Solving LLM Token Limit Issues: Understanding and Approaches
Solving LLM Token Limit Issues: Understanding and Approaches
Solving LLM Token Limit Issues: Understanding and Approaches
Rehan Asif
Oct 27, 2024




Large Language Models (LLMs) such as ChatGPT represent a significant breakthrough in artificial intelligence. These models process and generate human-like text by learning from vast datasets of existing text. By understanding context and nuances in language, LLMs can engage in conversations, answer queries, and even create content that is often indistinguishable from that written by humans.
In Natural Language Processing (NLP), LLMs have transformed how machines understand and interact with human language. Their ability to analyze, interpret, and predict text data has applications across numerous industries, from automating customer service interactions to aiding in complex decision-making processes.
Challenges in Natural Language Processing with Large Documents
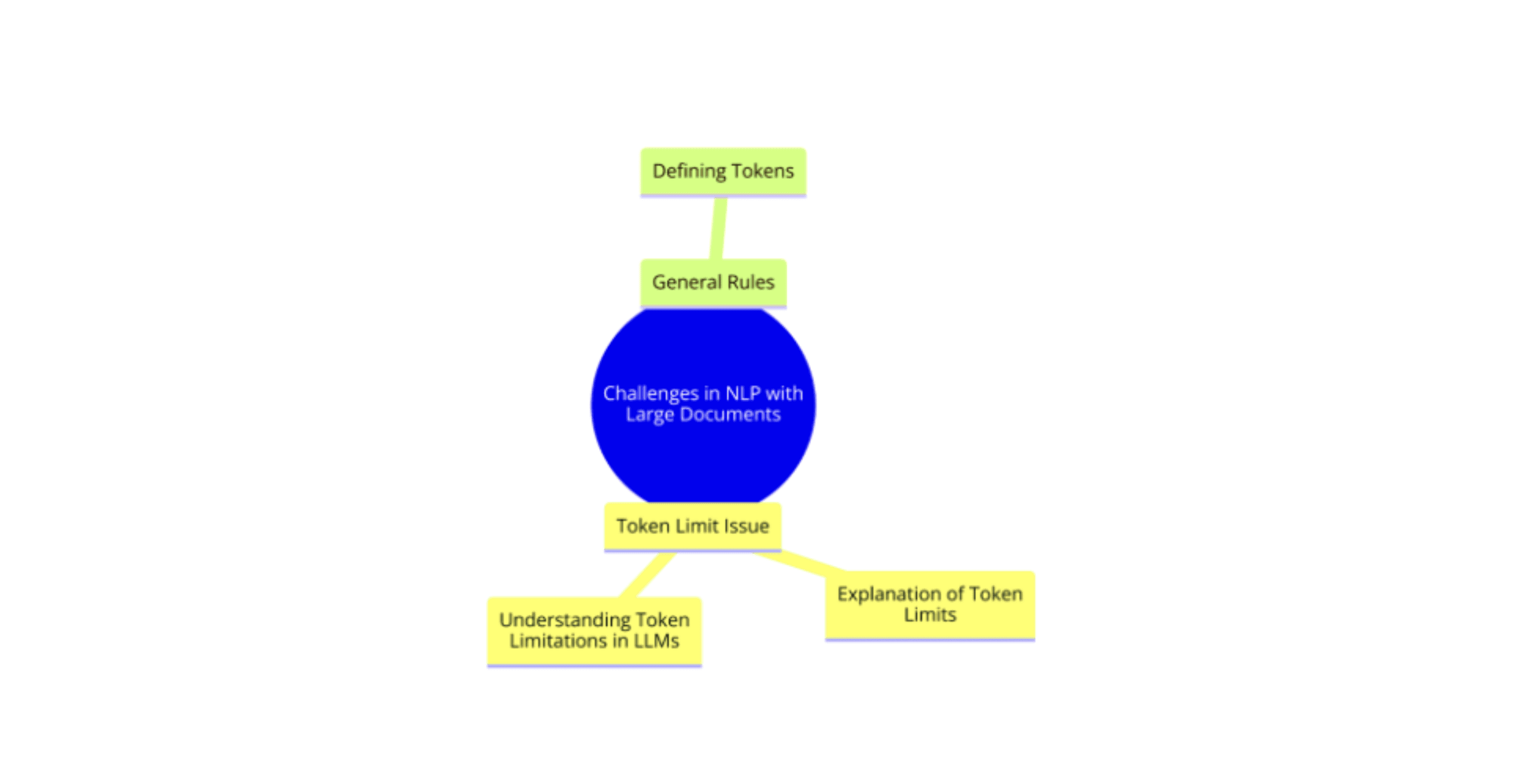
Despite their advanced capabilities, LLMs need help with processing large documents. These challenges primarily arise from the inherent limitations in handling lengthy texts due to the input data size or the computational demands required to process them effectively.
