Building Your First LLM Agent Framework Application
Building Your First LLM Agent Framework Application
Building Your First LLM Agent Framework Application
Rehan Asif
Nov 4, 2024
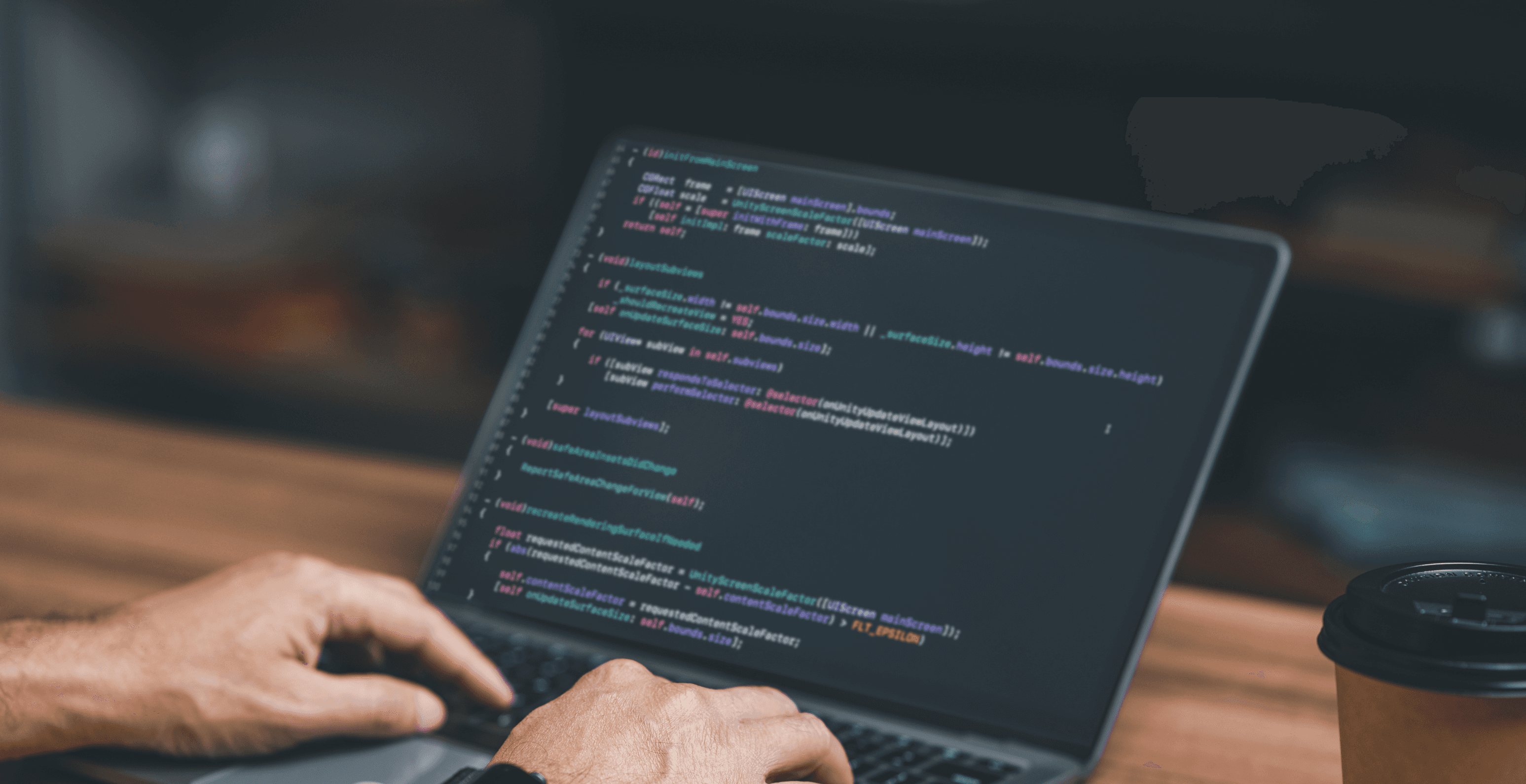
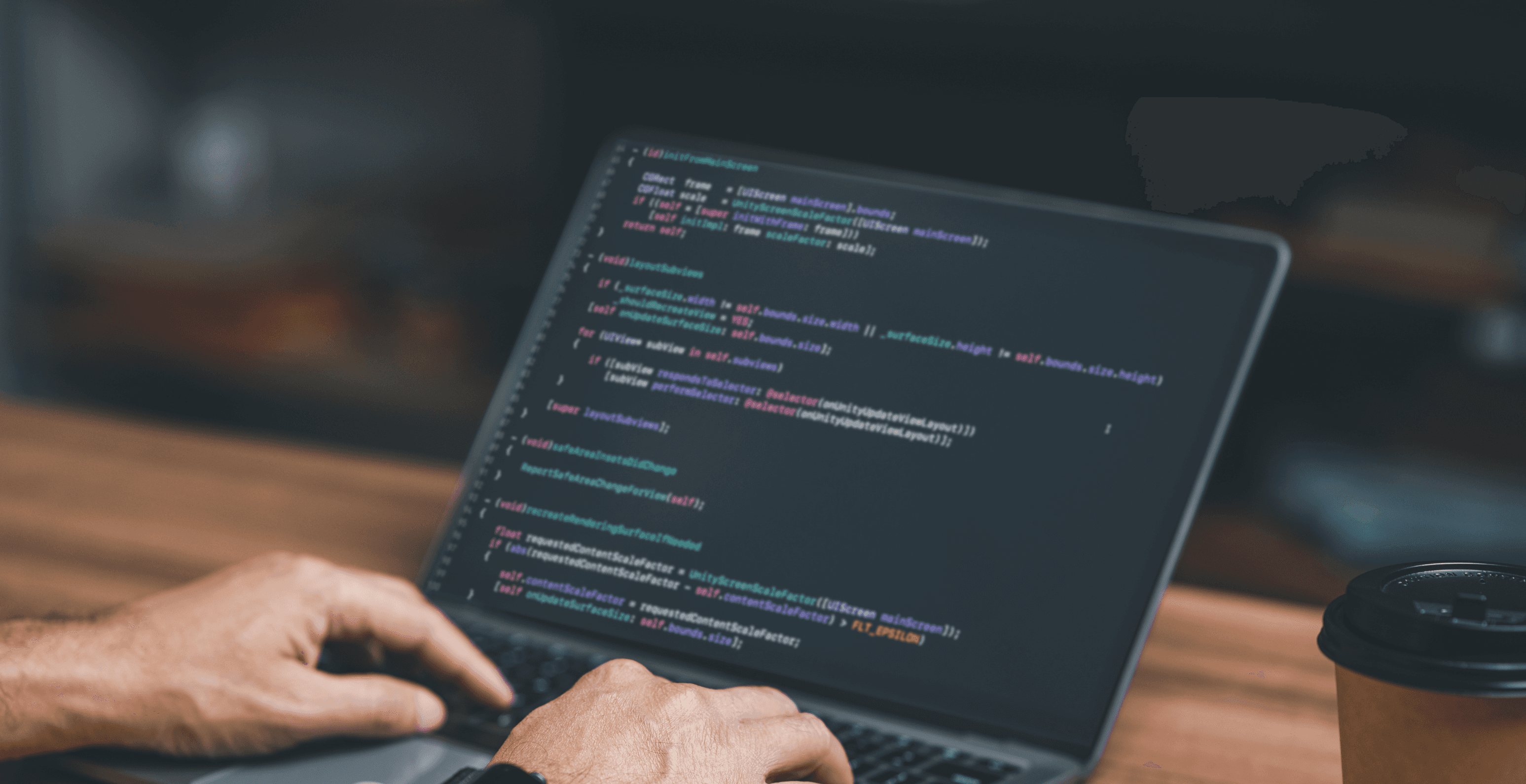
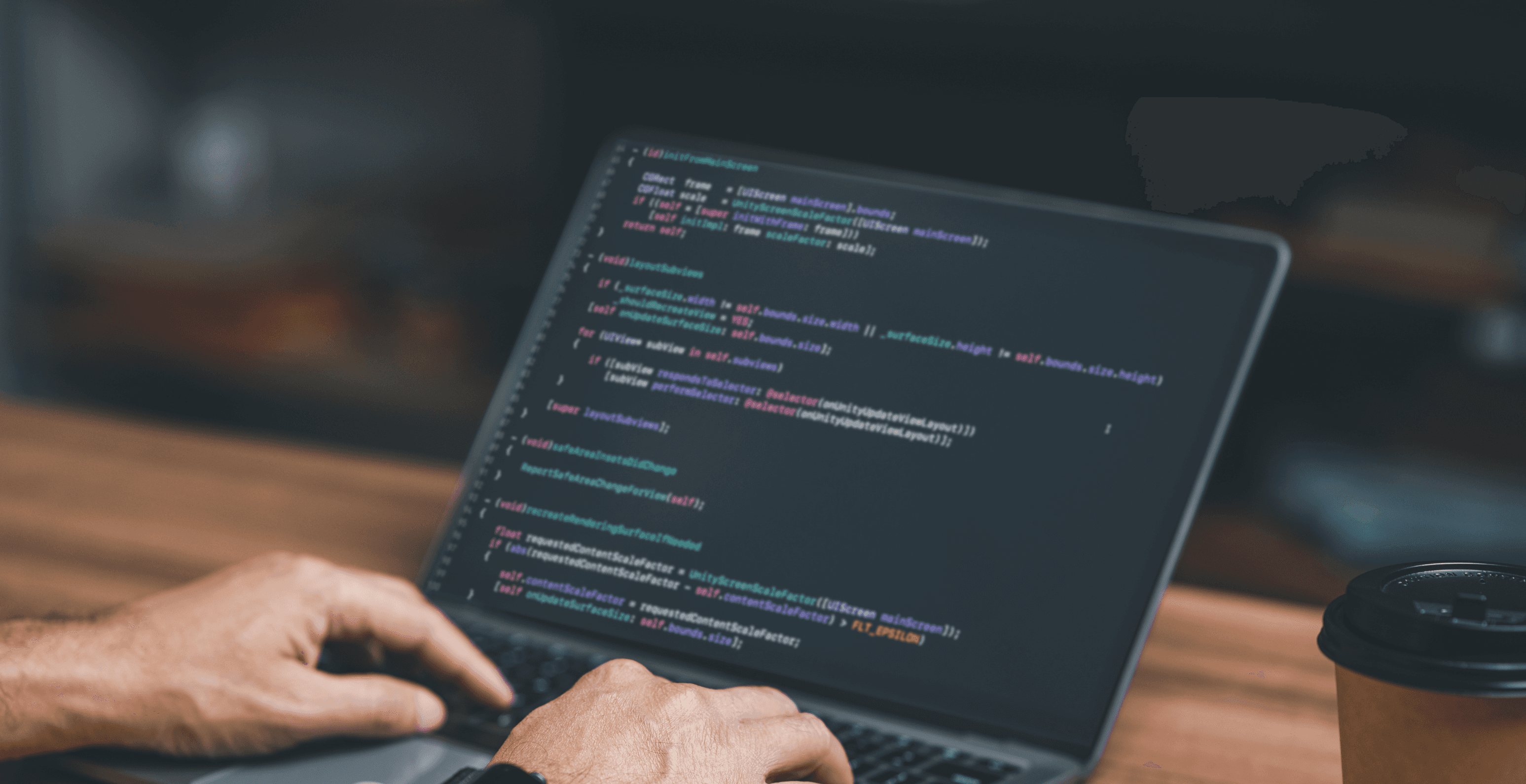
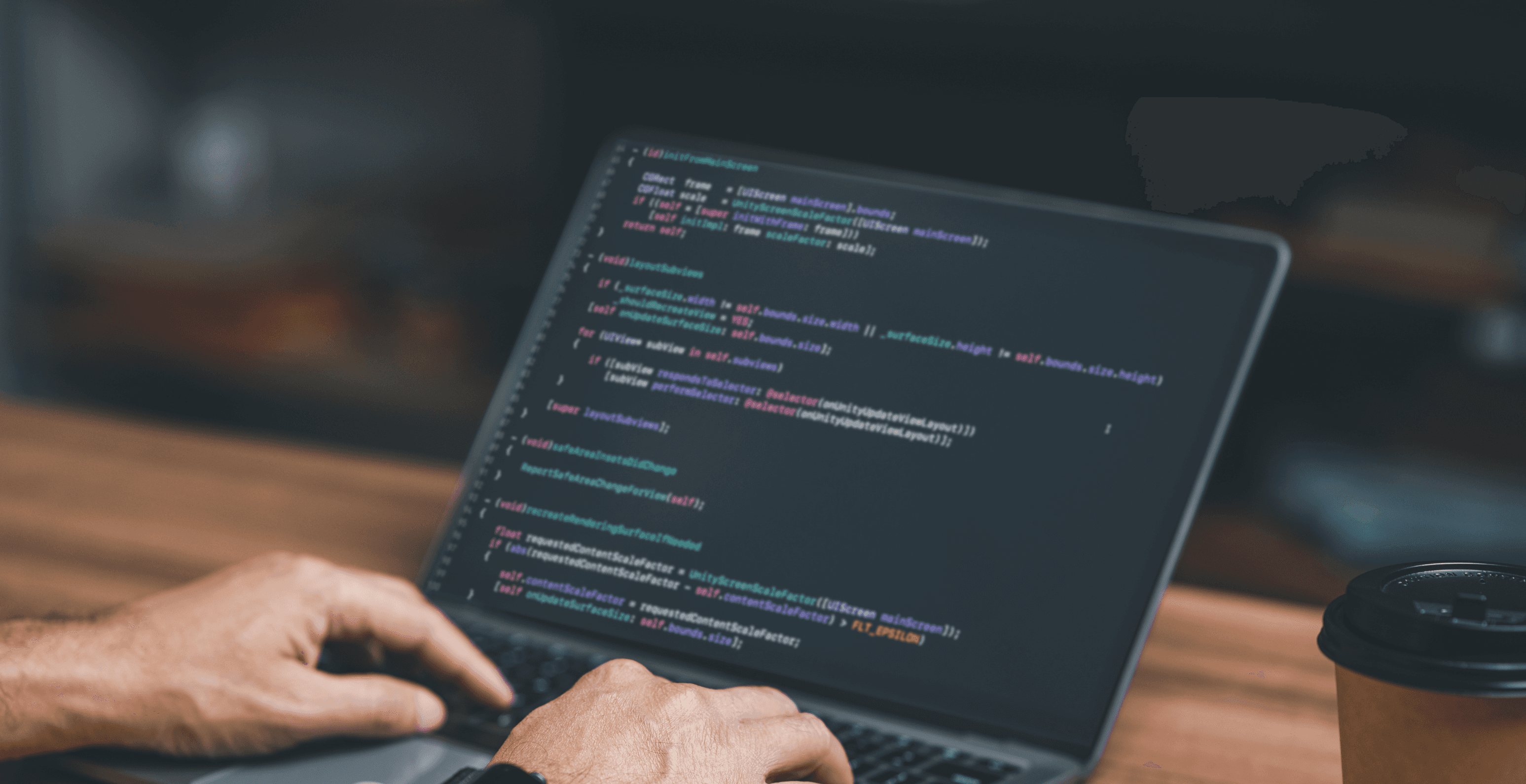
Building your first LLM agent framework application can feel daunting, but it doesn’t have to be. Understanding the core elements—agent cores, memory modules, agent tools, and planning modules—is crucial for success.
In today’s rapidly evolving AI landscape, developing an LLM agent framework application can significantly enhance your AI capabilities. This blog will explore the available implementation frameworks, compare single-agent and multi-agent systems, and highlight popular options to help you make informed decisions. Let’s dive into the developer ecosystem for LLM agents.
LLM Agent Framework Application: Overview
In this section, we will break down the key concepts, starting with LLM agent frameworks, how they differ from traditional chatbots, and the essential components of developing a robust LLM agent application.
LLM Agent Frameworks
LLM agent frameworks are designed to enable large language models to perform complex tasks by integrating multiple functionalities and tools.
Unlike simple chatbot applications, these frameworks allow dynamic problem-solving and advanced data processing. Key aspects include:
Integration of Various Modules
Memory and Planning Modules: These frameworks integrate different components, such as memory modules that help the agent remember past interactions and planning modules that break down complex tasks into simpler, executable steps.
Functional Diversity: They support various functionalities, from data retrieval to executing computational tasks, ensuring a holistic approach to problem-solving.
Enhanced Flexibility
Dynamic Task Handling: Unlike rigid chatbots, LLM agent frameworks can adapt to various tasks and queries, making them suitable for diverse applications.
Learning and Adaptation: They continuously improve from interactions, adapting to new tasks and refining their responses.
Scalability
Single-Agent to Multi-Agent Systems: These frameworks can be scaled from single-agent systems that handle specific tasks to multi-agent systems that manage more complex, interrelated activities.
Resource Management: Efficiently manage computational resources to maintain performance even as the complexity of tasks increases.
LLM Agent Framework Applications vs. Conventional Chatbot Applications
While both LLM agent frameworks and traditional chatbots are used to interact with users through natural language, there are significant differences:
Complexity
LLM Agent Frameworks: Capable of handling multifaceted tasks involving advanced reasoning and decision-making processes. They are designed to go beyond primary query responses to solve complex problems.
Conventional Chatbots: Typically follow predefined scripts and respond to straightforward queries with limited capacity for complex problem-solving.
Adaptability
LLM Agent Frameworks: Continuously learn from each interaction, allowing for improvement and adaptation to new and varied tasks. They can handle unexpected queries and adapt their strategies accordingly.
