Strategic Approach To Testing AI-Powered Applications And Systems
Strategic Approach To Testing AI-Powered Applications And Systems
Strategic Approach To Testing AI-Powered Applications And Systems
Rehan Asif
Apr 30, 2024
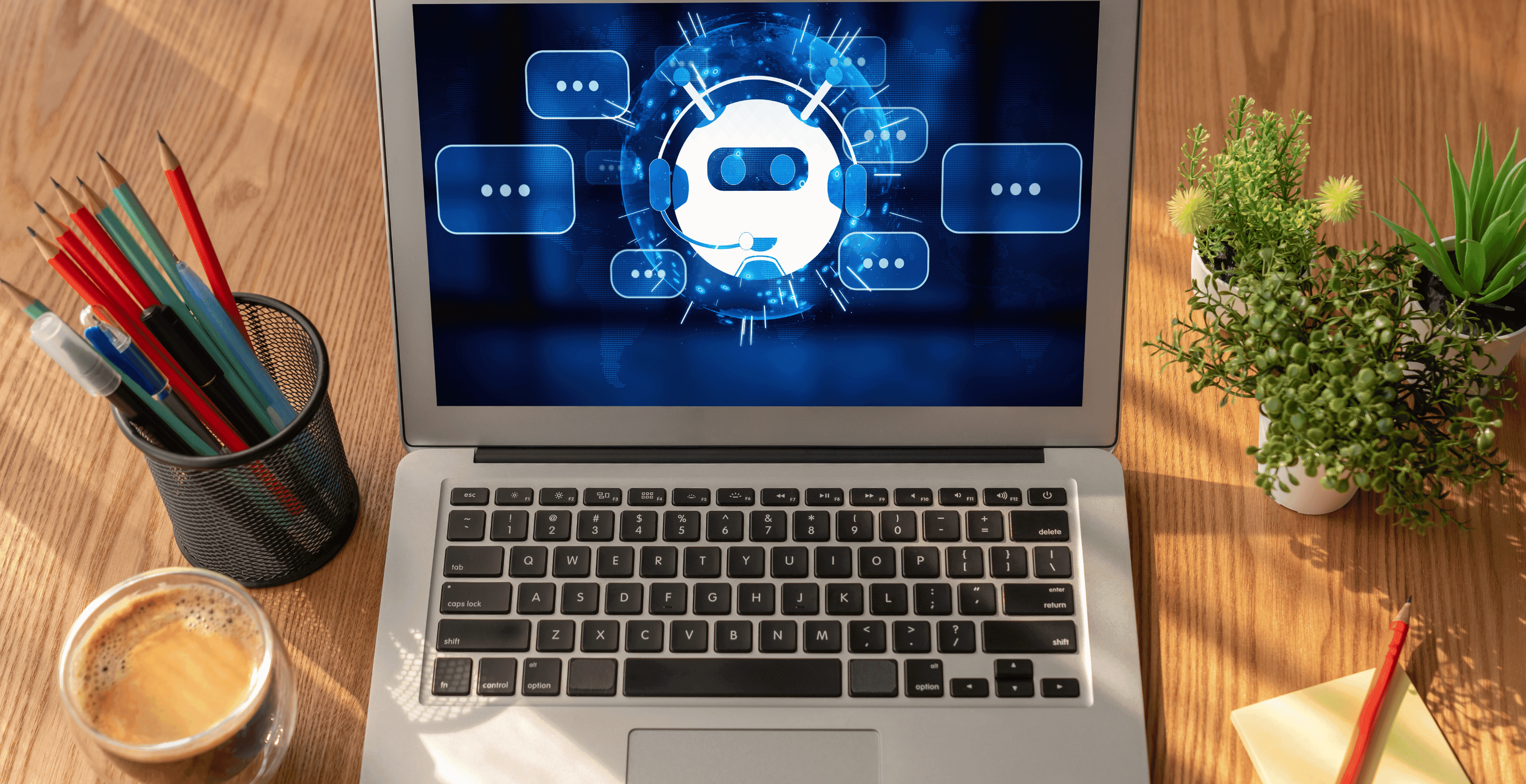
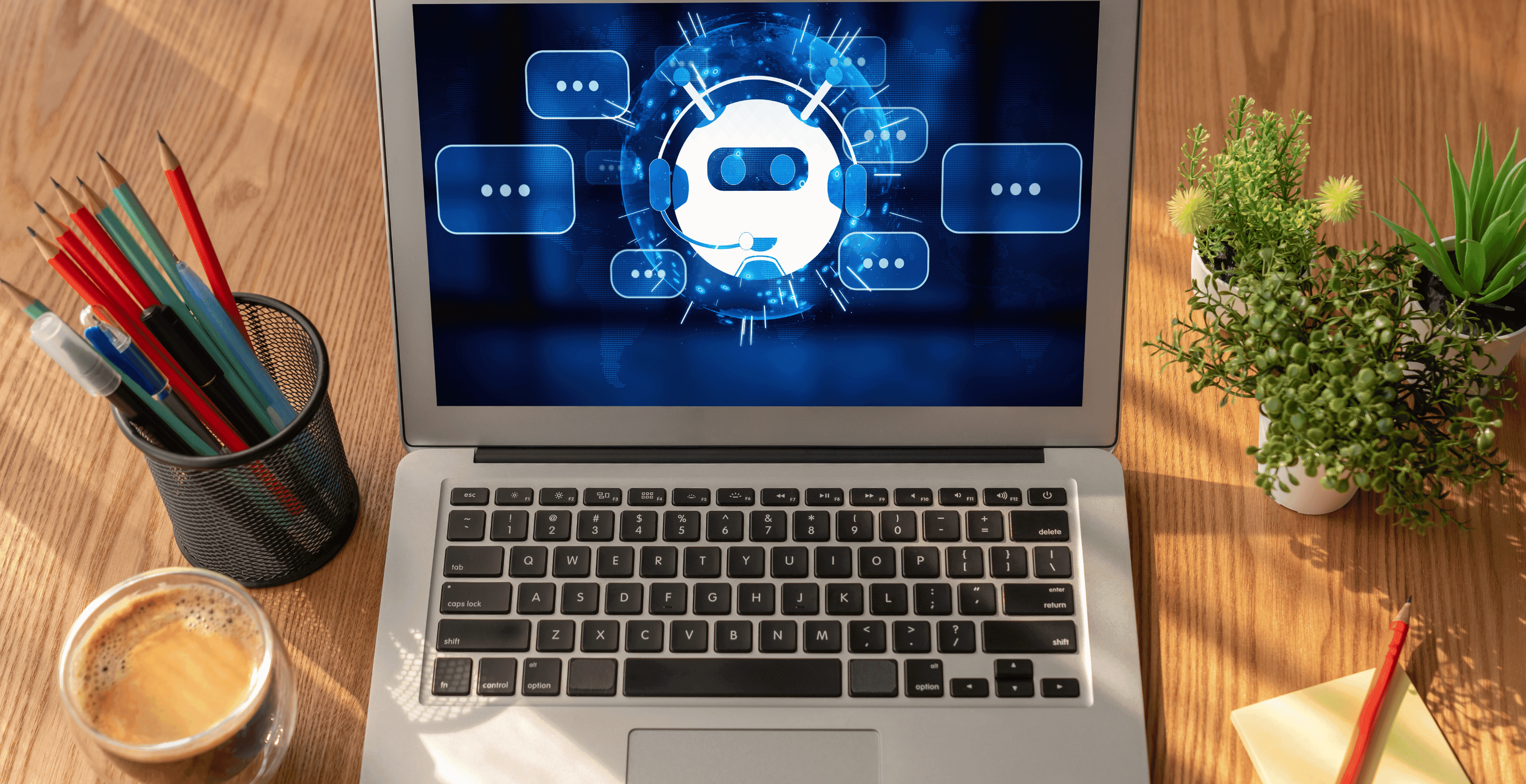
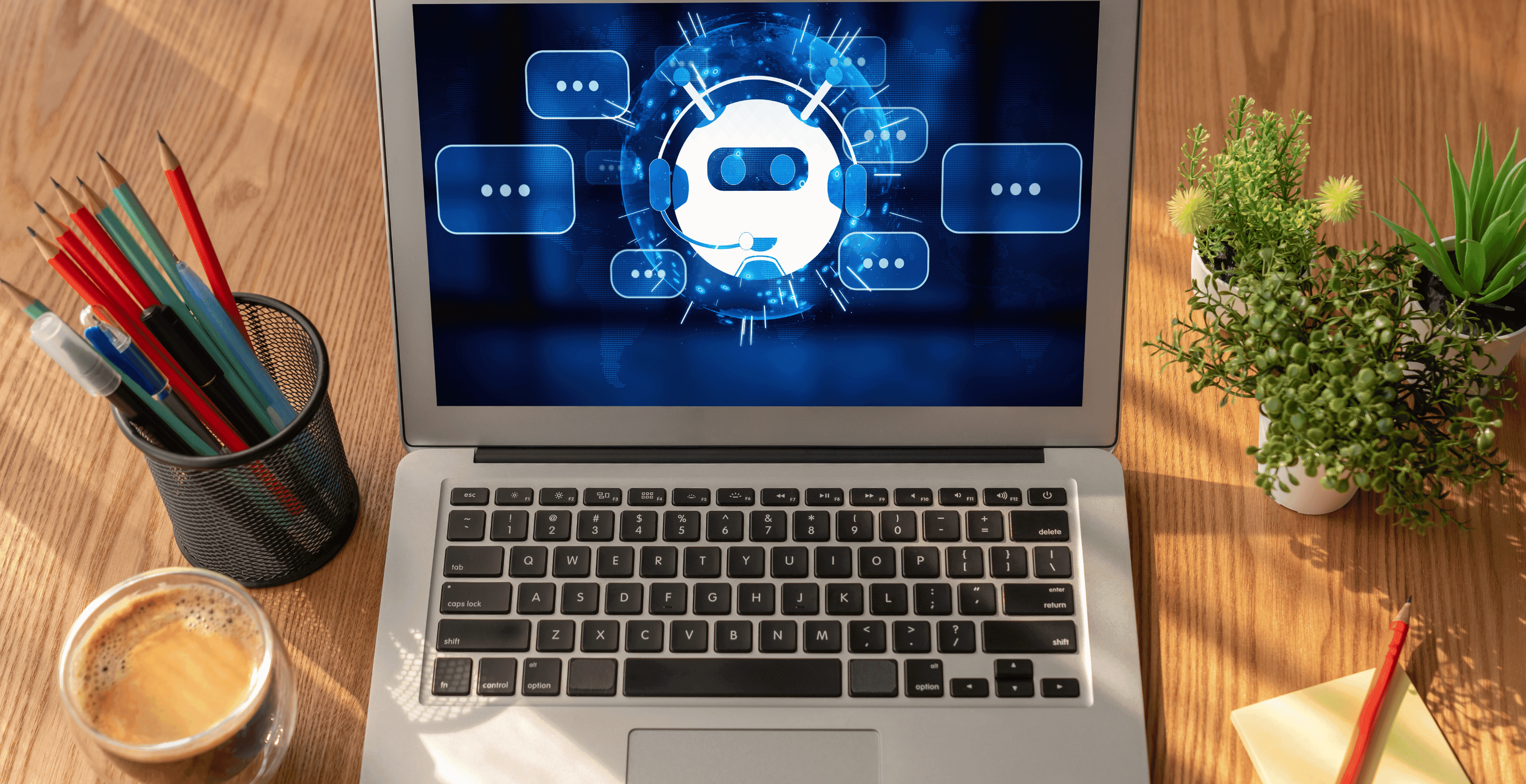
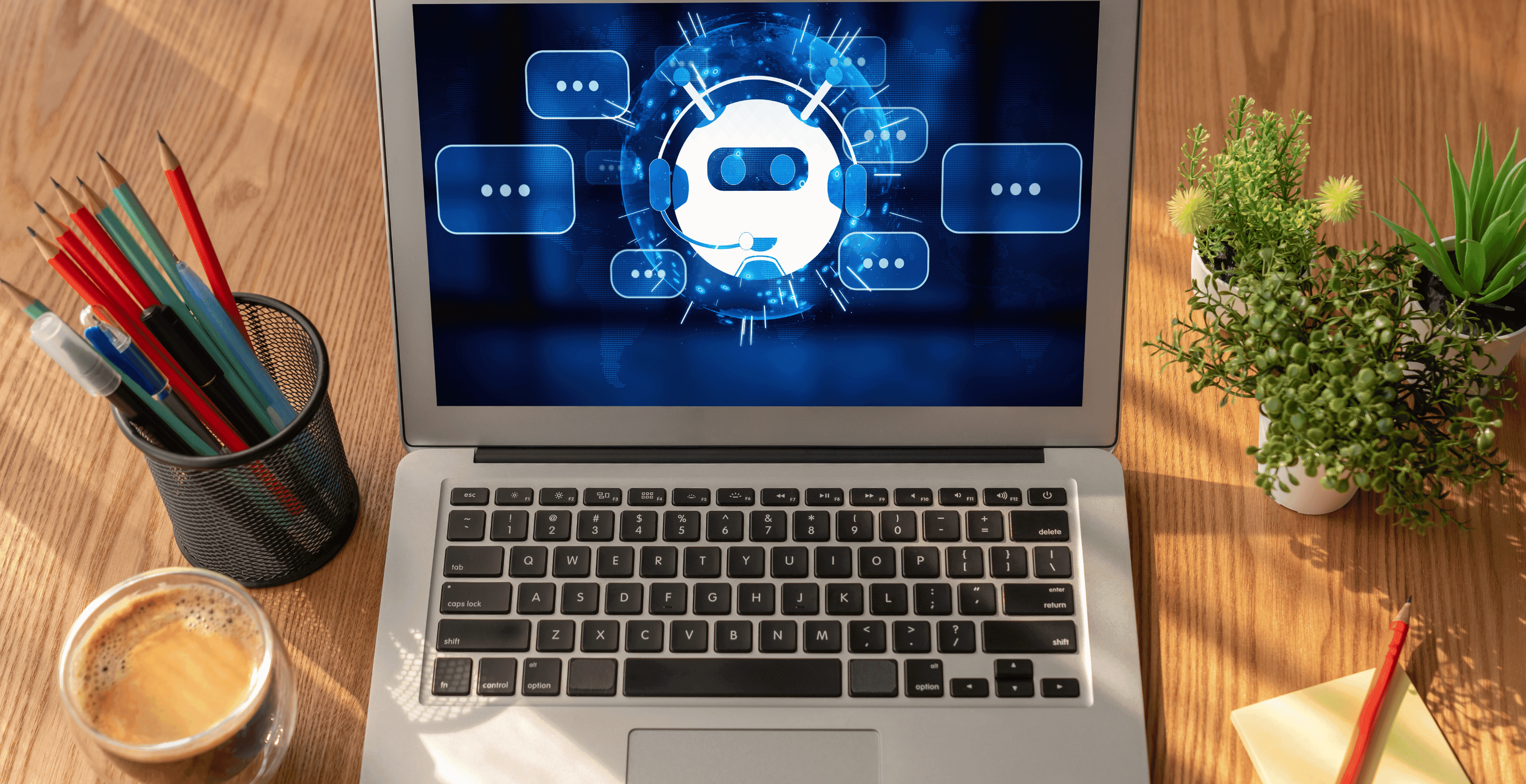
Testing AI (Artificial Intelligence) and ML (Machine Learning) applications involves a series of processes to ensure these systems perform as expected. This includes validating their functionality, accuracy, and reliability under various conditions.
