, dataset_path):
tokenizer = AutoTokenizer.from_pretrained(model_name)
model = AutoModel.from_pretrained(model_name)
# Load dataset
dataset = load_dataset(dataset_path)
# Data collator
data_collator = DataCollatorForLanguageModeling(tokenizer=tokenizer, mlm=True)
# Training arguments
training_args = TrainingArguments(
output_dir='./results',
num_train_epochs=3,
per_device_train_batch_size=8,
save_steps=10_000,
save_total_limit=2,
)
# Trainer
trainer = Trainer(
model=model,
args=training_args,
data_collator=data_collator,
train_dataset=dataset,
)
# Train the model
trainer.train()
return model
# Fine-tune the model with domain-specific data
fine_tuned_model = fine_tune_model('bert-base-uncased', 'domain_specific_data.txt')
Diagram of Experimentation and Improvement Workflow:
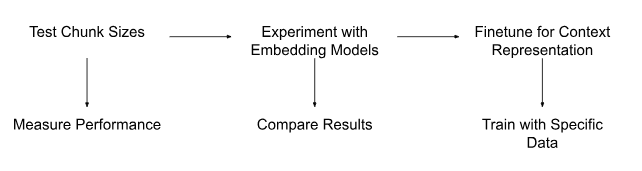
By experimenting with different configurations and models and fine-tuning your system, you can continuously improve the performance of your RAG application. This iterative process ensures that the application remains effective and efficient. Next, we will discuss examples of RAG LLM techniques for serving and scaling your RAG application to handle real-world demands.
Application Serving, Scaling, and Continuous Improvement
Building a robust RAG application is only the beginning. To make it truly useful, you must serve it efficiently, scale it to meet demand, and continuously improve it based on user feedback and performance metrics. Let’s delve into these aspects.
Serving the RAG Application
Serving your RAG application means making it accessible for users to query. This typically involves setting up an API endpoint that handles incoming requests and returns generated responses.
Example:
from flask import Flask, request, jsonify
app = Flask(__name__)
# Load the model and other necessary components
query_rag = setup_rag_application(data_dir, tokenizer, model, generator, index)
@app.route('/query', methods=['POST'])
def query():
data = request.get_json()
query_text = data['query']
response = query_rag(query_text)
return jsonify({'response': response})
if __name__ == '__main__':
app.run(debug=True)
Scaling the RAG Application
To handle multiple queries simultaneously and ensure fast response times, you need to scale your application. Using a RAG LLM example, this involves deploying your application on a robust infrastructure that can manage high traffic and distribute load effectively.
Example:
Horizontal Scaling: Deploy multiple instances of your application and use a load balancer to distribute traffic.
Vertical Scaling: Increase the computational resources (CPU, RAM) of your existing server to handle more requests.
Techniques for Scaling:
Containerization: Use Docker to containerize your application, making it easy to deploy and scale across different environments.
Example:
# Dockerfile for RAG application
FROM python:3.8-slim
WORKDIR /app
COPY requirements.txt requirements.txt
RUN pip install -r requirements.txt
COPY . .
CMD ["python", "app.py"]
Orchestration: Use Kubernetes to manage and scale your containerized application.
Example:
apiVersion: apps/v1
kind: Deployment
metadata:
name: rag-deployment
spec:
replicas: 3
selector:
matchLabels:
app: rag-app
template:
metadata:
labels:
app: rag-app
spec:
containers:
- name: rag-container
image: rag-image
ports:
- containerPort: 5000
Continuous Improvement
To ensure your application remains effective, continuous improvement is crucial. This involves regularly updating your models, refining data, and incorporating user feedback.
Strategies for Continuous Improvement:
Monitor Performance: Regularly track key metrics such as response time, accuracy, and user satisfaction.
Example of Monitoring Metrics:

Feedback Loop: Implement a feedback mechanism where users can rate responses and provide comments. Use this feedback to improve your models and data.
Regular Updates: Schedule regular updates for your models and data to incorporate the latest information and improvements.
Diagram of Application Serving and Scaling Workflow:
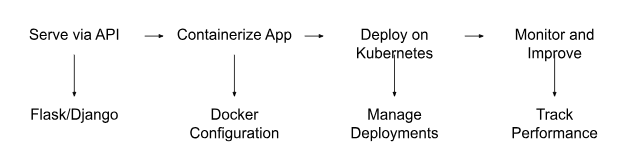
By efficiently serving, scaling, and continuously improving your RAG application, you ensure it remains reliable, responsive, and effective. Next, we will summarize the entire process and discuss the future prospects of integrating RAG into LLM applications.
Conclusion
Building a Retrieval Augmented Generation (RAG) application is a comprehensive process that involves several crucial steps. Using a RAG LLM example, you'll start by understanding the basics of RAG, preparing your database, processing data, building, implementing, and continuously improving your application. Each step plays a vital role in ensuring the success of your project. By following the detailed instructions provided, you can create a robust RAG application that delivers accurate and contextually relevant responses, enhancing the overall user experience.
Raga AI offers a state-of-the-art platform designed to simplify the development and deployment of AI applications. With features such as automated issue detection, diagnosis, and resolution, Raga AI ensures your applications are reliable and high-performing. If you're looking to enhance your AI projects with the latest in RAG technology, consider exploring Raga AI's comprehensive solutions. Start your journey with Raga AI today and take your AI applications to the next level.
If you've been exploring the world of large language models (LLMs), you've likely encountered their impressive capabilities and notable limitations. One of the significant challenges with LLMs is their tendency to produce hallucinations or inaccurate information, especially when generating responses without sufficient contextual grounding.
This is where Retrieval Augmented Generation (RAG) comes in. For a great RAG LLM example, RAG enhances LLMs by integrating them with external databases, allowing the model to retrieve relevant information and use it to generate more accurate and contextually appropriate responses. This technique not only improves the quality of the generated content but also significantly reduces the risk of hallucinations, making LLM applications more reliable and effective.
In this guide, you'll learn how to build your own RAG-based LLM application from scratch. We'll start with a clear definition of what RAG is and why it's essential for addressing some of the common issues associated with LLMs. For a practical Rag LLM example, we'll walk you through preparing your database, processing the necessary data, and implementing the RAG application. By the end of this guide, you'll have a solid understanding of integrating RAG into your LLM applications, enhancing their performance and reliability. Now, let's dive into understanding RAG and how it tackles the hallucination problem in LLMs.
Understanding RAG
Retrieval Augmented Generation (RAG) is a powerful technique designed to address some limitations of large language models (LLMs). By integrating external knowledge sources, RAG enhances the accuracy and contextual relevance of the generated responses. Let's explain how RAG works and why it's a valuable addition to LLM applications.
How RAG Tackles the Hallucination Problem
One of the biggest issues with LLMs is their tendency to produce hallucinations or responses that seem plausible but are factually incorrect. RAG addresses this by pulling in relevant information from external databases before generating a response. This means the model can access accurate and up-to-date information, significantly reducing the likelihood of hallucinations.
Learn more about LLM hallucinations in this article.
Example:
Consider a query about the capital of New Jersey. Based on its training data, an LLM might generate a wrong answer. However, with RAG, the model retrieves information from a reliable source, ensuring the response is accurate.
from transformers import pipeline, RagTokenizer, RagRetriever, RagTokenForGeneration
# Initialize tokenizer and retriever
tokenizer = RagTokenizer.from_pretrained("facebook/rag-token-base")
retriever = RagRetriever.from_pretrained("facebook/rag-token-base")
# Initialize model
model = RagTokenForGeneration.from_pretrained("facebook/rag-token-base", retriever=retriever)
# Input query
query = "What is the capital of New Jersey?"
# Generate response
input_ids = tokenizer(query, return_tensors="pt").input_ids
outputs = model.generate(input_ids=input_ids)
answer = tokenizer.decode(outputs[0], skip_special_tokens=True)
print(answer)
The Architectural Overview of RAG
RAG combines the strengths of generative models with the precision of retrieval systems. Here's a high-level overview of its architecture:
Retrieval Component: This part of the system searches external databases for relevant information based on the input query. The retrieved documents or data chunks are then fed into the generative model.
Generative Component: Using the retrieved information, the generative model creates a response that is both accurate and contextually appropriate.
Diagram of RAG Architecture:
Practical Benefits of RAG
Improved Accuracy: By integrating external data sources, RAG ensures responses are grounded in factual information.
Enhanced Context: RAG provides additional context to LLMs, making responses more relevant and informative.
Versatility: RAG can be applied to various applications, from customer support chatbots to content generation.
Key Components of RAG
Database Preparation: Ensuring that the external knowledge source is comprehensive and regularly updated.
Data Processing: Efficiently extracting, chunking, and embedding data for quick retrieval.
Integration: Seamlessly combining the retrieval and generative components to work in harmony.
By understanding the foundational principles of RAG, you're now equipped to explore its implementation in your LLM applications. To give you a clear RAG LLM example, we'll next dive into the specifics of preparing your database for RAG.
Preparing the Database for RAG
Setting up a robust database is crucial for the success of your Retrieval Augmented Generation (RAG) application. The database is the backbone, providing the external knowledge needed to generate accurate and contextually relevant responses. Let's break down the steps involved in preparing your database for RAG.
Loading Data into a Local Directory
The first step in preparing your database is to load your data into a local directory. This data can come from various sources such as text files, PDFs, or structured data files like CSVs. Here’s a simple example of how to load text data into a directory.
Example:
import os
# Define the directory path
data_dir = 'rag_data'
# Create the directory if it doesn't exist
if not os.path.exists(data_dir):
os.makedirs(data_dir)
# Sample data
documents = [
"New Jersey's capital is Trenton.",
"Python is a versatile programming language."
]
# Save each document as a separate text file
for i, doc in enumerate(documents):
with open(os.path.join(data_dir, f'doc_{i}.txt'), 'w') as f:
f.write(doc)
Creating Scalable Datasets
Once your data is loaded, the next step is to create scalable datasets that can be efficiently processed. This involves structuring your data in a way that allows for quick retrieval and minimal processing time.
Steps:
Chunking Data: Break down large documents into smaller, manageable chunks.
Embedding Data: Convert text chunks into numerical vectors using pre-trained models.
Example:
from transformers import AutoTokenizer, AutoModel
# Load a pre-trained tokenizer and model
tokenizer = AutoTokenizer.from_pretrained('bert-base-uncased')
model = AutoModel.from_pretrained('bert-base-uncased')
# Function to chunk text
def chunk_text(text, chunk_size=512):
tokens = tokenizer.tokenize(text)
chunks = [tokens[i:i + chunk_size] for i in range(0, len(tokens), chunk_size)]
return [' '.join(chunk) for chunk in chunks]
# Function to embed text
def embed_text(text):
inputs = tokenizer(text, return_tensors='pt')
outputs = model(**inputs)
return outputs.last_hidden_state.mean(dim=1).detach().numpy()
# Example usage
chunks = chunk_text(documents[0])
embeddings = [embed_text(chunk) for chunk in chunks]
Indexing Chunks for Rapid Retrieval
Indexing your data chunks is vital for quick retrieval during query processing. You can use various indexing techniques, such as inverted indices or vector databases, to achieve this.