Conventional Chatbots: Restricted to their initial programming with minimal learning capabilities. They struggle with queries outside their predefined scope.
LLM Agent Applications: Overview of Essential Components Required
Creating a functional LLM agent framework involves integrating several critical components that work together seamlessly:
Agent Core
Central Processing Unit: This unit manages all tasks, decisions, and actions within the framework. It is the brain of the LLM agent, orchestrating how other components interact.
Task Management: Ensures tasks are executed correctly and coordinates between different modules and tools.
Agent Tools
Specialized Functionalities: Include tools for data retrieval, computational analysis, and external API integrations. These tools extend the agent’s capabilities to perform specific tasks efficiently.
Tool Integration: Seamlessly integrates with external tools and services to enhance the agent’s functionality and provide more comprehensive solutions.
Planning Module
Task Decomposition: Breaks down complex queries into manageable sub-tasks, ensuring logical and efficient execution.
Execution Strategy: Develops a plan for tackling each subtask and integrate results to provide coherent answers to complex queries.
By understanding these components, you can build a more efficient and capable LLM agent framework application, setting the stage for advanced AI solutions. This foundational knowledge will help you navigate the complexities of AI development, leading to the successful implementation and deployment of LLM agents in your projects.
With a solid grasp of the fundamental concepts and components of LLM agent framework applications, it's time to explore the tools and frameworks available for developers. Let's dive into the developer ecosystem for LLM agents.
LLM Agent Framework: Developing Ecosystem
The development of the LLM agent framework is supported by a robust ecosystem of frameworks and tools, each offering unique features and capabilities. Understanding these options is essential for selecting the proper framework for your project.
This section will introduce you to the available implementation frameworks, compare single-agent and multi-agent systems, highlight popular frameworks, and discuss community-built solutions.
Developing LLM Agent Framework: Introduction to Available Implementation Frameworks
Several frameworks are available for developing LLM agents, each designed to streamline the development process and enhance functionality. These frameworks provide the building blocks needed to create sophisticated AI agents capable of handling complex tasks.
LangChain: A versatile framework that supports the creation of modular agents with various tools and integrations.
LLaMaIndex: Focuses on indexing and retrieving data efficiently, making it ideal for data-heavy applications.
HayStack: A robust framework designed for building search and question-answering systems.
Example code to initialize a LangChain agent:
from langchain.agents import Agent
# Initialize LangChain agent
agent = Agent()
agent.initialize()
Comparison of Single-Agent vs. Multi-Agent Frameworks
Choosing between single-agent and multi-agent frameworks depends on your application's complexity and requirements. Each type offers distinct advantages and is suited to different use cases.
Single-Agent Frameworks
These frameworks focus on a single AI agent that handles specific tasks independently, offering simplicity and ease of implementation.
Simplicity: Easier to implement and manage.
Focused Tasks: Ideal for applications with specific, isolated tasks.
Example Framework: LangChain.
Example code to create a single-agent system:
from langchain.agents import SingleAgent
# Create a single-agent instance
agent = SingleAgent()
agent.run()
Multi-Agent Frameworks
These frameworks involve multiple AI agents working together, enabling complex interactions and scalability for more intricate applications.
Complex Interactions: Capable of handling multiple, interconnected tasks.
Scalability: Suitable for large-scale applications requiring coordination between agents.
Example Framework: AgentVerse.
Example code to create a multi-agent system:
from agentverse import MultiAgentSystem
# Create a multi-agent system
system = MultiAgentSystem()
system.initialize_agents(num_agents=5)
system.run()
Highlighting Popular Frameworks
Several frameworks have gained popularity due to their robust features and community support. Here are some of the top choices for developing LLM agents:
LangChain Agents
Modular Design: Easily integrates with various tools and services.
Strong Community: Extensive documentation and community support.
from langchain.agents import LangChainAgent
# Initialize LangChain agent with specific tools
agent = LangChainAgent(tools=['tool1', 'tool2'])
agent.run()
LLaMaIndex Agents
Efficient Data Handling: Optimized for indexing and data retrieval.
Scalable: Handles large datasets effectively.
from llamaindex import LLaMaAgent
# Initialize LLaMa agent for data indexing
agent = LLaMaAgent(index_path='path/to/index')
agent.index_data(data_source='data/source/path')
HayStack Agents
Search and QA: Built for creating powerful search engines and question-answering systems.
Flexibility: Supports a variety of backends and data sources.
from haystack.agents import HayStackAgent
# Initialize HayStack agent for QA
agent = HayStackAgent()
agent.build_qa_pipeline(model_name='bert-base-uncased')
AutoGen
Automated Code Generation: Facilitates the automatic generation of code based on user input.
Ease of Use: Simplifies the development process with minimal manual coding.
from autogen import AutoGenAgent
# Initialize AutoGen agent
agent = AutoGenAgent()
agent.generate_code(prompt='Create a function to add two numbers')
AgentVerse
Multi-Agent Systems: Designed for creating and managing complex multi-agent interactions.
Simulation Environment: Provides tools for simulating agent interactions.
from agentverse import AgentVerseSystem
# Initialize AgentVerse system
system = AgentVerseSystem()
system.add_agents(['agent1', 'agent2'])
system.simulate()
ChatDev
Chatbot Development: Specialized in creating advanced chatbot applications.
Customization: Highly customizable to suit specific business needs.
from chatdev import ChatBot
# Initialize ChatDev chatbot
chatbot = ChatBot()
chatbot.train(training_data='path/to/training/data')
Generative Agents
Simulated Human Behavior: Creates agents that mimic human interactions.
Advanced AI: Uses cutting-edge AI techniques for more realistic simulations.
from generativeagents import SimulacraAgent
# Initialize Generative Agent
agent = SimulacraAgent()
agent.simulate_behavior(behavior_model='human-like')
Explanation of Community-Built Frameworks, Including Usage and Features
Community-built frameworks often provide innovative solutions and additional features, contributed by developers worldwide. These frameworks can be invaluable for specific needs and offer unique advantages.
Customization: Tailored to niche use cases and specific project requirements.
Open Source: Freely available and often supported by active developer communities.
Innovative Features: Community contributions often lead to rapidly developing new and valuable features.
With these tools and frameworks, you can develop powerful LLM agent applications. Next, let's explore how to choose the right agent framework for your specific needs.
LLM Agent Framework App Build: Choosing the Right Agent Framework
Selecting the right agent framework is crucial in building practical LLM agent applications. The choice depends on various factors, including the complexity of tasks, scalability requirements, and the specific features needed for the LLM agent framework application build.
This section will guide you through the evaluation criteria for selecting an agent framework, highlight the differences between single-agent and multi-agent frameworks, and discuss the importance of simulation environments for multi-agent systems.
Evaluation Criteria for Selecting an Agent Framework
When choosing an agent framework, it’s essential to consider several key criteria to ensure that the framework aligns with your project requirements and goals.
Scalability
Handling Load: The framework should be capable of handling the maximum load you expect, whether it’s the number of requests per second or the amount of data processed.
Growth Potential: It should be able to grow with your project, accommodating more complex tasks and larger datasets over time.
Flexibility
Modular Design: Supports adding, removing, or modifying components without significant rework.
Community and Support
Documentation: Comprehensive and clear documentation to help you understand and use the framework effectively.
Community Engagement: An active community can provide support, plugins, and shared experiences that can be invaluable.
Ease of Use
Learning Curve: How quickly your team can get up to speed with the framework.
Implementation Simplicity: How easy it is to implement and start building with the framework.
Importance of Simulation Environments for Multi-Agent Systems
Simulation environments play a vital role in the development and testing of multi-agent systems. They provide a controlled setting where agents can interact, perform tasks, and adapt to various scenarios, ensuring robust performance before deployment.
Controlled Testing
Allows for thorough testing of agent interactions and behaviors in a risk-free environment.
Identifies potential issues and bugs in the early stages of development.
Performance Optimization
Helps in identifying bottlenecks and optimizing agent performance.
Provides insights into how agents can be improved and fine-tuned for better results.
Scenario Analysis
Enables the simulation of different scenarios to assess agent responses and adapt strategies accordingly.
Tests the robustness of agents under various conditions and stresses.
With these guidelines, you can make informed decisions when selecting the right agent framework for your project, ensuring that it meets your specific needs and delivers optimal performance.
Now, let's delve deeper into the building blocks of an LLM agent framework application.
LLM Agent Frameworks: Building Blocks
Creating a practical LLM agent framework requires assembling several vital components that work together seamlessly. These components form the backbone of any robust LLM agent, ensuring it can handle complex tasks and provide accurate responses.
This section will explore the essential tools and modules needed to develop a QA agent, discuss the significance of the Retrieval-augmented generation (RAG) pipeline, and delve into the development of mathematical tools, question decomposition modules, and memory modules.
Tools Required for Developing a QA Agent
Developing a QA agent necessitates a suite of tools that enhance its ability to accurately understand and respond to queries. These tools are integral to building a responsive and efficient agent.
Natural Language Processing (NLP) Library
Tools like SpaCy or NLTK for text processing and understanding.
Pre-trained models for language comprehension.
Machine Learning Framework
Libraries such as TensorFlow or PyTorch for model development and training.
Pre-trained models like BERT or GPT for question answering.
Data Retrieval Tools
Systems like Elasticsearch for indexing and retrieving relevant documents.
APIs to fetch data from external sources.
Retrieval-Augmented Generation (RAG) Pipeline & Its Significance
RAG pipeline is a critical component for enhancing the accuracy and relevance of responses in LLM agents. It combines the strengths of retrieval-based and generation-based methods to provide precise answers.
Components of RAG
Retriever: Fetches relevant documents or passages from a large corpus.
Generator: Uses the retrieved documents to generate detailed and accurate answers.
from transformers import RagTokenizer, RagRetriever, RagSequenceForGeneration
# Initialize tokenizer, retriever, and generator
tokenizer = RagTokenizer.from_pretrained("facebook/rag-sequence-nq")
retriever = RagRetriever.from_pretrained("facebook/rag-sequence-nq", index_name="exact")
rag_sequence = RagSequenceForGeneration.from_pretrained("facebook/rag-sequence-nq", retriever=retriever)
input_ids = tokenizer("What is the capital of France?", return_tensors="pt")["input_ids"]
generated = rag_sequence.generate(input_ids)
print(tokenizer.batch_decode(generated, skip_special_tokens=True))
Significance of RAG
Improved Accuracy: Enhances the precision of responses by leveraging vast amounts of data.
Development of a Mathematical Tool for Planning
A mathematical tool is essential for performing calculations and planning tasks within the agent framework. This tool helps the agent handle numerical data and perform necessary computations.
Capabilities
Basic Calculations: Addition, subtraction, multiplication, and division.
Advanced Functions: Algebra, calculus, and statistical analysis.
import sympy as sp
# Define symbols
x, y = sp.symbols('x y')
# Perform calculations
equation = sp.Eq(x + y, 10)
solution = sp.solve(equation, x)
print(solution)
Integration
Easily integrates with other modules to perform computations as required.
Provides accurate and reliable results for complex queries.
Construction of a Question Decomposition Module
A question decomposition module is vital for breaking down complex questions into simpler, manageable parts. This ensures that the agent can tackle intricate queries methodically.
Functionality
Question Parsing: Identifies and separates different parts of a complex question.
Task Delegation: Assigns sub-tasks to appropriate modules for processing.
def decompose_question(question):
# Example decomposition logic
if "and" in question:
parts = question.split("and")
else:
parts = [question]
return parts
# Decompose a complex question
question = "What is the capital of France and who is the president?"
sub_questions = decompose_question(question)
print(sub_questions)
With these building blocks in place, you can develop a robust and efficient LLM agent framework application capable of handling complex tasks and providing accurate answers. Next, we will explore how to develop the core of your LLM agent, integrating these components into a cohesive system.
Developing the Core of Your LLM Agent
The core of your LLM agent is the central processing unit that orchestrates all its operations. This section covers the different types of solvers used in the agent core, the integration process for various components such as tools, planners, and memory modules, and the evaluation of the mental model to ensure the agent's effectiveness.
Developing a robust core is crucial for the agent's ability to handle complex tasks efficiently and accurately.
Introduction to Different Solver Types for the Agent Core
Choosing the right solver type for your agent core is essential for optimizing performance and task management. Different solvers offer unique benefits and are suited to various levels of complexity in problem-solving.
Linear Solver
Simple Execution: Follows a straightforward, linear path to solve tasks.
Ease of Implementation: Suitable for simpler tasks that do not require complex decision-making processes.
def linear_solver(tasks):
results = []
for task in tasks:
result = perform_task(task)
results.append(result)
return results
def perform_task(task):
# Define task execution logic here
return f"Result of {task}"
tasks = ["task1", "task2", "task3"]
print(linear_solver(tasks))
Single-Thread Recursive Solver
Depth-First Approach: Solves tasks by recursively breaking them into sub-tasks and processing them individually.
Improved Problem-Solving: Suitable for tasks that require a more detailed and step-by-step approach.
def single_thread_recursive_solver(task):
if is_complex_task(task):
sub_tasks = decompose_task(task)
results = [single_thread_recursive_solver(sub_task) for sub_task in sub_tasks]
return combine_results(results)
else:
return perform_task(task)
def is_complex_task(task):
# Define logic to determine if a task is complex
return "complex" in task
def decompose_task(task):
# Define logic to decompose task into sub-tasks
return [f"{task}_part1", f"{task}_part2"]
def combine_results(results):
# Define logic to combine results of sub-tasks
return " & ".join(results)
def perform_task(task):
# Define task execution logic here
return f"Result of {task}"
task = "complex_task"
print(single_thread_recursive_solver(task)
Multi-Thread Recursive Solver
Parallel Processing: Executes multiple tasks or sub-tasks simultaneously, improving efficiency and reducing latency.
High Complexity Handling: Ideal for handling highly complex tasks that benefit from concurrent execution.
import concurrent.futures
def multi_thread_recursive_solver(task):
if is_complex_task(task):
sub_tasks = decompose_task(task)
with concurrent.futures.ThreadPoolExecutor() as executor:
results = list(executor.map(multi_thread_recursive_solver, sub_tasks))
return combine_results(results)
else:
return perform_task(task)
# Reuse the previously defined functions is_complex_task, decompose_task, combine_results, and perform_task
task = "complex_task"
print(multi_thread_recursive_solver(task))
Integration Process of Tools, Planner, and Memory Module in the Agent Setup
Integrating the various components of your LLM agent—such as tools, the planner, and the memory module—is critical to ensure they work together seamlessly and efficiently. This process involves setting up communication channels and workflows between these components.
Tools Integration
Setup and Configuration: Initialize and configure the tools required for the agent to perform specific tasks.
Inter-Component Communication: Ensure that the tools can communicate effectively with other components like the planner and memory module.
class Agent:
def __init__(self, tools, planner, memory):
self.tools = tools
self.planner = planner
self.memory = memory
def integrate_tools(self):
for tool in self.tools:
tool.setup()
print("Tools integrated successfully")
class Tool:
def setup(self):
# Define tool setup logic here
print("Tool setup complete")
tools = [Tool(), Tool()]
agent = Agent(tools, None, None)
agent.integrate_tools()
Planner Integration
Task Decomposition: The planner decomposes complex tasks into manageable sub-tasks.
Task Assignment: Assigns sub-tasks to the appropriate tools and manages their execution.
class Planner:
def __init__(self):
self.plan = []
def create_plan(self, task):
self.plan = self.decompose_task(task)
def decompose_task(self, task):
# Decompose the task into sub-tasks
return [f"{task}_sub1", f"{task}_sub2"]
def assign_tasks(self, agent):
for sub_task in self.plan:
agent.execute_task(sub_task)
planner = Planner()
planner.create_plan("complex_task")
Memory Module Integration
Information Retrieval: Retrieves relevant information from past interactions to aid in current task processing.
class MemoryModule:
def __init__(self):
self.memory = []
def store_interaction(self, question, answer):
self.memory.append({"question": question, "answer": answer})
def retrieve_interaction(self, question):
for interaction in self.memory:
if interaction["question"] == question:
return interaction["answer"]
return None
memory = MemoryModule()
memory.store_interaction("What is the capital of France?", "Paris")
Full Integration Example
Combining All Components: Integrate tools, the planner, and the memory module into the agent setup.
class FullAgent:
def __init__(self, tools, planner, memory):
self.tools = tools
self.planner = planner
self.memory = memory
def execute_task(self, task):
if task in [t.get_task_name() for t in self.tools]:
tool = next(t for t in self.tools if t.get_task_name() == task)
return tool.perform_task()
else:
return "Task not supported"
tools = [Tool(), Tool()]
planner = Planner()
memory = MemoryModule()
agent = FullAgent(tools, planner, memory)
# Integrate tools, create plan, and execute tasks
agent.integrate_tools()
planner.create_plan("complex_task")
planner.assign_tasks(agent)
Evaluation of the Mental Model for Agent Effectiveness
Evaluating your LLM agent's mental model is crucial to ensuring its effectiveness and efficiency. This involves testing the agent's reasoning capabilities, ability to handle various tasks, and overall performance in real-world scenarios.
Reasoning Capabilities
Logic and Decision-Making: Assess how well the agent can make logical decisions based on available information.
Complex Problem Solving: Test the agent's ability to solve complex problems through reasoning.
Task Handling
Task Diversity: Evaluate the agent's ability to handle various tasks, from simple queries to complex multi-step processes.
Efficiency: Measure the speed and accuracy with which the agent completes tasks.