The importance of testing cannot be overstated—it ensures that AI systems are safe, effective, and free from biases that could lead to unfair or harmful outcomes.
Traditional software testing typically involves checking code for bugs and ensuring it meets specified requirements.
However, testing AI systems is inherently more complex due to their probabilistic nature. Unlike traditional software that behaves predictably, AI systems can produce different outputs with the same input, depending on their learning and adaptive algorithms. This unpredictability requires a fundamentally different approach to testing.
Challenges Unique to AI and ML Testing
AI and ML systems pose unique testing challenges due to their reliance on data quality, the complexity of their models, and the need for interpretability. Ensuring these systems function correctly across all possible scenarios can be daunting because they continuously learn and evolve based on new data, potentially leading to changes in their behaviour over time.
As AI technologies continue to permeate various sectors—from healthcare and finance to autonomous driving and customer service—it becomes crucial to adapt testing strategies to address the specific risks associated with AI decision-making. Effective testing strategies help mitigate risks, ensuring that AI systems perform reliably and ethically in real-world applications.
Best Practices for Testing AI/ML Systems
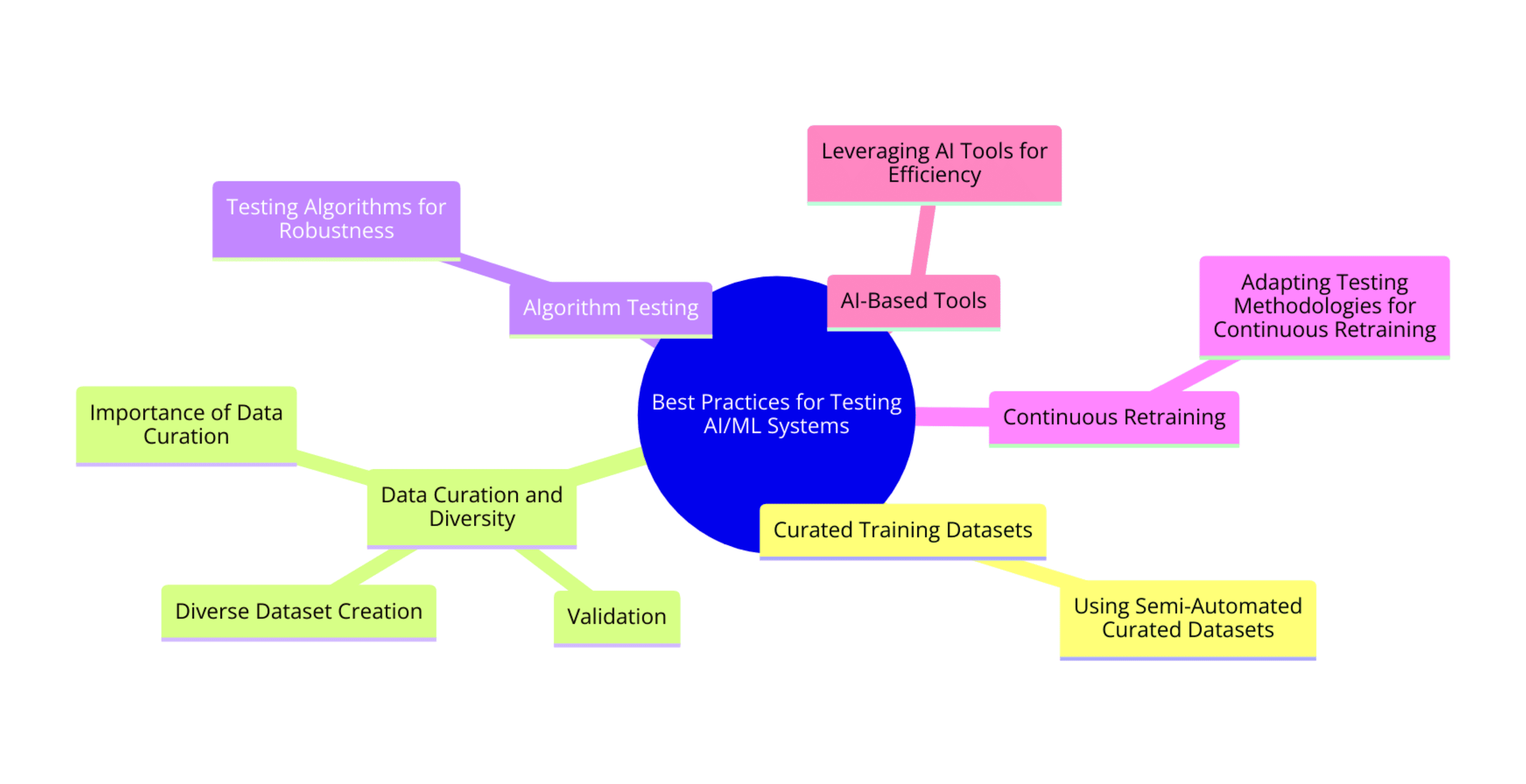
Let's discuss some best practices for effectively testing AI and ML systems. These strategies ensure the systems are functional, efficient, fair, and transparent.
Using Semi-Automated Curated Training Datasets for Effective Testing
One of the foundational steps in testing AI systems is to ensure that the training datasets are well-curated and representative of real-world scenarios. Employing semi-automated tools to curate and verify the quality and diversity of these datasets helps minimise bias and improves the overall robustness of the models.
Importance of Data Curation, Validation, and Diverse Dataset Creation
Data curation and validation are critical to preparing datasets that accurately reflect the complexity of the tasks the AI is designed to perform. This involves removing erroneous data, ensuring data is correctly labelled, and creating datasets that include diverse scenarios and demographics to prevent bias in model training.
Algorithm Testing
Testing AI algorithms involves more than just assessing performance metrics like accuracy or speed. It also includes evaluating the security aspects to prevent adversarial attacks and ensuring that the algorithms integrate well with other software components or systems, maintaining functionality across the technology stack.
# Example code for performance testing of an AI model
from sklearn.metrics import accuracy_score
def test_model_performance(model, features, labels):
predictions = model.predict(features)
accuracy = accuracy_score(labels, predictions)
return accuracy
# Assuming 'model', 'test_features', and 'test_labels' are predefined
model_accuracy = test_model_performance(model, test_features, test_labels)
print(f"Model Accuracy: {model_accuracy}")
Adapting Testing Methodologies for Sustained Testing Due to Continuous Model Retraining
As AI models often undergo continuous retraining to improve their performance or adapt to new data, testing methodologies must also be adapted to accommodate these changes. This includes regular re-evaluation of models to ensure that updates do not degrade the system's performance or introduce new biases.
Leveraging AI-Based Tools for More Efficient Testing Processes
AI-based tools can automate and enhance the testing process. They can simulate various conditions and scenarios faster than manual testing, providing comprehensive insights into model behaviour and potential weaknesses.
# Example code for using an AI-based tool to automate test scenario generation
# Assume 'generate_test_scenarios' is a function provided by an AI-based testing tool
test_scenarios = generate_test_scenarios(model, num_scenarios=100)
results = [test_model(model, scenario) for scenario in test_scenarios]
Employing these best practices in testing AI and ML systems ensures their reliability and efficiency and upholds ethical standards by actively preventing biases and ensuring transparency. Next, we will explore the tools and technologies available for testing AI applications, providing you with practical resources to implement these best practices.
Tools and Technologies for AI Application Testing
Let's explore the various tools and technologies available for testing AI applications. These specialised resources can significantly enhance the efficiency and effectiveness of testing processes.
TensorFlow Extended (TFX)
TensorFlow Extended (TFX) is another powerful tool that offers a suite of libraries and capabilities designed to facilitate the production and testing of ML models. TFX provides components for validating data and model quality, critical for maintaining robust AI systems.
Open Source Tools
There are several open-source tools and libraries that can be used for AI-driven testing:
TensorFlow: A free and open-source software library for machine learning and artificial intelligence. It can be used across a range of applications, including testing.
Selenium: A popular open-source web automation framework for browser automation. While not AI-specific, Selenium provides a foundation for building AI-powered testing tools.
Appium: An open-source test automation framework for mobile apps. It uses the WebDriver protocol to automate mobile apps on iOS and Android platforms.
Robot Framework: A generic test automation framework for acceptance testing and acceptance test-driven development (ATDD). It uses a keyword-driven testing approach and supports various programming languages.
Watir (Web Application Testing in Ruby): Provides open-source Ruby libraries for automating web browsers. It uses Selenium under the hood and supports multiple browsers.
JUnit: A unit testing framework for Java. While not AI-focused, it provides a foundation for building automated tests and can be integrated with AI libraries.
Robotium: An open-source Android UI testing framework that supports testing of native and hybrid Android apps.
These open-source tools and libraries offer a solid starting point for incorporating AI into testing workflows. However, it's important to note that building a fully AI-driven testing solution requires significant effort and expertise. Integrating these tools with AI frameworks like TensorFlow can enable advanced capabilities such as visual testing, self-healing tests, and predictive analytics.
Benefits of Using AI-powered Tools for Smarter, Faster Test Creation and Maintenance
The use of AI-powered tools in testing offers several benefits:
Efficiency: AI tools can quickly generate test cases and scenarios that cover a broad range of conditions, significantly reducing the manual effort required.
Accuracy: These tools help ensure high test accuracy by automatically detecting and adjusting for changes in the application or data that might be missed manually.
Maintenance: AI tools can adapt to changes in the application, automatically updating tests to remain relevant as the application evolves.
These tools and technologies provide critical support in effectively testing AI applications, ensuring that they function as intended and adhere to high standards of quality and ethics. Next, we will explore the different tests that can be conducted on AI-powered applications to ensure comprehensive coverage.
Types of Testing for AI-Powered Applications
Let's explore the diverse types of testing specifically tailored for AI-powered applications, ensuring these systems function correctly across various scenarios and meet all necessary performance benchmarks.
AI-powered applications require thorough testing to ensure they meet functional requirements and perform optimally under different conditions:
Functional Testing checks if the system does what it’s supposed to do according to its requirements.
Usability Testing assesses how easy and intuitive the application is for end-users.
Performance Testing ensures the application performs well under expected workload scenarios.
Integration Testing verifies that the AI integrates seamlessly with other system components.
API Testing confirms that the application programming interfaces work correctly across different platforms.
Security Testing is crucial to ensure the AI system is secure from external threats and data breaches.
Unique Types of Testing
Specialised testing types also play a critical role in AI validation:
Black Box Testing: Testing without prior knowledge of the system architecture or code, focusing solely on the outputs given specific inputs.
White Box Testing involves examining an application's internal structures or workings. It is often used in algorithm testing.
Metamorphic Testing: Involves testing cases where there are no known outputs. It’s beneficial for AI systems where defining test cases is inherently complex.
Non-Functional Testing: Assesses aspects not directly related to specific behaviours or functions, such as scalability and reliability.
Importance of Other Strategies
Model Backtesting: Essential for applications like financial forecasting, where historical data is used to test predictive models.
Performance Testing: Checks the model’s response times and accuracy under various computational loads.
Dual Coding/Algorithm Ensemble Strategies: Using multiple algorithms or models to validate each other’s outputs can enhance reliability and accuracy.
# Example of performance testing using Python
import time
def test_model_speed(model, data):
start_time = time.time()
predictions = model.predict(data)
end_time = time.time()
print(f"Model processed {len(data)} records in {end_time - start_time} seconds.")
# Assuming 'model' and 'data' are predefined
test_model_speed(model, data)
This simple example measures how long it takes to process a data set, providing insight into the model's performance under operational conditions.
Testing AI-powered applications with these comprehensive strategies ensures they are robust, reliable, and ready for real-world deployment. As AI continues to evolve, so too will the approaches to testing. Next, we'll discuss the crucial role of addressing biases and ensuring fairness in AI systems.
Addressing Bias and Fairness in AI Systems
Let's address bias and fairness in AI systems, which are critical to ensuring that AI applications are equitable and do not perpetuate existing disparities.
Strategies to Mitigate Data Skewness, Prediction Bias, and Relational Bias
Bias in AI systems can stem from various sources, particularly from the data used to train these systems. Implementing strategies to mitigate such biases is crucial:
Data Skewness: Ensuring that the training data is representative of the real-world scenarios the AI will encounter can help minimise skewness. This involves including diverse data samples that cover various demographics and conditions.
Prediction Bias: Regularly testing the AI's predictions across different groups and adjusting the model to ensure all groups are treated fairly.
Relational Bias involves analysing the relationships and correlations learned by the AI to ensure they are valid and not based on biased assumptions.
The Role of Fairness Testing Tools in Identifying and Reducing Biases
Fairness testing tools are essential for systematically identifying and mitigating biases in AI systems. These tools can analyze how AI models make decisions and whether certain groups are unfairly treated based on sensitive attributes like race, gender, or age.
Highlighting the Importance of Diverse Testing Scenarios to Ensure AI Fairness
Creating diverse testing scenarios that simulate real-world situations is crucial for evaluating AI fairness. This includes:
Testing AI systems across a broad range of demographic groups.
Synthetic data simulates rare conditions not well-represented in the training data.
Employing adversarial testing to challenge the AI with complex or edge cases.
# Example of using AI Fairness 360 to check for bias in a dataset
from aif360.datasets import BinaryLabelDataset
from aif360.metrics import BinaryLabelDatasetMetric
def assess_dataset_bias(dataset, privileged_groups, unprivileged_groups):
binary_dataset = BinaryLabelDataset(df=dataset, label_names=['label'], protected_attribute_names=['protected_attribute'])
metric = BinaryLabelDatasetMetric(binary_dataset,
unprivileged_groups=unprivileged_groups,
privileged_groups=privileged_groups)
print("Disparate Impact: {:.2f}".format(metric.disparate_impact()))
print("Statistical Parity Difference: {:.2f}".format(metric.statistical_parity_difference()))
# Assuming 'dataset', 'privileged_groups', and 'unprivileged_groups' are predefined
assess_dataset_bias(dataset, privileged_groups, unprivileged_groups)
This snippet demonstrates how to use tools like AI Fairness 360 to assess potential biases in datasets, ensuring that AI models are tested for fairness across all groups.
Addressing bias and ensuring fairness in AI systems are ongoing challenges that require continuous attention and strategy adaptation. These efforts are crucial for building trust and ensuring the ethical deployment of AI technologies. Next, we'll explore practical considerations in AI system testing.
Practical Considerations in AI System Testing
Let's now discuss the practical considerations essential when testing AI systems. These considerations help ensure that AI technologies are developed and maintained with high accuracy, reliability, and ethical standards.