Token Limit Issue Explained
One particular challenge is the token limit issue. In NLP, texts are broken down into tokens (which can be words or parts of words), and LLMs like ChatGPT have a maximum number of tokens they can process in one go. This limit often restricts the model's ability to handle longer documents, affecting its effectiveness in contexts requiring detailed document analysis.
Understanding Token Limitations in LLMs
A "token" is a language model's basic text unit in language processing. Depending on the model's design, a token can be a word, part of a word (like a syllable), or even punctuation. Tokens are crucial because they are input data for language models to analyze and generate text. The more effectively a model can break down and understand these tokens, the better it can perform tasks involving language comprehension and generation.
General Rules for Defining Tokens
Tokens are typically defined using tokenization, where text is split into manageable pieces. The rules for tokenization can vary depending on the language, the task's specifics, or the language model's design. For instance, some models treat words as tokens, while others include spaces and punctuation.
Tokenization by Example and Methods
For example, the sentence "Hello, world!" could be tokenized into ["Hello", ",", "world", "!"] in a word-based approach. In contrast, using a tool like the Keras Tokenizer, which is popular in machine learning applications, might involve converting each token into numerical values representing their position in a learned index for processing efficiency.
from keras.preprocessing.text import Tokenizer
# Example of setting up a Keras Tokenizer
tokenizer = Tokenizer()
text = ["Hello, world!"]
tokenizer.fit_on_texts(text)
sequence = tokenizer.texts_to_sequences(text)
print(sequence)
Strategies to Overcome Token Limitations

Truncation with Example of Truncating Text in Python
One straightforward approach to manage token limitations is truncation, where the text exceeding the token limit is simply cut off. While this method is easy to implement, it risks losing important information that might be at the end of the text. Here’s how you might truncate text in Python:
def truncate_text(text, max_length):
# Split the text into tokens
tokens = text.split()
# Truncate the tokens to the maximum length allowed
if len(tokens) > max_length:
tokens = tokens[:max_length]
return ' '.join(tokens)
# Example usage
sample_text = "This is an example text that might be too long for certain processing limits."
truncated_text = truncate_text(sample_text, 10) # Limit to 10 tokens
print(truncated_text)
Chunk Processing Including Processing Text in Smaller Chunks
To ensure no critical information is missed, the text can be divided into smaller chunks that fit within the token limit. This method involves processing each chunk separately and combining the results, if necessary. This approach is beneficial for tasks like sentiment analysis or when the overall context does not need to be continuous.
Summarization Techniques to Reduce Token Count
Another effective strategy is to use summarization techniques to condense the text without losing critical information. Automated summarization tools can reduce the length of the text while retaining key points, thereby decreasing the number of tokens.
Remove Redundant Terms with an Example of Stop Word Removal Using NLTK
Removing redundant terms, such as common stop words, can also help reduce the token count. Here's an example using the Natural Language Toolkit (NLTK) to remove stop words:
import nltk
from nltk.corpus import stopwords
nltk.download('stopwords')
def remove_stop_words(text):
stop_words = set(stopwords.words('english'))
tokens = text.split()
filtered_tokens = [word for word in tokens if not word in stop_words]
return ' '.join(filtered_tokens)
# Example usage
text_with_stopwords = "This is an example where the stop words will be removed from the text."
cleaned_text = remove_stop_words(text_with_stopwords)
print(cleaned_text)
Fine-tuning Language Models
Fine-tuning language models on specific domains or shorter texts can also help them perform better within the constraints of token limits, adapting their capabilities to more focused content types.
Document Chunking with Description and Example Code for Splitting
Document chunking involves breaking a document into smaller, more manageable pieces. Here's a simple way to chunk text based on a specified number of tokens:
def chunk_text(text, chunk_size):
tokens = text.split()
for i in range(0, len(tokens), chunk_size):
yield ' '.join(tokens[i:i+chunk_size])
# Example usage
full_text = "Here is some longer text that needs to be split into smaller chunks for processing."
chunks = list(chunk_text(full_text, 10)) # Each chunk will have 10 tokens
print(chunks)
Parallel Processing with Explanation and Sample Code for Concurrent Processing
Parallel processing can handle multiple chunks concurrently, speeding up the overall processing time. This approach utilizes various CPU or GPU resources to process different text sections simultaneously.
Model Distribution Strategy with Example for Assigning Models
Distributing the workload across multiple model instances can also help manage significant texts more effectively. This might involve setting up a server cluster where different models handle document chunks.