Performance Metrics
Accuracy: Determine the accuracy of the agent's responses and its success rate in task completion.
User Satisfaction: Gather user feedback to assess the effectiveness and usability of the agent.
class AgentEvaluator:
def __init__(self, agent):
self.agent = agent
def evaluate(self, tasks):
results = []
for task in tasks:
result = self.agent.execute_task(task)
results.append(result)
return results
def calculate_accuracy(self, results, expected_results):
correct = sum([1 for result, expected in zip(results, expected_results) if result == expected])
return correct / len(results)
# Example usage
evaluator = AgentEvaluator(agent)
tasks = ["simple_task", "complex_task_sub1", "complex_task_sub2"]
results = evaluator.evaluate(tasks)
expected_results = ["Result of simple_task", "Result of complex_task_sub1", "Result of complex_task_sub2"]
accuracy = evaluator.calculate_accuracy(results, expected_results)
print(f"Agent Accuracy: {accuracy * 100}%")
By developing a robust core and integrating various components effectively, your LLM agent can perform complex tasks with high efficiency and accuracy. Evaluating the agent's mental model ensures that it remains effective and reliable in real-world applications.
Recommended Reading and Resources
Exploring additional resources and reading materials is essential to further enhancing your understanding and capabilities in developing LLM agent frameworks.
These resources provide in-depth knowledge, case studies, and practical examples to help you build more sophisticated and effective LLM agents.
AutoGPT, Voyager, OlaGPT, MRKL Systems, Generative Agents: Interactive Simulacra of Human Behavior
Exploring the following projects and systems will give you a comprehensive view of the state-of-the-art LLM agent development and the potential for creating advanced AI applications.
AutoGPT
Overview: AutoGPT is an innovative project that automates the generation of GPT-based agents. It showcases the potential for automating complex tasks using language models.
Key Features: Automated task handling, minimal human intervention, and adaptive learning capabilities.
Useful Links: AutoGPT GitHub Repository
Voyager
Overview: Voyager is a project by NVIDIA Research that focuses on self-improving agents capable of learning new tools and building new capabilities without external intervention.
Key Features: Self-improvement, autonomous tool learning, and advanced problem-solving skills.
Useful Links: Voyager Project Page
OlaGPT
Overview: OlaGPT is a conceptual framework that stimulates ideas on how to extend the capabilities of basic agents. It focuses on enhancing agent interactions and performance.
Key Features: Conceptual extensions, interaction enhancements, and performance optimization.
Useful Links: OlaGPT Research Paper
MRKL Systems
Overview: MRKL Systems (Modular Reasoning, Knowledge, and Language) combine large language models with external knowledge sources and discrete reasoning for executing complex tasks.
Key Features: Integration of language models with knowledge bases, modular architecture, and enhanced reasoning capabilities.
Useful Links: MRKL Systems Paper
Generative Agents: Interactive Simulacra of Human Behavior
Overview: This project explores the creation of agents that simulate human behavior through decentralized interactions. It's one of the pioneering efforts in developing swarm intelligence.
Key Features: Decentralized agent interactions, human-like behavior simulation, and advanced AI techniques.
Useful Links: Generative Agents Research
LLM-Powered Agent List for Further Reading and Resources
The Awesome LLM-Powered Agent list is a curated collection of resources, tools, and examples that can provide further insights and practical knowledge for developing LLM agents. It is a valuable resource for both beginners and experienced developers.
Overview
The list includes various topics, from foundational theories to advanced implementations, and is regularly updated by the community.
Key Sections
Tutorials and Guides: Step-by-step tutorials and guides for building LLM agents.
Libraries and Frameworks: Information on various libraries and frameworks that support LLM agent development.
Case Studies: Real-world examples and case studies showcasing successful LLM agent implementations.
Research Papers: Access to significant research papers that provide theoretical and practical insights.
Useful Links
Excellent LLM-Powered Agent List: Awesome LLM-Powered Agent GitHub Repository
Additional Resources: NVIDIA AI Playground
By diving into these recommended readings and resources, you will gain a deeper understanding of the advanced concepts and practical applications of LLM agent frameworks. These materials will help you stay updated with the latest developments and enhance your skills in building sophisticated AI agents.
Summary
Building an LLM agent framework application involves understanding and integrating key components such as solvers, tools, planners, and memory modules. Each element is crucial in ensuring the agent's efficiency, accuracy, and contextual relevance. You can create robust and effective LLM agents by leveraging recommended frameworks and resources.
Looking ahead, the future of LLM agent applications is bright. They have the potential to revolutionize various industries by automating complex tasks and enhancing user interactions. The continuous advancements in AI and machine learning will drive further improvements in agent capabilities and applications. Embracing these innovations will allow businesses to unlock new levels of productivity and efficiency.
How Can RAGA AI Help You in Developing Your First LLM Agent Framework Application?
RAGA AI offers a comprehensive and user-friendly platform designed to simplify developing your first LLM agent framework application. With its robust set of tools and integrated modules, RAGA AI enables you to seamlessly build, test, and deploy advanced LLM agents without extensive technical expertise.
Additionally, RAGA AI's innovative features, such as automated issue detection, memory modules, and planning tools, ensure that your agents perform efficiently and accurately, help.
So Sign Up for RAGA's LLM Hub and get your LLM applications ready 3X faster!
Building your first LLM agent framework application can feel daunting, but it doesn’t have to be. Understanding the core elements—agent cores, memory modules, agent tools, and planning modules—is crucial for success.
In today’s rapidly evolving AI landscape, developing an LLM agent framework application can significantly enhance your AI capabilities. This blog will explore the available implementation frameworks, compare single-agent and multi-agent systems, and highlight popular options to help you make informed decisions. Let’s dive into the developer ecosystem for LLM agents.
LLM Agent Framework Application: Overview
In this section, we will break down the key concepts, starting with LLM agent frameworks, how they differ from traditional chatbots, and the essential components of developing a robust LLM agent application.
LLM Agent Frameworks
LLM agent frameworks are designed to enable large language models to perform complex tasks by integrating multiple functionalities and tools.
Unlike simple chatbot applications, these frameworks allow dynamic problem-solving and advanced data processing. Key aspects include:
Integration of Various Modules
Memory and Planning Modules: These frameworks integrate different components, such as memory modules that help the agent remember past interactions and planning modules that break down complex tasks into simpler, executable steps.
Functional Diversity: They support various functionalities, from data retrieval to executing computational tasks, ensuring a holistic approach to problem-solving.
Enhanced Flexibility
Dynamic Task Handling: Unlike rigid chatbots, LLM agent frameworks can adapt to various tasks and queries, making them suitable for diverse applications.
Learning and Adaptation: They continuously improve from interactions, adapting to new tasks and refining their responses.
Scalability
Single-Agent to Multi-Agent Systems: These frameworks can be scaled from single-agent systems that handle specific tasks to multi-agent systems that manage more complex, interrelated activities.
Resource Management: Efficiently manage computational resources to maintain performance even as the complexity of tasks increases.
LLM Agent Framework Applications vs. Conventional Chatbot Applications
While both LLM agent frameworks and traditional chatbots are used to interact with users through natural language, there are significant differences:
Complexity
LLM Agent Frameworks: Capable of handling multifaceted tasks involving advanced reasoning and decision-making processes. They are designed to go beyond primary query responses to solve complex problems.
Conventional Chatbots: Typically follow predefined scripts and respond to straightforward queries with limited capacity for complex problem-solving.
Adaptability
LLM Agent Frameworks: Continuously learn from each interaction, allowing for improvement and adaptation to new and varied tasks. They can handle unexpected queries and adapt their strategies accordingly.
Conventional Chatbots: Restricted to their initial programming with minimal learning capabilities. They struggle with queries outside their predefined scope.
LLM Agent Applications: Overview of Essential Components Required
Creating a functional LLM agent framework involves integrating several critical components that work together seamlessly:
Agent Core
Central Processing Unit: This unit manages all tasks, decisions, and actions within the framework. It is the brain of the LLM agent, orchestrating how other components interact.
Task Management: Ensures tasks are executed correctly and coordinates between different modules and tools.
Agent Tools
Specialized Functionalities: Include tools for data retrieval, computational analysis, and external API integrations. These tools extend the agent’s capabilities to perform specific tasks efficiently.
Tool Integration: Seamlessly integrates with external tools and services to enhance the agent’s functionality and provide more comprehensive solutions.
Planning Module
Task Decomposition: Breaks down complex queries into manageable sub-tasks, ensuring logical and efficient execution.
Execution Strategy: Develops a plan for tackling each subtask and integrate results to provide coherent answers to complex queries.
By understanding these components, you can build a more efficient and capable LLM agent framework application, setting the stage for advanced AI solutions. This foundational knowledge will help you navigate the complexities of AI development, leading to the successful implementation and deployment of LLM agents in your projects.
With a solid grasp of the fundamental concepts and components of LLM agent framework applications, it's time to explore the tools and frameworks available for developers. Let's dive into the developer ecosystem for LLM agents.
LLM Agent Framework: Developing Ecosystem
The development of the LLM agent framework is supported by a robust ecosystem of frameworks and tools, each offering unique features and capabilities. Understanding these options is essential for selecting the proper framework for your project.
This section will introduce you to the available implementation frameworks, compare single-agent and multi-agent systems, highlight popular frameworks, and discuss community-built solutions.
Developing LLM Agent Framework: Introduction to Available Implementation Frameworks
Several frameworks are available for developing LLM agents, each designed to streamline the development process and enhance functionality. These frameworks provide the building blocks needed to create sophisticated AI agents capable of handling complex tasks.
LangChain: A versatile framework that supports the creation of modular agents with various tools and integrations.
LLaMaIndex: Focuses on indexing and retrieving data efficiently, making it ideal for data-heavy applications.
HayStack: A robust framework designed for building search and question-answering systems.
Example code to initialize a LangChain agent:
from langchain.agents import Agent
# Initialize LangChain agent
agent = Agent()
agent.initialize()
Comparison of Single-Agent vs. Multi-Agent Frameworks
Choosing between single-agent and multi-agent frameworks depends on your application's complexity and requirements. Each type offers distinct advantages and is suited to different use cases.
Single-Agent Frameworks
These frameworks focus on a single AI agent that handles specific tasks independently, offering simplicity and ease of implementation.
Simplicity: Easier to implement and manage.
Focused Tasks: Ideal for applications with specific, isolated tasks.
Example Framework: LangChain.
Example code to create a single-agent system:
from langchain.agents import SingleAgent
# Create a single-agent instance
agent = SingleAgent()
agent.run()
Multi-Agent Frameworks
These frameworks involve multiple AI agents working together, enabling complex interactions and scalability for more intricate applications.
Complex Interactions: Capable of handling multiple, interconnected tasks.
Scalability: Suitable for large-scale applications requiring coordination between agents.
Example Framework: AgentVerse.
Example code to create a multi-agent system:
from agentverse import MultiAgentSystem
# Create a multi-agent system
system = MultiAgentSystem()
system.initialize_agents(num_agents=5)
system.run()
Highlighting Popular Frameworks
Several frameworks have gained popularity due to their robust features and community support. Here are some of the top choices for developing LLM agents:
LangChain Agents
Modular Design: Easily integrates with various tools and services.
Strong Community: Extensive documentation and community support.
from langchain.agents import LangChainAgent
# Initialize LangChain agent with specific tools
agent = LangChainAgent(tools=['tool1', 'tool2'])
agent.run()
LLaMaIndex Agents
Efficient Data Handling: Optimized for indexing and data retrieval.
Scalable: Handles large datasets effectively.
from llamaindex import LLaMaAgent
# Initialize LLaMa agent for data indexing
agent = LLaMaAgent(index_path='path/to/index')
agent.index_data(data_source='data/source/path')
HayStack Agents
Search and QA: Built for creating powerful search engines and question-answering systems.
Flexibility: Supports a variety of backends and data sources.
from haystack.agents import HayStackAgent
# Initialize HayStack agent for QA
agent = HayStackAgent()
agent.build_qa_pipeline(model_name='bert-base-uncased')
AutoGen
Automated Code Generation: Facilitates the automatic generation of code based on user input.
Ease of Use: Simplifies the development process with minimal manual coding.
from autogen import AutoGenAgent
# Initialize AutoGen agent
agent = AutoGenAgent()
agent.generate_code(prompt='Create a function to add two numbers')
AgentVerse
Multi-Agent Systems: Designed for creating and managing complex multi-agent interactions.
Simulation Environment: Provides tools for simulating agent interactions.
from agentverse import AgentVerseSystem
# Initialize AgentVerse system
system = AgentVerseSystem()
system.add_agents(['agent1', 'agent2'])
system.simulate()
ChatDev
Chatbot Development: Specialized in creating advanced chatbot applications.
Customization: Highly customizable to suit specific business needs.
from chatdev import ChatBot
# Initialize ChatDev chatbot
chatbot = ChatBot()
chatbot.train(training_data='path/to/training/data')
Generative Agents
Simulated Human Behavior: Creates agents that mimic human interactions.
Advanced AI: Uses cutting-edge AI techniques for more realistic simulations.
from generativeagents import SimulacraAgent
# Initialize Generative Agent
agent = SimulacraAgent()
agent.simulate_behavior(behavior_model='human-like')
Explanation of Community-Built Frameworks, Including Usage and Features
Community-built frameworks often provide innovative solutions and additional features, contributed by developers worldwide. These frameworks can be invaluable for specific needs and offer unique advantages.
Customization: Tailored to niche use cases and specific project requirements.
Open Source: Freely available and often supported by active developer communities.
Innovative Features: Community contributions often lead to rapidly developing new and valuable features.
With these tools and frameworks, you can develop powerful LLM agent applications. Next, let's explore how to choose the right agent framework for your specific needs.
LLM Agent Framework App Build: Choosing the Right Agent Framework
Selecting the right agent framework is crucial in building practical LLM agent applications. The choice depends on various factors, including the complexity of tasks, scalability requirements, and the specific features needed for the LLM agent framework application build.
This section will guide you through the evaluation criteria for selecting an agent framework, highlight the differences between single-agent and multi-agent frameworks, and discuss the importance of simulation environments for multi-agent systems.
Evaluation Criteria for Selecting an Agent Framework
When choosing an agent framework, it’s essential to consider several key criteria to ensure that the framework aligns with your project requirements and goals.
Scalability
Handling Load: The framework should be capable of handling the maximum load you expect, whether it’s the number of requests per second or the amount of data processed.
Growth Potential: It should be able to grow with your project, accommodating more complex tasks and larger datasets over time.
Flexibility
Modular Design: Supports adding, removing, or modifying components without significant rework.
Community and Support
Documentation: Comprehensive and clear documentation to help you understand and use the framework effectively.
Community Engagement: An active community can provide support, plugins, and shared experiences that can be invaluable.
Ease of Use
Learning Curve: How quickly your team can get up to speed with the framework.
Implementation Simplicity: How easy it is to implement and start building with the framework.
Importance of Simulation Environments for Multi-Agent Systems
Simulation environments play a vital role in the development and testing of multi-agent systems. They provide a controlled setting where agents can interact, perform tasks, and adapt to various scenarios, ensuring robust performance before deployment.
Controlled Testing
Allows for thorough testing of agent interactions and behaviors in a risk-free environment.
Identifies potential issues and bugs in the early stages of development.
Performance Optimization
Helps in identifying bottlenecks and optimizing agent performance.
Provides insights into how agents can be improved and fine-tuned for better results.
Scenario Analysis
Enables the simulation of different scenarios to assess agent responses and adapt strategies accordingly.
Tests the robustness of agents under various conditions and stresses.
With these guidelines, you can make informed decisions when selecting the right agent framework for your project, ensuring that it meets your specific needs and delivers optimal performance.
Now, let's delve deeper into the building blocks of an LLM agent framework application.
LLM Agent Frameworks: Building Blocks
Creating a practical LLM agent framework requires assembling several vital components that work together seamlessly. These components form the backbone of any robust LLM agent, ensuring it can handle complex tasks and provide accurate responses.
This section will explore the essential tools and modules needed to develop a QA agent, discuss the significance of the Retrieval-augmented generation (RAG) pipeline, and delve into the development of mathematical tools, question decomposition modules, and memory modules.
Tools Required for Developing a QA Agent
Developing a QA agent necessitates a suite of tools that enhance its ability to accurately understand and respond to queries. These tools are integral to building a responsive and efficient agent.
Natural Language Processing (NLP) Library
Tools like SpaCy or NLTK for text processing and understanding.
Pre-trained models for language comprehension.
Machine Learning Framework
Libraries such as TensorFlow or PyTorch for model development and training.
Pre-trained models like BERT or GPT for question answering.
Data Retrieval Tools
Systems like Elasticsearch for indexing and retrieving relevant documents.
APIs to fetch data from external sources.
Retrieval-Augmented Generation (RAG) Pipeline & Its Significance
RAG pipeline is a critical component for enhancing the accuracy and relevance of responses in LLM agents. It combines the strengths of retrieval-based and generation-based methods to provide precise answers.
Components of RAG
Retriever: Fetches relevant documents or passages from a large corpus.
Generator: Uses the retrieved documents to generate detailed and accurate answers.
from transformers import RagTokenizer, RagRetriever, RagSequenceForGeneration
# Initialize tokenizer, retriever, and generator
tokenizer = RagTokenizer.from_pretrained("facebook/rag-sequence-nq")
retriever = RagRetriever.from_pretrained("facebook/rag-sequence-nq", index_name="exact")
rag_sequence = RagSequenceForGeneration.from_pretrained("facebook/rag-sequence-nq", retriever=retriever)
input_ids = tokenizer("What is the capital of France?", return_tensors="pt")["input_ids"]
generated = rag_sequence.generate(input_ids)
print(tokenizer.batch_decode(generated, skip_special_tokens=True))
Significance of RAG
Improved Accuracy: Enhances the precision of responses by leveraging vast amounts of data.