Example:
from sklearn.neighbors import NearestNeighbors
import numpy as np
# Create an index of embeddings
index = NearestNeighbors(n_neighbors=1, algorithm='ball_tree')
index.fit(np.vstack(embeddings))
# Save the index
import joblib
joblib.dump(index, 'rag_index.pkl')
Diagram of the Data Preparation Process
By ensuring your database is well-prepared and efficiently indexed, you set a solid foundation for your RAG application.
Next, we’ll examine the data processing for RAG, which includes extracting, chunking, and embedding data sections.
Processing Data for RAG
Once you have your data loaded and organized, the next step is to process it for use in your Retrieval Augmented Generation (RAG) application. This involves extracting relevant information, chunking it into manageable pieces, and embedding these chunks for efficient retrieval. Let's dive into these processes in detail to illustrate with a RAG LLM example.
Extracting Data
Extracting data means pulling relevant information from your sources. Depending on your data format, this might involve parsing text files, scraping web content, or querying databases.
Example:
import os
# Directory containing the data
data_dir = 'rag_data'
# Function to read text files from the directory
def read_files(directory):
documents = []
for filename in os.listdir(directory):
if filename.endswith('.txt'):
with open(os.path.join(directory, filename), 'r') as f:
documents.append(f.read())
return documents
# Read data
documents = read_files(data_dir)
print(documents)
Chunking Data
Chunking involves breaking down large documents into smaller, more manageable pieces. This is important for both efficiency and accuracy, as smaller chunks are easier to process and retrieve.
Example:
def chunk_text(text, chunk_size=512):
tokens = tokenizer.tokenize(text)
chunks = [tokens[i:i + chunk_size] for i in range(0, len(tokens), chunk_size)]
return [' '.join(chunk) for chunk in chunks]
# Chunk all documents
chunked_documents = [chunk_text(doc) for doc in documents]
print(chunked_documents)
Embedding Data
Embedding converts text chunks into numerical vectors that can be used for efficient retrieval. Using a pre-trained model, you can transform each chunk into a vector representation.
Example:
from transformers import AutoTokenizer, AutoModel
import torch
# Load a pre-trained tokenizer and model
tokenizer = AutoTokenizer.from_pretrained('bert-base-uncased')
model = AutoModel.from_pretrained('bert-base-uncased')
# Function to embed text
def embed_text(text):
inputs = tokenizer(text, return_tensors='pt', truncation=True, padding=True)
outputs = model(**inputs)
return outputs.last_hidden_state.mean(dim=1).detach().numpy()
# Embed all chunks
embeddings = [embed_text(chunk) for doc in chunked_documents for chunk in doc]
print(embeddings)
Indexing Chunks
Indexing the embedded chunks allows for quick retrieval during the query process. Various indexing techniques can be used, such as using k-nearest neighbors for efficient lookups.
Example:
from sklearn.neighbors import NearestNeighbors
import numpy as np
# Flatten the list of embeddings for indexing
flat_embeddings = np.vstack(embeddings)
# Create an index
index = NearestNeighbors(n_neighbors=5, algorithm='ball_tree')
index.fit(flat_embeddings)
# Save the index for later use
import joblib
joblib.dump(index, 'rag_index.pkl')
Diagram of Data Processing Workflow:
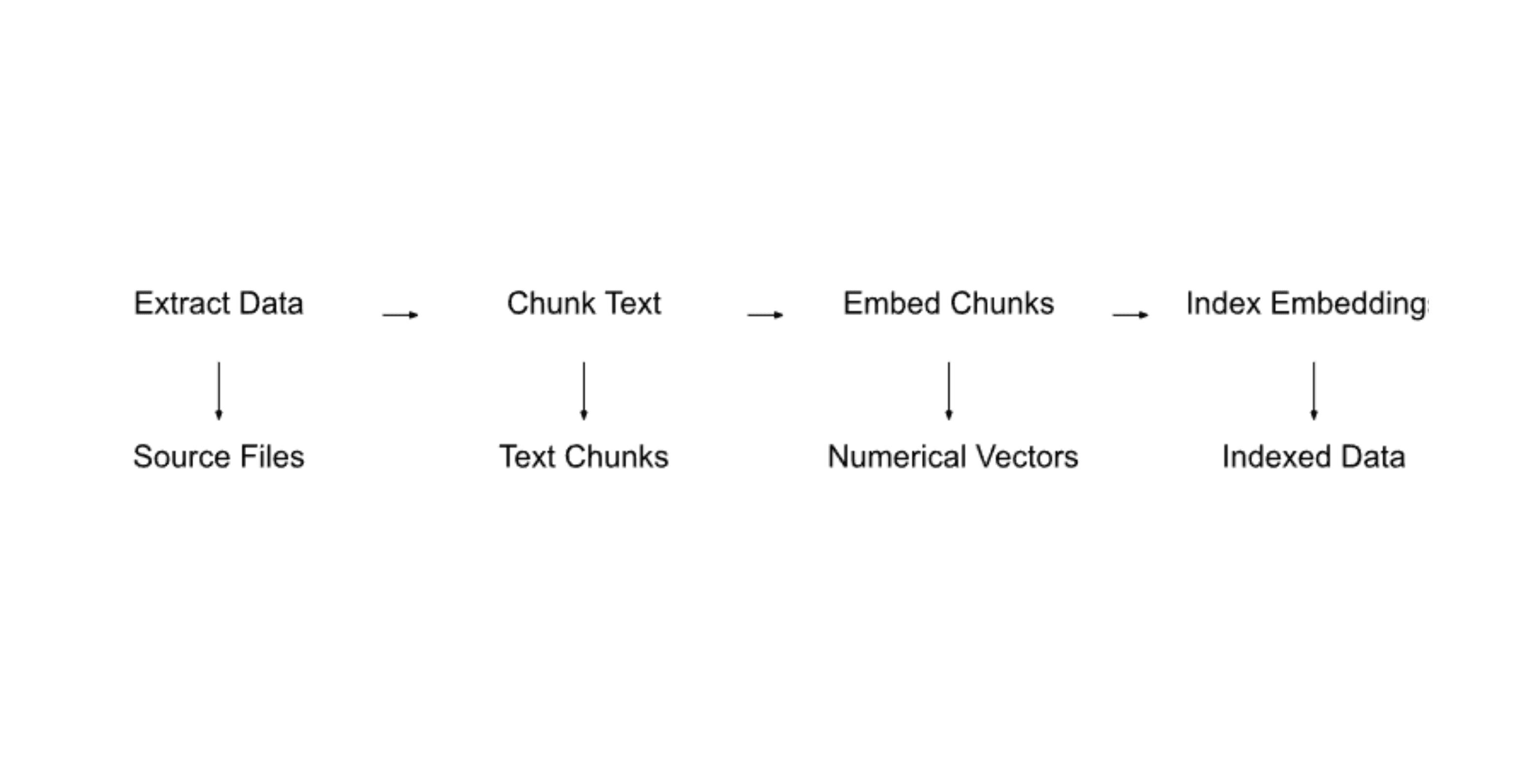
By efficiently processing your data through extraction, chunking, embedding, and indexing, you set the stage for building a powerful RAG application. Next, we will focus on implementing the RAG application, including query retrieval and response generation.
Learn more about the LLM parameters.
Building the RAG Application
Now that your data is processed and ready, it's time to build the Retrieval Augmented Generation (RAG) application. This involves setting up the system for query retrieval, generating responses using the embedded data, and optimizing the application for performance. Let's break down the steps to build your RAG application.
Implementing Query Retrieval
The first step in building your RAG application is implementing the query retrieval system. This system will handle user queries and retrieve relevant data chunks from the indexed database.
Example:
# Function to retrieve relevant data chunks for a query
def retrieve_chunks(query, index, tokenizer, model):
query_embedding = embed_text(query)
distances, indices = index.kneighbors(query_embedding)
return indices[0]
# Example query
query = "What is the capital of New Jersey?"
retrieved_indices = retrieve_chunks(query, index, tokenizer, model)
print(retrieved_indices)
Generating Responses
Once the relevant data chunks are retrieved, the next step is to generate a response using the LLM. The retrieved data provides context, helping the model to produce accurate and relevant answers.
Example:
from transformers import pipeline
# Load a pre-trained generative model
generator = pipeline('text-generation', model='gpt-3.5-turbo')
# Function to generate a response using the retrieved chunks
def generate_response(query, retrieved_indices, data_dir):
context = []
for idx in retrieved_indices:
with open(os.path.join(data_dir, f'doc_{idx}.txt'), 'r') as f:
context.append(f.read())
context = ' '.join(context)
response = generator(f"Context: {context} Query: {query}", max_length=100)
return response[0]['generated_text']
# Generate a response
response = generate_response(query, retrieved_indices, data_dir)
print(response)
Configurations and Optimizations
To ensure your RAG application runs efficiently, you need to configure and optimize various system aspects. This includes tuning the retrieval process and the response generation model.