Human involvement remains crucial in the loop of AI system development, particularly in data gathering and dataset improvement processes. Humans can provide essential insights into the nuances of data that AI might overlook, such as cultural contexts or implicit meanings. They can also help refine datasets by identifying and correcting errors that automated systems may propagate.
Challenges and Metrics for AI Data Sourcing
Sourcing high-quality data for AI training involves several challenges:
Data Variety: Ensuring that the data covers many scenarios, including rare edge cases, but critical for comprehensive training.
Data Veracity: Maintaining the accuracy and authenticity of data.
Data Volume: Collecting sufficiently large datasets to train robust models.
Metrics for evaluating the effectiveness of AI data sourcing include data accuracy, diversity scores, and the frequency of data updates.
The Evolving Role of QA Specialists
The role of Quality Assurance (QA) specialists is rapidly evolving in the context of AI:
From Manual to Automated: QA roles are shifting from manual testing to overseeing automated testing systems that can handle the complexity and scale of AI applications.
Specialization in AI Ethics and Bias: QA specialists are increasingly required to know AI ethics, focusing on identifying and mitigating biases in AI models.
Continuous Learning and Adaptation: As AI systems continuously learn and adapt, QA specialists must also continuously update their testing strategies and tools to keep pace with the changes in AI behavior.
# Example of a QA process in AI testing
def evaluate_model_quality(model, test_data, metrics):
results = model.predict(test_data)
quality_scores = {metric: metrics[metric](test_data, results) for metric in metrics}
return quality_scores
# Assuming 'model', 'test_data', and 'metrics' are predefined
quality_results = evaluate_model_quality(model, test_data, {'accuracy': accuracy_score, 'f1_score': f1_score})
print("Quality Evaluation Results:", quality_results)
This example illustrates how a QA specialist might use automated tools to evaluate the quality of an AI model, applying various metrics to ensure it meets the required standards.
Understanding these practical considerations is vital for effectively testing and maintaining AI systems, ensuring they function as intended and adhere to ethical guidelines. Next, we will explore the future of AI testing, looking at how emerging trends and technologies are shaping the field.
The Future of AI Testing
Let's explore the future of AI testing, focusing on how emerging technologies and methodologies are expected to enhance and transform the testing landscape. This will give us insights into the continuous evolution of AI and its implications for testing practices.
Predictions on the Integration of AI in Software Testing
The integration of AI into software testing is set to revolutionize the field by automating complex tasks and providing deeper insights into software behavior. AI can analyze vast amounts of data to identify patterns and predict potential issues before they become apparent, significantly improving the efficiency and effectiveness of testing processes.
Improving the Testing Cycle
AI's capability for continuous learning makes it ideal for constant testing, where systems are constantly evaluated in real-time. This approach allows for immediate feedback and rapid iteration, which is crucial in fast-paced development environments. AI can automate the testing cycle's repetitive parts, freeing human testers to focus on more strategic activities.
Example: AI tools can monitor the performance of live systems and automatically trigger tests in response to changes or newly detected conditions. This ensures the system is continually validated and reduces the time to detect and resolve issues.
Emerging Trends and Technologies in AI and ML Testing
Several trends and technologies are shaping the future of AI testing:
Increased Use of Simulation and Virtual Testing Environments: Advanced simulation tools allow testers to create detailed, realistic environments to test AI behaviors without the risks and costs associated with real-world testing.
Growth of Predictive Analytics in Testing: AI-driven predictive analytics can forecast potential failure points and suggest optimizations, making testing proactive rather than reactive.
Expansion of Testing Capabilities with Generative AI: Generative AI models can create new test cases and data scenarios, expanding test coverage beyond what human testers might conceive.
# Example code for using AI to generate test scenarios
from some_ai_testing_library import AITestGenerator
ai_test_gen = AITestGenerator()
test_scenarios = ai_test_gen.generate_scenarios('path/to/model')
for scenario in test_scenarios:
result = run_test(scenario)
if not result.passed:
print(f"Failed scenario: {scenario.description}")
This hypothetical example illustrates how generative AI could automatically produce test scenarios, assessing a model across a broader range of conditions than manually predefined tests.
Conclusion
The future of AI testing is rich with potential, promising to make testing more proactive, efficient, and comprehensive. As AI technologies evolve, so will the methods and tools used to ensure they are safe, reliable, and effective. This concludes our exploration of AI testing. If you have any questions or need further information on any aspects discussed, feel free to ask!
RagaAI has developed a comprehensive AI testing platform that offers over 300 tests to automatically detect issues, diagnose and fix them instantly. The platform supports various data types such as large language models (LLMs), images, videos, 3D, and audio. Embrace the future of AI with Raga AI—where innovation meets integrity.
Take the next step in your AI journey. Visit Raga AI's website today to learn more about how our synthetic data platforms can revolutionize your applications.
Testing AI (Artificial Intelligence) and ML (Machine Learning) applications involves a series of processes to ensure these systems perform as expected. This includes validating their functionality, accuracy, and reliability under various conditions.
The importance of testing cannot be overstated—it ensures that AI systems are safe, effective, and free from biases that could lead to unfair or harmful outcomes.
Traditional software testing typically involves checking code for bugs and ensuring it meets specified requirements.
However, testing AI systems is inherently more complex due to their probabilistic nature. Unlike traditional software that behaves predictably, AI systems can produce different outputs with the same input, depending on their learning and adaptive algorithms. This unpredictability requires a fundamentally different approach to testing.
Challenges Unique to AI and ML Testing
AI and ML systems pose unique testing challenges due to their reliance on data quality, the complexity of their models, and the need for interpretability. Ensuring these systems function correctly across all possible scenarios can be daunting because they continuously learn and evolve based on new data, potentially leading to changes in their behaviour over time.
As AI technologies continue to permeate various sectors—from healthcare and finance to autonomous driving and customer service—it becomes crucial to adapt testing strategies to address the specific risks associated with AI decision-making. Effective testing strategies help mitigate risks, ensuring that AI systems perform reliably and ethically in real-world applications.
Best Practices for Testing AI/ML Systems
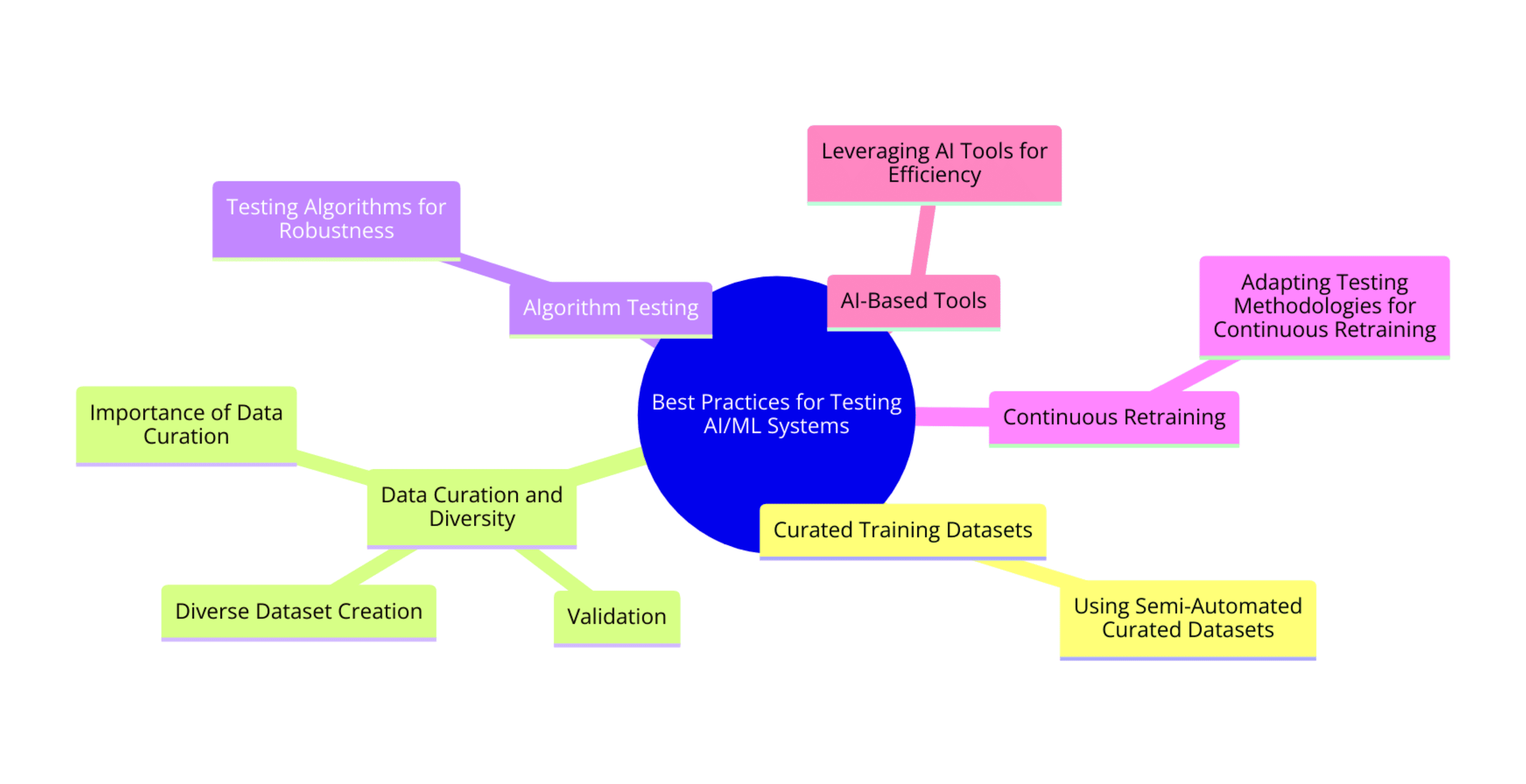
Let's discuss some best practices for effectively testing AI and ML systems. These strategies ensure the systems are functional, efficient, fair, and transparent.
Using Semi-Automated Curated Training Datasets for Effective Testing
One of the foundational steps in testing AI systems is to ensure that the training datasets are well-curated and representative of real-world scenarios. Employing semi-automated tools to curate and verify the quality and diversity of these datasets helps minimise bias and improves the overall robustness of the models.
Importance of Data Curation, Validation, and Diverse Dataset Creation
Data curation and validation are critical to preparing datasets that accurately reflect the complexity of the tasks the AI is designed to perform. This involves removing erroneous data, ensuring data is correctly labelled, and creating datasets that include diverse scenarios and demographics to prevent bias in model training.
Algorithm Testing
Testing AI algorithms involves more than just assessing performance metrics like accuracy or speed. It also includes evaluating the security aspects to prevent adversarial attacks and ensuring that the algorithms integrate well with other software components or systems, maintaining functionality across the technology stack.
# Example code for performance testing of an AI model
from sklearn.metrics import accuracy_score
def test_model_performance(model, features, labels):
predictions = model.predict(features)
accuracy = accuracy_score(labels, predictions)
return accuracy
# Assuming 'model', 'test_features', and 'test_labels' are predefined
model_accuracy = test_model_performance(model, test_features, test_labels)
print(f"Model Accuracy: {model_accuracy}")
Adapting Testing Methodologies for Sustained Testing Due to Continuous Model Retraining
As AI models often undergo continuous retraining to improve their performance or adapt to new data, testing methodologies must also be adapted to accommodate these changes. This includes regular re-evaluation of models to ensure that updates do not degrade the system's performance or introduce new biases.
Leveraging AI-Based Tools for More Efficient Testing Processes
AI-based tools can automate and enhance the testing process. They can simulate various conditions and scenarios faster than manual testing, providing comprehensive insights into model behaviour and potential weaknesses.
# Example code for using an AI-based tool to automate test scenario generation
# Assume 'generate_test_scenarios' is a function provided by an AI-based testing tool
test_scenarios = generate_test_scenarios(model, num_scenarios=100)
results = [test_model(model, scenario) for scenario in test_scenarios]
Employing these best practices in testing AI and ML systems ensures their reliability and efficiency and upholds ethical standards by actively preventing biases and ensuring transparency. Next, we will explore the tools and technologies available for testing AI applications, providing you with practical resources to implement these best practices.
Tools and Technologies for AI Application Testing
Let's explore the various tools and technologies available for testing AI applications. These specialised resources can significantly enhance the efficiency and effectiveness of testing processes.
TensorFlow Extended (TFX)
TensorFlow Extended (TFX) is another powerful tool that offers a suite of libraries and capabilities designed to facilitate the production and testing of ML models. TFX provides components for validating data and model quality, critical for maintaining robust AI systems.
Open Source Tools
There are several open-source tools and libraries that can be used for AI-driven testing:
TensorFlow: A free and open-source software library for machine learning and artificial intelligence. It can be used across a range of applications, including testing.
Selenium: A popular open-source web automation framework for browser automation. While not AI-specific, Selenium provides a foundation for building AI-powered testing tools.
Appium: An open-source test automation framework for mobile apps. It uses the WebDriver protocol to automate mobile apps on iOS and Android platforms.
Robot Framework: A generic test automation framework for acceptance testing and acceptance test-driven development (ATDD). It uses a keyword-driven testing approach and supports various programming languages.
Watir (Web Application Testing in Ruby): Provides open-source Ruby libraries for automating web browsers. It uses Selenium under the hood and supports multiple browsers.
JUnit: A unit testing framework for Java. While not AI-focused, it provides a foundation for building automated tests and can be integrated with AI libraries.
Robotium: An open-source Android UI testing framework that supports testing of native and hybrid Android apps.
These open-source tools and libraries offer a solid starting point for incorporating AI into testing workflows. However, it's important to note that building a fully AI-driven testing solution requires significant effort and expertise. Integrating these tools with AI frameworks like TensorFlow can enable advanced capabilities such as visual testing, self-healing tests, and predictive analytics.
Benefits of Using AI-powered Tools for Smarter, Faster Test Creation and Maintenance
The use of AI-powered tools in testing offers several benefits:
Efficiency: AI tools can quickly generate test cases and scenarios that cover a broad range of conditions, significantly reducing the manual effort required.
Accuracy: These tools help ensure high test accuracy by automatically detecting and adjusting for changes in the application or data that might be missed manually.
Maintenance: AI tools can adapt to changes in the application, automatically updating tests to remain relevant as the application evolves.
These tools and technologies provide critical support in effectively testing AI applications, ensuring that they function as intended and adhere to high standards of quality and ethics. Next, we will explore the different tests that can be conducted on AI-powered applications to ensure comprehensive coverage.
Types of Testing for AI-Powered Applications
Let's explore the diverse types of testing specifically tailored for AI-powered applications, ensuring these systems function correctly across various scenarios and meet all necessary performance benchmarks.
AI-powered applications require thorough testing to ensure they meet functional requirements and perform optimally under different conditions:
Functional Testing checks if the system does what it’s supposed to do according to its requirements.
Usability Testing assesses how easy and intuitive the application is for end-users.
Performance Testing ensures the application performs well under expected workload scenarios.
Integration Testing verifies that the AI integrates seamlessly with other system components.
API Testing confirms that the application programming interfaces work correctly across different platforms.