Development of a Mathematical Tool for Planning
A mathematical tool is essential for performing calculations and planning tasks within the agent framework. This tool helps the agent handle numerical data and perform necessary computations.
Capabilities
Basic Calculations: Addition, subtraction, multiplication, and division.
Advanced Functions: Algebra, calculus, and statistical analysis.
import sympy as sp
# Define symbols
x, y = sp.symbols('x y')
# Perform calculations
equation = sp.Eq(x + y, 10)
solution = sp.solve(equation, x)
print(solution)
Integration
Easily integrates with other modules to perform computations as required.
Provides accurate and reliable results for complex queries.
Construction of a Question Decomposition Module
A question decomposition module is vital for breaking down complex questions into simpler, manageable parts. This ensures that the agent can tackle intricate queries methodically.
Functionality
Question Parsing: Identifies and separates different parts of a complex question.
Task Delegation: Assigns sub-tasks to appropriate modules for processing.
def decompose_question(question):
# Example decomposition logic
if "and" in question:
parts = question.split("and")
else:
parts = [question]
return parts
# Decompose a complex question
question = "What is the capital of France and who is the president?"
sub_questions = decompose_question(question)
print(sub_questions)
With these building blocks in place, you can develop a robust and efficient LLM agent framework application capable of handling complex tasks and providing accurate answers. Next, we will explore how to develop the core of your LLM agent, integrating these components into a cohesive system.
Developing the Core of Your LLM Agent
The core of your LLM agent is the central processing unit that orchestrates all its operations. This section covers the different types of solvers used in the agent core, the integration process for various components such as tools, planners, and memory modules, and the evaluation of the mental model to ensure the agent's effectiveness.
Developing a robust core is crucial for the agent's ability to handle complex tasks efficiently and accurately.
Introduction to Different Solver Types for the Agent Core
Choosing the right solver type for your agent core is essential for optimizing performance and task management. Different solvers offer unique benefits and are suited to various levels of complexity in problem-solving.
Linear Solver
Simple Execution: Follows a straightforward, linear path to solve tasks.
Ease of Implementation: Suitable for simpler tasks that do not require complex decision-making processes.
def linear_solver(tasks):
results = []
for task in tasks:
result = perform_task(task)
results.append(result)
return results
def perform_task(task):
# Define task execution logic here
return f"Result of {task}"
tasks = ["task1", "task2", "task3"]
print(linear_solver(tasks))
Single-Thread Recursive Solver
Depth-First Approach: Solves tasks by recursively breaking them into sub-tasks and processing them individually.
Improved Problem-Solving: Suitable for tasks that require a more detailed and step-by-step approach.
def single_thread_recursive_solver(task):
if is_complex_task(task):
sub_tasks = decompose_task(task)
results = [single_thread_recursive_solver(sub_task) for sub_task in sub_tasks]
return combine_results(results)
else:
return perform_task(task)
def is_complex_task(task):
# Define logic to determine if a task is complex
return "complex" in task
def decompose_task(task):
# Define logic to decompose task into sub-tasks
return [f"{task}_part1", f"{task}_part2"]
def combine_results(results):
# Define logic to combine results of sub-tasks
return " & ".join(results)
def perform_task(task):
# Define task execution logic here
return f"Result of {task}"
task = "complex_task"
print(single_thread_recursive_solver(task)
Multi-Thread Recursive Solver
Parallel Processing: Executes multiple tasks or sub-tasks simultaneously, improving efficiency and reducing latency.
High Complexity Handling: Ideal for handling highly complex tasks that benefit from concurrent execution.
import concurrent.futures
def multi_thread_recursive_solver(task):
if is_complex_task(task):
sub_tasks = decompose_task(task)
with concurrent.futures.ThreadPoolExecutor() as executor:
results = list(executor.map(multi_thread_recursive_solver, sub_tasks))
return combine_results(results)
else:
return perform_task(task)
# Reuse the previously defined functions is_complex_task, decompose_task, combine_results, and perform_task
task = "complex_task"
print(multi_thread_recursive_solver(task))
Integration Process of Tools, Planner, and Memory Module in the Agent Setup
Integrating the various components of your LLM agent—such as tools, the planner, and the memory module—is critical to ensure they work together seamlessly and efficiently. This process involves setting up communication channels and workflows between these components.
Tools Integration
Setup and Configuration: Initialize and configure the tools required for the agent to perform specific tasks.
Inter-Component Communication: Ensure that the tools can communicate effectively with other components like the planner and memory module.
class Agent:
def __init__(self, tools, planner, memory):
self.tools = tools
self.planner = planner
self.memory = memory
def integrate_tools(self):
for tool in self.tools:
tool.setup()
print("Tools integrated successfully")
class Tool:
def setup(self):
# Define tool setup logic here
print("Tool setup complete")
tools = [Tool(), Tool()]
agent = Agent(tools, None, None)
agent.integrate_tools()
Planner Integration
Task Decomposition: The planner decomposes complex tasks into manageable sub-tasks.
Task Assignment: Assigns sub-tasks to the appropriate tools and manages their execution.
class Planner:
def __init__(self):
self.plan = []
def create_plan(self, task):
self.plan = self.decompose_task(task)
def decompose_task(self, task):
# Decompose the task into sub-tasks
return [f"{task}_sub1", f"{task}_sub2"]
def assign_tasks(self, agent):
for sub_task in self.plan:
agent.execute_task(sub_task)
planner = Planner()
planner.create_plan("complex_task")
Memory Module Integration
Information Retrieval: Retrieves relevant information from past interactions to aid in current task processing.
class MemoryModule:
def __init__(self):
self.memory = []
def store_interaction(self, question, answer):
self.memory.append({"question": question, "answer": answer})
def retrieve_interaction(self, question):
for interaction in self.memory:
if interaction["question"] == question:
return interaction["answer"]
return None
memory = MemoryModule()
memory.store_interaction("What is the capital of France?", "Paris")
Full Integration Example
Combining All Components: Integrate tools, the planner, and the memory module into the agent setup.
class FullAgent:
def __init__(self, tools, planner, memory):
self.tools = tools
self.planner = planner
self.memory = memory
def execute_task(self, task):
if task in [t.get_task_name() for t in self.tools]:
tool = next(t for t in self.tools if t.get_task_name() == task)
return tool.perform_task()
else:
return "Task not supported"
tools = [Tool(), Tool()]
planner = Planner()
memory = MemoryModule()
agent = FullAgent(tools, planner, memory)
# Integrate tools, create plan, and execute tasks
agent.integrate_tools()
planner.create_plan("complex_task")
planner.assign_tasks(agent)
Evaluation of the Mental Model for Agent Effectiveness
Evaluating your LLM agent's mental model is crucial to ensuring its effectiveness and efficiency. This involves testing the agent's reasoning capabilities, ability to handle various tasks, and overall performance in real-world scenarios.
Reasoning Capabilities
Logic and Decision-Making: Assess how well the agent can make logical decisions based on available information.
Complex Problem Solving: Test the agent's ability to solve complex problems through reasoning.
Task Handling
Task Diversity: Evaluate the agent's ability to handle various tasks, from simple queries to complex multi-step processes.
Efficiency: Measure the speed and accuracy with which the agent completes tasks.
Performance Metrics
Accuracy: Determine the accuracy of the agent's responses and its success rate in task completion.
User Satisfaction: Gather user feedback to assess the effectiveness and usability of the agent.
class AgentEvaluator:
def __init__(self, agent):
self.agent = agent
def evaluate(self, tasks):
results = []
for task in tasks:
result = self.agent.execute_task(task)
results.append(result)
return results
def calculate_accuracy(self, results, expected_results):
correct = sum([1 for result, expected in zip(results, expected_results) if result == expected])
return correct / len(results)
# Example usage
evaluator = AgentEvaluator(agent)
tasks = ["simple_task", "complex_task_sub1", "complex_task_sub2"]
results = evaluator.evaluate(tasks)
expected_results = ["Result of simple_task", "Result of complex_task_sub1", "Result of complex_task_sub2"]
accuracy = evaluator.calculate_accuracy(results, expected_results)
print(f"Agent Accuracy: {accuracy * 100}%")
By developing a robust core and integrating various components effectively, your LLM agent can perform complex tasks with high efficiency and accuracy. Evaluating the agent's mental model ensures that it remains effective and reliable in real-world applications.
Recommended Reading and Resources
Exploring additional resources and reading materials is essential to further enhancing your understanding and capabilities in developing LLM agent frameworks.
These resources provide in-depth knowledge, case studies, and practical examples to help you build more sophisticated and effective LLM agents.
AutoGPT, Voyager, OlaGPT, MRKL Systems, Generative Agents: Interactive Simulacra of Human Behavior
Exploring the following projects and systems will give you a comprehensive view of the state-of-the-art LLM agent development and the potential for creating advanced AI applications.
AutoGPT
Overview: AutoGPT is an innovative project that automates the generation of GPT-based agents. It showcases the potential for automating complex tasks using language models.
Key Features: Automated task handling, minimal human intervention, and adaptive learning capabilities.
Useful Links: AutoGPT GitHub Repository
Voyager
Overview: Voyager is a project by NVIDIA Research that focuses on self-improving agents capable of learning new tools and building new capabilities without external intervention.
Key Features: Self-improvement, autonomous tool learning, and advanced problem-solving skills.
Useful Links: Voyager Project Page
OlaGPT
Overview: OlaGPT is a conceptual framework that stimulates ideas on how to extend the capabilities of basic agents. It focuses on enhancing agent interactions and performance.
Key Features: Conceptual extensions, interaction enhancements, and performance optimization.
Useful Links: OlaGPT Research Paper
MRKL Systems
Overview: MRKL Systems (Modular Reasoning, Knowledge, and Language) combine large language models with external knowledge sources and discrete reasoning for executing complex tasks.
Key Features: Integration of language models with knowledge bases, modular architecture, and enhanced reasoning capabilities.
Useful Links: MRKL Systems Paper
Generative Agents: Interactive Simulacra of Human Behavior
Overview: This project explores the creation of agents that simulate human behavior through decentralized interactions. It's one of the pioneering efforts in developing swarm intelligence.
Key Features: Decentralized agent interactions, human-like behavior simulation, and advanced AI techniques.
Useful Links: Generative Agents Research
LLM-Powered Agent List for Further Reading and Resources
The Awesome LLM-Powered Agent list is a curated collection of resources, tools, and examples that can provide further insights and practical knowledge for developing LLM agents. It is a valuable resource for both beginners and experienced developers.
Overview
The list includes various topics, from foundational theories to advanced implementations, and is regularly updated by the community.
Key Sections
Tutorials and Guides: Step-by-step tutorials and guides for building LLM agents.
Libraries and Frameworks: Information on various libraries and frameworks that support LLM agent development.
Case Studies: Real-world examples and case studies showcasing successful LLM agent implementations.
Research Papers: Access to significant research papers that provide theoretical and practical insights.
Useful Links
Excellent LLM-Powered Agent List: Awesome LLM-Powered Agent GitHub Repository
Additional Resources: NVIDIA AI Playground
By diving into these recommended readings and resources, you will gain a deeper understanding of the advanced concepts and practical applications of LLM agent frameworks. These materials will help you stay updated with the latest developments and enhance your skills in building sophisticated AI agents.
Summary
Building an LLM agent framework application involves understanding and integrating key components such as solvers, tools, planners, and memory modules. Each element is crucial in ensuring the agent's efficiency, accuracy, and contextual relevance. You can create robust and effective LLM agents by leveraging recommended frameworks and resources.
Looking ahead, the future of LLM agent applications is bright. They have the potential to revolutionize various industries by automating complex tasks and enhancing user interactions. The continuous advancements in AI and machine learning will drive further improvements in agent capabilities and applications. Embracing these innovations will allow businesses to unlock new levels of productivity and efficiency.
How Can RAGA AI Help You in Developing Your First LLM Agent Framework Application?
RAGA AI offers a comprehensive and user-friendly platform designed to simplify developing your first LLM agent framework application. With its robust set of tools and integrated modules, RAGA AI enables you to seamlessly build, test, and deploy advanced LLM agents without extensive technical expertise.
Additionally, RAGA AI's innovative features, such as automated issue detection, memory modules, and planning tools, ensure that your agents perform efficiently and accurately, help.
So Sign Up for RAGA's LLM Hub and get your LLM applications ready 3X faster!
Building your first LLM agent framework application can feel daunting, but it doesn’t have to be. Understanding the core elements—agent cores, memory modules, agent tools, and planning modules—is crucial for success.
In today’s rapidly evolving AI landscape, developing an LLM agent framework application can significantly enhance your AI capabilities. This blog will explore the available implementation frameworks, compare single-agent and multi-agent systems, and highlight popular options to help you make informed decisions. Let’s dive into the developer ecosystem for LLM agents.
LLM Agent Framework Application: Overview
In this section, we will break down the key concepts, starting with LLM agent frameworks, how they differ from traditional chatbots, and the essential components of developing a robust LLM agent application.
LLM Agent Frameworks
LLM agent frameworks are designed to enable large language models to perform complex tasks by integrating multiple functionalities and tools.
Unlike simple chatbot applications, these frameworks allow dynamic problem-solving and advanced data processing. Key aspects include:
Integration of Various Modules
Memory and Planning Modules: These frameworks integrate different components, such as memory modules that help the agent remember past interactions and planning modules that break down complex tasks into simpler, executable steps.
Functional Diversity: They support various functionalities, from data retrieval to executing computational tasks, ensuring a holistic approach to problem-solving.
Enhanced Flexibility
Dynamic Task Handling: Unlike rigid chatbots, LLM agent frameworks can adapt to various tasks and queries, making them suitable for diverse applications.
Learning and Adaptation: They continuously improve from interactions, adapting to new tasks and refining their responses.
Scalability
Single-Agent to Multi-Agent Systems: These frameworks can be scaled from single-agent systems that handle specific tasks to multi-agent systems that manage more complex, interrelated activities.
Resource Management: Efficiently manage computational resources to maintain performance even as the complexity of tasks increases.
LLM Agent Framework Applications vs. Conventional Chatbot Applications
While both LLM agent frameworks and traditional chatbots are used to interact with users through natural language, there are significant differences:
Complexity
LLM Agent Frameworks: Capable of handling multifaceted tasks involving advanced reasoning and decision-making processes. They are designed to go beyond primary query responses to solve complex problems.
Conventional Chatbots: Typically follow predefined scripts and respond to straightforward queries with limited capacity for complex problem-solving.
Adaptability
LLM Agent Frameworks: Continuously learn from each interaction, allowing for improvement and adaptation to new and varied tasks. They can handle unexpected queries and adapt their strategies accordingly.
Conventional Chatbots: Restricted to their initial programming with minimal learning capabilities. They struggle with queries outside their predefined scope.
LLM Agent Applications: Overview of Essential Components Required
Creating a functional LLM agent framework involves integrating several critical components that work together seamlessly:
Agent Core
Central Processing Unit: This unit manages all tasks, decisions, and actions within the framework. It is the brain of the LLM agent, orchestrating how other components interact.
Task Management: Ensures tasks are executed correctly and coordinates between different modules and tools.
Agent Tools
Specialized Functionalities: Include tools for data retrieval, computational analysis, and external API integrations. These tools extend the agent’s capabilities to perform specific tasks efficiently.
Tool Integration: Seamlessly integrates with external tools and services to enhance the agent’s functionality and provide more comprehensive solutions.
Planning Module
Task Decomposition: Breaks down complex queries into manageable sub-tasks, ensuring logical and efficient execution.
Execution Strategy: Develops a plan for tackling each subtask and integrate results to provide coherent answers to complex queries.
By understanding these components, you can build a more efficient and capable LLM agent framework application, setting the stage for advanced AI solutions. This foundational knowledge will help you navigate the complexities of AI development, leading to the successful implementation and deployment of LLM agents in your projects.
With a solid grasp of the fundamental concepts and components of LLM agent framework applications, it's time to explore the tools and frameworks available for developers. Let's dive into the developer ecosystem for LLM agents.
LLM Agent Framework: Developing Ecosystem
The development of the LLM agent framework is supported by a robust ecosystem of frameworks and tools, each offering unique features and capabilities. Understanding these options is essential for selecting the proper framework for your project.
This section will introduce you to the available implementation frameworks, compare single-agent and multi-agent systems, highlight popular frameworks, and discuss community-built solutions.
Developing LLM Agent Framework: Introduction to Available Implementation Frameworks
Several frameworks are available for developing LLM agents, each designed to streamline the development process and enhance functionality. These frameworks provide the building blocks needed to create sophisticated AI agents capable of handling complex tasks.
LangChain: A versatile framework that supports the creation of modular agents with various tools and integrations.
LLaMaIndex: Focuses on indexing and retrieving data efficiently, making it ideal for data-heavy applications.
HayStack: A robust framework designed for building search and question-answering systems.
Example code to initialize a LangChain agent:
from langchain.agents import Agent
# Initialize LangChain agent
agent = Agent()
agent.initialize()
Comparison of Single-Agent vs. Multi-Agent Frameworks
Choosing between single-agent and multi-agent frameworks depends on your application's complexity and requirements. Each type offers distinct advantages and is suited to different use cases.