Example:
# Configurations for query retrieval
NUM_NEIGHBORS = 5 # Number of nearest neighbors to retrieve
# Optimizations for response generation
GENERATION_MAX_LENGTH = 150 # Maximum length of generated responses
# Function to optimize retrieval and generation
def optimized_generate_response(query, index, tokenizer, model, generator, data_dir):
query_embedding = embed_text(query)
distances, indices = index.kneighbors(query_embedding, n_neighbors=NUM_NEIGHBORS)
context = []
for idx in indices[0]:
with open(os.path.join(data_dir, f'doc_{idx}.txt'), 'r') as f:
context.append(f.read())
context = ' '.join(context)
response = generator(f"Context: {context} Query: {query}", max_length=GENERATION_MAX_LENGTH)
return response[0]['generated_text']
# Generate an optimized response
optimized_response = optimized_generate_response(query, index, tokenizer, model, generator, data_dir)
print(optimized_response)
Diagram of the RAG Application Workflow:
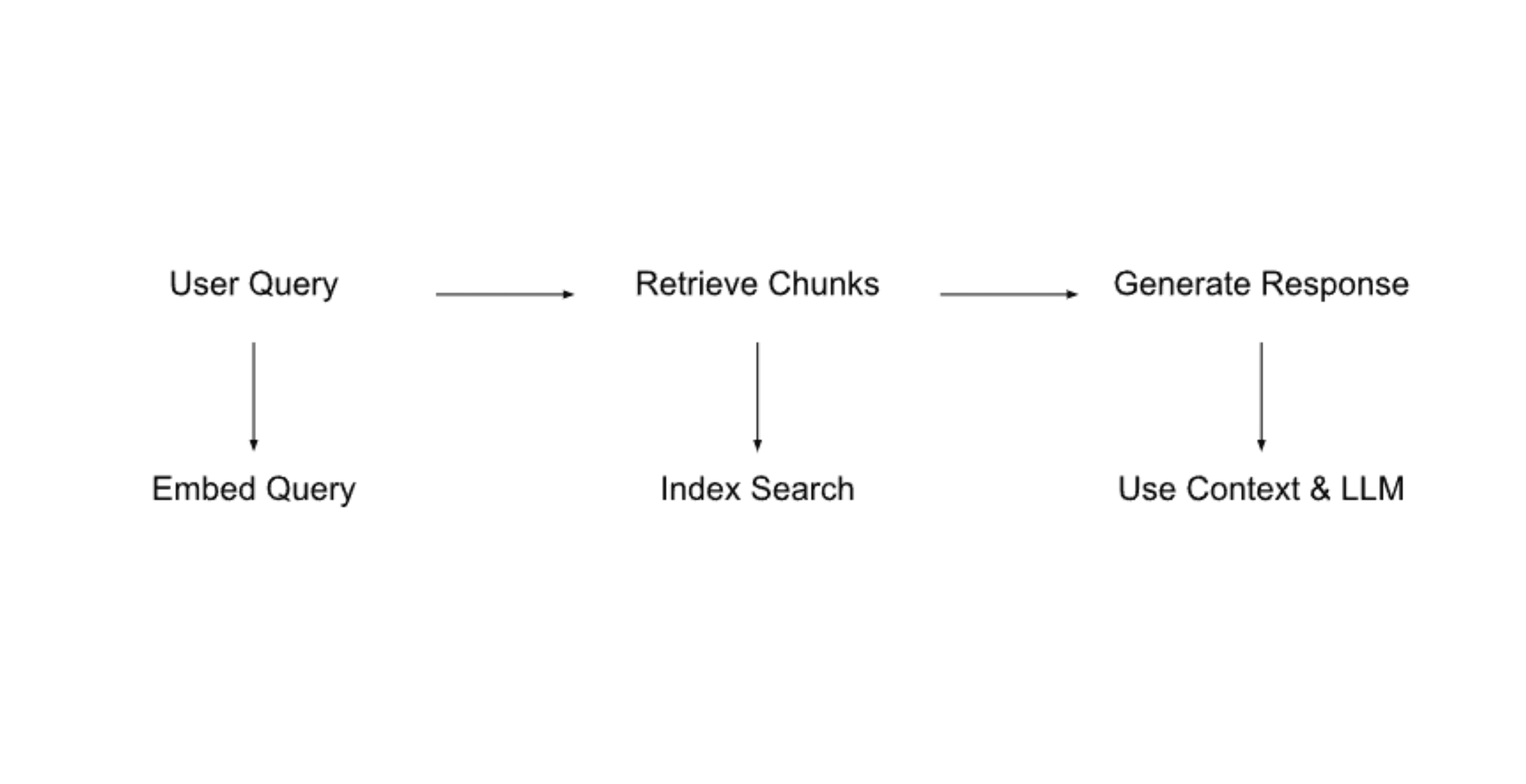
By carefully implementing query retrieval, generating responses with context, and optimizing your configurations, you create a robust RAG application.
To learn more, visit this webinar on building RAG applications and ensuring safe and reliable GenAI.
Next, we will look at how to implement and test the RAG application to ensure it functions correctly.
Implementing and Testing the RAG Application
With your RAG application built, the next crucial step is to implement it and ensure it works correctly. This involves setting up the application for querying, running tests to validate its performance, and fine-tuning as necessary. To illustrate with a RAG LLM example, let's go through these steps in detail.
Setting Up the RAG Application for Querying
The initial implementation step is to set up the RAG application so it can handle queries effectively. This involves integrating all components and ensuring they work together seamlessly.
Example:
# Function to set up the RAG application
def setup_rag_application(data_dir, tokenizer, model, generator, index):
def query_rag(query):
query_embedding = embed_text(query)
distances, indices = index.kneighbors(query_embedding, n_neighbors=5)
context = []
for idx in indices[0]:
with open(os.path.join(data_dir, f'doc_{idx}.txt'), 'r') as f:
context.append(f.read())
context = ' '.join(context)
response = generator(f"Context: {context} Query: {query}", max_length=150)
return response[0]['generated_text']
return query_rag
# Initialize the RAG application
query_rag = setup_rag_application(data_dir, tokenizer, model, generator, index)
Examples and Validation
Testing your RAG application with different queries is essential to validate its performance. By running several tests, you can ensure the system retrieves relevant information and generates accurate responses.
Example Query Test:
# Test the RAG application with a sample query
sample_query = "What is the capital of New Jersey?"
response = query_rag(sample_query)
print(f"Query: {sample_query}\nResponse: {response}")
Validation with Multiple Queries
To thoroughly test the application, use a set of diverse queries and evaluate the responses.
Example:
# List of sample queries
queries = [
"Who wrote 'Pride and Prejudice'?",
"What is the boiling point of water?",
"Define machine learning."
]
# Validate responses
for query in queries:
response = query_rag(query)
print(f"Query: {query}\nResponse: {response}\n")
Performance Metrics:

To quantify the effectiveness of your RAG application, consider using performance metrics such as accuracy, response time, and user satisfaction.
Example of Performance Metrics:
Diagram of Implementation and Testing Workflow:
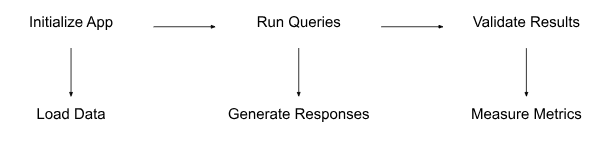
Raga AI’s testing platform is a comprehensive tool that automates the process to ensure you don’t have any data, model, or operational issues. Check out how it is the future of AI testing.
By systematically setting up, implementing, and validating your RAG application, you ensure it performs effectively and meets user needs. Next, we will explore optimizing and evaluating the RAG implementation to enhance performance.
Optimizing and Evaluating the RAG Implementation
Once your RAG application is up and running, the next step is to optimize its performance and evaluate its effectiveness. This ensures that the application not only works but works well. Using a RAG LLM example, optimization and evaluation involve tweaking configurations, conducting thorough assessments, and refining the system based on feedback. Let's explore these processes in detail.
Exploring Different Configurations
Experimenting with different configurations can significantly impact the performance of your RAG application. Adjusting parameters like chunk size, embedding model, and query retrieval settings can enhance efficiency and accuracy.
Example:
# Adjust configurations
CHUNK_SIZE = 256 # Smaller chunks for more precise retrieval
NUM_NEIGHBORS = 3 # Fewer neighbors for faster response
# Function to adjust configurations
def configure_rag(chunk_size, num_neighbors):
# Update chunking and retrieval settings
def chunk_text(text, chunk_size=chunk_size):
tokens = tokenizer.tokenize(text)
chunks = [tokens[i:i + chunk_size] for i in range(0, len(tokens), chunk_size)]
return [' '.join(chunk) for chunk in chunks]
def query_rag(query):
query_embedding = embed_text(query)
distances, indices = index.kneighbors(query_embedding, n_neighbors=num_neighbors)
context = []
for idx in indices[0]:
with open(os.path.join(data_dir, f'doc_{idx}.txt'), 'r') as f:
context.append(f.read())
context = ' '.join(context)
response = generator(f"Context: {context} Query: {query}", max_length=150)
return response[0]['generated_text']
return query_rag
# Apply new configurations
query_rag = configure_rag(CHUNK_SIZE, NUM_NEIGHBORS)
Conducting Evaluations
Systematic evaluations are crucial for understanding how well your RAG application performs. Evaluations can be conducted for both individual components and the entire system.
Example:
# List of sample queries for evaluation
queries = [
"What is the capital of New Jersey?",
"Who wrote 'To Kill a Mockingbird'?",
"Explain the theory of relativity."
]
# Evaluate responses
responses = [query_rag(query) for query in queries]
for query, response in zip(queries, responses):
print(f"Query: {query}\nResponse: {response}\n")
Methods to Quantitatively Assess Generative Tasks
To ensure your RAG application is functioning optimally, assess its performance using quantitative metrics. Common metrics include precision, recall, F1 score, and response time.
Example of Performance Metrics:

Diagram of Optimization and Evaluation Workflow:
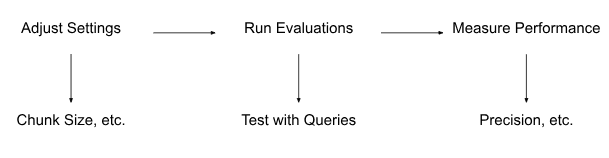
By methodically optimizing configurations and conducting detailed evaluations, you can significantly enhance the performance of your RAG application. Using a RAG LLM example, this approach ensures that it meets the required standards for efficiency and accuracy. Next, we will explore strategies for addressing the cold start problem, which is crucial for maintaining high performance from the start.
Addressing the Cold Start Problem
The cold start problem can be a significant hurdle when implementing a RAG application. It refers to the challenge of getting your model to perform well with little or no initial data. This issue can affect both the quality of responses and the overall user experience. Using a RAG LLM example, here’s how you can address this problem effectively.
Generating Synthetic Q&A Pairs
One of the most effective ways to combat the cold start problem is by generating synthetic Q&A pairs. This involves creating artificial data that can help train your model and improve its initial performance.
Example:
# Function to generate synthetic Q&A pairs
def generate_synthetic_pairs(num_pairs):
synthetic_pairs = []
for _ in range(num_pairs):
question = f"Sample question {_}?"
answer = f"Sample answer for question {_}."
synthetic_pairs.append((question, answer))
return synthetic_pairs
# Generate synthetic pairs
synthetic_data = generate_synthetic_pairs(100)
print(synthetic_data[:5])
Using Pre-trained Models
Leveraging pre-trained models can also help mitigate the cold start problem. These models have already been trained on large datasets and can provide a strong foundation for your application.
Example:
from transformers import AutoModelForQuestionAnswering, AutoTokenizer
# Load a pre-trained model and tokenizer
tokenizer = AutoTokenizer.from_pretrained('bert-large-uncased-whole-word-masking-finetuned-squad')
model = AutoModelForQuestionAnswering.from_pretrained('bert-large-uncased-whole-word-masking-finetuned-squad')
# Function to use pre-trained model for answering questions
def answer_question(question, context):
inputs = tokenizer.encode_plus(question, context, add_special_tokens=True, return_tensors='pt')
answer_start_scores, answer_end_scores = model(**inputs)
answer_start = torch.argmax(answer_start_scores)
answer_end = torch.argmax(answer_end_scores) + 1
answer = tokenizer.convert_tokens_to_string(tokenizer.convert_ids_to_tokens(inputs['input_ids'][0][answer_start:answer_end]))
return answer
# Example usage
context = "New Jersey's capital is Trenton."
question = "What is the capital of New Jersey?"
answer = answer_question(question, context)
print(answer)
Pre-training with Domain-Specific Data
Another effective strategy is to pre-train your model with domain-specific data. This approach ensures that the model is familiar with the specific type of queries it will encounter, thereby improving its performance from the start.