Security Testing is crucial to ensure the AI system is secure from external threats and data breaches.
Unique Types of Testing
Specialised testing types also play a critical role in AI validation:
Black Box Testing: Testing without prior knowledge of the system architecture or code, focusing solely on the outputs given specific inputs.
White Box Testing involves examining an application's internal structures or workings. It is often used in algorithm testing.
Metamorphic Testing: Involves testing cases where there are no known outputs. It’s beneficial for AI systems where defining test cases is inherently complex.
Non-Functional Testing: Assesses aspects not directly related to specific behaviours or functions, such as scalability and reliability.
Importance of Other Strategies
Model Backtesting: Essential for applications like financial forecasting, where historical data is used to test predictive models.
Performance Testing: Checks the model’s response times and accuracy under various computational loads.
Dual Coding/Algorithm Ensemble Strategies: Using multiple algorithms or models to validate each other’s outputs can enhance reliability and accuracy.
# Example of performance testing using Python
import time
def test_model_speed(model, data):
start_time = time.time()
predictions = model.predict(data)
end_time = time.time()
print(f"Model processed {len(data)} records in {end_time - start_time} seconds.")
# Assuming 'model' and 'data' are predefined
test_model_speed(model, data)
This simple example measures how long it takes to process a data set, providing insight into the model's performance under operational conditions.
Testing AI-powered applications with these comprehensive strategies ensures they are robust, reliable, and ready for real-world deployment. As AI continues to evolve, so too will the approaches to testing. Next, we'll discuss the crucial role of addressing biases and ensuring fairness in AI systems.
Addressing Bias and Fairness in AI Systems
Let's address bias and fairness in AI systems, which are critical to ensuring that AI applications are equitable and do not perpetuate existing disparities.
Strategies to Mitigate Data Skewness, Prediction Bias, and Relational Bias
Bias in AI systems can stem from various sources, particularly from the data used to train these systems. Implementing strategies to mitigate such biases is crucial:
Data Skewness: Ensuring that the training data is representative of the real-world scenarios the AI will encounter can help minimise skewness. This involves including diverse data samples that cover various demographics and conditions.
Prediction Bias: Regularly testing the AI's predictions across different groups and adjusting the model to ensure all groups are treated fairly.
Relational Bias involves analysing the relationships and correlations learned by the AI to ensure they are valid and not based on biased assumptions.
The Role of Fairness Testing Tools in Identifying and Reducing Biases
Fairness testing tools are essential for systematically identifying and mitigating biases in AI systems. These tools can analyze how AI models make decisions and whether certain groups are unfairly treated based on sensitive attributes like race, gender, or age.
Highlighting the Importance of Diverse Testing Scenarios to Ensure AI Fairness
Creating diverse testing scenarios that simulate real-world situations is crucial for evaluating AI fairness. This includes:
Testing AI systems across a broad range of demographic groups.
Synthetic data simulates rare conditions not well-represented in the training data.
Employing adversarial testing to challenge the AI with complex or edge cases.
# Example of using AI Fairness 360 to check for bias in a dataset
from aif360.datasets import BinaryLabelDataset
from aif360.metrics import BinaryLabelDatasetMetric
def assess_dataset_bias(dataset, privileged_groups, unprivileged_groups):
binary_dataset = BinaryLabelDataset(df=dataset, label_names=['label'], protected_attribute_names=['protected_attribute'])
metric = BinaryLabelDatasetMetric(binary_dataset,
unprivileged_groups=unprivileged_groups,
privileged_groups=privileged_groups)
print("Disparate Impact: {:.2f}".format(metric.disparate_impact()))
print("Statistical Parity Difference: {:.2f}".format(metric.statistical_parity_difference()))
# Assuming 'dataset', 'privileged_groups', and 'unprivileged_groups' are predefined
assess_dataset_bias(dataset, privileged_groups, unprivileged_groups)
This snippet demonstrates how to use tools like AI Fairness 360 to assess potential biases in datasets, ensuring that AI models are tested for fairness across all groups.
Addressing bias and ensuring fairness in AI systems are ongoing challenges that require continuous attention and strategy adaptation. These efforts are crucial for building trust and ensuring the ethical deployment of AI technologies. Next, we'll explore practical considerations in AI system testing.
Practical Considerations in AI System Testing
Let's now discuss the practical considerations essential when testing AI systems. These considerations help ensure that AI technologies are developed and maintained with high accuracy, reliability, and ethical standards.
Human involvement remains crucial in the loop of AI system development, particularly in data gathering and dataset improvement processes. Humans can provide essential insights into the nuances of data that AI might overlook, such as cultural contexts or implicit meanings. They can also help refine datasets by identifying and correcting errors that automated systems may propagate.
Challenges and Metrics for AI Data Sourcing
Sourcing high-quality data for AI training involves several challenges:
Data Variety: Ensuring that the data covers many scenarios, including rare edge cases, but critical for comprehensive training.
Data Veracity: Maintaining the accuracy and authenticity of data.
Data Volume: Collecting sufficiently large datasets to train robust models.
Metrics for evaluating the effectiveness of AI data sourcing include data accuracy, diversity scores, and the frequency of data updates.
The Evolving Role of QA Specialists
The role of Quality Assurance (QA) specialists is rapidly evolving in the context of AI:
From Manual to Automated: QA roles are shifting from manual testing to overseeing automated testing systems that can handle the complexity and scale of AI applications.
Specialization in AI Ethics and Bias: QA specialists are increasingly required to know AI ethics, focusing on identifying and mitigating biases in AI models.
Continuous Learning and Adaptation: As AI systems continuously learn and adapt, QA specialists must also continuously update their testing strategies and tools to keep pace with the changes in AI behavior.
# Example of a QA process in AI testing
def evaluate_model_quality(model, test_data, metrics):
results = model.predict(test_data)
quality_scores = {metric: metrics[metric](test_data, results) for metric in metrics}
return quality_scores
# Assuming 'model', 'test_data', and 'metrics' are predefined
quality_results = evaluate_model_quality(model, test_data, {'accuracy': accuracy_score, 'f1_score': f1_score})
print("Quality Evaluation Results:", quality_results)
This example illustrates how a QA specialist might use automated tools to evaluate the quality of an AI model, applying various metrics to ensure it meets the required standards.
Understanding these practical considerations is vital for effectively testing and maintaining AI systems, ensuring they function as intended and adhere to ethical guidelines. Next, we will explore the future of AI testing, looking at how emerging trends and technologies are shaping the field.
The Future of AI Testing
Let's explore the future of AI testing, focusing on how emerging technologies and methodologies are expected to enhance and transform the testing landscape. This will give us insights into the continuous evolution of AI and its implications for testing practices.
Predictions on the Integration of AI in Software Testing
The integration of AI into software testing is set to revolutionize the field by automating complex tasks and providing deeper insights into software behavior. AI can analyze vast amounts of data to identify patterns and predict potential issues before they become apparent, significantly improving the efficiency and effectiveness of testing processes.
Improving the Testing Cycle
AI's capability for continuous learning makes it ideal for constant testing, where systems are constantly evaluated in real-time. This approach allows for immediate feedback and rapid iteration, which is crucial in fast-paced development environments. AI can automate the testing cycle's repetitive parts, freeing human testers to focus on more strategic activities.
Example: AI tools can monitor the performance of live systems and automatically trigger tests in response to changes or newly detected conditions. This ensures the system is continually validated and reduces the time to detect and resolve issues.
Emerging Trends and Technologies in AI and ML Testing
Several trends and technologies are shaping the future of AI testing:
Increased Use of Simulation and Virtual Testing Environments: Advanced simulation tools allow testers to create detailed, realistic environments to test AI behaviors without the risks and costs associated with real-world testing.
Growth of Predictive Analytics in Testing: AI-driven predictive analytics can forecast potential failure points and suggest optimizations, making testing proactive rather than reactive.
Expansion of Testing Capabilities with Generative AI: Generative AI models can create new test cases and data scenarios, expanding test coverage beyond what human testers might conceive.
# Example code for using AI to generate test scenarios
from some_ai_testing_library import AITestGenerator
ai_test_gen = AITestGenerator()
test_scenarios = ai_test_gen.generate_scenarios('path/to/model')
for scenario in test_scenarios:
result = run_test(scenario)
if not result.passed:
print(f"Failed scenario: {scenario.description}")
This hypothetical example illustrates how generative AI could automatically produce test scenarios, assessing a model across a broader range of conditions than manually predefined tests.
Conclusion
The future of AI testing is rich with potential, promising to make testing more proactive, efficient, and comprehensive. As AI technologies evolve, so will the methods and tools used to ensure they are safe, reliable, and effective. This concludes our exploration of AI testing. If you have any questions or need further information on any aspects discussed, feel free to ask!
RagaAI has developed a comprehensive AI testing platform that offers over 300 tests to automatically detect issues, diagnose and fix them instantly. The platform supports various data types such as large language models (LLMs), images, videos, 3D, and audio. Embrace the future of AI with Raga AI—where innovation meets integrity.
Take the next step in your AI journey. Visit Raga AI's website today to learn more about how our synthetic data platforms can revolutionize your applications.
Testing AI (Artificial Intelligence) and ML (Machine Learning) applications involves a series of processes to ensure these systems perform as expected. This includes validating their functionality, accuracy, and reliability under various conditions.
The importance of testing cannot be overstated—it ensures that AI systems are safe, effective, and free from biases that could lead to unfair or harmful outcomes.
Traditional software testing typically involves checking code for bugs and ensuring it meets specified requirements.
However, testing AI systems is inherently more complex due to their probabilistic nature. Unlike traditional software that behaves predictably, AI systems can produce different outputs with the same input, depending on their learning and adaptive algorithms. This unpredictability requires a fundamentally different approach to testing.
Challenges Unique to AI and ML Testing
AI and ML systems pose unique testing challenges due to their reliance on data quality, the complexity of their models, and the need for interpretability. Ensuring these systems function correctly across all possible scenarios can be daunting because they continuously learn and evolve based on new data, potentially leading to changes in their behaviour over time.
As AI technologies continue to permeate various sectors—from healthcare and finance to autonomous driving and customer service—it becomes crucial to adapt testing strategies to address the specific risks associated with AI decision-making. Effective testing strategies help mitigate risks, ensuring that AI systems perform reliably and ethically in real-world applications.
Best Practices for Testing AI/ML Systems
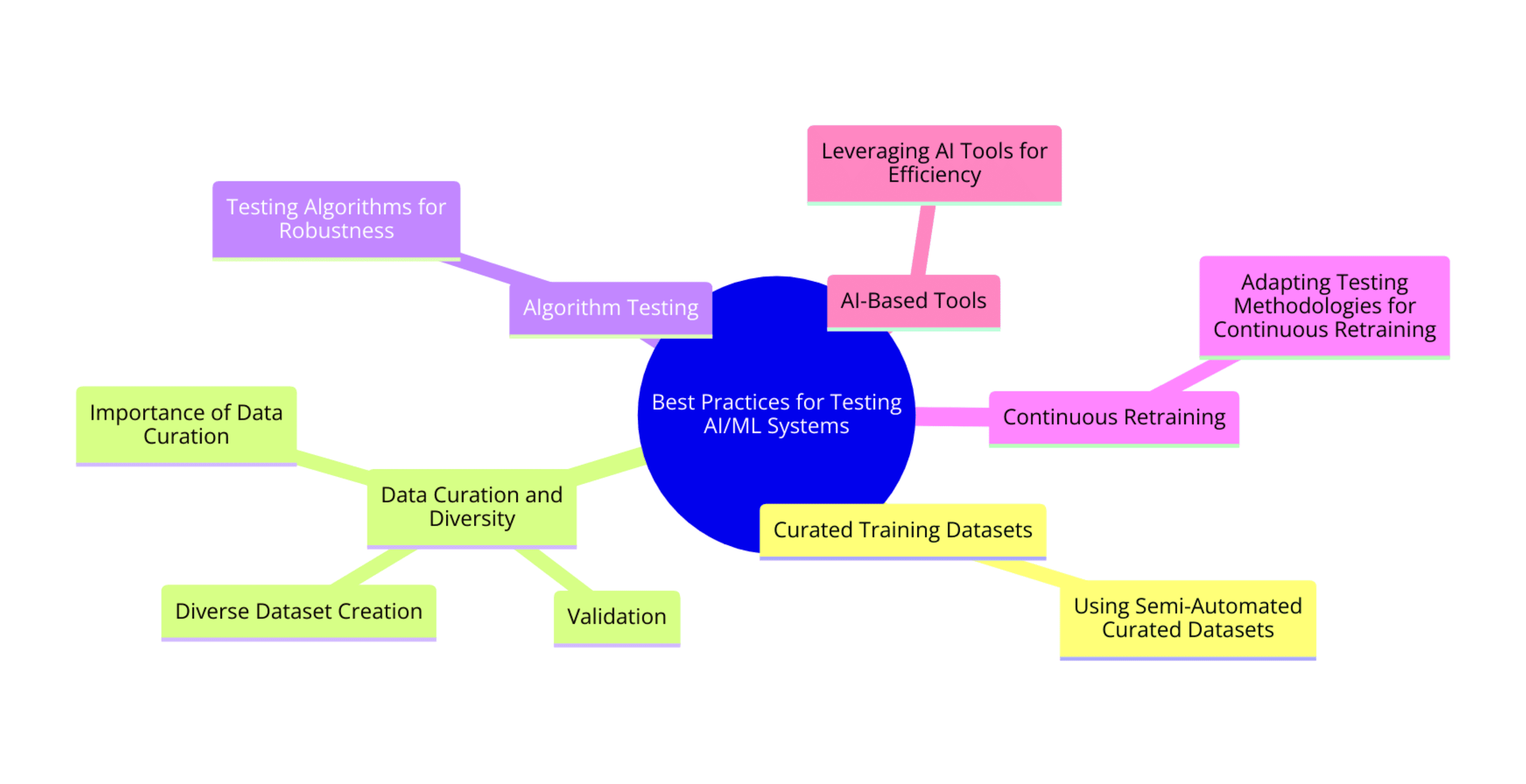
Let's discuss some best practices for effectively testing AI and ML systems. These strategies ensure the systems are functional, efficient, fair, and transparent.
Using Semi-Automated Curated Training Datasets for Effective Testing
One of the foundational steps in testing AI systems is to ensure that the training datasets are well-curated and representative of real-world scenarios. Employing semi-automated tools to curate and verify the quality and diversity of these datasets helps minimise bias and improves the overall robustness of the models.
Importance of Data Curation, Validation, and Diverse Dataset Creation
Data curation and validation are critical to preparing datasets that accurately reflect the complexity of the tasks the AI is designed to perform. This involves removing erroneous data, ensuring data is correctly labelled, and creating datasets that include diverse scenarios and demographics to prevent bias in model training.
Algorithm Testing
Testing AI algorithms involves more than just assessing performance metrics like accuracy or speed. It also includes evaluating the security aspects to prevent adversarial attacks and ensuring that the algorithms integrate well with other software components or systems, maintaining functionality across the technology stack.