Single-Agent Frameworks
These frameworks focus on a single AI agent that handles specific tasks independently, offering simplicity and ease of implementation.
Simplicity: Easier to implement and manage.
Focused Tasks: Ideal for applications with specific, isolated tasks.
Example Framework: LangChain.
Example code to create a single-agent system:
from langchain.agents import SingleAgent
# Create a single-agent instance
agent = SingleAgent()
agent.run()
Multi-Agent Frameworks
These frameworks involve multiple AI agents working together, enabling complex interactions and scalability for more intricate applications.
Complex Interactions: Capable of handling multiple, interconnected tasks.
Scalability: Suitable for large-scale applications requiring coordination between agents.
Example Framework: AgentVerse.
Example code to create a multi-agent system:
from agentverse import MultiAgentSystem
# Create a multi-agent system
system = MultiAgentSystem()
system.initialize_agents(num_agents=5)
system.run()
Highlighting Popular Frameworks
Several frameworks have gained popularity due to their robust features and community support. Here are some of the top choices for developing LLM agents:
LangChain Agents
Modular Design: Easily integrates with various tools and services.
Strong Community: Extensive documentation and community support.
from langchain.agents import LangChainAgent
# Initialize LangChain agent with specific tools
agent = LangChainAgent(tools=['tool1', 'tool2'])
agent.run()
LLaMaIndex Agents
Efficient Data Handling: Optimized for indexing and data retrieval.
Scalable: Handles large datasets effectively.
from llamaindex import LLaMaAgent
# Initialize LLaMa agent for data indexing
agent = LLaMaAgent(index_path='path/to/index')
agent.index_data(data_source='data/source/path')
HayStack Agents
Search and QA: Built for creating powerful search engines and question-answering systems.
Flexibility: Supports a variety of backends and data sources.
from haystack.agents import HayStackAgent
# Initialize HayStack agent for QA
agent = HayStackAgent()
agent.build_qa_pipeline(model_name='bert-base-uncased')
AutoGen
Automated Code Generation: Facilitates the automatic generation of code based on user input.
Ease of Use: Simplifies the development process with minimal manual coding.
from autogen import AutoGenAgent
# Initialize AutoGen agent
agent = AutoGenAgent()
agent.generate_code(prompt='Create a function to add two numbers')
AgentVerse
Multi-Agent Systems: Designed for creating and managing complex multi-agent interactions.
Simulation Environment: Provides tools for simulating agent interactions.
from agentverse import AgentVerseSystem
# Initialize AgentVerse system
system = AgentVerseSystem()
system.add_agents(['agent1', 'agent2'])
system.simulate()
ChatDev
Chatbot Development: Specialized in creating advanced chatbot applications.
Customization: Highly customizable to suit specific business needs.
from chatdev import ChatBot
# Initialize ChatDev chatbot
chatbot = ChatBot()
chatbot.train(training_data='path/to/training/data')
Generative Agents
Simulated Human Behavior: Creates agents that mimic human interactions.
Advanced AI: Uses cutting-edge AI techniques for more realistic simulations.
from generativeagents import SimulacraAgent
# Initialize Generative Agent
agent = SimulacraAgent()
agent.simulate_behavior(behavior_model='human-like')
Explanation of Community-Built Frameworks, Including Usage and Features
Community-built frameworks often provide innovative solutions and additional features, contributed by developers worldwide. These frameworks can be invaluable for specific needs and offer unique advantages.
Customization: Tailored to niche use cases and specific project requirements.
Open Source: Freely available and often supported by active developer communities.
Innovative Features: Community contributions often lead to rapidly developing new and valuable features.
With these tools and frameworks, you can develop powerful LLM agent applications. Next, let's explore how to choose the right agent framework for your specific needs.
LLM Agent Framework App Build: Choosing the Right Agent Framework
Selecting the right agent framework is crucial in building practical LLM agent applications. The choice depends on various factors, including the complexity of tasks, scalability requirements, and the specific features needed for the LLM agent framework application build.
This section will guide you through the evaluation criteria for selecting an agent framework, highlight the differences between single-agent and multi-agent frameworks, and discuss the importance of simulation environments for multi-agent systems.
Evaluation Criteria for Selecting an Agent Framework
When choosing an agent framework, it’s essential to consider several key criteria to ensure that the framework aligns with your project requirements and goals.
Scalability
Handling Load: The framework should be capable of handling the maximum load you expect, whether it’s the number of requests per second or the amount of data processed.
Growth Potential: It should be able to grow with your project, accommodating more complex tasks and larger datasets over time.
Flexibility
Modular Design: Supports adding, removing, or modifying components without significant rework.
Community and Support
Documentation: Comprehensive and clear documentation to help you understand and use the framework effectively.
Community Engagement: An active community can provide support, plugins, and shared experiences that can be invaluable.
Ease of Use
Learning Curve: How quickly your team can get up to speed with the framework.
Implementation Simplicity: How easy it is to implement and start building with the framework.
Importance of Simulation Environments for Multi-Agent Systems
Simulation environments play a vital role in the development and testing of multi-agent systems. They provide a controlled setting where agents can interact, perform tasks, and adapt to various scenarios, ensuring robust performance before deployment.
Controlled Testing
Allows for thorough testing of agent interactions and behaviors in a risk-free environment.
Identifies potential issues and bugs in the early stages of development.
Performance Optimization
Helps in identifying bottlenecks and optimizing agent performance.
Provides insights into how agents can be improved and fine-tuned for better results.
Scenario Analysis
Enables the simulation of different scenarios to assess agent responses and adapt strategies accordingly.
Tests the robustness of agents under various conditions and stresses.
With these guidelines, you can make informed decisions when selecting the right agent framework for your project, ensuring that it meets your specific needs and delivers optimal performance.
Now, let's delve deeper into the building blocks of an LLM agent framework application.
LLM Agent Frameworks: Building Blocks
Creating a practical LLM agent framework requires assembling several vital components that work together seamlessly. These components form the backbone of any robust LLM agent, ensuring it can handle complex tasks and provide accurate responses.
This section will explore the essential tools and modules needed to develop a QA agent, discuss the significance of the Retrieval-augmented generation (RAG) pipeline, and delve into the development of mathematical tools, question decomposition modules, and memory modules.
Tools Required for Developing a QA Agent
Developing a QA agent necessitates a suite of tools that enhance its ability to accurately understand and respond to queries. These tools are integral to building a responsive and efficient agent.
Natural Language Processing (NLP) Library
Tools like SpaCy or NLTK for text processing and understanding.
Pre-trained models for language comprehension.
Machine Learning Framework
Libraries such as TensorFlow or PyTorch for model development and training.
Pre-trained models like BERT or GPT for question answering.
Data Retrieval Tools
Systems like Elasticsearch for indexing and retrieving relevant documents.
APIs to fetch data from external sources.
Retrieval-Augmented Generation (RAG) Pipeline & Its Significance
RAG pipeline is a critical component for enhancing the accuracy and relevance of responses in LLM agents. It combines the strengths of retrieval-based and generation-based methods to provide precise answers.
Components of RAG
Retriever: Fetches relevant documents or passages from a large corpus.
Generator: Uses the retrieved documents to generate detailed and accurate answers.
from transformers import RagTokenizer, RagRetriever, RagSequenceForGeneration
# Initialize tokenizer, retriever, and generator
tokenizer = RagTokenizer.from_pretrained("facebook/rag-sequence-nq")
retriever = RagRetriever.from_pretrained("facebook/rag-sequence-nq", index_name="exact")
rag_sequence = RagSequenceForGeneration.from_pretrained("facebook/rag-sequence-nq", retriever=retriever)
input_ids = tokenizer("What is the capital of France?", return_tensors="pt")["input_ids"]
generated = rag_sequence.generate(input_ids)
print(tokenizer.batch_decode(generated, skip_special_tokens=True))
Significance of RAG
Improved Accuracy: Enhances the precision of responses by leveraging vast amounts of data.
Development of a Mathematical Tool for Planning
A mathematical tool is essential for performing calculations and planning tasks within the agent framework. This tool helps the agent handle numerical data and perform necessary computations.
Capabilities
Basic Calculations: Addition, subtraction, multiplication, and division.
Advanced Functions: Algebra, calculus, and statistical analysis.
import sympy as sp
# Define symbols
x, y = sp.symbols('x y')
# Perform calculations
equation = sp.Eq(x + y, 10)
solution = sp.solve(equation, x)
print(solution)
Integration
Easily integrates with other modules to perform computations as required.
Provides accurate and reliable results for complex queries.
Construction of a Question Decomposition Module
A question decomposition module is vital for breaking down complex questions into simpler, manageable parts. This ensures that the agent can tackle intricate queries methodically.
Functionality
Question Parsing: Identifies and separates different parts of a complex question.
Task Delegation: Assigns sub-tasks to appropriate modules for processing.
def decompose_question(question):
# Example decomposition logic
if "and" in question:
parts = question.split("and")
else:
parts = [question]
return parts
# Decompose a complex question
question = "What is the capital of France and who is the president?"
sub_questions = decompose_question(question)
print(sub_questions)
With these building blocks in place, you can develop a robust and efficient LLM agent framework application capable of handling complex tasks and providing accurate answers. Next, we will explore how to develop the core of your LLM agent, integrating these components into a cohesive system.
Developing the Core of Your LLM Agent
The core of your LLM agent is the central processing unit that orchestrates all its operations. This section covers the different types of solvers used in the agent core, the integration process for various components such as tools, planners, and memory modules, and the evaluation of the mental model to ensure the agent's effectiveness.
Developing a robust core is crucial for the agent's ability to handle complex tasks efficiently and accurately.
Introduction to Different Solver Types for the Agent Core
Choosing the right solver type for your agent core is essential for optimizing performance and task management. Different solvers offer unique benefits and are suited to various levels of complexity in problem-solving.
Linear Solver
Simple Execution: Follows a straightforward, linear path to solve tasks.
Ease of Implementation: Suitable for simpler tasks that do not require complex decision-making processes.
def linear_solver(tasks):
results = []
for task in tasks:
result = perform_task(task)
results.append(result)
return results
def perform_task(task):
# Define task execution logic here
return f"Result of {task}"
tasks = ["task1", "task2", "task3"]
print(linear_solver(tasks))
Single-Thread Recursive Solver
Depth-First Approach: Solves tasks by recursively breaking them into sub-tasks and processing them individually.
Improved Problem-Solving: Suitable for tasks that require a more detailed and step-by-step approach.
def single_thread_recursive_solver(task):
if is_complex_task(task):
sub_tasks = decompose_task(task)
results = [single_thread_recursive_solver(sub_task) for sub_task in sub_tasks]
return combine_results(results)
else:
return perform_task(task)
def is_complex_task(task):
# Define logic to determine if a task is complex
return "complex" in task
def decompose_task(task):
# Define logic to decompose task into sub-tasks
return [f"{task}_part1", f"{task}_part2"]
def combine_results(results):
# Define logic to combine results of sub-tasks
return " & ".join(results)
def perform_task(task):
# Define task execution logic here
return f"Result of {task}"
task = "complex_task"
print(single_thread_recursive_solver(task)
Multi-Thread Recursive Solver
Parallel Processing: Executes multiple tasks or sub-tasks simultaneously, improving efficiency and reducing latency.
High Complexity Handling: Ideal for handling highly complex tasks that benefit from concurrent execution.
import concurrent.futures
def multi_thread_recursive_solver(task):
if is_complex_task(task):
sub_tasks = decompose_task(task)
with concurrent.futures.ThreadPoolExecutor() as executor:
results = list(executor.map(multi_thread_recursive_solver, sub_tasks))
return combine_results(results)
else:
return perform_task(task)
# Reuse the previously defined functions is_complex_task, decompose_task, combine_results, and perform_task
task = "complex_task"
print(multi_thread_recursive_solver(task))
Integration Process of Tools, Planner, and Memory Module in the Agent Setup
Integrating the various components of your LLM agent—such as tools, the planner, and the memory module—is critical to ensure they work together seamlessly and efficiently. This process involves setting up communication channels and workflows between these components.
Tools Integration
Setup and Configuration: Initialize and configure the tools required for the agent to perform specific tasks.
Inter-Component Communication: Ensure that the tools can communicate effectively with other components like the planner and memory module.
class Agent:
def __init__(self, tools, planner, memory):
self.tools = tools
self.planner = planner
self.memory = memory
def integrate_tools(self):
for tool in self.tools:
tool.setup()
print("Tools integrated successfully")
class Tool:
def setup(self):
# Define tool setup logic here
print("Tool setup complete")
tools = [Tool(), Tool()]
agent = Agent(tools, None, None)
agent.integrate_tools()
Planner Integration
Task Decomposition: The planner decomposes complex tasks into manageable sub-tasks.
Task Assignment: Assigns sub-tasks to the appropriate tools and manages their execution.
class Planner:
def __init__(self):
self.plan = []
def create_plan(self, task):
self.plan = self.decompose_task(task)
def decompose_task(self, task):
# Decompose the task into sub-tasks
return [f"{task}_sub1", f"{task}_sub2"]
def assign_tasks(self, agent):
for sub_task in self.plan:
agent.execute_task(sub_task)
planner = Planner()
planner.create_plan("complex_task")
Memory Module Integration
Information Retrieval: Retrieves relevant information from past interactions to aid in current task processing.
class MemoryModule:
def __init__(self):
self.memory = []
def store_interaction(self, question, answer):
self.memory.append({"question": question, "answer": answer})
def retrieve_interaction(self, question):
for interaction in self.memory:
if interaction["question"] == question:
return interaction["answer"]
return None
memory = MemoryModule()
memory.store_interaction("What is the capital of France?", "Paris")
Full Integration Example
Combining All Components: Integrate tools, the planner, and the memory module into the agent setup.
class FullAgent:
def __init__(self, tools, planner, memory):
self.tools = tools
self.planner = planner
self.memory = memory
def execute_task(self, task):
if task in [t.get_task_name() for t in self.tools]:
tool = next(t for t in self.tools if t.get_task_name() == task)
return tool.perform_task()
else:
return "Task not supported"
tools = [Tool(), Tool()]
planner = Planner()
memory = MemoryModule()
agent = FullAgent(tools, planner, memory)
# Integrate tools, create plan, and execute tasks
agent.integrate_tools()
planner.create_plan("complex_task")
planner.assign_tasks(agent)
Evaluation of the Mental Model for Agent Effectiveness
Evaluating your LLM agent's mental model is crucial to ensuring its effectiveness and efficiency. This involves testing the agent's reasoning capabilities, ability to handle various tasks, and overall performance in real-world scenarios.
Reasoning Capabilities
Logic and Decision-Making: Assess how well the agent can make logical decisions based on available information.
Complex Problem Solving: Test the agent's ability to solve complex problems through reasoning.
Task Handling
Task Diversity: Evaluate the agent's ability to handle various tasks, from simple queries to complex multi-step processes.
Efficiency: Measure the speed and accuracy with which the agent completes tasks.
Performance Metrics
Accuracy: Determine the accuracy of the agent's responses and its success rate in task completion.
User Satisfaction: Gather user feedback to assess the effectiveness and usability of the agent.
class AgentEvaluator:
def __init__(self, agent):
self.agent = agent
def evaluate(self, tasks):
results = []
for task in tasks:
result = self.agent.execute_task(task)
results.append(result)
return results
def calculate_accuracy(self, results, expected_results):
correct = sum([1 for result, expected in zip(results, expected_results) if result == expected])
return correct / len(results)
# Example usage
evaluator = AgentEvaluator(agent)
tasks = ["simple_task", "complex_task_sub1", "complex_task_sub2"]
results = evaluator.evaluate(tasks)
expected_results = ["Result of simple_task", "Result of complex_task_sub1", "Result of complex_task_sub2"]
accuracy = evaluator.calculate_accuracy(results, expected_results)
print(f"Agent Accuracy: {accuracy * 100}%")
By developing a robust core and integrating various components effectively, your LLM agent can perform complex tasks with high efficiency and accuracy. Evaluating the agent's mental model ensures that it remains effective and reliable in real-world applications.
Recommended Reading and Resources
Exploring additional resources and reading materials is essential to further enhancing your understanding and capabilities in developing LLM agent frameworks.
These resources provide in-depth knowledge, case studies, and practical examples to help you build more sophisticated and effective LLM agents.
AutoGPT, Voyager, OlaGPT, MRKL Systems, Generative Agents: Interactive Simulacra of Human Behavior
Exploring the following projects and systems will give you a comprehensive view of the state-of-the-art LLM agent development and the potential for creating advanced AI applications.
AutoGPT
Overview: AutoGPT is an innovative project that automates the generation of GPT-based agents. It showcases the potential for automating complex tasks using language models.
Key Features: Automated task handling, minimal human intervention, and adaptive learning capabilities.
Useful Links: AutoGPT GitHub Repository
Voyager
Overview: Voyager is a project by NVIDIA Research that focuses on self-improving agents capable of learning new tools and building new capabilities without external intervention.
Key Features: Self-improvement, autonomous tool learning, and advanced problem-solving skills.
Useful Links: Voyager Project Page
OlaGPT
Overview: OlaGPT is a conceptual framework that stimulates ideas on how to extend the capabilities of basic agents. It focuses on enhancing agent interactions and performance.
Key Features: Conceptual extensions, interaction enhancements, and performance optimization.
Useful Links: OlaGPT Research Paper
MRKL Systems
Overview: MRKL Systems (Modular Reasoning, Knowledge, and Language) combine large language models with external knowledge sources and discrete reasoning for executing complex tasks.