Example:
from transformers import TextDataset, DataCollatorForLanguageModeling, Trainer, TrainingArguments
# Function to load domain-specific data for pre-training
def load_dataset(file_path):
return TextDataset(tokenizer=tokenizer, file_path=file_path, block_size=128)
# Load the dataset
dataset = load_dataset('domain_specific_data.txt')
# Data collator
data_collator = DataCollatorForLanguageModeling(tokenizer=tokenizer, mlm=True)
# Training arguments
training_args = TrainingArguments(
output_dir='./results',
overwrite_output_dir=True,
num_train_epochs=3,
per_device_train_batch_size=8,
save_steps=10_000,
save_total_limit=2,
)
# Trainer
trainer = Trainer(
model=model,
args=training_args,
data_collator=data_collator,
train_dataset=dataset,
)
# Train the model
trainer.train()
Diagram of Cold Start Mitigation Strategies:
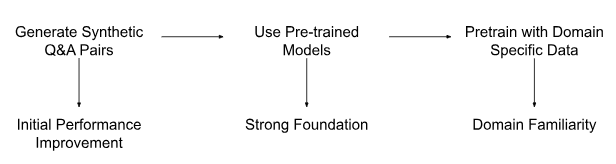
Employing these strategies can effectively address the cold start problem and ensure your RAG application performs well right from the beginning.
Read this case study on enhancing reliability with guardrails.
Next, we will explore how to experiment with different configurations and fine-tune the system for optimal performance.
Experimentation and Improvement
After addressing the cold start problem, the next step is to refine and enhance your RAG application. Using a RAG LLM example, experimentation and continuous improvement are vital to optimizing performance and ensuring the application meets your needs. This involves testing various configurations, models, and techniques to find the best setup. Let's dive into these processes.
Testing Different Chunk Sizes
The size of the text chunks used in your RAG application can significantly impact performance. Experimenting with different chunk sizes helps find the optimal balance between retrieval accuracy and processing efficiency.
Example:
# Function to experiment with different chunk sizes
def test_chunk_sizes(text, sizes=[128, 256, 512]):
results = {}
for size in sizes:
chunks = chunk_text(text, chunk_size=size)
embeddings = [embed_text(chunk) for chunk in chunks]
results[size] = embeddings
return results
# Sample text for chunking
text = "New Jersey's capital is Trenton. Python is a versatile programming language."
# Test different chunk sizes
chunk_results = test_chunk_sizes(text)
for size, embeddings in chunk_results.items():
print(f"Chunk Size: {size}, Number of Chunks: {len(embeddings)}")
Experimenting with Embedding Models
Different embedding models can produce varying results in terms of accuracy and performance. Testing multiple models helps identify which one works best for your specific use case.
Example:
from transformers import AutoModel
# Function to test different embedding models
def test_embedding_models(text, models=['bert-base-uncased', 'roberta-base']):
results = {}
for model_name in models:
tokenizer = AutoTokenizer.from_pretrained(model_name)
model = AutoModel.from_pretrained(model_name)
inputs = tokenizer(text, return_tensors='pt', truncation=True, padding=True)
outputs = model(**inputs)
embeddings = outputs.last_hidden_state.mean(dim=1).detach().numpy()
results[model_name] = embeddings
return results
# Test text for embeddings
test_text = "Machine learning is a branch of artificial intelligence."
# Test different embedding models
embedding_results = test_embedding_models(test_text)
for model_name, embedding in embedding_results.items():
print(f"Model: {model_name}, Embedding Shape: {embedding.shape}")
Fine-Tuning for Better Context Representation
Fine-tuning your models with domain-specific data can significantly improve their ability to represent context accurately. This process involves training the model on data that is similar to what it will encounter in actual use.
Example:
from transformers import Trainer, TrainingArguments
# Function to fine-tune a model
def fine_tune_model(model_name, dataset_path):
tokenizer = AutoTokenizer.from_pretrained(model_name)
model = AutoModel.from_pretrained(model_name)
# Load dataset
dataset = load_dataset(dataset_path)
# Data collator
data_collator = DataCollatorForLanguageModeling(tokenizer=tokenizer, mlm=True)
# Training arguments
training_args = TrainingArguments(
output_dir='./results',
num_train_epochs=3,
per_device_train_batch_size=8,
save_steps=10_000,
save_total_limit=2,
)
# Trainer
trainer = Trainer(
model=model,
args=training_args,
data_collator=data_collator,
train_dataset=dataset,
)
# Train the model
trainer.train()
return model
# Fine-tune the model with domain-specific data
fine_tuned_model = fine_tune_model('bert-base-uncased', 'domain_specific_data.txt')
Diagram of Experimentation and Improvement Workflow:
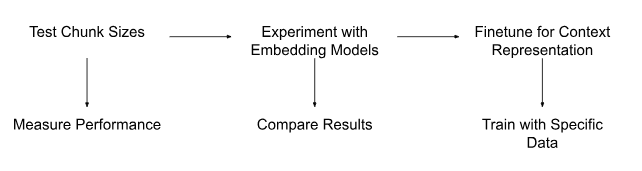
By experimenting with different configurations and models and fine-tuning your system, you can continuously improve the performance of your RAG application. This iterative process ensures that the application remains effective and efficient. Next, we will discuss examples of RAG LLM techniques for serving and scaling your RAG application to handle real-world demands.
Application Serving, Scaling, and Continuous Improvement
Building a robust RAG application is only the beginning. To make it truly useful, you must serve it efficiently, scale it to meet demand, and continuously improve it based on user feedback and performance metrics. Let’s delve into these aspects.
Serving the RAG Application
Serving your RAG application means making it accessible for users to query. This typically involves setting up an API endpoint that handles incoming requests and returns generated responses.
Example:
from flask import Flask, request, jsonify
app = Flask(__name__)
# Load the model and other necessary components
query_rag = setup_rag_application(data_dir, tokenizer, model, generator, index)
@app.route('/query', methods=['POST'])
def query():
data = request.get_json()
query_text = data['query']
response = query_rag(query_text)
return jsonify({'response': response})
if __name__ == '__main__':
app.run(debug=True)
Scaling the RAG Application
To handle multiple queries simultaneously and ensure fast response times, you need to scale your application. Using a RAG LLM example, this involves deploying your application on a robust infrastructure that can manage high traffic and distribute load effectively.
Example:
Horizontal Scaling: Deploy multiple instances of your application and use a load balancer to distribute traffic.
Vertical Scaling: Increase the computational resources (CPU, RAM) of your existing server to handle more requests.
Techniques for Scaling:
Containerization: Use Docker to containerize your application, making it easy to deploy and scale across different environments.
Example:
# Dockerfile for RAG application
FROM python:3.8-slim
WORKDIR /app
COPY requirements.txt requirements.txt
RUN pip install -r requirements.txt
COPY . .
CMD ["python", "app.py"]
Orchestration: Use Kubernetes to manage and scale your containerized application.
Example:
apiVersion: apps/v1
kind: Deployment
metadata:
name: rag-deployment
spec:
replicas: 3
selector:
matchLabels:
app: rag-app
template:
metadata:
labels:
app: rag-app
spec:
containers:
- name: rag-container
image: rag-image
ports:
- containerPort: 5000
Continuous Improvement
To ensure your application remains effective, continuous improvement is crucial. This involves regularly updating your models, refining data, and incorporating user feedback.
Strategies for Continuous Improvement:
Monitor Performance: Regularly track key metrics such as response time, accuracy, and user satisfaction.
Example of Monitoring Metrics:

Feedback Loop: Implement a feedback mechanism where users can rate responses and provide comments. Use this feedback to improve your models and data.
Regular Updates: Schedule regular updates for your models and data to incorporate the latest information and improvements.
Diagram of Application Serving and Scaling Workflow:
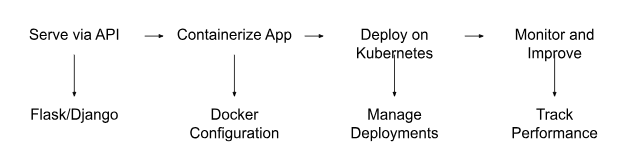
By efficiently serving, scaling, and continuously improving your RAG application, you ensure it remains reliable, responsive, and effective. Next, we will summarize the entire process and discuss the future prospects of integrating RAG into LLM applications.
Conclusion
Building a Retrieval Augmented Generation (RAG) application is a comprehensive process that involves several crucial steps. Using a RAG LLM example, you'll start by understanding the basics of RAG, preparing your database, processing data, building, implementing, and continuously improving your application. Each step plays a vital role in ensuring the success of your project. By following the detailed instructions provided, you can create a robust RAG application that delivers accurate and contextually relevant responses, enhancing the overall user experience.
Raga AI offers a state-of-the-art platform designed to simplify the development and deployment of AI applications. With features such as automated issue detection, diagnosis, and resolution, Raga AI ensures your applications are reliable and high-performing. If you're looking to enhance your AI projects with the latest in RAG technology, consider exploring Raga AI's comprehensive solutions. Start your journey with Raga AI today and take your AI applications to the next level.
If you've been exploring the world of large language models (LLMs), you've likely encountered their impressive capabilities and notable limitations. One of the significant challenges with LLMs is their tendency to produce hallucinations or inaccurate information, especially when generating responses without sufficient contextual grounding.
This is where Retrieval Augmented Generation (RAG) comes in. For a great RAG LLM example, RAG enhances LLMs by integrating them with external databases, allowing the model to retrieve relevant information and use it to generate more accurate and contextually appropriate responses. This technique not only improves the quality of the generated content but also significantly reduces the risk of hallucinations, making LLM applications more reliable and effective.
In this guide, you'll learn how to build your own RAG-based LLM application from scratch. We'll start with a clear definition of what RAG is and why it's essential for addressing some of the common issues associated with LLMs. For a practical Rag LLM example, we'll walk you through preparing your database, processing the necessary data, and implementing the RAG application. By the end of this guide, you'll have a solid understanding of integrating RAG into your LLM applications, enhancing their performance and reliability. Now, let's dive into understanding RAG and how it tackles the hallucination problem in LLMs.
Understanding RAG
Retrieval Augmented Generation (RAG) is a powerful technique designed to address some limitations of large language models (LLMs). By integrating external knowledge sources, RAG enhances the accuracy and contextual relevance of the generated responses. Let's explain how RAG works and why it's a valuable addition to LLM applications.