# Example code for performance testing of an AI model
from sklearn.metrics import accuracy_score
def test_model_performance(model, features, labels):
predictions = model.predict(features)
accuracy = accuracy_score(labels, predictions)
return accuracy
# Assuming 'model', 'test_features', and 'test_labels' are predefined
model_accuracy = test_model_performance(model, test_features, test_labels)
print(f"Model Accuracy: {model_accuracy}")
Adapting Testing Methodologies for Sustained Testing Due to Continuous Model Retraining
As AI models often undergo continuous retraining to improve their performance or adapt to new data, testing methodologies must also be adapted to accommodate these changes. This includes regular re-evaluation of models to ensure that updates do not degrade the system's performance or introduce new biases.
Leveraging AI-Based Tools for More Efficient Testing Processes
AI-based tools can automate and enhance the testing process. They can simulate various conditions and scenarios faster than manual testing, providing comprehensive insights into model behaviour and potential weaknesses.
# Example code for using an AI-based tool to automate test scenario generation
# Assume 'generate_test_scenarios' is a function provided by an AI-based testing tool
test_scenarios = generate_test_scenarios(model, num_scenarios=100)
results = [test_model(model, scenario) for scenario in test_scenarios]
Employing these best practices in testing AI and ML systems ensures their reliability and efficiency and upholds ethical standards by actively preventing biases and ensuring transparency. Next, we will explore the tools and technologies available for testing AI applications, providing you with practical resources to implement these best practices.
Tools and Technologies for AI Application Testing
Let's explore the various tools and technologies available for testing AI applications. These specialised resources can significantly enhance the efficiency and effectiveness of testing processes.
TensorFlow Extended (TFX)
TensorFlow Extended (TFX) is another powerful tool that offers a suite of libraries and capabilities designed to facilitate the production and testing of ML models. TFX provides components for validating data and model quality, critical for maintaining robust AI systems.
Open Source Tools
There are several open-source tools and libraries that can be used for AI-driven testing:
TensorFlow: A free and open-source software library for machine learning and artificial intelligence. It can be used across a range of applications, including testing.
Selenium: A popular open-source web automation framework for browser automation. While not AI-specific, Selenium provides a foundation for building AI-powered testing tools.
Appium: An open-source test automation framework for mobile apps. It uses the WebDriver protocol to automate mobile apps on iOS and Android platforms.
Robot Framework: A generic test automation framework for acceptance testing and acceptance test-driven development (ATDD). It uses a keyword-driven testing approach and supports various programming languages.
Watir (Web Application Testing in Ruby): Provides open-source Ruby libraries for automating web browsers. It uses Selenium under the hood and supports multiple browsers.
JUnit: A unit testing framework for Java. While not AI-focused, it provides a foundation for building automated tests and can be integrated with AI libraries.
Robotium: An open-source Android UI testing framework that supports testing of native and hybrid Android apps.
These open-source tools and libraries offer a solid starting point for incorporating AI into testing workflows. However, it's important to note that building a fully AI-driven testing solution requires significant effort and expertise. Integrating these tools with AI frameworks like TensorFlow can enable advanced capabilities such as visual testing, self-healing tests, and predictive analytics.
Benefits of Using AI-powered Tools for Smarter, Faster Test Creation and Maintenance
The use of AI-powered tools in testing offers several benefits:
Efficiency: AI tools can quickly generate test cases and scenarios that cover a broad range of conditions, significantly reducing the manual effort required.
Accuracy: These tools help ensure high test accuracy by automatically detecting and adjusting for changes in the application or data that might be missed manually.
Maintenance: AI tools can adapt to changes in the application, automatically updating tests to remain relevant as the application evolves.
These tools and technologies provide critical support in effectively testing AI applications, ensuring that they function as intended and adhere to high standards of quality and ethics. Next, we will explore the different tests that can be conducted on AI-powered applications to ensure comprehensive coverage.
Types of Testing for AI-Powered Applications
Let's explore the diverse types of testing specifically tailored for AI-powered applications, ensuring these systems function correctly across various scenarios and meet all necessary performance benchmarks.
AI-powered applications require thorough testing to ensure they meet functional requirements and perform optimally under different conditions:
Functional Testing checks if the system does what it’s supposed to do according to its requirements.
Usability Testing assesses how easy and intuitive the application is for end-users.
Performance Testing ensures the application performs well under expected workload scenarios.
Integration Testing verifies that the AI integrates seamlessly with other system components.
API Testing confirms that the application programming interfaces work correctly across different platforms.
Security Testing is crucial to ensure the AI system is secure from external threats and data breaches.
Unique Types of Testing
Specialised testing types also play a critical role in AI validation:
Black Box Testing: Testing without prior knowledge of the system architecture or code, focusing solely on the outputs given specific inputs.
White Box Testing involves examining an application's internal structures or workings. It is often used in algorithm testing.
Metamorphic Testing: Involves testing cases where there are no known outputs. It’s beneficial for AI systems where defining test cases is inherently complex.
Non-Functional Testing: Assesses aspects not directly related to specific behaviours or functions, such as scalability and reliability.
Importance of Other Strategies
Model Backtesting: Essential for applications like financial forecasting, where historical data is used to test predictive models.
Performance Testing: Checks the model’s response times and accuracy under various computational loads.
Dual Coding/Algorithm Ensemble Strategies: Using multiple algorithms or models to validate each other’s outputs can enhance reliability and accuracy.
# Example of performance testing using Python
import time
def test_model_speed(model, data):
start_time = time.time()
predictions = model.predict(data)
end_time = time.time()
print(f"Model processed {len(data)} records in {end_time - start_time} seconds.")
# Assuming 'model' and 'data' are predefined
test_model_speed(model, data)
This simple example measures how long it takes to process a data set, providing insight into the model's performance under operational conditions.
Testing AI-powered applications with these comprehensive strategies ensures they are robust, reliable, and ready for real-world deployment. As AI continues to evolve, so too will the approaches to testing. Next, we'll discuss the crucial role of addressing biases and ensuring fairness in AI systems.
Addressing Bias and Fairness in AI Systems
Let's address bias and fairness in AI systems, which are critical to ensuring that AI applications are equitable and do not perpetuate existing disparities.
Strategies to Mitigate Data Skewness, Prediction Bias, and Relational Bias
Bias in AI systems can stem from various sources, particularly from the data used to train these systems. Implementing strategies to mitigate such biases is crucial:
Data Skewness: Ensuring that the training data is representative of the real-world scenarios the AI will encounter can help minimise skewness. This involves including diverse data samples that cover various demographics and conditions.
Prediction Bias: Regularly testing the AI's predictions across different groups and adjusting the model to ensure all groups are treated fairly.
Relational Bias involves analysing the relationships and correlations learned by the AI to ensure they are valid and not based on biased assumptions.
The Role of Fairness Testing Tools in Identifying and Reducing Biases
Fairness testing tools are essential for systematically identifying and mitigating biases in AI systems. These tools can analyze how AI models make decisions and whether certain groups are unfairly treated based on sensitive attributes like race, gender, or age.
Highlighting the Importance of Diverse Testing Scenarios to Ensure AI Fairness
Creating diverse testing scenarios that simulate real-world situations is crucial for evaluating AI fairness. This includes:
Testing AI systems across a broad range of demographic groups.
Synthetic data simulates rare conditions not well-represented in the training data.
Employing adversarial testing to challenge the AI with complex or edge cases.
# Example of using AI Fairness 360 to check for bias in a dataset
from aif360.datasets import BinaryLabelDataset
from aif360.metrics import BinaryLabelDatasetMetric
def assess_dataset_bias(dataset, privileged_groups, unprivileged_groups):
binary_dataset = BinaryLabelDataset(df=dataset, label_names=['label'], protected_attribute_names=['protected_attribute'])
metric = BinaryLabelDatasetMetric(binary_dataset,
unprivileged_groups=unprivileged_groups,
privileged_groups=privileged_groups)
print("Disparate Impact: {:.2f}".format(metric.disparate_impact()))
print("Statistical Parity Difference: {:.2f}".format(metric.statistical_parity_difference()))
# Assuming 'dataset', 'privileged_groups', and 'unprivileged_groups' are predefined
assess_dataset_bias(dataset, privileged_groups, unprivileged_groups)
This snippet demonstrates how to use tools like AI Fairness 360 to assess potential biases in datasets, ensuring that AI models are tested for fairness across all groups.
Addressing bias and ensuring fairness in AI systems are ongoing challenges that require continuous attention and strategy adaptation. These efforts are crucial for building trust and ensuring the ethical deployment of AI technologies. Next, we'll explore practical considerations in AI system testing.
Practical Considerations in AI System Testing
Let's now discuss the practical considerations essential when testing AI systems. These considerations help ensure that AI technologies are developed and maintained with high accuracy, reliability, and ethical standards.
Human involvement remains crucial in the loop of AI system development, particularly in data gathering and dataset improvement processes. Humans can provide essential insights into the nuances of data that AI might overlook, such as cultural contexts or implicit meanings. They can also help refine datasets by identifying and correcting errors that automated systems may propagate.
Challenges and Metrics for AI Data Sourcing
Sourcing high-quality data for AI training involves several challenges:
Data Variety: Ensuring that the data covers many scenarios, including rare edge cases, but critical for comprehensive training.
Data Veracity: Maintaining the accuracy and authenticity of data.
Data Volume: Collecting sufficiently large datasets to train robust models.
Metrics for evaluating the effectiveness of AI data sourcing include data accuracy, diversity scores, and the frequency of data updates.
The Evolving Role of QA Specialists
The role of Quality Assurance (QA) specialists is rapidly evolving in the context of AI:
From Manual to Automated: QA roles are shifting from manual testing to overseeing automated testing systems that can handle the complexity and scale of AI applications.
Specialization in AI Ethics and Bias: QA specialists are increasingly required to know AI ethics, focusing on identifying and mitigating biases in AI models.
Continuous Learning and Adaptation: As AI systems continuously learn and adapt, QA specialists must also continuously update their testing strategies and tools to keep pace with the changes in AI behavior.
# Example of a QA process in AI testing
def evaluate_model_quality(model, test_data, metrics):
results = model.predict(test_data)
quality_scores = {metric: metrics[metric](test_data, results) for metric in metrics}
return quality_scores
# Assuming 'model', 'test_data', and 'metrics' are predefined
quality_results = evaluate_model_quality(model, test_data, {'accuracy': accuracy_score, 'f1_score': f1_score})
print("Quality Evaluation Results:", quality_results)
This example illustrates how a QA specialist might use automated tools to evaluate the quality of an AI model, applying various metrics to ensure it meets the required standards.
Understanding these practical considerations is vital for effectively testing and maintaining AI systems, ensuring they function as intended and adhere to ethical guidelines. Next, we will explore the future of AI testing, looking at how emerging trends and technologies are shaping the field.
The Future of AI Testing
Let's explore the future of AI testing, focusing on how emerging technologies and methodologies are expected to enhance and transform the testing landscape. This will give us insights into the continuous evolution of AI and its implications for testing practices.
Predictions on the Integration of AI in Software Testing
The integration of AI into software testing is set to revolutionize the field by automating complex tasks and providing deeper insights into software behavior. AI can analyze vast amounts of data to identify patterns and predict potential issues before they become apparent, significantly improving the efficiency and effectiveness of testing processes.
Improving the Testing Cycle
AI's capability for continuous learning makes it ideal for constant testing, where systems are constantly evaluated in real-time. This approach allows for immediate feedback and rapid iteration, which is crucial in fast-paced development environments. AI can automate the testing cycle's repetitive parts, freeing human testers to focus on more strategic activities.
Example: AI tools can monitor the performance of live systems and automatically trigger tests in response to changes or newly detected conditions. This ensures the system is continually validated and reduces the time to detect and resolve issues.
Emerging Trends and Technologies in AI and ML Testing
Several trends and technologies are shaping the future of AI testing:
Increased Use of Simulation and Virtual Testing Environments: Advanced simulation tools allow testers to create detailed, realistic environments to test AI behaviors without the risks and costs associated with real-world testing.
Growth of Predictive Analytics in Testing: AI-driven predictive analytics can forecast potential failure points and suggest optimizations, making testing proactive rather than reactive.
Expansion of Testing Capabilities with Generative AI: Generative AI models can create new test cases and data scenarios, expanding test coverage beyond what human testers might conceive.
# Example code for using AI to generate test scenarios
from some_ai_testing_library import AITestGenerator
ai_test_gen = AITestGenerator()
test_scenarios = ai_test_gen.generate_scenarios('path/to/model')
for scenario in test_scenarios:
result = run_test(scenario)
if not result.passed:
print(f"Failed scenario: {scenario.description}")
This hypothetical example illustrates how generative AI could automatically produce test scenarios, assessing a model across a broader range of conditions than manually predefined tests.
Conclusion
The future of AI testing is rich with potential, promising to make testing more proactive, efficient, and comprehensive. As AI technologies evolve, so will the methods and tools used to ensure they are safe, reliable, and effective. This concludes our exploration of AI testing. If you have any questions or need further information on any aspects discussed, feel free to ask!
RagaAI has developed a comprehensive AI testing platform that offers over 300 tests to automatically detect issues, diagnose and fix them instantly. The platform supports various data types such as large language models (LLMs), images, videos, 3D, and audio. Embrace the future of AI with Raga AI—where innovation meets integrity.
Take the next step in your AI journey. Visit Raga AI's website today to learn more about how our synthetic data platforms can revolutionize your applications.
Testing AI (Artificial Intelligence) and ML (Machine Learning) applications involves a series of processes to ensure these systems perform as expected. This includes validating their functionality, accuracy, and reliability under various conditions.
The importance of testing cannot be overstated—it ensures that AI systems are safe, effective, and free from biases that could lead to unfair or harmful outcomes.
Traditional software testing typically involves checking code for bugs and ensuring it meets specified requirements.
However, testing AI systems is inherently more complex due to their probabilistic nature. Unlike traditional software that behaves predictably, AI systems can produce different outputs with the same input, depending on their learning and adaptive algorithms. This unpredictability requires a fundamentally different approach to testing.
Challenges Unique to AI and ML Testing
AI and ML systems pose unique testing challenges due to their reliance on data quality, the complexity of their models, and the need for interpretability. Ensuring these systems function correctly across all possible scenarios can be daunting because they continuously learn and evolve based on new data, potentially leading to changes in their behaviour over time.
As AI technologies continue to permeate various sectors—from healthcare and finance to autonomous driving and customer service—it becomes crucial to adapt testing strategies to address the specific risks associated with AI decision-making. Effective testing strategies help mitigate risks, ensuring that AI systems perform reliably and ethically in real-world applications.