Key Features: Integration of language models with knowledge bases, modular architecture, and enhanced reasoning capabilities.
Useful Links: MRKL Systems Paper
Generative Agents: Interactive Simulacra of Human Behavior
Overview: This project explores the creation of agents that simulate human behavior through decentralized interactions. It's one of the pioneering efforts in developing swarm intelligence.
Key Features: Decentralized agent interactions, human-like behavior simulation, and advanced AI techniques.
Useful Links: Generative Agents Research
LLM-Powered Agent List for Further Reading and Resources
The Awesome LLM-Powered Agent list is a curated collection of resources, tools, and examples that can provide further insights and practical knowledge for developing LLM agents. It is a valuable resource for both beginners and experienced developers.
Overview
The list includes various topics, from foundational theories to advanced implementations, and is regularly updated by the community.
Key Sections
Tutorials and Guides: Step-by-step tutorials and guides for building LLM agents.
Libraries and Frameworks: Information on various libraries and frameworks that support LLM agent development.
Case Studies: Real-world examples and case studies showcasing successful LLM agent implementations.
Research Papers: Access to significant research papers that provide theoretical and practical insights.
Useful Links
Excellent LLM-Powered Agent List: Awesome LLM-Powered Agent GitHub Repository
Additional Resources: NVIDIA AI Playground
By diving into these recommended readings and resources, you will gain a deeper understanding of the advanced concepts and practical applications of LLM agent frameworks. These materials will help you stay updated with the latest developments and enhance your skills in building sophisticated AI agents.
Summary
Building an LLM agent framework application involves understanding and integrating key components such as solvers, tools, planners, and memory modules. Each element is crucial in ensuring the agent's efficiency, accuracy, and contextual relevance. You can create robust and effective LLM agents by leveraging recommended frameworks and resources.
Looking ahead, the future of LLM agent applications is bright. They have the potential to revolutionize various industries by automating complex tasks and enhancing user interactions. The continuous advancements in AI and machine learning will drive further improvements in agent capabilities and applications. Embracing these innovations will allow businesses to unlock new levels of productivity and efficiency.
How Can RAGA AI Help You in Developing Your First LLM Agent Framework Application?
RAGA AI offers a comprehensive and user-friendly platform designed to simplify developing your first LLM agent framework application. With its robust set of tools and integrated modules, RAGA AI enables you to seamlessly build, test, and deploy advanced LLM agents without extensive technical expertise.
Additionally, RAGA AI's innovative features, such as automated issue detection, memory modules, and planning tools, ensure that your agents perform efficiently and accurately, help.
So Sign Up for RAGA's LLM Hub and get your LLM applications ready 3X faster!
Building your first LLM agent framework application can feel daunting, but it doesn’t have to be. Understanding the core elements—agent cores, memory modules, agent tools, and planning modules—is crucial for success.
In today’s rapidly evolving AI landscape, developing an LLM agent framework application can significantly enhance your AI capabilities. This blog will explore the available implementation frameworks, compare single-agent and multi-agent systems, and highlight popular options to help you make informed decisions. Let’s dive into the developer ecosystem for LLM agents.
LLM Agent Framework Application: Overview
In this section, we will break down the key concepts, starting with LLM agent frameworks, how they differ from traditional chatbots, and the essential components of developing a robust LLM agent application.
LLM Agent Frameworks
LLM agent frameworks are designed to enable large language models to perform complex tasks by integrating multiple functionalities and tools.
Unlike simple chatbot applications, these frameworks allow dynamic problem-solving and advanced data processing. Key aspects include:
Integration of Various Modules
Memory and Planning Modules: These frameworks integrate different components, such as memory modules that help the agent remember past interactions and planning modules that break down complex tasks into simpler, executable steps.
Functional Diversity: They support various functionalities, from data retrieval to executing computational tasks, ensuring a holistic approach to problem-solving.
Enhanced Flexibility
Dynamic Task Handling: Unlike rigid chatbots, LLM agent frameworks can adapt to various tasks and queries, making them suitable for diverse applications.
Learning and Adaptation: They continuously improve from interactions, adapting to new tasks and refining their responses.
Scalability
Single-Agent to Multi-Agent Systems: These frameworks can be scaled from single-agent systems that handle specific tasks to multi-agent systems that manage more complex, interrelated activities.
Resource Management: Efficiently manage computational resources to maintain performance even as the complexity of tasks increases.
LLM Agent Framework Applications vs. Conventional Chatbot Applications
While both LLM agent frameworks and traditional chatbots are used to interact with users through natural language, there are significant differences:
Complexity
LLM Agent Frameworks: Capable of handling multifaceted tasks involving advanced reasoning and decision-making processes. They are designed to go beyond primary query responses to solve complex problems.
Conventional Chatbots: Typically follow predefined scripts and respond to straightforward queries with limited capacity for complex problem-solving.
Adaptability
LLM Agent Frameworks: Continuously learn from each interaction, allowing for improvement and adaptation to new and varied tasks. They can handle unexpected queries and adapt their strategies accordingly.
Conventional Chatbots: Restricted to their initial programming with minimal learning capabilities. They struggle with queries outside their predefined scope.
LLM Agent Applications: Overview of Essential Components Required
Creating a functional LLM agent framework involves integrating several critical components that work together seamlessly:
Agent Core
Central Processing Unit: This unit manages all tasks, decisions, and actions within the framework. It is the brain of the LLM agent, orchestrating how other components interact.
Task Management: Ensures tasks are executed correctly and coordinates between different modules and tools.
Agent Tools
Specialized Functionalities: Include tools for data retrieval, computational analysis, and external API integrations. These tools extend the agent’s capabilities to perform specific tasks efficiently.
Tool Integration: Seamlessly integrates with external tools and services to enhance the agent’s functionality and provide more comprehensive solutions.
Planning Module
Task Decomposition: Breaks down complex queries into manageable sub-tasks, ensuring logical and efficient execution.
Execution Strategy: Develops a plan for tackling each subtask and integrate results to provide coherent answers to complex queries.
By understanding these components, you can build a more efficient and capable LLM agent framework application, setting the stage for advanced AI solutions. This foundational knowledge will help you navigate the complexities of AI development, leading to the successful implementation and deployment of LLM agents in your projects.
With a solid grasp of the fundamental concepts and components of LLM agent framework applications, it's time to explore the tools and frameworks available for developers. Let's dive into the developer ecosystem for LLM agents.
LLM Agent Framework: Developing Ecosystem
The development of the LLM agent framework is supported by a robust ecosystem of frameworks and tools, each offering unique features and capabilities. Understanding these options is essential for selecting the proper framework for your project.
This section will introduce you to the available implementation frameworks, compare single-agent and multi-agent systems, highlight popular frameworks, and discuss community-built solutions.
Developing LLM Agent Framework: Introduction to Available Implementation Frameworks
Several frameworks are available for developing LLM agents, each designed to streamline the development process and enhance functionality. These frameworks provide the building blocks needed to create sophisticated AI agents capable of handling complex tasks.
LangChain: A versatile framework that supports the creation of modular agents with various tools and integrations.
LLaMaIndex: Focuses on indexing and retrieving data efficiently, making it ideal for data-heavy applications.
HayStack: A robust framework designed for building search and question-answering systems.
Example code to initialize a LangChain agent:
from langchain.agents import Agent
# Initialize LangChain agent
agent = Agent()
agent.initialize()
Comparison of Single-Agent vs. Multi-Agent Frameworks
Choosing between single-agent and multi-agent frameworks depends on your application's complexity and requirements. Each type offers distinct advantages and is suited to different use cases.
Single-Agent Frameworks
These frameworks focus on a single AI agent that handles specific tasks independently, offering simplicity and ease of implementation.
Simplicity: Easier to implement and manage.
Focused Tasks: Ideal for applications with specific, isolated tasks.
Example Framework: LangChain.
Example code to create a single-agent system:
from langchain.agents import SingleAgent
# Create a single-agent instance
agent = SingleAgent()
agent.run()
Multi-Agent Frameworks
These frameworks involve multiple AI agents working together, enabling complex interactions and scalability for more intricate applications.
Complex Interactions: Capable of handling multiple, interconnected tasks.
Scalability: Suitable for large-scale applications requiring coordination between agents.
Example Framework: AgentVerse.
Example code to create a multi-agent system:
from agentverse import MultiAgentSystem
# Create a multi-agent system
system = MultiAgentSystem()
system.initialize_agents(num_agents=5)
system.run()
Highlighting Popular Frameworks
Several frameworks have gained popularity due to their robust features and community support. Here are some of the top choices for developing LLM agents:
LangChain Agents
Modular Design: Easily integrates with various tools and services.
Strong Community: Extensive documentation and community support.
from langchain.agents import LangChainAgent
# Initialize LangChain agent with specific tools
agent = LangChainAgent(tools=['tool1', 'tool2'])
agent.run()
LLaMaIndex Agents
Efficient Data Handling: Optimized for indexing and data retrieval.
Scalable: Handles large datasets effectively.
from llamaindex import LLaMaAgent
# Initialize LLaMa agent for data indexing
agent = LLaMaAgent(index_path='path/to/index')
agent.index_data(data_source='data/source/path')
HayStack Agents
Search and QA: Built for creating powerful search engines and question-answering systems.
Flexibility: Supports a variety of backends and data sources.
from haystack.agents import HayStackAgent
# Initialize HayStack agent for QA
agent = HayStackAgent()
agent.build_qa_pipeline(model_name='bert-base-uncased')
AutoGen
Automated Code Generation: Facilitates the automatic generation of code based on user input.
Ease of Use: Simplifies the development process with minimal manual coding.
from autogen import AutoGenAgent
# Initialize AutoGen agent
agent = AutoGenAgent()
agent.generate_code(prompt='Create a function to add two numbers')
AgentVerse
Multi-Agent Systems: Designed for creating and managing complex multi-agent interactions.
Simulation Environment: Provides tools for simulating agent interactions.
from agentverse import AgentVerseSystem
# Initialize AgentVerse system
system = AgentVerseSystem()
system.add_agents(['agent1', 'agent2'])
system.simulate()
ChatDev
Chatbot Development: Specialized in creating advanced chatbot applications.
Customization: Highly customizable to suit specific business needs.
from chatdev import ChatBot
# Initialize ChatDev chatbot
chatbot = ChatBot()
chatbot.train(training_data='path/to/training/data')
Generative Agents
Simulated Human Behavior: Creates agents that mimic human interactions.
Advanced AI: Uses cutting-edge AI techniques for more realistic simulations.
from generativeagents import SimulacraAgent
# Initialize Generative Agent
agent = SimulacraAgent()
agent.simulate_behavior(behavior_model='human-like')
Explanation of Community-Built Frameworks, Including Usage and Features
Community-built frameworks often provide innovative solutions and additional features, contributed by developers worldwide. These frameworks can be invaluable for specific needs and offer unique advantages.
Customization: Tailored to niche use cases and specific project requirements.
Open Source: Freely available and often supported by active developer communities.
Innovative Features: Community contributions often lead to rapidly developing new and valuable features.
With these tools and frameworks, you can develop powerful LLM agent applications. Next, let's explore how to choose the right agent framework for your specific needs.
LLM Agent Framework App Build: Choosing the Right Agent Framework
Selecting the right agent framework is crucial in building practical LLM agent applications. The choice depends on various factors, including the complexity of tasks, scalability requirements, and the specific features needed for the LLM agent framework application build.
This section will guide you through the evaluation criteria for selecting an agent framework, highlight the differences between single-agent and multi-agent frameworks, and discuss the importance of simulation environments for multi-agent systems.
Evaluation Criteria for Selecting an Agent Framework
When choosing an agent framework, it’s essential to consider several key criteria to ensure that the framework aligns with your project requirements and goals.
Scalability
Handling Load: The framework should be capable of handling the maximum load you expect, whether it’s the number of requests per second or the amount of data processed.
Growth Potential: It should be able to grow with your project, accommodating more complex tasks and larger datasets over time.
Flexibility
Modular Design: Supports adding, removing, or modifying components without significant rework.
Community and Support
Documentation: Comprehensive and clear documentation to help you understand and use the framework effectively.
Community Engagement: An active community can provide support, plugins, and shared experiences that can be invaluable.
Ease of Use
Learning Curve: How quickly your team can get up to speed with the framework.
Implementation Simplicity: How easy it is to implement and start building with the framework.
Importance of Simulation Environments for Multi-Agent Systems
Simulation environments play a vital role in the development and testing of multi-agent systems. They provide a controlled setting where agents can interact, perform tasks, and adapt to various scenarios, ensuring robust performance before deployment.
Controlled Testing
Allows for thorough testing of agent interactions and behaviors in a risk-free environment.
Identifies potential issues and bugs in the early stages of development.
Performance Optimization
Helps in identifying bottlenecks and optimizing agent performance.
Provides insights into how agents can be improved and fine-tuned for better results.
Scenario Analysis
Enables the simulation of different scenarios to assess agent responses and adapt strategies accordingly.
Tests the robustness of agents under various conditions and stresses.
With these guidelines, you can make informed decisions when selecting the right agent framework for your project, ensuring that it meets your specific needs and delivers optimal performance.
Now, let's delve deeper into the building blocks of an LLM agent framework application.
LLM Agent Frameworks: Building Blocks
Creating a practical LLM agent framework requires assembling several vital components that work together seamlessly. These components form the backbone of any robust LLM agent, ensuring it can handle complex tasks and provide accurate responses.
This section will explore the essential tools and modules needed to develop a QA agent, discuss the significance of the Retrieval-augmented generation (RAG) pipeline, and delve into the development of mathematical tools, question decomposition modules, and memory modules.
Tools Required for Developing a QA Agent
Developing a QA agent necessitates a suite of tools that enhance its ability to accurately understand and respond to queries. These tools are integral to building a responsive and efficient agent.
Natural Language Processing (NLP) Library
Tools like SpaCy or NLTK for text processing and understanding.
Pre-trained models for language comprehension.
Machine Learning Framework
Libraries such as TensorFlow or PyTorch for model development and training.
Pre-trained models like BERT or GPT for question answering.
Data Retrieval Tools
Systems like Elasticsearch for indexing and retrieving relevant documents.
APIs to fetch data from external sources.
Retrieval-Augmented Generation (RAG) Pipeline & Its Significance
RAG pipeline is a critical component for enhancing the accuracy and relevance of responses in LLM agents. It combines the strengths of retrieval-based and generation-based methods to provide precise answers.
Components of RAG
Retriever: Fetches relevant documents or passages from a large corpus.
Generator: Uses the retrieved documents to generate detailed and accurate answers.
from transformers import RagTokenizer, RagRetriever, RagSequenceForGeneration
# Initialize tokenizer, retriever, and generator
tokenizer = RagTokenizer.from_pretrained("facebook/rag-sequence-nq")
retriever = RagRetriever.from_pretrained("facebook/rag-sequence-nq", index_name="exact")
rag_sequence = RagSequenceForGeneration.from_pretrained("facebook/rag-sequence-nq", retriever=retriever)
input_ids = tokenizer("What is the capital of France?", return_tensors="pt")["input_ids"]
generated = rag_sequence.generate(input_ids)
print(tokenizer.batch_decode(generated, skip_special_tokens=True))
Significance of RAG
Improved Accuracy: Enhances the precision of responses by leveraging vast amounts of data.
Development of a Mathematical Tool for Planning
A mathematical tool is essential for performing calculations and planning tasks within the agent framework. This tool helps the agent handle numerical data and perform necessary computations.
Capabilities
Basic Calculations: Addition, subtraction, multiplication, and division.
Advanced Functions: Algebra, calculus, and statistical analysis.
import sympy as sp
# Define symbols
x, y = sp.symbols('x y')
# Perform calculations
equation = sp.Eq(x + y, 10)
solution = sp.solve(equation, x)
print(solution)
Integration
Easily integrates with other modules to perform computations as required.
Provides accurate and reliable results for complex queries.
Construction of a Question Decomposition Module
A question decomposition module is vital for breaking down complex questions into simpler, manageable parts. This ensures that the agent can tackle intricate queries methodically.
Functionality
Question Parsing: Identifies and separates different parts of a complex question.
Task Delegation: Assigns sub-tasks to appropriate modules for processing.
def decompose_question(question):
# Example decomposition logic
if "and" in question:
parts = question.split("and")
else:
parts = [question]
return parts
# Decompose a complex question
question = "What is the capital of France and who is the president?"
sub_questions = decompose_question(question)
print(sub_questions)
With these building blocks in place, you can develop a robust and efficient LLM agent framework application capable of handling complex tasks and providing accurate answers. Next, we will explore how to develop the core of your LLM agent, integrating these components into a cohesive system.
Developing the Core of Your LLM Agent
The core of your LLM agent is the central processing unit that orchestrates all its operations. This section covers the different types of solvers used in the agent core, the integration process for various components such as tools, planners, and memory modules, and the evaluation of the mental model to ensure the agent's effectiveness.
Developing a robust core is crucial for the agent's ability to handle complex tasks efficiently and accurately.
Introduction to Different Solver Types for the Agent Core
Choosing the right solver type for your agent core is essential for optimizing performance and task management. Different solvers offer unique benefits and are suited to various levels of complexity in problem-solving.
Linear Solver
Simple Execution: Follows a straightforward, linear path to solve tasks.