How RAG Tackles the Hallucination Problem
One of the biggest issues with LLMs is their tendency to produce hallucinations or responses that seem plausible but are factually incorrect. RAG addresses this by pulling in relevant information from external databases before generating a response. This means the model can access accurate and up-to-date information, significantly reducing the likelihood of hallucinations.
Learn more about LLM hallucinations in this article.
Example:
Consider a query about the capital of New Jersey. Based on its training data, an LLM might generate a wrong answer. However, with RAG, the model retrieves information from a reliable source, ensuring the response is accurate.
from transformers import pipeline, RagTokenizer, RagRetriever, RagTokenForGeneration
# Initialize tokenizer and retriever
tokenizer = RagTokenizer.from_pretrained("facebook/rag-token-base")
retriever = RagRetriever.from_pretrained("facebook/rag-token-base")
# Initialize model
model = RagTokenForGeneration.from_pretrained("facebook/rag-token-base", retriever=retriever)
# Input query
query = "What is the capital of New Jersey?"
# Generate response
input_ids = tokenizer(query, return_tensors="pt").input_ids
outputs = model.generate(input_ids=input_ids)
answer = tokenizer.decode(outputs[0], skip_special_tokens=True)
print(answer)
The Architectural Overview of RAG
RAG combines the strengths of generative models with the precision of retrieval systems. Here's a high-level overview of its architecture:
Retrieval Component: This part of the system searches external databases for relevant information based on the input query. The retrieved documents or data chunks are then fed into the generative model.
Generative Component: Using the retrieved information, the generative model creates a response that is both accurate and contextually appropriate.
Diagram of RAG Architecture:
Practical Benefits of RAG
Improved Accuracy: By integrating external data sources, RAG ensures responses are grounded in factual information.
Enhanced Context: RAG provides additional context to LLMs, making responses more relevant and informative.
Versatility: RAG can be applied to various applications, from customer support chatbots to content generation.
Key Components of RAG
Database Preparation: Ensuring that the external knowledge source is comprehensive and regularly updated.
Data Processing: Efficiently extracting, chunking, and embedding data for quick retrieval.
Integration: Seamlessly combining the retrieval and generative components to work in harmony.
By understanding the foundational principles of RAG, you're now equipped to explore its implementation in your LLM applications. To give you a clear RAG LLM example, we'll next dive into the specifics of preparing your database for RAG.
Preparing the Database for RAG
Setting up a robust database is crucial for the success of your Retrieval Augmented Generation (RAG) application. The database is the backbone, providing the external knowledge needed to generate accurate and contextually relevant responses. Let's break down the steps involved in preparing your database for RAG.
Loading Data into a Local Directory
The first step in preparing your database is to load your data into a local directory. This data can come from various sources such as text files, PDFs, or structured data files like CSVs. Here’s a simple example of how to load text data into a directory.
Example:
import os
# Define the directory path
data_dir = 'rag_data'
# Create the directory if it doesn't exist
if not os.path.exists(data_dir):
os.makedirs(data_dir)
# Sample data
documents = [
"New Jersey's capital is Trenton.",
"Python is a versatile programming language."
]
# Save each document as a separate text file
for i, doc in enumerate(documents):
with open(os.path.join(data_dir, f'doc_{i}.txt'), 'w') as f:
f.write(doc)
Creating Scalable Datasets
Once your data is loaded, the next step is to create scalable datasets that can be efficiently processed. This involves structuring your data in a way that allows for quick retrieval and minimal processing time.
Steps:
Chunking Data: Break down large documents into smaller, manageable chunks.
Embedding Data: Convert text chunks into numerical vectors using pre-trained models.
Example:
from transformers import AutoTokenizer, AutoModel
# Load a pre-trained tokenizer and model
tokenizer = AutoTokenizer.from_pretrained('bert-base-uncased')
model = AutoModel.from_pretrained('bert-base-uncased')
# Function to chunk text
def chunk_text(text, chunk_size=512):
tokens = tokenizer.tokenize(text)
chunks = [tokens[i:i + chunk_size] for i in range(0, len(tokens), chunk_size)]
return [' '.join(chunk) for chunk in chunks]
# Function to embed text
def embed_text(text):
inputs = tokenizer(text, return_tensors='pt')
outputs = model(**inputs)
return outputs.last_hidden_state.mean(dim=1).detach().numpy()
# Example usage
chunks = chunk_text(documents[0])
embeddings = [embed_text(chunk) for chunk in chunks]
Indexing Chunks for Rapid Retrieval
Indexing your data chunks is vital for quick retrieval during query processing. You can use various indexing techniques, such as inverted indices or vector databases, to achieve this.
Example:
from sklearn.neighbors import NearestNeighbors
import numpy as np
# Create an index of embeddings
index = NearestNeighbors(n_neighbors=1, algorithm='ball_tree')
index.fit(np.vstack(embeddings))
# Save the index
import joblib
joblib.dump(index, 'rag_index.pkl')
Diagram of the Data Preparation Process
By ensuring your database is well-prepared and efficiently indexed, you set a solid foundation for your RAG application.
Next, we’ll examine the data processing for RAG, which includes extracting, chunking, and embedding data sections.
Processing Data for RAG
Once you have your data loaded and organized, the next step is to process it for use in your Retrieval Augmented Generation (RAG) application. This involves extracting relevant information, chunking it into manageable pieces, and embedding these chunks for efficient retrieval. Let's dive into these processes in detail to illustrate with a RAG LLM example.
Extracting Data
Extracting data means pulling relevant information from your sources. Depending on your data format, this might involve parsing text files, scraping web content, or querying databases.
Example:
import os
# Directory containing the data
data_dir = 'rag_data'
# Function to read text files from the directory
def read_files(directory):
documents = []
for filename in os.listdir(directory):
if filename.endswith('.txt'):
with open(os.path.join(directory, filename), 'r') as f:
documents.append(f.read())
return documents
# Read data
documents = read_files(data_dir)
print(documents)
Chunking Data
Chunking involves breaking down large documents into smaller, more manageable pieces. This is important for both efficiency and accuracy, as smaller chunks are easier to process and retrieve.
Example:
def chunk_text(text, chunk_size=512):
tokens = tokenizer.tokenize(text)
chunks = [tokens[i:i + chunk_size] for i in range(0, len(tokens), chunk_size)]
return [' '.join(chunk) for chunk in chunks]
# Chunk all documents
chunked_documents = [chunk_text(doc) for doc in documents]
print(chunked_documents)
Embedding Data
Embedding converts text chunks into numerical vectors that can be used for efficient retrieval. Using a pre-trained model, you can transform each chunk into a vector representation.
Example:
from transformers import AutoTokenizer, AutoModel
import torch
# Load a pre-trained tokenizer and model
tokenizer = AutoTokenizer.from_pretrained('bert-base-uncased')
model = AutoModel.from_pretrained('bert-base-uncased')
# Function to embed text
def embed_text(text):
inputs = tokenizer(text, return_tensors='pt', truncation=True, padding=True)
outputs = model(**inputs)
return outputs.last_hidden_state.mean(dim=1).detach().numpy()
# Embed all chunks
embeddings = [embed_text(chunk) for doc in chunked_documents for chunk in doc]
print(embeddings)
Indexing Chunks
Indexing the embedded chunks allows for quick retrieval during the query process. Various indexing techniques can be used, such as using k-nearest neighbors for efficient lookups.
Example:
from sklearn.neighbors import NearestNeighbors
import numpy as np
# Flatten the list of embeddings for indexing
flat_embeddings = np.vstack(embeddings)
# Create an index
index = NearestNeighbors(n_neighbors=5, algorithm='ball_tree')
index.fit(flat_embeddings)
# Save the index for later use
import joblib
joblib.dump(index, 'rag_index.pkl')
Diagram of Data Processing Workflow:
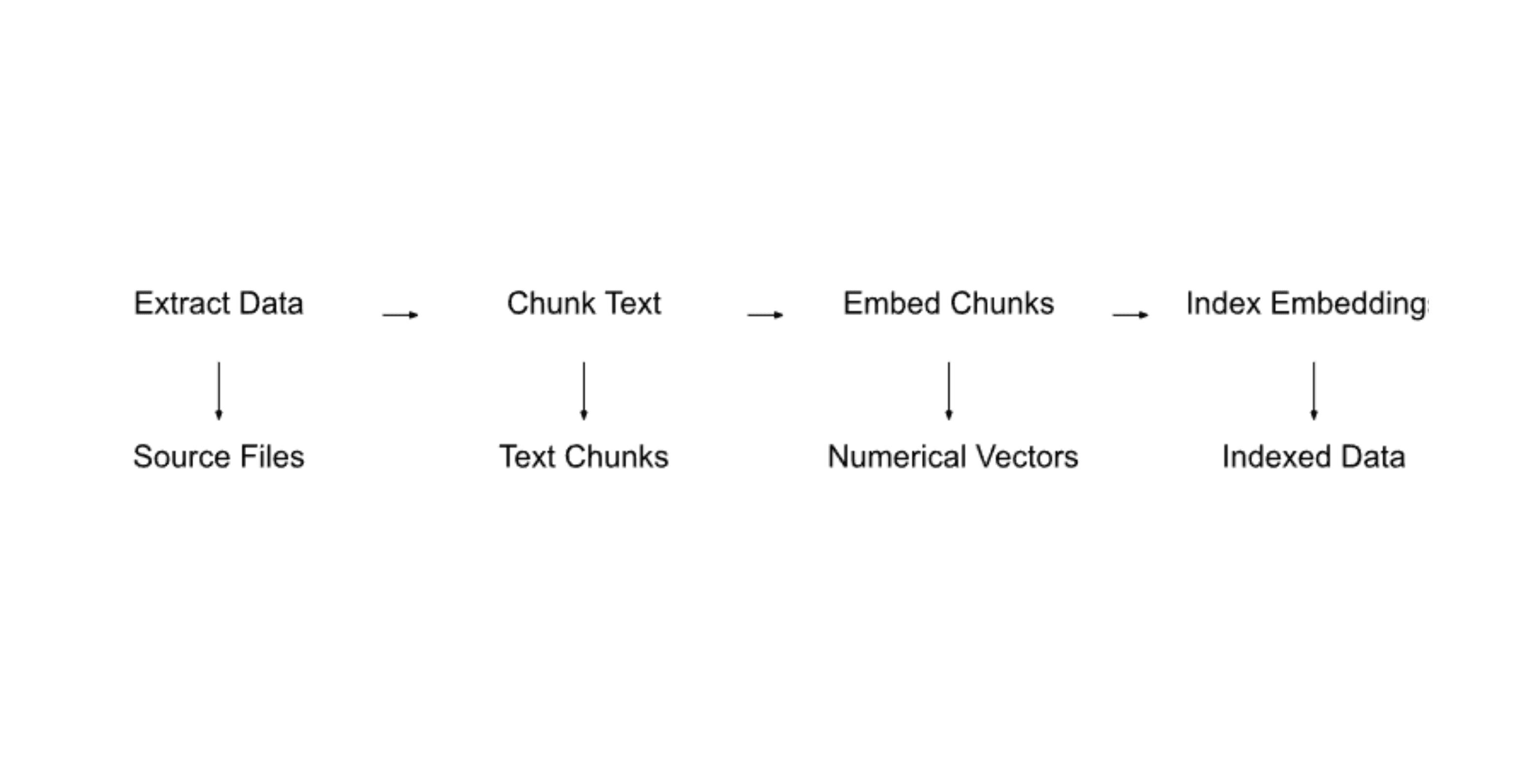
By efficiently processing your data through extraction, chunking, embedding, and indexing, you set the stage for building a powerful RAG application. Next, we will focus on implementing the RAG application, including query retrieval and response generation.