Best Practices for Testing AI/ML Systems
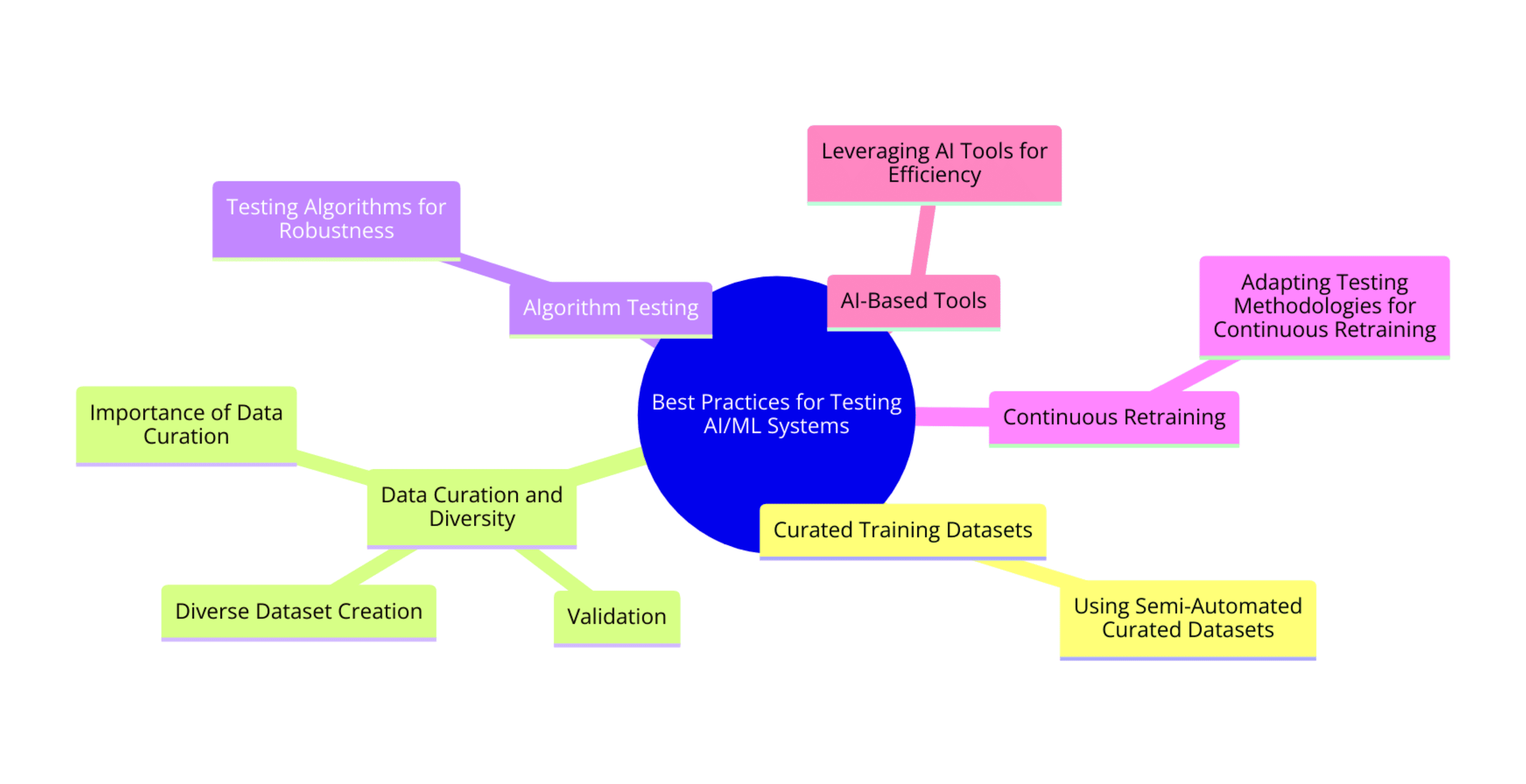
Let's discuss some best practices for effectively testing AI and ML systems. These strategies ensure the systems are functional, efficient, fair, and transparent.
Using Semi-Automated Curated Training Datasets for Effective Testing
One of the foundational steps in testing AI systems is to ensure that the training datasets are well-curated and representative of real-world scenarios. Employing semi-automated tools to curate and verify the quality and diversity of these datasets helps minimise bias and improves the overall robustness of the models.
Importance of Data Curation, Validation, and Diverse Dataset Creation
Data curation and validation are critical to preparing datasets that accurately reflect the complexity of the tasks the AI is designed to perform. This involves removing erroneous data, ensuring data is correctly labelled, and creating datasets that include diverse scenarios and demographics to prevent bias in model training.
Algorithm Testing
Testing AI algorithms involves more than just assessing performance metrics like accuracy or speed. It also includes evaluating the security aspects to prevent adversarial attacks and ensuring that the algorithms integrate well with other software components or systems, maintaining functionality across the technology stack.
# Example code for performance testing of an AI model
from sklearn.metrics import accuracy_score
def test_model_performance(model, features, labels):
predictions = model.predict(features)
accuracy = accuracy_score(labels, predictions)
return accuracy
# Assuming 'model', 'test_features', and 'test_labels' are predefined
model_accuracy = test_model_performance(model, test_features, test_labels)
print(f"Model Accuracy: {model_accuracy}")
Adapting Testing Methodologies for Sustained Testing Due to Continuous Model Retraining
As AI models often undergo continuous retraining to improve their performance or adapt to new data, testing methodologies must also be adapted to accommodate these changes. This includes regular re-evaluation of models to ensure that updates do not degrade the system's performance or introduce new biases.
Leveraging AI-Based Tools for More Efficient Testing Processes
AI-based tools can automate and enhance the testing process. They can simulate various conditions and scenarios faster than manual testing, providing comprehensive insights into model behaviour and potential weaknesses.
# Example code for using an AI-based tool to automate test scenario generation
# Assume 'generate_test_scenarios' is a function provided by an AI-based testing tool
test_scenarios = generate_test_scenarios(model, num_scenarios=100)
results = [test_model(model, scenario) for scenario in test_scenarios]
Employing these best practices in testing AI and ML systems ensures their reliability and efficiency and upholds ethical standards by actively preventing biases and ensuring transparency. Next, we will explore the tools and technologies available for testing AI applications, providing you with practical resources to implement these best practices.
Tools and Technologies for AI Application Testing
Let's explore the various tools and technologies available for testing AI applications. These specialised resources can significantly enhance the efficiency and effectiveness of testing processes.
TensorFlow Extended (TFX)
TensorFlow Extended (TFX) is another powerful tool that offers a suite of libraries and capabilities designed to facilitate the production and testing of ML models. TFX provides components for validating data and model quality, critical for maintaining robust AI systems.
Open Source Tools
There are several open-source tools and libraries that can be used for AI-driven testing:
TensorFlow: A free and open-source software library for machine learning and artificial intelligence. It can be used across a range of applications, including testing.
Selenium: A popular open-source web automation framework for browser automation. While not AI-specific, Selenium provides a foundation for building AI-powered testing tools.
Appium: An open-source test automation framework for mobile apps. It uses the WebDriver protocol to automate mobile apps on iOS and Android platforms.
Robot Framework: A generic test automation framework for acceptance testing and acceptance test-driven development (ATDD). It uses a keyword-driven testing approach and supports various programming languages.
Watir (Web Application Testing in Ruby): Provides open-source Ruby libraries for automating web browsers. It uses Selenium under the hood and supports multiple browsers.
JUnit: A unit testing framework for Java. While not AI-focused, it provides a foundation for building automated tests and can be integrated with AI libraries.
Robotium: An open-source Android UI testing framework that supports testing of native and hybrid Android apps.
These open-source tools and libraries offer a solid starting point for incorporating AI into testing workflows. However, it's important to note that building a fully AI-driven testing solution requires significant effort and expertise. Integrating these tools with AI frameworks like TensorFlow can enable advanced capabilities such as visual testing, self-healing tests, and predictive analytics.
Benefits of Using AI-powered Tools for Smarter, Faster Test Creation and Maintenance
The use of AI-powered tools in testing offers several benefits:
Efficiency: AI tools can quickly generate test cases and scenarios that cover a broad range of conditions, significantly reducing the manual effort required.
Accuracy: These tools help ensure high test accuracy by automatically detecting and adjusting for changes in the application or data that might be missed manually.
Maintenance: AI tools can adapt to changes in the application, automatically updating tests to remain relevant as the application evolves.
These tools and technologies provide critical support in effectively testing AI applications, ensuring that they function as intended and adhere to high standards of quality and ethics. Next, we will explore the different tests that can be conducted on AI-powered applications to ensure comprehensive coverage.
Types of Testing for AI-Powered Applications
Let's explore the diverse types of testing specifically tailored for AI-powered applications, ensuring these systems function correctly across various scenarios and meet all necessary performance benchmarks.
AI-powered applications require thorough testing to ensure they meet functional requirements and perform optimally under different conditions:
Functional Testing checks if the system does what it’s supposed to do according to its requirements.
Usability Testing assesses how easy and intuitive the application is for end-users.
Performance Testing ensures the application performs well under expected workload scenarios.
Integration Testing verifies that the AI integrates seamlessly with other system components.
API Testing confirms that the application programming interfaces work correctly across different platforms.
Security Testing is crucial to ensure the AI system is secure from external threats and data breaches.
Unique Types of Testing
Specialised testing types also play a critical role in AI validation:
Black Box Testing: Testing without prior knowledge of the system architecture or code, focusing solely on the outputs given specific inputs.
White Box Testing involves examining an application's internal structures or workings. It is often used in algorithm testing.
Metamorphic Testing: Involves testing cases where there are no known outputs. It’s beneficial for AI systems where defining test cases is inherently complex.
Non-Functional Testing: Assesses aspects not directly related to specific behaviours or functions, such as scalability and reliability.
Importance of Other Strategies
Model Backtesting: Essential for applications like financial forecasting, where historical data is used to test predictive models.
Performance Testing: Checks the model’s response times and accuracy under various computational loads.
Dual Coding/Algorithm Ensemble Strategies: Using multiple algorithms or models to validate each other’s outputs can enhance reliability and accuracy.
# Example of performance testing using Python
import time
def test_model_speed(model, data):
start_time = time.time()
predictions = model.predict(data)
end_time = time.time()
print(f"Model processed {len(data)} records in {end_time - start_time} seconds.")
# Assuming 'model' and 'data' are predefined
test_model_speed(model, data)
This simple example measures how long it takes to process a data set, providing insight into the model's performance under operational conditions.
Testing AI-powered applications with these comprehensive strategies ensures they are robust, reliable, and ready for real-world deployment. As AI continues to evolve, so too will the approaches to testing. Next, we'll discuss the crucial role of addressing biases and ensuring fairness in AI systems.
Addressing Bias and Fairness in AI Systems
Let's address bias and fairness in AI systems, which are critical to ensuring that AI applications are equitable and do not perpetuate existing disparities.
Strategies to Mitigate Data Skewness, Prediction Bias, and Relational Bias
Bias in AI systems can stem from various sources, particularly from the data used to train these systems. Implementing strategies to mitigate such biases is crucial:
Data Skewness: Ensuring that the training data is representative of the real-world scenarios the AI will encounter can help minimise skewness. This involves including diverse data samples that cover various demographics and conditions.
Prediction Bias: Regularly testing the AI's predictions across different groups and adjusting the model to ensure all groups are treated fairly.
Relational Bias involves analysing the relationships and correlations learned by the AI to ensure they are valid and not based on biased assumptions.
The Role of Fairness Testing Tools in Identifying and Reducing Biases
Fairness testing tools are essential for systematically identifying and mitigating biases in AI systems. These tools can analyze how AI models make decisions and whether certain groups are unfairly treated based on sensitive attributes like race, gender, or age.
Highlighting the Importance of Diverse Testing Scenarios to Ensure AI Fairness
Creating diverse testing scenarios that simulate real-world situations is crucial for evaluating AI fairness. This includes:
Testing AI systems across a broad range of demographic groups.
Synthetic data simulates rare conditions not well-represented in the training data.
Employing adversarial testing to challenge the AI with complex or edge cases.
# Example of using AI Fairness 360 to check for bias in a dataset
from aif360.datasets import BinaryLabelDataset
from aif360.metrics import BinaryLabelDatasetMetric
def assess_dataset_bias(dataset, privileged_groups, unprivileged_groups):
binary_dataset = BinaryLabelDataset(df=dataset, label_names=['label'], protected_attribute_names=['protected_attribute'])
metric = BinaryLabelDatasetMetric(binary_dataset,
unprivileged_groups=unprivileged_groups,
privileged_groups=privileged_groups)
print("Disparate Impact: {:.2f}".format(metric.disparate_impact()))
print("Statistical Parity Difference: {:.2f}".format(metric.statistical_parity_difference()))
# Assuming 'dataset', 'privileged_groups', and 'unprivileged_groups' are predefined
assess_dataset_bias(dataset, privileged_groups, unprivileged_groups)
This snippet demonstrates how to use tools like AI Fairness 360 to assess potential biases in datasets, ensuring that AI models are tested for fairness across all groups.
Addressing bias and ensuring fairness in AI systems are ongoing challenges that require continuous attention and strategy adaptation. These efforts are crucial for building trust and ensuring the ethical deployment of AI technologies. Next, we'll explore practical considerations in AI system testing.
Practical Considerations in AI System Testing
Let's now discuss the practical considerations essential when testing AI systems. These considerations help ensure that AI technologies are developed and maintained with high accuracy, reliability, and ethical standards.
Human involvement remains crucial in the loop of AI system development, particularly in data gathering and dataset improvement processes. Humans can provide essential insights into the nuances of data that AI might overlook, such as cultural contexts or implicit meanings. They can also help refine datasets by identifying and correcting errors that automated systems may propagate.
Challenges and Metrics for AI Data Sourcing
Sourcing high-quality data for AI training involves several challenges:
Data Variety: Ensuring that the data covers many scenarios, including rare edge cases, but critical for comprehensive training.
Data Veracity: Maintaining the accuracy and authenticity of data.
Data Volume: Collecting sufficiently large datasets to train robust models.
Metrics for evaluating the effectiveness of AI data sourcing include data accuracy, diversity scores, and the frequency of data updates.
The Evolving Role of QA Specialists
The role of Quality Assurance (QA) specialists is rapidly evolving in the context of AI:
From Manual to Automated: QA roles are shifting from manual testing to overseeing automated testing systems that can handle the complexity and scale of AI applications.
Specialization in AI Ethics and Bias: QA specialists are increasingly required to know AI ethics, focusing on identifying and mitigating biases in AI models.
Continuous Learning and Adaptation: As AI systems continuously learn and adapt, QA specialists must also continuously update their testing strategies and tools to keep pace with the changes in AI behavior.
# Example of a QA process in AI testing
def evaluate_model_quality(model, test_data, metrics):
results = model.predict(test_data)
quality_scores = {metric: metrics[metric](test_data, results) for metric in metrics}
return quality_scores
# Assuming 'model', 'test_data', and 'metrics' are predefined
quality_results = evaluate_model_quality(model, test_data, {'accuracy': accuracy_score, 'f1_score': f1_score})
print("Quality Evaluation Results:", quality_results)
This example illustrates how a QA specialist might use automated tools to evaluate the quality of an AI model, applying various metrics to ensure it meets the required standards.
Understanding these practical considerations is vital for effectively testing and maintaining AI systems, ensuring they function as intended and adhere to ethical guidelines. Next, we will explore the future of AI testing, looking at how emerging trends and technologies are shaping the field.