Ease of Implementation: Suitable for simpler tasks that do not require complex decision-making processes.
def linear_solver(tasks):
results = []
for task in tasks:
result = perform_task(task)
results.append(result)
return results
def perform_task(task):
# Define task execution logic here
return f"Result of {task}"
tasks = ["task1", "task2", "task3"]
print(linear_solver(tasks))
Single-Thread Recursive Solver
Depth-First Approach: Solves tasks by recursively breaking them into sub-tasks and processing them individually.
Improved Problem-Solving: Suitable for tasks that require a more detailed and step-by-step approach.
def single_thread_recursive_solver(task):
if is_complex_task(task):
sub_tasks = decompose_task(task)
results = [single_thread_recursive_solver(sub_task) for sub_task in sub_tasks]
return combine_results(results)
else:
return perform_task(task)
def is_complex_task(task):
# Define logic to determine if a task is complex
return "complex" in task
def decompose_task(task):
# Define logic to decompose task into sub-tasks
return [f"{task}_part1", f"{task}_part2"]
def combine_results(results):
# Define logic to combine results of sub-tasks
return " & ".join(results)
def perform_task(task):
# Define task execution logic here
return f"Result of {task}"
task = "complex_task"
print(single_thread_recursive_solver(task)
Multi-Thread Recursive Solver
Parallel Processing: Executes multiple tasks or sub-tasks simultaneously, improving efficiency and reducing latency.
High Complexity Handling: Ideal for handling highly complex tasks that benefit from concurrent execution.
import concurrent.futures
def multi_thread_recursive_solver(task):
if is_complex_task(task):
sub_tasks = decompose_task(task)
with concurrent.futures.ThreadPoolExecutor() as executor:
results = list(executor.map(multi_thread_recursive_solver, sub_tasks))
return combine_results(results)
else:
return perform_task(task)
# Reuse the previously defined functions is_complex_task, decompose_task, combine_results, and perform_task
task = "complex_task"
print(multi_thread_recursive_solver(task))
Integration Process of Tools, Planner, and Memory Module in the Agent Setup
Integrating the various components of your LLM agent—such as tools, the planner, and the memory module—is critical to ensure they work together seamlessly and efficiently. This process involves setting up communication channels and workflows between these components.
Tools Integration
Setup and Configuration: Initialize and configure the tools required for the agent to perform specific tasks.
Inter-Component Communication: Ensure that the tools can communicate effectively with other components like the planner and memory module.
class Agent:
def __init__(self, tools, planner, memory):
self.tools = tools
self.planner = planner
self.memory = memory
def integrate_tools(self):
for tool in self.tools:
tool.setup()
print("Tools integrated successfully")
class Tool:
def setup(self):
# Define tool setup logic here
print("Tool setup complete")
tools = [Tool(), Tool()]
agent = Agent(tools, None, None)
agent.integrate_tools()
Planner Integration
Task Decomposition: The planner decomposes complex tasks into manageable sub-tasks.
Task Assignment: Assigns sub-tasks to the appropriate tools and manages their execution.
class Planner:
def __init__(self):
self.plan = []
def create_plan(self, task):
self.plan = self.decompose_task(task)
def decompose_task(self, task):
# Decompose the task into sub-tasks
return [f"{task}_sub1", f"{task}_sub2"]
def assign_tasks(self, agent):
for sub_task in self.plan:
agent.execute_task(sub_task)
planner = Planner()
planner.create_plan("complex_task")
Memory Module Integration
Information Retrieval: Retrieves relevant information from past interactions to aid in current task processing.
class MemoryModule:
def __init__(self):
self.memory = []
def store_interaction(self, question, answer):
self.memory.append({"question": question, "answer": answer})
def retrieve_interaction(self, question):
for interaction in self.memory:
if interaction["question"] == question:
return interaction["answer"]
return None
memory = MemoryModule()
memory.store_interaction("What is the capital of France?", "Paris")
Full Integration Example
Combining All Components: Integrate tools, the planner, and the memory module into the agent setup.
class FullAgent:
def __init__(self, tools, planner, memory):
self.tools = tools
self.planner = planner
self.memory = memory
def execute_task(self, task):
if task in [t.get_task_name() for t in self.tools]:
tool = next(t for t in self.tools if t.get_task_name() == task)
return tool.perform_task()
else:
return "Task not supported"
tools = [Tool(), Tool()]
planner = Planner()
memory = MemoryModule()
agent = FullAgent(tools, planner, memory)
# Integrate tools, create plan, and execute tasks
agent.integrate_tools()
planner.create_plan("complex_task")
planner.assign_tasks(agent)
Evaluation of the Mental Model for Agent Effectiveness
Evaluating your LLM agent's mental model is crucial to ensuring its effectiveness and efficiency. This involves testing the agent's reasoning capabilities, ability to handle various tasks, and overall performance in real-world scenarios.
Reasoning Capabilities
Logic and Decision-Making: Assess how well the agent can make logical decisions based on available information.
Complex Problem Solving: Test the agent's ability to solve complex problems through reasoning.
Task Handling
Task Diversity: Evaluate the agent's ability to handle various tasks, from simple queries to complex multi-step processes.
Efficiency: Measure the speed and accuracy with which the agent completes tasks.
Performance Metrics
Accuracy: Determine the accuracy of the agent's responses and its success rate in task completion.
User Satisfaction: Gather user feedback to assess the effectiveness and usability of the agent.
class AgentEvaluator:
def __init__(self, agent):
self.agent = agent
def evaluate(self, tasks):
results = []
for task in tasks:
result = self.agent.execute_task(task)
results.append(result)
return results
def calculate_accuracy(self, results, expected_results):
correct = sum([1 for result, expected in zip(results, expected_results) if result == expected])
return correct / len(results)
# Example usage
evaluator = AgentEvaluator(agent)
tasks = ["simple_task", "complex_task_sub1", "complex_task_sub2"]
results = evaluator.evaluate(tasks)
expected_results = ["Result of simple_task", "Result of complex_task_sub1", "Result of complex_task_sub2"]
accuracy = evaluator.calculate_accuracy(results, expected_results)
print(f"Agent Accuracy: {accuracy * 100}%")
By developing a robust core and integrating various components effectively, your LLM agent can perform complex tasks with high efficiency and accuracy. Evaluating the agent's mental model ensures that it remains effective and reliable in real-world applications.
Recommended Reading and Resources
Exploring additional resources and reading materials is essential to further enhancing your understanding and capabilities in developing LLM agent frameworks.
These resources provide in-depth knowledge, case studies, and practical examples to help you build more sophisticated and effective LLM agents.
AutoGPT, Voyager, OlaGPT, MRKL Systems, Generative Agents: Interactive Simulacra of Human Behavior
Exploring the following projects and systems will give you a comprehensive view of the state-of-the-art LLM agent development and the potential for creating advanced AI applications.
AutoGPT
Overview: AutoGPT is an innovative project that automates the generation of GPT-based agents. It showcases the potential for automating complex tasks using language models.
Key Features: Automated task handling, minimal human intervention, and adaptive learning capabilities.
Useful Links: AutoGPT GitHub Repository
Voyager
Overview: Voyager is a project by NVIDIA Research that focuses on self-improving agents capable of learning new tools and building new capabilities without external intervention.
Key Features: Self-improvement, autonomous tool learning, and advanced problem-solving skills.
Useful Links: Voyager Project Page
OlaGPT
Overview: OlaGPT is a conceptual framework that stimulates ideas on how to extend the capabilities of basic agents. It focuses on enhancing agent interactions and performance.
Key Features: Conceptual extensions, interaction enhancements, and performance optimization.
Useful Links: OlaGPT Research Paper
MRKL Systems
Overview: MRKL Systems (Modular Reasoning, Knowledge, and Language) combine large language models with external knowledge sources and discrete reasoning for executing complex tasks.
Key Features: Integration of language models with knowledge bases, modular architecture, and enhanced reasoning capabilities.
Useful Links: MRKL Systems Paper
Generative Agents: Interactive Simulacra of Human Behavior
Overview: This project explores the creation of agents that simulate human behavior through decentralized interactions. It's one of the pioneering efforts in developing swarm intelligence.
Key Features: Decentralized agent interactions, human-like behavior simulation, and advanced AI techniques.
Useful Links: Generative Agents Research
LLM-Powered Agent List for Further Reading and Resources
The Awesome LLM-Powered Agent list is a curated collection of resources, tools, and examples that can provide further insights and practical knowledge for developing LLM agents. It is a valuable resource for both beginners and experienced developers.
Overview
The list includes various topics, from foundational theories to advanced implementations, and is regularly updated by the community.
Key Sections
Tutorials and Guides: Step-by-step tutorials and guides for building LLM agents.
Libraries and Frameworks: Information on various libraries and frameworks that support LLM agent development.
Case Studies: Real-world examples and case studies showcasing successful LLM agent implementations.
Research Papers: Access to significant research papers that provide theoretical and practical insights.
Useful Links
Excellent LLM-Powered Agent List: Awesome LLM-Powered Agent GitHub Repository
Additional Resources: NVIDIA AI Playground
By diving into these recommended readings and resources, you will gain a deeper understanding of the advanced concepts and practical applications of LLM agent frameworks. These materials will help you stay updated with the latest developments and enhance your skills in building sophisticated AI agents.
Summary
Building an LLM agent framework application involves understanding and integrating key components such as solvers, tools, planners, and memory modules. Each element is crucial in ensuring the agent's efficiency, accuracy, and contextual relevance. You can create robust and effective LLM agents by leveraging recommended frameworks and resources.
Looking ahead, the future of LLM agent applications is bright. They have the potential to revolutionize various industries by automating complex tasks and enhancing user interactions. The continuous advancements in AI and machine learning will drive further improvements in agent capabilities and applications. Embracing these innovations will allow businesses to unlock new levels of productivity and efficiency.
How Can RAGA AI Help You in Developing Your First LLM Agent Framework Application?
RAGA AI offers a comprehensive and user-friendly platform designed to simplify developing your first LLM agent framework application. With its robust set of tools and integrated modules, RAGA AI enables you to seamlessly build, test, and deploy advanced LLM agents without extensive technical expertise.
Additionally, RAGA AI's innovative features, such as automated issue detection, memory modules, and planning tools, ensure that your agents perform efficiently and accurately, help.
So Sign Up for RAGA's LLM Hub and get your LLM applications ready 3X faster!
Building your first LLM agent framework application can feel daunting, but it doesn’t have to be. Understanding the core elements—agent cores, memory modules, agent tools, and planning modules—is crucial for success.
In today’s rapidly evolving AI landscape, developing an LLM agent framework application can significantly enhance your AI capabilities. This blog will explore the available implementation frameworks, compare single-agent and multi-agent systems, and highlight popular options to help you make informed decisions. Let’s dive into the developer ecosystem for LLM agents.
LLM Agent Framework Application: Overview
In this section, we will break down the key concepts, starting with LLM agent frameworks, how they differ from traditional chatbots, and the essential components of developing a robust LLM agent application.
LLM Agent Frameworks
LLM agent frameworks are designed to enable large language models to perform complex tasks by integrating multiple functionalities and tools.
Unlike simple chatbot applications, these frameworks allow dynamic problem-solving and advanced data processing. Key aspects include:
Integration of Various Modules
Memory and Planning Modules: These frameworks integrate different components, such as memory modules that help the agent remember past interactions and planning modules that break down complex tasks into simpler, executable steps.
Functional Diversity: They support various functionalities, from data retrieval to executing computational tasks, ensuring a holistic approach to problem-solving.
Enhanced Flexibility
Dynamic Task Handling: Unlike rigid chatbots, LLM agent frameworks can adapt to various tasks and queries, making them suitable for diverse applications.
Learning and Adaptation: They continuously improve from interactions, adapting to new tasks and refining their responses.
Scalability
Single-Agent to Multi-Agent Systems: These frameworks can be scaled from single-agent systems that handle specific tasks to multi-agent systems that manage more complex, interrelated activities.
Resource Management: Efficiently manage computational resources to maintain performance even as the complexity of tasks increases.
LLM Agent Framework Applications vs. Conventional Chatbot Applications
While both LLM agent frameworks and traditional chatbots are used to interact with users through natural language, there are significant differences:
Complexity
LLM Agent Frameworks: Capable of handling multifaceted tasks involving advanced reasoning and decision-making processes. They are designed to go beyond primary query responses to solve complex problems.
Conventional Chatbots: Typically follow predefined scripts and respond to straightforward queries with limited capacity for complex problem-solving.
Adaptability
LLM Agent Frameworks: Continuously learn from each interaction, allowing for improvement and adaptation to new and varied tasks. They can handle unexpected queries and adapt their strategies accordingly.
Conventional Chatbots: Restricted to their initial programming with minimal learning capabilities. They struggle with queries outside their predefined scope.
LLM Agent Applications: Overview of Essential Components Required
Creating a functional LLM agent framework involves integrating several critical components that work together seamlessly:
Agent Core
Central Processing Unit: This unit manages all tasks, decisions, and actions within the framework. It is the brain of the LLM agent, orchestrating how other components interact.
Task Management: Ensures tasks are executed correctly and coordinates between different modules and tools.
Agent Tools
Specialized Functionalities: Include tools for data retrieval, computational analysis, and external API integrations. These tools extend the agent’s capabilities to perform specific tasks efficiently.
Tool Integration: Seamlessly integrates with external tools and services to enhance the agent’s functionality and provide more comprehensive solutions.
Planning Module
Task Decomposition: Breaks down complex queries into manageable sub-tasks, ensuring logical and efficient execution.
Execution Strategy: Develops a plan for tackling each subtask and integrate results to provide coherent answers to complex queries.
By understanding these components, you can build a more efficient and capable LLM agent framework application, setting the stage for advanced AI solutions. This foundational knowledge will help you navigate the complexities of AI development, leading to the successful implementation and deployment of LLM agents in your projects.
With a solid grasp of the fundamental concepts and components of LLM agent framework applications, it's time to explore the tools and frameworks available for developers. Let's dive into the developer ecosystem for LLM agents.
LLM Agent Framework: Developing Ecosystem
The development of the LLM agent framework is supported by a robust ecosystem of frameworks and tools, each offering unique features and capabilities. Understanding these options is essential for selecting the proper framework for your project.
This section will introduce you to the available implementation frameworks, compare single-agent and multi-agent systems, highlight popular frameworks, and discuss community-built solutions.
Developing LLM Agent Framework: Introduction to Available Implementation Frameworks
Several frameworks are available for developing LLM agents, each designed to streamline the development process and enhance functionality. These frameworks provide the building blocks needed to create sophisticated AI agents capable of handling complex tasks.
LangChain: A versatile framework that supports the creation of modular agents with various tools and integrations.
LLaMaIndex: Focuses on indexing and retrieving data efficiently, making it ideal for data-heavy applications.
HayStack: A robust framework designed for building search and question-answering systems.
Example code to initialize a LangChain agent:
from langchain.agents import Agent
# Initialize LangChain agent
agent = Agent()
agent.initialize()
Comparison of Single-Agent vs. Multi-Agent Frameworks
Choosing between single-agent and multi-agent frameworks depends on your application's complexity and requirements. Each type offers distinct advantages and is suited to different use cases.
Single-Agent Frameworks
These frameworks focus on a single AI agent that handles specific tasks independently, offering simplicity and ease of implementation.
Simplicity: Easier to implement and manage.
Focused Tasks: Ideal for applications with specific, isolated tasks.
Example Framework: LangChain.
Example code to create a single-agent system:
from langchain.agents import SingleAgent
# Create a single-agent instance
agent = SingleAgent()
agent.run()
Multi-Agent Frameworks
These frameworks involve multiple AI agents working together, enabling complex interactions and scalability for more intricate applications.
Complex Interactions: Capable of handling multiple, interconnected tasks.
Scalability: Suitable for large-scale applications requiring coordination between agents.
Example Framework: AgentVerse.
Example code to create a multi-agent system:
from agentverse import MultiAgentSystem
# Create a multi-agent system
system = MultiAgentSystem()
system.initialize_agents(num_agents=5)
system.run()
Highlighting Popular Frameworks
Several frameworks have gained popularity due to their robust features and community support. Here are some of the top choices for developing LLM agents:
LangChain Agents
Modular Design: Easily integrates with various tools and services.
Strong Community: Extensive documentation and community support.
from langchain.agents import LangChainAgent
# Initialize LangChain agent with specific tools
agent = LangChainAgent(tools=['tool1', 'tool2'])
agent.run()
LLaMaIndex Agents
Efficient Data Handling: Optimized for indexing and data retrieval.
Scalable: Handles large datasets effectively.
from llamaindex import LLaMaAgent
# Initialize LLaMa agent for data indexing
agent = LLaMaAgent(index_path='path/to/index')
agent.index_data(data_source='data/source/path')
HayStack Agents
Search and QA: Built for creating powerful search engines and question-answering systems.
Flexibility: Supports a variety of backends and data sources.
from haystack.agents import HayStackAgent
# Initialize HayStack agent for QA
agent = HayStackAgent()
agent.build_qa_pipeline(model_name='bert-base-uncased')
AutoGen
Automated Code Generation: Facilitates the automatic generation of code based on user input.
Ease of Use: Simplifies the development process with minimal manual coding.
from autogen import AutoGenAgent
# Initialize AutoGen agent
agent = AutoGenAgent()
agent.generate_code(prompt='Create a function to add two numbers')
AgentVerse
Multi-Agent Systems: Designed for creating and managing complex multi-agent interactions.
Simulation Environment: Provides tools for simulating agent interactions.
from agentverse import AgentVerseSystem
# Initialize AgentVerse system
system = AgentVerseSystem()
system.add_agents(['agent1', 'agent2'])
system.simulate()
ChatDev
Chatbot Development: Specialized in creating advanced chatbot applications.