Learn more about the LLM parameters.
Building the RAG Application
Now that your data is processed and ready, it's time to build the Retrieval Augmented Generation (RAG) application. This involves setting up the system for query retrieval, generating responses using the embedded data, and optimizing the application for performance. Let's break down the steps to build your RAG application.
Implementing Query Retrieval
The first step in building your RAG application is implementing the query retrieval system. This system will handle user queries and retrieve relevant data chunks from the indexed database.
Example:
# Function to retrieve relevant data chunks for a query
def retrieve_chunks(query, index, tokenizer, model):
query_embedding = embed_text(query)
distances, indices = index.kneighbors(query_embedding)
return indices[0]
# Example query
query = "What is the capital of New Jersey?"
retrieved_indices = retrieve_chunks(query, index, tokenizer, model)
print(retrieved_indices)
Generating Responses
Once the relevant data chunks are retrieved, the next step is to generate a response using the LLM. The retrieved data provides context, helping the model to produce accurate and relevant answers.
Example:
from transformers import pipeline
# Load a pre-trained generative model
generator = pipeline('text-generation', model='gpt-3.5-turbo')
# Function to generate a response using the retrieved chunks
def generate_response(query, retrieved_indices, data_dir):
context = []
for idx in retrieved_indices:
with open(os.path.join(data_dir, f'doc_{idx}.txt'), 'r') as f:
context.append(f.read())
context = ' '.join(context)
response = generator(f"Context: {context} Query: {query}", max_length=100)
return response[0]['generated_text']
# Generate a response
response = generate_response(query, retrieved_indices, data_dir)
print(response)
Configurations and Optimizations
To ensure your RAG application runs efficiently, you need to configure and optimize various system aspects. This includes tuning the retrieval process and the response generation model.
Example:
# Configurations for query retrieval
NUM_NEIGHBORS = 5 # Number of nearest neighbors to retrieve
# Optimizations for response generation
GENERATION_MAX_LENGTH = 150 # Maximum length of generated responses
# Function to optimize retrieval and generation
def optimized_generate_response(query, index, tokenizer, model, generator, data_dir):
query_embedding = embed_text(query)
distances, indices = index.kneighbors(query_embedding, n_neighbors=NUM_NEIGHBORS)
context = []
for idx in indices[0]:
with open(os.path.join(data_dir, f'doc_{idx}.txt'), 'r') as f:
context.append(f.read())
context = ' '.join(context)
response = generator(f"Context: {context} Query: {query}", max_length=GENERATION_MAX_LENGTH)
return response[0]['generated_text']
# Generate an optimized response
optimized_response = optimized_generate_response(query, index, tokenizer, model, generator, data_dir)
print(optimized_response)
Diagram of the RAG Application Workflow:
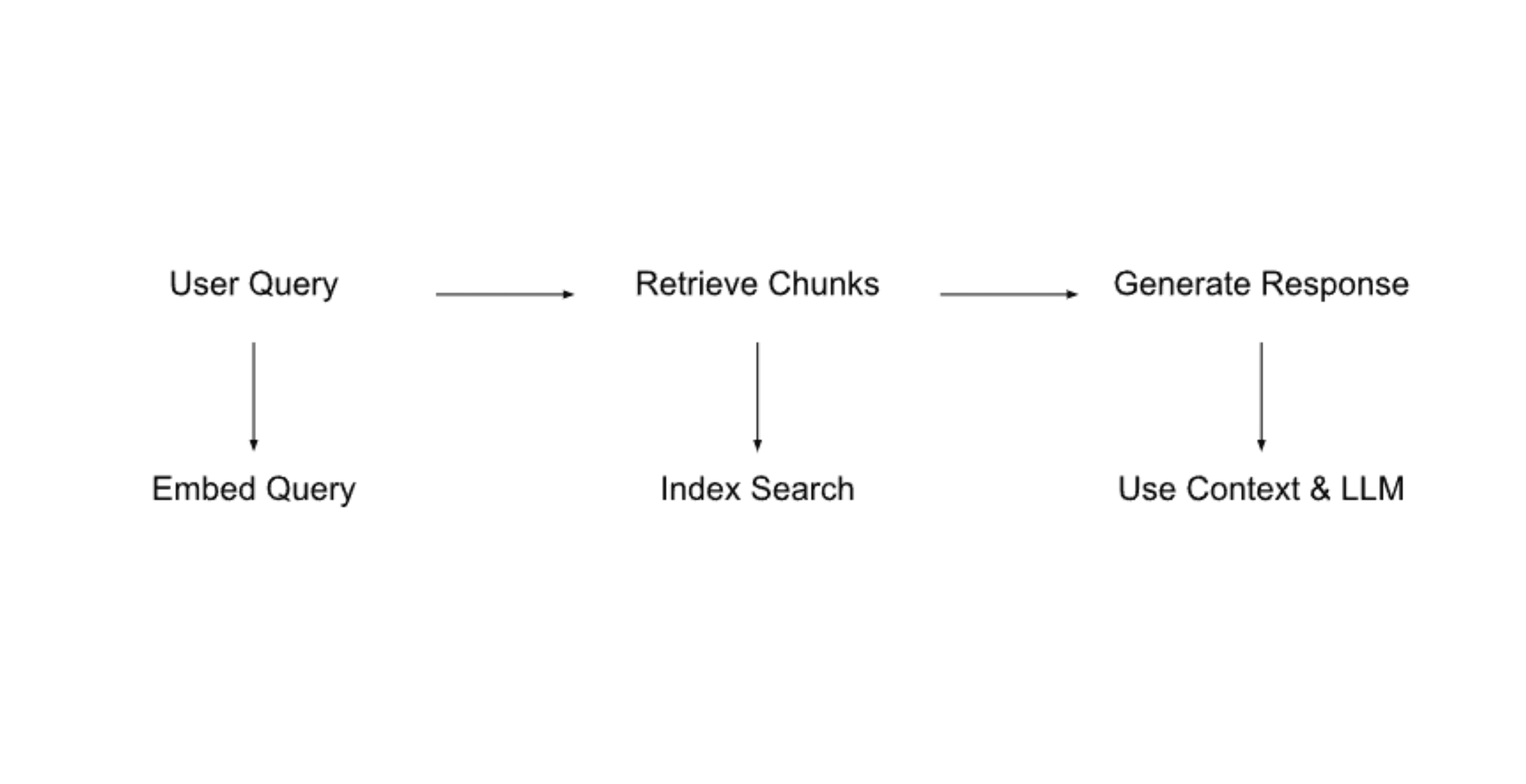
By carefully implementing query retrieval, generating responses with context, and optimizing your configurations, you create a robust RAG application.
To learn more, visit this webinar on building RAG applications and ensuring safe and reliable GenAI.
Next, we will look at how to implement and test the RAG application to ensure it functions correctly.
Implementing and Testing the RAG Application
With your RAG application built, the next crucial step is to implement it and ensure it works correctly. This involves setting up the application for querying, running tests to validate its performance, and fine-tuning as necessary. To illustrate with a RAG LLM example, let's go through these steps in detail.
Setting Up the RAG Application for Querying
The initial implementation step is to set up the RAG application so it can handle queries effectively. This involves integrating all components and ensuring they work together seamlessly.
Example:
# Function to set up the RAG application
def setup_rag_application(data_dir, tokenizer, model, generator, index):
def query_rag(query):
query_embedding = embed_text(query)
distances, indices = index.kneighbors(query_embedding, n_neighbors=5)
context = []
for idx in indices[0]:
with open(os.path.join(data_dir, f'doc_{idx}.txt'), 'r') as f:
context.append(f.read())
context = ' '.join(context)
response = generator(f"Context: {context} Query: {query}", max_length=150)
return response[0]['generated_text']
return query_rag
# Initialize the RAG application
query_rag = setup_rag_application(data_dir, tokenizer, model, generator, index)
Examples and Validation
Testing your RAG application with different queries is essential to validate its performance. By running several tests, you can ensure the system retrieves relevant information and generates accurate responses.
Example Query Test:
# Test the RAG application with a sample query
sample_query = "What is the capital of New Jersey?"
response = query_rag(sample_query)
print(f"Query: {sample_query}\nResponse: {response}")
Validation with Multiple Queries
To thoroughly test the application, use a set of diverse queries and evaluate the responses.
Example:
# List of sample queries
queries = [
"Who wrote 'Pride and Prejudice'?",
"What is the boiling point of water?",
"Define machine learning."
]
# Validate responses
for query in queries:
response = query_rag(query)
print(f"Query: {query}\nResponse: {response}\n")
Performance Metrics:

To quantify the effectiveness of your RAG application, consider using performance metrics such as accuracy, response time, and user satisfaction.
Example of Performance Metrics:
Diagram of Implementation and Testing Workflow:
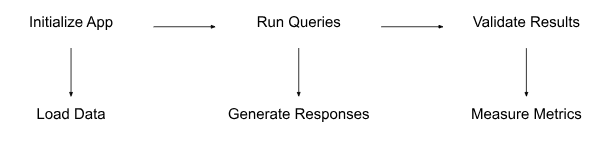
Raga AI’s testing platform is a comprehensive tool that automates the process to ensure you don’t have any data, model, or operational issues. Check out how it is the future of AI testing.
By systematically setting up, implementing, and validating your RAG application, you ensure it performs effectively and meets user needs. Next, we will explore optimizing and evaluating the RAG implementation to enhance performance.
Optimizing and Evaluating the RAG Implementation
Once your RAG application is up and running, the next step is to optimize its performance and evaluate its effectiveness. This ensures that the application not only works but works well. Using a RAG LLM example, optimization and evaluation involve tweaking configurations, conducting thorough assessments, and refining the system based on feedback. Let's explore these processes in detail.
Exploring Different Configurations
Experimenting with different configurations can significantly impact the performance of your RAG application. Adjusting parameters like chunk size, embedding model, and query retrieval settings can enhance efficiency and accuracy.