The Future of AI Testing
Let's explore the future of AI testing, focusing on how emerging technologies and methodologies are expected to enhance and transform the testing landscape. This will give us insights into the continuous evolution of AI and its implications for testing practices.
Predictions on the Integration of AI in Software Testing
The integration of AI into software testing is set to revolutionize the field by automating complex tasks and providing deeper insights into software behavior. AI can analyze vast amounts of data to identify patterns and predict potential issues before they become apparent, significantly improving the efficiency and effectiveness of testing processes.
Improving the Testing Cycle
AI's capability for continuous learning makes it ideal for constant testing, where systems are constantly evaluated in real-time. This approach allows for immediate feedback and rapid iteration, which is crucial in fast-paced development environments. AI can automate the testing cycle's repetitive parts, freeing human testers to focus on more strategic activities.
Example: AI tools can monitor the performance of live systems and automatically trigger tests in response to changes or newly detected conditions. This ensures the system is continually validated and reduces the time to detect and resolve issues.
Emerging Trends and Technologies in AI and ML Testing
Several trends and technologies are shaping the future of AI testing:
Increased Use of Simulation and Virtual Testing Environments: Advanced simulation tools allow testers to create detailed, realistic environments to test AI behaviors without the risks and costs associated with real-world testing.
Growth of Predictive Analytics in Testing: AI-driven predictive analytics can forecast potential failure points and suggest optimizations, making testing proactive rather than reactive.
Expansion of Testing Capabilities with Generative AI: Generative AI models can create new test cases and data scenarios, expanding test coverage beyond what human testers might conceive.
# Example code for using AI to generate test scenarios
from some_ai_testing_library import AITestGenerator
ai_test_gen = AITestGenerator()
test_scenarios = ai_test_gen.generate_scenarios('path/to/model')
for scenario in test_scenarios:
result = run_test(scenario)
if not result.passed:
print(f"Failed scenario: {scenario.description}")
This hypothetical example illustrates how generative AI could automatically produce test scenarios, assessing a model across a broader range of conditions than manually predefined tests.
Conclusion
The future of AI testing is rich with potential, promising to make testing more proactive, efficient, and comprehensive. As AI technologies evolve, so will the methods and tools used to ensure they are safe, reliable, and effective. This concludes our exploration of AI testing. If you have any questions or need further information on any aspects discussed, feel free to ask!
RagaAI has developed a comprehensive AI testing platform that offers over 300 tests to automatically detect issues, diagnose and fix them instantly. The platform supports various data types such as large language models (LLMs), images, videos, 3D, and audio. Embrace the future of AI with Raga AI—where innovation meets integrity.
Take the next step in your AI journey. Visit Raga AI's website today to learn more about how our synthetic data platforms can revolutionize your applications.
Testing AI (Artificial Intelligence) and ML (Machine Learning) applications involves a series of processes to ensure these systems perform as expected. This includes validating their functionality, accuracy, and reliability under various conditions.
The importance of testing cannot be overstated—it ensures that AI systems are safe, effective, and free from biases that could lead to unfair or harmful outcomes.
Traditional software testing typically involves checking code for bugs and ensuring it meets specified requirements.
However, testing AI systems is inherently more complex due to their probabilistic nature. Unlike traditional software that behaves predictably, AI systems can produce different outputs with the same input, depending on their learning and adaptive algorithms. This unpredictability requires a fundamentally different approach to testing.
Challenges Unique to AI and ML Testing
AI and ML systems pose unique testing challenges due to their reliance on data quality, the complexity of their models, and the need for interpretability. Ensuring these systems function correctly across all possible scenarios can be daunting because they continuously learn and evolve based on new data, potentially leading to changes in their behaviour over time.
As AI technologies continue to permeate various sectors—from healthcare and finance to autonomous driving and customer service—it becomes crucial to adapt testing strategies to address the specific risks associated with AI decision-making. Effective testing strategies help mitigate risks, ensuring that AI systems perform reliably and ethically in real-world applications.
Best Practices for Testing AI/ML Systems
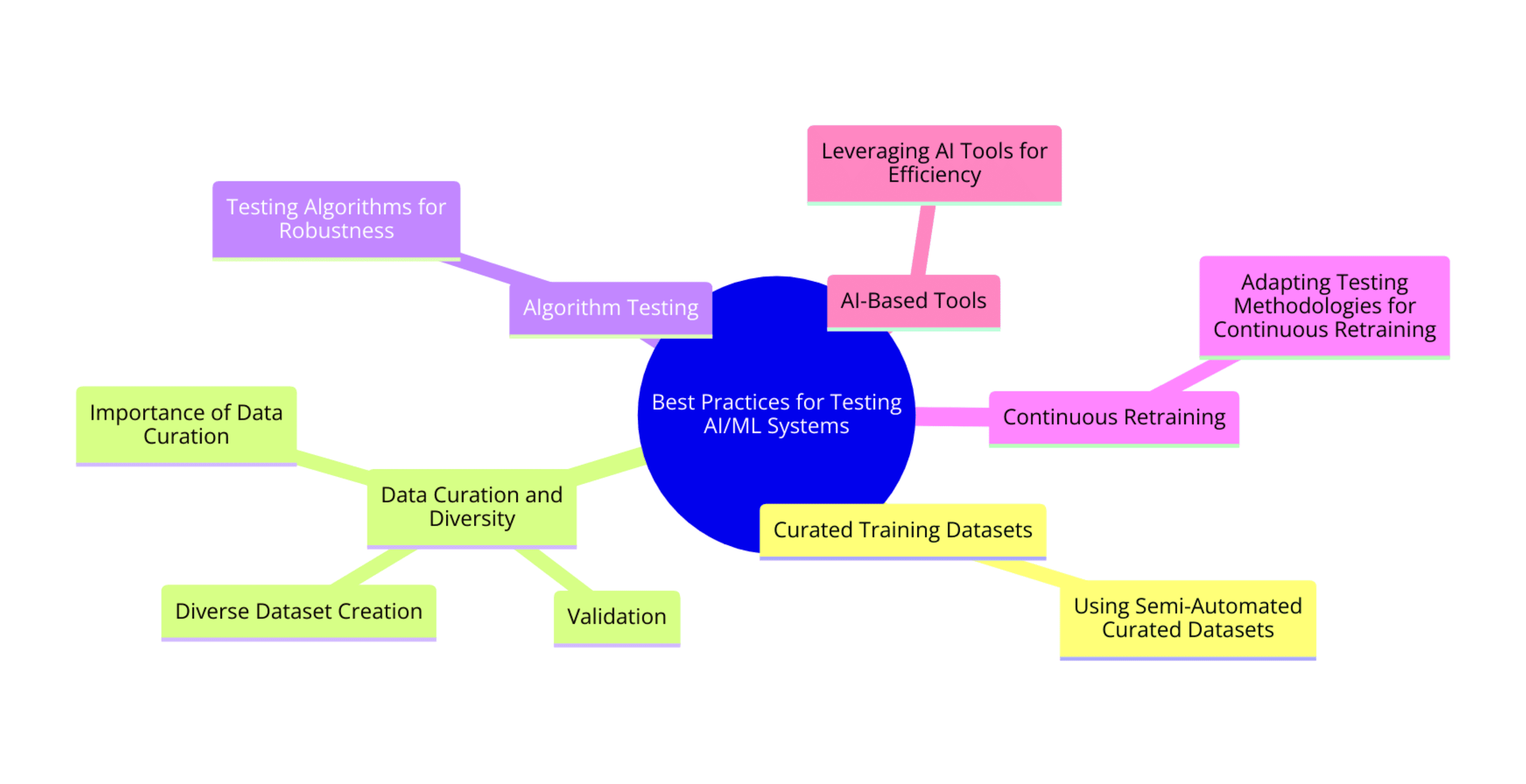
Let's discuss some best practices for effectively testing AI and ML systems. These strategies ensure the systems are functional, efficient, fair, and transparent.
Using Semi-Automated Curated Training Datasets for Effective Testing
One of the foundational steps in testing AI systems is to ensure that the training datasets are well-curated and representative of real-world scenarios. Employing semi-automated tools to curate and verify the quality and diversity of these datasets helps minimise bias and improves the overall robustness of the models.
Importance of Data Curation, Validation, and Diverse Dataset Creation
Data curation and validation are critical to preparing datasets that accurately reflect the complexity of the tasks the AI is designed to perform. This involves removing erroneous data, ensuring data is correctly labelled, and creating datasets that include diverse scenarios and demographics to prevent bias in model training.
Algorithm Testing
Testing AI algorithms involves more than just assessing performance metrics like accuracy or speed. It also includes evaluating the security aspects to prevent adversarial attacks and ensuring that the algorithms integrate well with other software components or systems, maintaining functionality across the technology stack.
# Example code for performance testing of an AI model
from sklearn.metrics import accuracy_score
def test_model_performance(model, features, labels):
predictions = model.predict(features)
accuracy = accuracy_score(labels, predictions)
return accuracy
# Assuming 'model', 'test_features', and 'test_labels' are predefined
model_accuracy = test_model_performance(model, test_features, test_labels)
print(f"Model Accuracy: {model_accuracy}")
Adapting Testing Methodologies for Sustained Testing Due to Continuous Model Retraining
As AI models often undergo continuous retraining to improve their performance or adapt to new data, testing methodologies must also be adapted to accommodate these changes. This includes regular re-evaluation of models to ensure that updates do not degrade the system's performance or introduce new biases.
Leveraging AI-Based Tools for More Efficient Testing Processes
AI-based tools can automate and enhance the testing process. They can simulate various conditions and scenarios faster than manual testing, providing comprehensive insights into model behaviour and potential weaknesses.
# Example code for using an AI-based tool to automate test scenario generation
# Assume 'generate_test_scenarios' is a function provided by an AI-based testing tool
test_scenarios = generate_test_scenarios(model, num_scenarios=100)
results = [test_model(model, scenario) for scenario in test_scenarios]
Employing these best practices in testing AI and ML systems ensures their reliability and efficiency and upholds ethical standards by actively preventing biases and ensuring transparency. Next, we will explore the tools and technologies available for testing AI applications, providing you with practical resources to implement these best practices.
Tools and Technologies for AI Application Testing
Let's explore the various tools and technologies available for testing AI applications. These specialised resources can significantly enhance the efficiency and effectiveness of testing processes.
TensorFlow Extended (TFX)
TensorFlow Extended (TFX) is another powerful tool that offers a suite of libraries and capabilities designed to facilitate the production and testing of ML models. TFX provides components for validating data and model quality, critical for maintaining robust AI systems.
Open Source Tools
There are several open-source tools and libraries that can be used for AI-driven testing:
TensorFlow: A free and open-source software library for machine learning and artificial intelligence. It can be used across a range of applications, including testing.
Selenium: A popular open-source web automation framework for browser automation. While not AI-specific, Selenium provides a foundation for building AI-powered testing tools.
Appium: An open-source test automation framework for mobile apps. It uses the WebDriver protocol to automate mobile apps on iOS and Android platforms.
Robot Framework: A generic test automation framework for acceptance testing and acceptance test-driven development (ATDD). It uses a keyword-driven testing approach and supports various programming languages.
Watir (Web Application Testing in Ruby): Provides open-source Ruby libraries for automating web browsers. It uses Selenium under the hood and supports multiple browsers.
JUnit: A unit testing framework for Java. While not AI-focused, it provides a foundation for building automated tests and can be integrated with AI libraries.
Robotium: An open-source Android UI testing framework that supports testing of native and hybrid Android apps.
These open-source tools and libraries offer a solid starting point for incorporating AI into testing workflows. However, it's important to note that building a fully AI-driven testing solution requires significant effort and expertise. Integrating these tools with AI frameworks like TensorFlow can enable advanced capabilities such as visual testing, self-healing tests, and predictive analytics.
Benefits of Using AI-powered Tools for Smarter, Faster Test Creation and Maintenance
The use of AI-powered tools in testing offers several benefits:
Efficiency: AI tools can quickly generate test cases and scenarios that cover a broad range of conditions, significantly reducing the manual effort required.
Accuracy: These tools help ensure high test accuracy by automatically detecting and adjusting for changes in the application or data that might be missed manually.
Maintenance: AI tools can adapt to changes in the application, automatically updating tests to remain relevant as the application evolves.
These tools and technologies provide critical support in effectively testing AI applications, ensuring that they function as intended and adhere to high standards of quality and ethics. Next, we will explore the different tests that can be conducted on AI-powered applications to ensure comprehensive coverage.
Types of Testing for AI-Powered Applications
Let's explore the diverse types of testing specifically tailored for AI-powered applications, ensuring these systems function correctly across various scenarios and meet all necessary performance benchmarks.
AI-powered applications require thorough testing to ensure they meet functional requirements and perform optimally under different conditions:
Functional Testing checks if the system does what it’s supposed to do according to its requirements.
Usability Testing assesses how easy and intuitive the application is for end-users.
Performance Testing ensures the application performs well under expected workload scenarios.
Integration Testing verifies that the AI integrates seamlessly with other system components.
API Testing confirms that the application programming interfaces work correctly across different platforms.
Security Testing is crucial to ensure the AI system is secure from external threats and data breaches.
Unique Types of Testing
Specialised testing types also play a critical role in AI validation:
Black Box Testing: Testing without prior knowledge of the system architecture or code, focusing solely on the outputs given specific inputs.
White Box Testing involves examining an application's internal structures or workings. It is often used in algorithm testing.
Metamorphic Testing: Involves testing cases where there are no known outputs. It’s beneficial for AI systems where defining test cases is inherently complex.
Non-Functional Testing: Assesses aspects not directly related to specific behaviours or functions, such as scalability and reliability.
Importance of Other Strategies
Model Backtesting: Essential for applications like financial forecasting, where historical data is used to test predictive models.
Performance Testing: Checks the model’s response times and accuracy under various computational loads.
Dual Coding/Algorithm Ensemble Strategies: Using multiple algorithms or models to validate each other’s outputs can enhance reliability and accuracy.
# Example of performance testing using Python
import time
def test_model_speed(model, data):
start_time = time.time()
predictions = model.predict(data)
end_time = time.time()
print(f"Model processed {len(data)} records in {end_time - start_time} seconds.")
# Assuming 'model' and 'data' are predefined
test_model_speed(model, data)
This simple example measures how long it takes to process a data set, providing insight into the model's performance under operational conditions.
Testing AI-powered applications with these comprehensive strategies ensures they are robust, reliable, and ready for real-world deployment. As AI continues to evolve, so too will the approaches to testing. Next, we'll discuss the crucial role of addressing biases and ensuring fairness in AI systems.
Addressing Bias and Fairness in AI Systems
Let's address bias and fairness in AI systems, which are critical to ensuring that AI applications are equitable and do not perpetuate existing disparities.
Strategies to Mitigate Data Skewness, Prediction Bias, and Relational Bias
Bias in AI systems can stem from various sources, particularly from the data used to train these systems. Implementing strategies to mitigate such biases is crucial:
Data Skewness: Ensuring that the training data is representative of the real-world scenarios the AI will encounter can help minimise skewness. This involves including diverse data samples that cover various demographics and conditions.