Customization: Highly customizable to suit specific business needs.
from chatdev import ChatBot
# Initialize ChatDev chatbot
chatbot = ChatBot()
chatbot.train(training_data='path/to/training/data')
Generative Agents
Simulated Human Behavior: Creates agents that mimic human interactions.
Advanced AI: Uses cutting-edge AI techniques for more realistic simulations.
from generativeagents import SimulacraAgent
# Initialize Generative Agent
agent = SimulacraAgent()
agent.simulate_behavior(behavior_model='human-like')
Explanation of Community-Built Frameworks, Including Usage and Features
Community-built frameworks often provide innovative solutions and additional features, contributed by developers worldwide. These frameworks can be invaluable for specific needs and offer unique advantages.
Customization: Tailored to niche use cases and specific project requirements.
Open Source: Freely available and often supported by active developer communities.
Innovative Features: Community contributions often lead to rapidly developing new and valuable features.
With these tools and frameworks, you can develop powerful LLM agent applications. Next, let's explore how to choose the right agent framework for your specific needs.
LLM Agent Framework App Build: Choosing the Right Agent Framework
Selecting the right agent framework is crucial in building practical LLM agent applications. The choice depends on various factors, including the complexity of tasks, scalability requirements, and the specific features needed for the LLM agent framework application build.
This section will guide you through the evaluation criteria for selecting an agent framework, highlight the differences between single-agent and multi-agent frameworks, and discuss the importance of simulation environments for multi-agent systems.
Evaluation Criteria for Selecting an Agent Framework
When choosing an agent framework, it’s essential to consider several key criteria to ensure that the framework aligns with your project requirements and goals.
Scalability
Handling Load: The framework should be capable of handling the maximum load you expect, whether it’s the number of requests per second or the amount of data processed.
Growth Potential: It should be able to grow with your project, accommodating more complex tasks and larger datasets over time.
Flexibility
Modular Design: Supports adding, removing, or modifying components without significant rework.
Community and Support
Documentation: Comprehensive and clear documentation to help you understand and use the framework effectively.
Community Engagement: An active community can provide support, plugins, and shared experiences that can be invaluable.
Ease of Use
Learning Curve: How quickly your team can get up to speed with the framework.
Implementation Simplicity: How easy it is to implement and start building with the framework.
Importance of Simulation Environments for Multi-Agent Systems
Simulation environments play a vital role in the development and testing of multi-agent systems. They provide a controlled setting where agents can interact, perform tasks, and adapt to various scenarios, ensuring robust performance before deployment.
Controlled Testing
Allows for thorough testing of agent interactions and behaviors in a risk-free environment.
Identifies potential issues and bugs in the early stages of development.
Performance Optimization
Helps in identifying bottlenecks and optimizing agent performance.
Provides insights into how agents can be improved and fine-tuned for better results.
Scenario Analysis
Enables the simulation of different scenarios to assess agent responses and adapt strategies accordingly.
Tests the robustness of agents under various conditions and stresses.
With these guidelines, you can make informed decisions when selecting the right agent framework for your project, ensuring that it meets your specific needs and delivers optimal performance.
Now, let's delve deeper into the building blocks of an LLM agent framework application.
LLM Agent Frameworks: Building Blocks
Creating a practical LLM agent framework requires assembling several vital components that work together seamlessly. These components form the backbone of any robust LLM agent, ensuring it can handle complex tasks and provide accurate responses.
This section will explore the essential tools and modules needed to develop a QA agent, discuss the significance of the Retrieval-augmented generation (RAG) pipeline, and delve into the development of mathematical tools, question decomposition modules, and memory modules.
Tools Required for Developing a QA Agent
Developing a QA agent necessitates a suite of tools that enhance its ability to accurately understand and respond to queries. These tools are integral to building a responsive and efficient agent.
Natural Language Processing (NLP) Library
Tools like SpaCy or NLTK for text processing and understanding.
Pre-trained models for language comprehension.
Machine Learning Framework
Libraries such as TensorFlow or PyTorch for model development and training.
Pre-trained models like BERT or GPT for question answering.
Data Retrieval Tools
Systems like Elasticsearch for indexing and retrieving relevant documents.
APIs to fetch data from external sources.
Retrieval-Augmented Generation (RAG) Pipeline & Its Significance
RAG pipeline is a critical component for enhancing the accuracy and relevance of responses in LLM agents. It combines the strengths of retrieval-based and generation-based methods to provide precise answers.
Components of RAG
Retriever: Fetches relevant documents or passages from a large corpus.
Generator: Uses the retrieved documents to generate detailed and accurate answers.
from transformers import RagTokenizer, RagRetriever, RagSequenceForGeneration
# Initialize tokenizer, retriever, and generator
tokenizer = RagTokenizer.from_pretrained("facebook/rag-sequence-nq")
retriever = RagRetriever.from_pretrained("facebook/rag-sequence-nq", index_name="exact")
rag_sequence = RagSequenceForGeneration.from_pretrained("facebook/rag-sequence-nq", retriever=retriever)
input_ids = tokenizer("What is the capital of France?", return_tensors="pt")["input_ids"]
generated = rag_sequence.generate(input_ids)
print(tokenizer.batch_decode(generated, skip_special_tokens=True))
Significance of RAG
Improved Accuracy: Enhances the precision of responses by leveraging vast amounts of data.
Development of a Mathematical Tool for Planning
A mathematical tool is essential for performing calculations and planning tasks within the agent framework. This tool helps the agent handle numerical data and perform necessary computations.
Capabilities
Basic Calculations: Addition, subtraction, multiplication, and division.
Advanced Functions: Algebra, calculus, and statistical analysis.
import sympy as sp
# Define symbols
x, y = sp.symbols('x y')
# Perform calculations
equation = sp.Eq(x + y, 10)
solution = sp.solve(equation, x)
print(solution)
Integration
Easily integrates with other modules to perform computations as required.
Provides accurate and reliable results for complex queries.
Construction of a Question Decomposition Module
A question decomposition module is vital for breaking down complex questions into simpler, manageable parts. This ensures that the agent can tackle intricate queries methodically.
Functionality
Question Parsing: Identifies and separates different parts of a complex question.
Task Delegation: Assigns sub-tasks to appropriate modules for processing.
def decompose_question(question):
# Example decomposition logic
if "and" in question:
parts = question.split("and")
else:
parts = [question]
return parts
# Decompose a complex question
question = "What is the capital of France and who is the president?"
sub_questions = decompose_question(question)
print(sub_questions)
With these building blocks in place, you can develop a robust and efficient LLM agent framework application capable of handling complex tasks and providing accurate answers. Next, we will explore how to develop the core of your LLM agent, integrating these components into a cohesive system.
Developing the Core of Your LLM Agent
The core of your LLM agent is the central processing unit that orchestrates all its operations. This section covers the different types of solvers used in the agent core, the integration process for various components such as tools, planners, and memory modules, and the evaluation of the mental model to ensure the agent's effectiveness.
Developing a robust core is crucial for the agent's ability to handle complex tasks efficiently and accurately.
Introduction to Different Solver Types for the Agent Core
Choosing the right solver type for your agent core is essential for optimizing performance and task management. Different solvers offer unique benefits and are suited to various levels of complexity in problem-solving.
Linear Solver
Simple Execution: Follows a straightforward, linear path to solve tasks.
Ease of Implementation: Suitable for simpler tasks that do not require complex decision-making processes.
def linear_solver(tasks):
results = []
for task in tasks:
result = perform_task(task)
results.append(result)
return results
def perform_task(task):
# Define task execution logic here
return f"Result of {task}"
tasks = ["task1", "task2", "task3"]
print(linear_solver(tasks))
Single-Thread Recursive Solver
Depth-First Approach: Solves tasks by recursively breaking them into sub-tasks and processing them individually.
Improved Problem-Solving: Suitable for tasks that require a more detailed and step-by-step approach.
def single_thread_recursive_solver(task):
if is_complex_task(task):
sub_tasks = decompose_task(task)
results = [single_thread_recursive_solver(sub_task) for sub_task in sub_tasks]
return combine_results(results)
else:
return perform_task(task)
def is_complex_task(task):
# Define logic to determine if a task is complex
return "complex" in task
def decompose_task(task):
# Define logic to decompose task into sub-tasks
return [f"{task}_part1", f"{task}_part2"]
def combine_results(results):
# Define logic to combine results of sub-tasks
return " & ".join(results)
def perform_task(task):
# Define task execution logic here
return f"Result of {task}"
task = "complex_task"
print(single_thread_recursive_solver(task)
Multi-Thread Recursive Solver
Parallel Processing: Executes multiple tasks or sub-tasks simultaneously, improving efficiency and reducing latency.
High Complexity Handling: Ideal for handling highly complex tasks that benefit from concurrent execution.
import concurrent.futures
def multi_thread_recursive_solver(task):
if is_complex_task(task):
sub_tasks = decompose_task(task)
with concurrent.futures.ThreadPoolExecutor() as executor:
results = list(executor.map(multi_thread_recursive_solver, sub_tasks))
return combine_results(results)
else:
return perform_task(task)
# Reuse the previously defined functions is_complex_task, decompose_task, combine_results, and perform_task
task = "complex_task"
print(multi_thread_recursive_solver(task))
Integration Process of Tools, Planner, and Memory Module in the Agent Setup
Integrating the various components of your LLM agent—such as tools, the planner, and the memory module—is critical to ensure they work together seamlessly and efficiently. This process involves setting up communication channels and workflows between these components.
Tools Integration
Setup and Configuration: Initialize and configure the tools required for the agent to perform specific tasks.
Inter-Component Communication: Ensure that the tools can communicate effectively with other components like the planner and memory module.
class Agent:
def __init__(self, tools, planner, memory):
self.tools = tools
self.planner = planner
self.memory = memory
def integrate_tools(self):
for tool in self.tools:
tool.setup()
print("Tools integrated successfully")
class Tool:
def setup(self):
# Define tool setup logic here
print("Tool setup complete")
tools = [Tool(), Tool()]
agent = Agent(tools, None, None)
agent.integrate_tools()
Planner Integration
Task Decomposition: The planner decomposes complex tasks into manageable sub-tasks.
Task Assignment: Assigns sub-tasks to the appropriate tools and manages their execution.
class Planner:
def __init__(self):
self.plan = []
def create_plan(self, task):
self.plan = self.decompose_task(task)
def decompose_task(self, task):
# Decompose the task into sub-tasks
return [f"{task}_sub1", f"{task}_sub2"]
def assign_tasks(self, agent):
for sub_task in self.plan:
agent.execute_task(sub_task)
planner = Planner()
planner.create_plan("complex_task")
Memory Module Integration
Information Retrieval: Retrieves relevant information from past interactions to aid in current task processing.
class MemoryModule:
def __init__(self):
self.memory = []
def store_interaction(self, question, answer):
self.memory.append({"question": question, "answer": answer})
def retrieve_interaction(self, question):
for interaction in self.memory:
if interaction["question"] == question:
return interaction["answer"]
return None
memory = MemoryModule()
memory.store_interaction("What is the capital of France?", "Paris")
Full Integration Example
Combining All Components: Integrate tools, the planner, and the memory module into the agent setup.
class FullAgent:
def __init__(self, tools, planner, memory):
self.tools = tools
self.planner = planner
self.memory = memory
def execute_task(self, task):
if task in [t.get_task_name() for t in self.tools]:
tool = next(t for t in self.tools if t.get_task_name() == task)
return tool.perform_task()
else:
return "Task not supported"
tools = [Tool(), Tool()]
planner = Planner()
memory = MemoryModule()
agent = FullAgent(tools, planner, memory)
# Integrate tools, create plan, and execute tasks
agent.integrate_tools()
planner.create_plan("complex_task")
planner.assign_tasks(agent)
Evaluation of the Mental Model for Agent Effectiveness
Evaluating your LLM agent's mental model is crucial to ensuring its effectiveness and efficiency. This involves testing the agent's reasoning capabilities, ability to handle various tasks, and overall performance in real-world scenarios.
Reasoning Capabilities
Logic and Decision-Making: Assess how well the agent can make logical decisions based on available information.
Complex Problem Solving: Test the agent's ability to solve complex problems through reasoning.
Task Handling
Task Diversity: Evaluate the agent's ability to handle various tasks, from simple queries to complex multi-step processes.
Efficiency: Measure the speed and accuracy with which the agent completes tasks.
Performance Metrics
Accuracy: Determine the accuracy of the agent's responses and its success rate in task completion.
User Satisfaction: Gather user feedback to assess the effectiveness and usability of the agent.
class AgentEvaluator:
def __init__(self, agent):
self.agent = agent
def evaluate(self, tasks):
results = []
for task in tasks:
result = self.agent.execute_task(task)
results.append(result)
return results
def calculate_accuracy(self, results, expected_results):
correct = sum([1 for result, expected in zip(results, expected_results) if result == expected])
return correct / len(results)
# Example usage
evaluator = AgentEvaluator(agent)
tasks = ["simple_task", "complex_task_sub1", "complex_task_sub2"]
results = evaluator.evaluate(tasks)
expected_results = ["Result of simple_task", "Result of complex_task_sub1", "Result of complex_task_sub2"]
accuracy = evaluator.calculate_accuracy(results, expected_results)
print(f"Agent Accuracy: {accuracy * 100}%")
By developing a robust core and integrating various components effectively, your LLM agent can perform complex tasks with high efficiency and accuracy. Evaluating the agent's mental model ensures that it remains effective and reliable in real-world applications.
Recommended Reading and Resources
Exploring additional resources and reading materials is essential to further enhancing your understanding and capabilities in developing LLM agent frameworks.
These resources provide in-depth knowledge, case studies, and practical examples to help you build more sophisticated and effective LLM agents.
AutoGPT, Voyager, OlaGPT, MRKL Systems, Generative Agents: Interactive Simulacra of Human Behavior
Exploring the following projects and systems will give you a comprehensive view of the state-of-the-art LLM agent development and the potential for creating advanced AI applications.
AutoGPT
Overview: AutoGPT is an innovative project that automates the generation of GPT-based agents. It showcases the potential for automating complex tasks using language models.
Key Features: Automated task handling, minimal human intervention, and adaptive learning capabilities.
Useful Links: AutoGPT GitHub Repository
Voyager
Overview: Voyager is a project by NVIDIA Research that focuses on self-improving agents capable of learning new tools and building new capabilities without external intervention.
Key Features: Self-improvement, autonomous tool learning, and advanced problem-solving skills.
Useful Links: Voyager Project Page
OlaGPT
Overview: OlaGPT is a conceptual framework that stimulates ideas on how to extend the capabilities of basic agents. It focuses on enhancing agent interactions and performance.
Key Features: Conceptual extensions, interaction enhancements, and performance optimization.
Useful Links: OlaGPT Research Paper
MRKL Systems
Overview: MRKL Systems (Modular Reasoning, Knowledge, and Language) combine large language models with external knowledge sources and discrete reasoning for executing complex tasks.
Key Features: Integration of language models with knowledge bases, modular architecture, and enhanced reasoning capabilities.
Useful Links: MRKL Systems Paper
Generative Agents: Interactive Simulacra of Human Behavior
Overview: This project explores the creation of agents that simulate human behavior through decentralized interactions. It's one of the pioneering efforts in developing swarm intelligence.
Key Features: Decentralized agent interactions, human-like behavior simulation, and advanced AI techniques.
Useful Links: Generative Agents Research
LLM-Powered Agent List for Further Reading and Resources
The Awesome LLM-Powered Agent list is a curated collection of resources, tools, and examples that can provide further insights and practical knowledge for developing LLM agents. It is a valuable resource for both beginners and experienced developers.
Overview
The list includes various topics, from foundational theories to advanced implementations, and is regularly updated by the community.
Key Sections
Tutorials and Guides: Step-by-step tutorials and guides for building LLM agents.
Libraries and Frameworks: Information on various libraries and frameworks that support LLM agent development.
Case Studies: Real-world examples and case studies showcasing successful LLM agent implementations.
Research Papers: Access to significant research papers that provide theoretical and practical insights.
Useful Links
Excellent LLM-Powered Agent List: Awesome LLM-Powered Agent GitHub Repository
Additional Resources: NVIDIA AI Playground
By diving into these recommended readings and resources, you will gain a deeper understanding of the advanced concepts and practical applications of LLM agent frameworks. These materials will help you stay updated with the latest developments and enhance your skills in building sophisticated AI agents.
Summary
Building an LLM agent framework application involves understanding and integrating key components such as solvers, tools, planners, and memory modules. Each element is crucial in ensuring the agent's efficiency, accuracy, and contextual relevance. You can create robust and effective LLM agents by leveraging recommended frameworks and resources.
Looking ahead, the future of LLM agent applications is bright. They have the potential to revolutionize various industries by automating complex tasks and enhancing user interactions. The continuous advancements in AI and machine learning will drive further improvements in agent capabilities and applications. Embracing these innovations will allow businesses to unlock new levels of productivity and efficiency.
How Can RAGA AI Help You in Developing Your First LLM Agent Framework Application?
RAGA AI offers a comprehensive and user-friendly platform designed to simplify developing your first LLM agent framework application. With its robust set of tools and integrated modules, RAGA AI enables you to seamlessly build, test, and deploy advanced LLM agents without extensive technical expertise.
Additionally, RAGA AI's innovative features, such as automated issue detection, memory modules, and planning tools, ensure that your agents perform efficiently and accurately, help.
So Sign Up for RAGA's LLM Hub and get your LLM applications ready 3X faster!