Example:
# Adjust configurations
CHUNK_SIZE = 256 # Smaller chunks for more precise retrieval
NUM_NEIGHBORS = 3 # Fewer neighbors for faster response
# Function to adjust configurations
def configure_rag(chunk_size, num_neighbors):
# Update chunking and retrieval settings
def chunk_text(text, chunk_size=chunk_size):
tokens = tokenizer.tokenize(text)
chunks = [tokens[i:i + chunk_size] for i in range(0, len(tokens), chunk_size)]
return [' '.join(chunk) for chunk in chunks]
def query_rag(query):
query_embedding = embed_text(query)
distances, indices = index.kneighbors(query_embedding, n_neighbors=num_neighbors)
context = []
for idx in indices[0]:
with open(os.path.join(data_dir, f'doc_{idx}.txt'), 'r') as f:
context.append(f.read())
context = ' '.join(context)
response = generator(f"Context: {context} Query: {query}", max_length=150)
return response[0]['generated_text']
return query_rag
# Apply new configurations
query_rag = configure_rag(CHUNK_SIZE, NUM_NEIGHBORS)
Conducting Evaluations
Systematic evaluations are crucial for understanding how well your RAG application performs. Evaluations can be conducted for both individual components and the entire system.
Example:
# List of sample queries for evaluation
queries = [
"What is the capital of New Jersey?",
"Who wrote 'To Kill a Mockingbird'?",
"Explain the theory of relativity."
]
# Evaluate responses
responses = [query_rag(query) for query in queries]
for query, response in zip(queries, responses):
print(f"Query: {query}\nResponse: {response}\n")
Methods to Quantitatively Assess Generative Tasks
To ensure your RAG application is functioning optimally, assess its performance using quantitative metrics. Common metrics include precision, recall, F1 score, and response time.
Example of Performance Metrics:

Diagram of Optimization and Evaluation Workflow:
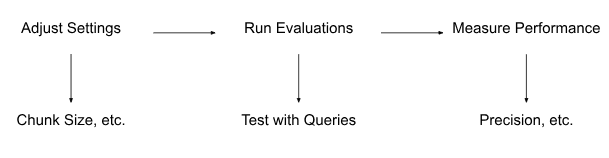
By methodically optimizing configurations and conducting detailed evaluations, you can significantly enhance the performance of your RAG application. Using a RAG LLM example, this approach ensures that it meets the required standards for efficiency and accuracy. Next, we will explore strategies for addressing the cold start problem, which is crucial for maintaining high performance from the start.
Addressing the Cold Start Problem
The cold start problem can be a significant hurdle when implementing a RAG application. It refers to the challenge of getting your model to perform well with little or no initial data. This issue can affect both the quality of responses and the overall user experience. Using a RAG LLM example, here’s how you can address this problem effectively.
Generating Synthetic Q&A Pairs
One of the most effective ways to combat the cold start problem is by generating synthetic Q&A pairs. This involves creating artificial data that can help train your model and improve its initial performance.
Example:
# Function to generate synthetic Q&A pairs
def generate_synthetic_pairs(num_pairs):
synthetic_pairs = []
for _ in range(num_pairs):
question = f"Sample question {_}?"
answer = f"Sample answer for question {_}."
synthetic_pairs.append((question, answer))
return synthetic_pairs
# Generate synthetic pairs
synthetic_data = generate_synthetic_pairs(100)
print(synthetic_data[:5])
Using Pre-trained Models
Leveraging pre-trained models can also help mitigate the cold start problem. These models have already been trained on large datasets and can provide a strong foundation for your application.
Example:
from transformers import AutoModelForQuestionAnswering, AutoTokenizer
# Load a pre-trained model and tokenizer
tokenizer = AutoTokenizer.from_pretrained('bert-large-uncased-whole-word-masking-finetuned-squad')
model = AutoModelForQuestionAnswering.from_pretrained('bert-large-uncased-whole-word-masking-finetuned-squad')
# Function to use pre-trained model for answering questions
def answer_question(question, context):
inputs = tokenizer.encode_plus(question, context, add_special_tokens=True, return_tensors='pt')
answer_start_scores, answer_end_scores = model(**inputs)
answer_start = torch.argmax(answer_start_scores)
answer_end = torch.argmax(answer_end_scores) + 1
answer = tokenizer.convert_tokens_to_string(tokenizer.convert_ids_to_tokens(inputs['input_ids'][0][answer_start:answer_end]))
return answer
# Example usage
context = "New Jersey's capital is Trenton."
question = "What is the capital of New Jersey?"
answer = answer_question(question, context)
print(answer)
Pre-training with Domain-Specific Data
Another effective strategy is to pre-train your model with domain-specific data. This approach ensures that the model is familiar with the specific type of queries it will encounter, thereby improving its performance from the start.
Example:
from transformers import TextDataset, DataCollatorForLanguageModeling, Trainer, TrainingArguments
# Function to load domain-specific data for pre-training
def load_dataset(file_path):
return TextDataset(tokenizer=tokenizer, file_path=file_path, block_size=128)
# Load the dataset
dataset = load_dataset('domain_specific_data.txt')
# Data collator
data_collator = DataCollatorForLanguageModeling(tokenizer=tokenizer, mlm=True)
# Training arguments
training_args = TrainingArguments(
output_dir='./results',
overwrite_output_dir=True,
num_train_epochs=3,
per_device_train_batch_size=8,
save_steps=10_000,
save_total_limit=2,
)
# Trainer
trainer = Trainer(
model=model,
args=training_args,
data_collator=data_collator,
train_dataset=dataset,
)
# Train the model
trainer.train()
Diagram of Cold Start Mitigation Strategies:
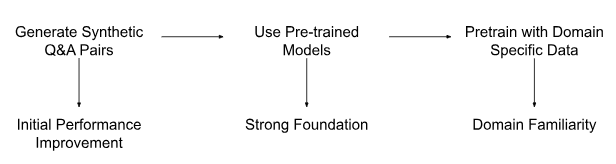
Employing these strategies can effectively address the cold start problem and ensure your RAG application performs well right from the beginning.
Read this case study on enhancing reliability with guardrails.
Next, we will explore how to experiment with different configurations and fine-tune the system for optimal performance.
Experimentation and Improvement
After addressing the cold start problem, the next step is to refine and enhance your RAG application. Using a RAG LLM example, experimentation and continuous improvement are vital to optimizing performance and ensuring the application meets your needs. This involves testing various configurations, models, and techniques to find the best setup. Let's dive into these processes.
Testing Different Chunk Sizes
The size of the text chunks used in your RAG application can significantly impact performance. Experimenting with different chunk sizes helps find the optimal balance between retrieval accuracy and processing efficiency.
Example:
# Function to experiment with different chunk sizes
def test_chunk_sizes(text, sizes=[128, 256, 512]):
results = {}
for size in sizes:
chunks = chunk_text(text, chunk_size=size)
embeddings = [embed_text(chunk) for chunk in chunks]
results[size] = embeddings
return results
# Sample text for chunking
text = "New Jersey's capital is Trenton. Python is a versatile programming language."
# Test different chunk sizes
chunk_results = test_chunk_sizes(text)
for size, embeddings in chunk_results.items():
print(f"Chunk Size: {size}, Number of Chunks: {len(embeddings)}")
Experimenting with Embedding Models
Different embedding models can produce varying results in terms of accuracy and performance. Testing multiple models helps identify which one works best for your specific use case.
Example:
from transformers import AutoModel
# Function to test different embedding models
def test_embedding_models(text, models=['bert-base-uncased', 'roberta-base']):
results = {}
for model_name in models:
tokenizer = AutoTokenizer.from_pretrained(model_name)
model = AutoModel.from_pretrained(model_name)
inputs = tokenizer(text, return_tensors='pt', truncation=True, padding=True)
outputs = model(**inputs)
embeddings = outputs.last_hidden_state.mean(dim=1).detach().numpy()
results[model_name] = embeddings
return results
# Test text for embeddings
test_text = "Machine learning is a branch of artificial intelligence."
# Test different embedding models
embedding_results = test_embedding_models(test_text)
for model_name, embedding in embedding_results.items():
print(f"Model: {model_name}, Embedding Shape: {embedding.shape}")
Fine-Tuning for Better Context Representation
Fine-tuning your models with domain-specific data can significantly improve their ability to represent context accurately. This process involves training the model on data that is similar to what it will encounter in actual use.
Example:
from transformers import Trainer, TrainingArguments
# Function to fine-tune a model
def fine_tune_model(model_name, dataset_path):
tokenizer = AutoTokenizer.from_pretrained(model_name)
model = AutoModel.from_pretrained(model_name)
# Load dataset
dataset = load_dataset(dataset_path)
# Data collator
data_collator = DataCollatorForLanguageModeling(tokenizer=tokenizer, mlm=True)
# Training arguments
training_args = TrainingArguments(
output_dir='./results',
num_train_epochs=3,
per_device_train_batch_size=8,
save_steps=10_000,
save_total_limit=2,
)
# Trainer
trainer = Trainer(
model=model,
args=training_args,
data_collator=data_collator,
train_dataset=dataset,
)
# Train the model
trainer.train()
return model
# Fine-tune the model with domain-specific data
fine_tuned_model = fine_tune_model('bert-base-uncased', 'domain_specific_data.txt')
Diagram of Experimentation and Improvement Workflow:
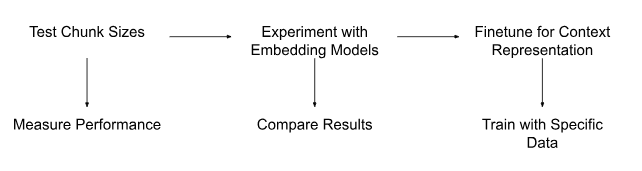
By experimenting with different configurations and models and fine-tuning your system, you can continuously improve the performance of your RAG application. This iterative process ensures that the application remains effective and efficient. Next, we will discuss examples of RAG LLM techniques for serving and scaling your RAG application to handle real-world demands.
Application Serving, Scaling, and Continuous Improvement
Building a robust RAG application is only the beginning. To make it truly useful, you must serve it efficiently, scale it to meet demand, and continuously improve it based on user feedback and performance metrics. Let’s delve into these aspects.
Serving the RAG Application
Serving your RAG application means making it accessible for users to query. This typically involves setting up an API endpoint that handles incoming requests and returns generated responses.
Example:
from flask import Flask, request, jsonify
app = Flask(__name__)
# Load the model and other necessary components
query_rag = setup_rag_application(data_dir, tokenizer, model, generator, index)
@app.route('/query', methods=['POST'])
def query():
data = request.get_json()
query_text = data['query']
response = query_rag(query_text)
return jsonify({'response': response})
if __name__ == '__main__':
app.run(debug=True)
Scaling the RAG Application
To handle multiple queries simultaneously and ensure fast response times, you need to scale your application. Using a RAG LLM example, this involves deploying your application on a robust infrastructure that can manage high traffic and distribute load effectively.