Prediction Bias: Regularly testing the AI's predictions across different groups and adjusting the model to ensure all groups are treated fairly.
Relational Bias involves analysing the relationships and correlations learned by the AI to ensure they are valid and not based on biased assumptions.
The Role of Fairness Testing Tools in Identifying and Reducing Biases
Fairness testing tools are essential for systematically identifying and mitigating biases in AI systems. These tools can analyze how AI models make decisions and whether certain groups are unfairly treated based on sensitive attributes like race, gender, or age.
Highlighting the Importance of Diverse Testing Scenarios to Ensure AI Fairness
Creating diverse testing scenarios that simulate real-world situations is crucial for evaluating AI fairness. This includes:
Testing AI systems across a broad range of demographic groups.
Synthetic data simulates rare conditions not well-represented in the training data.
Employing adversarial testing to challenge the AI with complex or edge cases.
# Example of using AI Fairness 360 to check for bias in a dataset
from aif360.datasets import BinaryLabelDataset
from aif360.metrics import BinaryLabelDatasetMetric
def assess_dataset_bias(dataset, privileged_groups, unprivileged_groups):
binary_dataset = BinaryLabelDataset(df=dataset, label_names=['label'], protected_attribute_names=['protected_attribute'])
metric = BinaryLabelDatasetMetric(binary_dataset,
unprivileged_groups=unprivileged_groups,
privileged_groups=privileged_groups)
print("Disparate Impact: {:.2f}".format(metric.disparate_impact()))
print("Statistical Parity Difference: {:.2f}".format(metric.statistical_parity_difference()))
# Assuming 'dataset', 'privileged_groups', and 'unprivileged_groups' are predefined
assess_dataset_bias(dataset, privileged_groups, unprivileged_groups)
This snippet demonstrates how to use tools like AI Fairness 360 to assess potential biases in datasets, ensuring that AI models are tested for fairness across all groups.
Addressing bias and ensuring fairness in AI systems are ongoing challenges that require continuous attention and strategy adaptation. These efforts are crucial for building trust and ensuring the ethical deployment of AI technologies. Next, we'll explore practical considerations in AI system testing.
Practical Considerations in AI System Testing
Let's now discuss the practical considerations essential when testing AI systems. These considerations help ensure that AI technologies are developed and maintained with high accuracy, reliability, and ethical standards.
Human involvement remains crucial in the loop of AI system development, particularly in data gathering and dataset improvement processes. Humans can provide essential insights into the nuances of data that AI might overlook, such as cultural contexts or implicit meanings. They can also help refine datasets by identifying and correcting errors that automated systems may propagate.
Challenges and Metrics for AI Data Sourcing
Sourcing high-quality data for AI training involves several challenges:
Data Variety: Ensuring that the data covers many scenarios, including rare edge cases, but critical for comprehensive training.
Data Veracity: Maintaining the accuracy and authenticity of data.
Data Volume: Collecting sufficiently large datasets to train robust models.
Metrics for evaluating the effectiveness of AI data sourcing include data accuracy, diversity scores, and the frequency of data updates.
The Evolving Role of QA Specialists
The role of Quality Assurance (QA) specialists is rapidly evolving in the context of AI:
From Manual to Automated: QA roles are shifting from manual testing to overseeing automated testing systems that can handle the complexity and scale of AI applications.
Specialization in AI Ethics and Bias: QA specialists are increasingly required to know AI ethics, focusing on identifying and mitigating biases in AI models.
Continuous Learning and Adaptation: As AI systems continuously learn and adapt, QA specialists must also continuously update their testing strategies and tools to keep pace with the changes in AI behavior.
# Example of a QA process in AI testing
def evaluate_model_quality(model, test_data, metrics):
results = model.predict(test_data)
quality_scores = {metric: metrics[metric](test_data, results) for metric in metrics}
return quality_scores
# Assuming 'model', 'test_data', and 'metrics' are predefined
quality_results = evaluate_model_quality(model, test_data, {'accuracy': accuracy_score, 'f1_score': f1_score})
print("Quality Evaluation Results:", quality_results)
This example illustrates how a QA specialist might use automated tools to evaluate the quality of an AI model, applying various metrics to ensure it meets the required standards.
Understanding these practical considerations is vital for effectively testing and maintaining AI systems, ensuring they function as intended and adhere to ethical guidelines. Next, we will explore the future of AI testing, looking at how emerging trends and technologies are shaping the field.
The Future of AI Testing
Let's explore the future of AI testing, focusing on how emerging technologies and methodologies are expected to enhance and transform the testing landscape. This will give us insights into the continuous evolution of AI and its implications for testing practices.
Predictions on the Integration of AI in Software Testing
The integration of AI into software testing is set to revolutionize the field by automating complex tasks and providing deeper insights into software behavior. AI can analyze vast amounts of data to identify patterns and predict potential issues before they become apparent, significantly improving the efficiency and effectiveness of testing processes.
Improving the Testing Cycle
AI's capability for continuous learning makes it ideal for constant testing, where systems are constantly evaluated in real-time. This approach allows for immediate feedback and rapid iteration, which is crucial in fast-paced development environments. AI can automate the testing cycle's repetitive parts, freeing human testers to focus on more strategic activities.
Example: AI tools can monitor the performance of live systems and automatically trigger tests in response to changes or newly detected conditions. This ensures the system is continually validated and reduces the time to detect and resolve issues.
Emerging Trends and Technologies in AI and ML Testing
Several trends and technologies are shaping the future of AI testing:
Increased Use of Simulation and Virtual Testing Environments: Advanced simulation tools allow testers to create detailed, realistic environments to test AI behaviors without the risks and costs associated with real-world testing.
Growth of Predictive Analytics in Testing: AI-driven predictive analytics can forecast potential failure points and suggest optimizations, making testing proactive rather than reactive.
Expansion of Testing Capabilities with Generative AI: Generative AI models can create new test cases and data scenarios, expanding test coverage beyond what human testers might conceive.
# Example code for using AI to generate test scenarios
from some_ai_testing_library import AITestGenerator
ai_test_gen = AITestGenerator()
test_scenarios = ai_test_gen.generate_scenarios('path/to/model')
for scenario in test_scenarios:
result = run_test(scenario)
if not result.passed:
print(f"Failed scenario: {scenario.description}")
This hypothetical example illustrates how generative AI could automatically produce test scenarios, assessing a model across a broader range of conditions than manually predefined tests.
Conclusion
The future of AI testing is rich with potential, promising to make testing more proactive, efficient, and comprehensive. As AI technologies evolve, so will the methods and tools used to ensure they are safe, reliable, and effective. This concludes our exploration of AI testing. If you have any questions or need further information on any aspects discussed, feel free to ask!
RagaAI has developed a comprehensive AI testing platform that offers over 300 tests to automatically detect issues, diagnose and fix them instantly. The platform supports various data types such as large language models (LLMs), images, videos, 3D, and audio. Embrace the future of AI with Raga AI—where innovation meets integrity.
Take the next step in your AI journey. Visit Raga AI's website today to learn more about how our synthetic data platforms can revolutionize your applications.
Subscribe to our newsletter to never miss an update
Other articles


Agents Talk, Models Think: A2A + MCP for Enterprise Agentic AI

Riya Parikh, Nitai Agarwal
Apr 27, 2025
Read the article
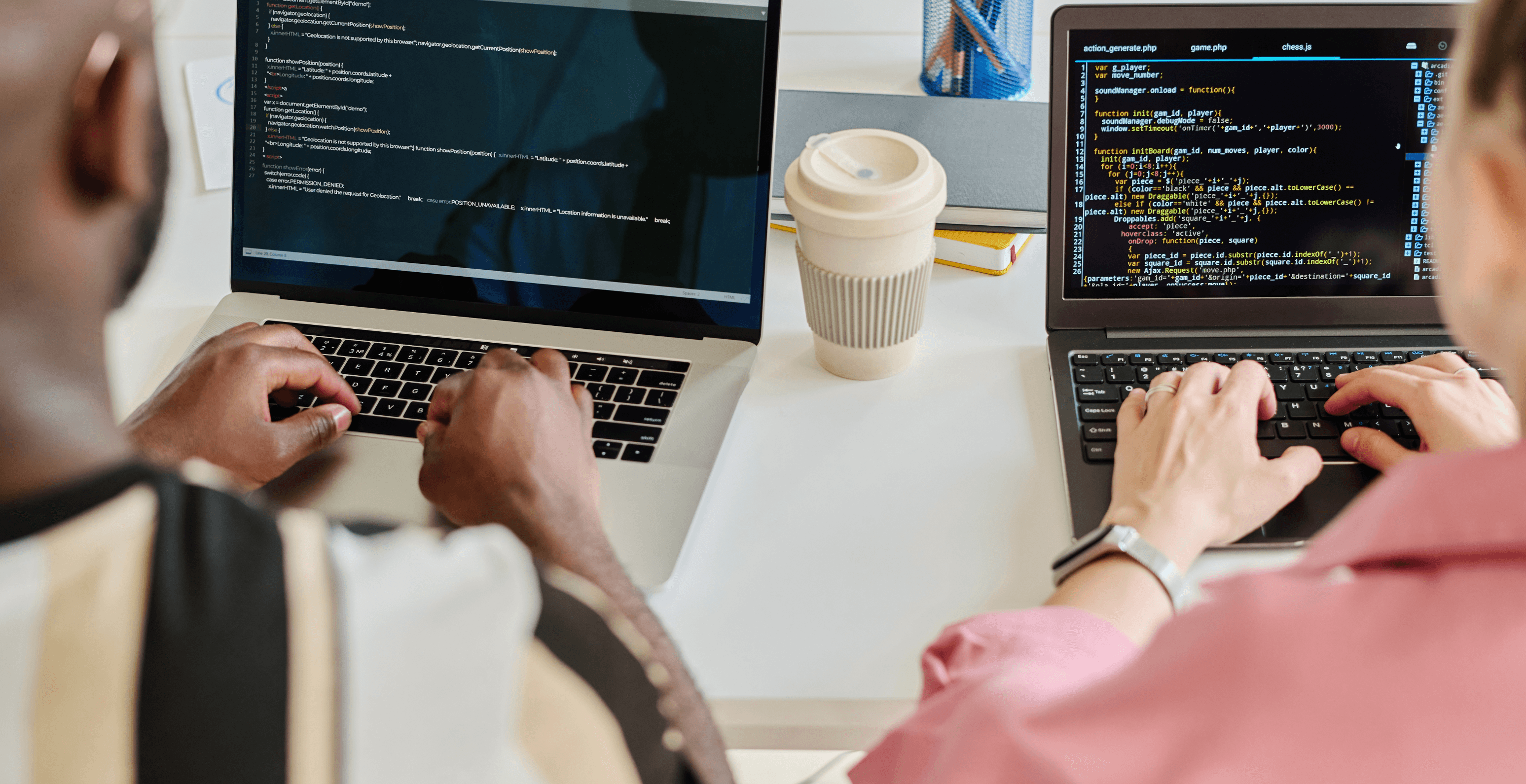
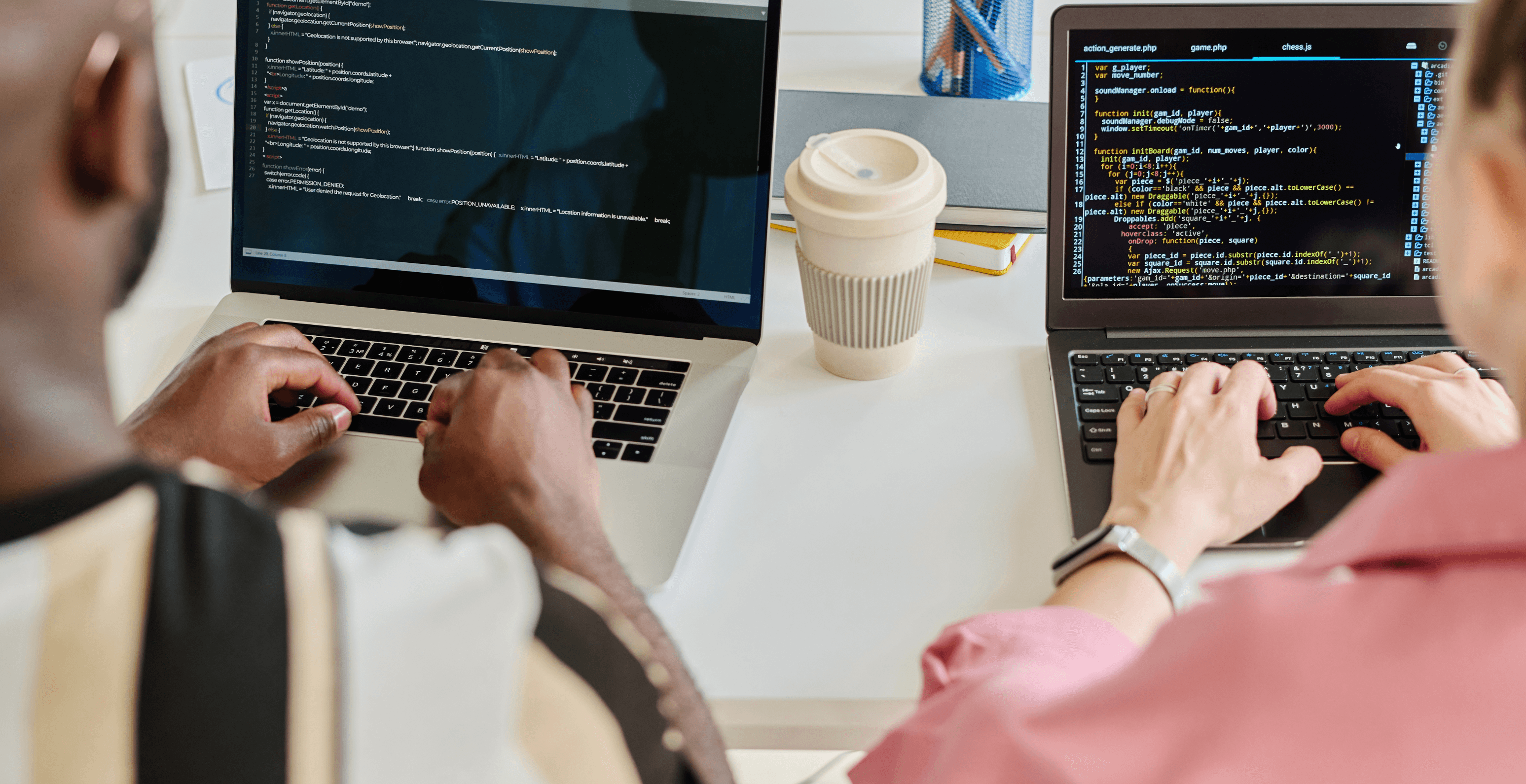
Understanding NeMo Guardrails: A Toolkit for LLM Security

Rehan Asif
Dec 24, 2024
Read the article


Understanding Differences in Large vs Small Language Models (LLM vs SLM)

Rehan Asif
Dec 21, 2024
Read the article