Example:
Horizontal Scaling: Deploy multiple instances of your application and use a load balancer to distribute traffic.
Vertical Scaling: Increase the computational resources (CPU, RAM) of your existing server to handle more requests.
Techniques for Scaling:
Containerization: Use Docker to containerize your application, making it easy to deploy and scale across different environments.
Example:
# Dockerfile for RAG application
FROM python:3.8-slim
WORKDIR /app
COPY requirements.txt requirements.txt
RUN pip install -r requirements.txt
COPY . .
CMD ["python", "app.py"]
Orchestration: Use Kubernetes to manage and scale your containerized application.
Example:
apiVersion: apps/v1
kind: Deployment
metadata:
name: rag-deployment
spec:
replicas: 3
selector:
matchLabels:
app: rag-app
template:
metadata:
labels:
app: rag-app
spec:
containers:
- name: rag-container
image: rag-image
ports:
- containerPort: 5000
Continuous Improvement
To ensure your application remains effective, continuous improvement is crucial. This involves regularly updating your models, refining data, and incorporating user feedback.
Strategies for Continuous Improvement:
Monitor Performance: Regularly track key metrics such as response time, accuracy, and user satisfaction.
Example of Monitoring Metrics:

Feedback Loop: Implement a feedback mechanism where users can rate responses and provide comments. Use this feedback to improve your models and data.
Regular Updates: Schedule regular updates for your models and data to incorporate the latest information and improvements.
Diagram of Application Serving and Scaling Workflow:
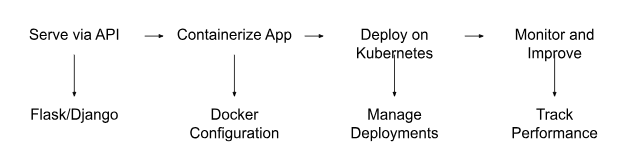
By efficiently serving, scaling, and continuously improving your RAG application, you ensure it remains reliable, responsive, and effective. Next, we will summarize the entire process and discuss the future prospects of integrating RAG into LLM applications.
Conclusion
Building a Retrieval Augmented Generation (RAG) application is a comprehensive process that involves several crucial steps. Using a RAG LLM example, you'll start by understanding the basics of RAG, preparing your database, processing data, building, implementing, and continuously improving your application. Each step plays a vital role in ensuring the success of your project. By following the detailed instructions provided, you can create a robust RAG application that delivers accurate and contextually relevant responses, enhancing the overall user experience.
Raga AI offers a state-of-the-art platform designed to simplify the development and deployment of AI applications. With features such as automated issue detection, diagnosis, and resolution, Raga AI ensures your applications are reliable and high-performing. If you're looking to enhance your AI projects with the latest in RAG technology, consider exploring Raga AI's comprehensive solutions. Start your journey with Raga AI today and take your AI applications to the next level.
Subscribe to our newsletter to never miss an update
Other articles


Agents Talk, Models Think: A2A + MCP for Enterprise Agentic AI

Riya Parikh, Nitai Agarwal
Apr 27, 2025
Read the article
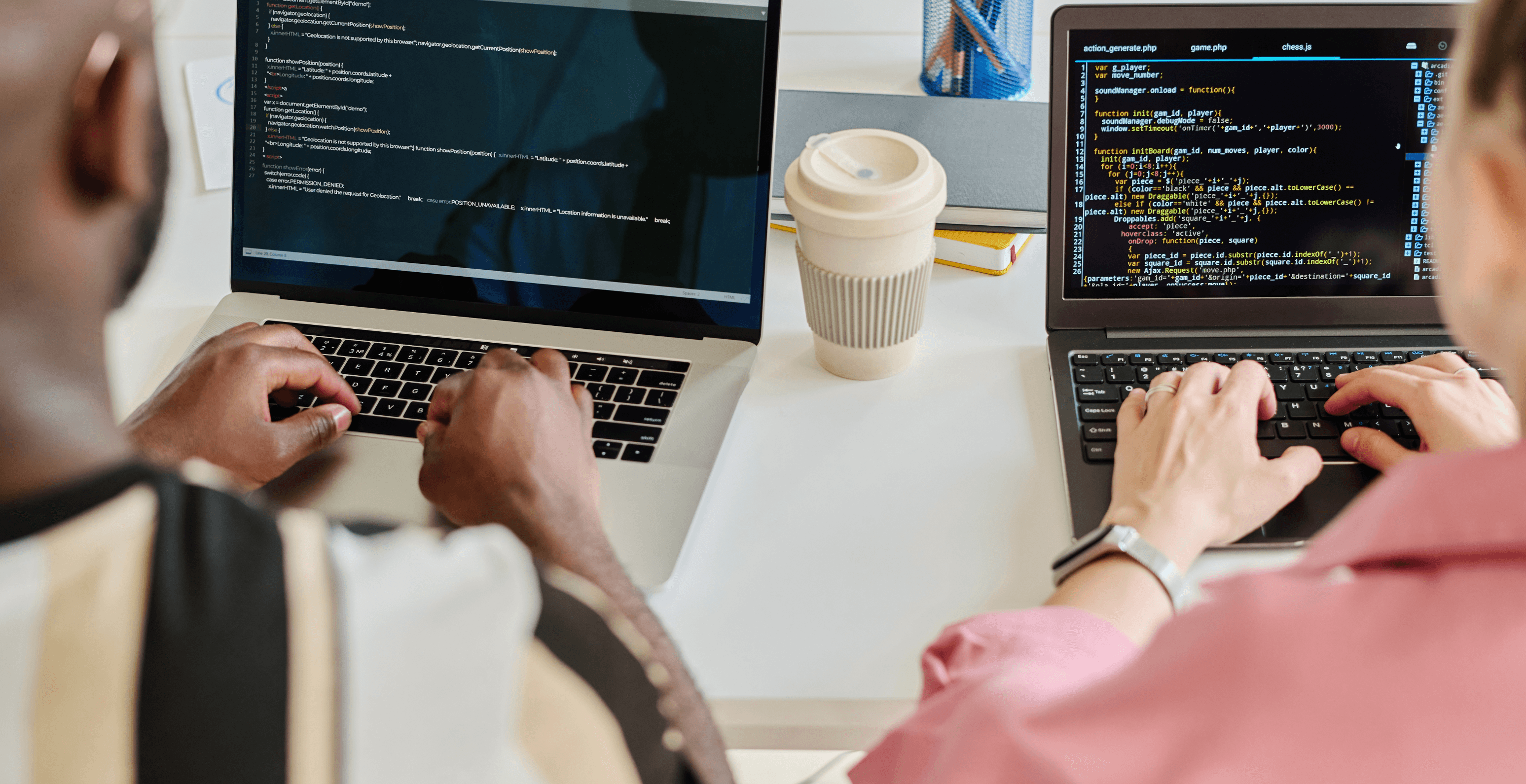
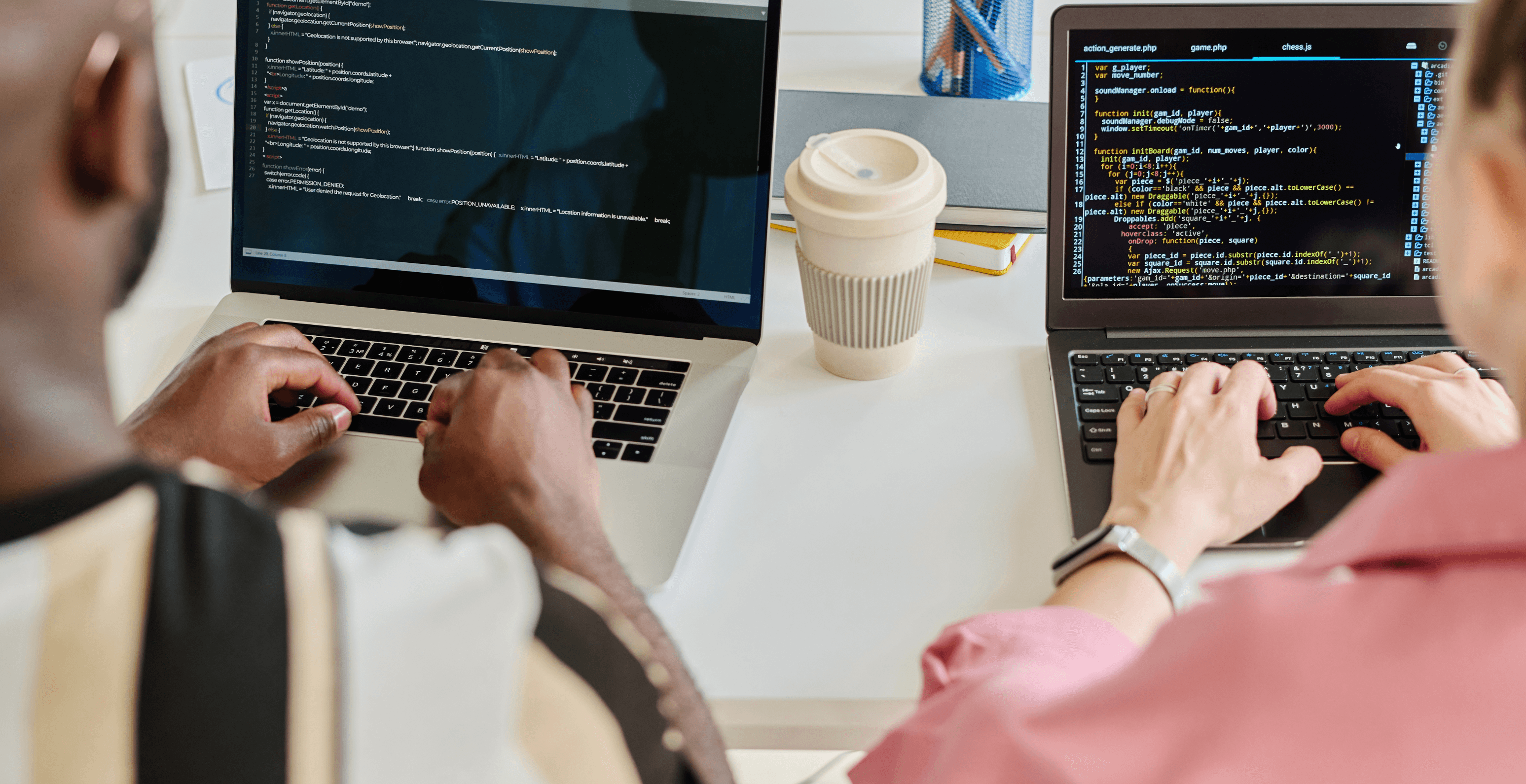
Understanding NeMo Guardrails: A Toolkit for LLM Security

Rehan Asif
Dec 24, 2024
Read the article


Understanding Differences in Large vs Small Language Models (LLM vs SLM)

Rehan Asif
Dec 21, 2024
Read the article