Response Aggregation Mechanism with Sample Code for Aggregating Results
Finally, aggregating responses from multiple chunks or models is crucial to form a cohesive output. This can be done through various techniques, depending on the task:
def aggregate_responses(responses):
# Simple aggregation example: concatenate responses
return ' '.join(responses)
# Example usage
responses = ["This is part one of the response,", "and this is part two of the response."]
final_response = aggregate_responses(responses)
print(final_response)
These strategies provide a comprehensive approach to utilizing LLMs despite token limitations, ensuring that even extensive documents can be processed accurately and efficiently. Are you ready to explore how these strategies are practically applied to address token and rate limit issues?
Addressing Token and Rate Limit Issues
Now, let's explore how effectively managing token and rate limits not only resolve specific technical issues but also enhances the overall efficiency and capability of Large Language Models (LLMs) in handling extensive data.
Overview of Benefits Relating to Token Limit Issue
Addressing token limitations is crucial for ensuring that LLMs can handle extensive documents or datasets without sacrificing performance or accuracy. By implementing strategies like chunking, summarization, and parallel processing, developers can maximize the utility of LLMs in scenarios where the volume of data would otherwise be prohibitive.
Summary of Advantages Concerning Rate Limit Issue
Similar strategies can also help manage rate limits, which restrict the frequency of data processing to prevent overload and ensure equitable resource distribution among users. Developers can avoid hitting these rate limits by optimizing how and when data is sent for processing, thus maintaining steady workflow efficiency.
How Strategies Optimize Processing Time
These strategies collectively optimize processing time by ensuring that each piece of data is handled most efficiently. For example:
Chunk Processing: Divides the workload into manageable parts, allowing for faster individual processing and more effective use of resources.
Parallel Processing: Utilizes multiple processors simultaneously, significantly reducing the time to handle large volumes of data.
Response Aggregation: By efficiently combining results from various processes, response aggregation minimizes the overhead and latency of handling large datasets.
Here’s how a combined strategy might look in practice:
# Example Python code for parallel processing and aggregation of results
from concurrent.futures import ThreadPoolExecutor
def process_chunk(chunk):
# Simulate processing (e.g., running a model prediction)
return f"Processed {chunk}"
def main(text, chunk_size):
chunks = list(chunk_text(text, chunk_size)) # Assuming chunk_text is defined
results = []
with ThreadPoolExecutor(max_workers=5) as executor:
future_to_chunk = {executor.submit(process_chunk, chunk): chunk for chunk in chunks}
for future in concurrent.futures.as_completed(future_to_chunk):
chunk = future_to_chunk[future]
try:
result = future.result()
except Exception as exc:
print(f'{chunk} generated an exception: {exc}')
else:
results.append(result)
return aggregate_responses(results) # Assuming aggregate_responses is defined
# Example usage
large_text = "Here is some very long text that needs to be split and processed in parallel to avoid token and rate limits."
print(main(large_text, 10))
This example demonstrates how to process text in parallel, reducing overall processing time and efficiently handling larger volumes than typically allowed by token and rate limits.
By effectively addressing token and rate limit issues, organizations can enhance the scalability and responsiveness of their LLM applications, ensuring they remain robust across a range of demanding scenarios.
Finally, as the field of AI continues to evolve, exploring low-code solutions that incorporate these strategies can significantly reduce the barrier to entry for deploying advanced AI technologies. Low-code platforms that support modular, scalable approaches to token management will be particularly valuable in democratizing AI, making powerful tools like LLMs accessible to a broader range of users and industries.
In conclusion, while token limitations present significant challenges, the strategies and technologies developed to overcome them not only solve practical issues but also drive forward the capabilities of AI in processing natural language at scale. As we refine these approaches and technologies, the scope of what's possible with LLMs will undoubtedly expand, offering exciting prospects for the future of AI and machine learning.
Conclusion
Understanding token limitations in LLMs is crucial for anyone developing or deploying natural language processing applications at scale. If not properly managed, these limitations can significantly restrict the effectiveness of LLMs, especially when dealing with large volumes of text. By employing strategic approaches to managing these limitations, developers can unlock the full potential of LLMs, making them more versatile and capable in various complex scenarios.
The strategies discussed—ranging from text truncation and chunk processing to advanced techniques like summarization and parallel processing—provide robust solutions to the challenges posed by token limits. These approaches ensure that large documents are processed efficiently and enhance the overall performance of LLMs by allowing them to handle more data without compromising speed or accuracy.
Real-world Impact: In practical applications, these strategies have proven highly effective. For instance, in legal and medical fields where document length often exceeds standard token limits, chunking, and summarization allow for thorough analysis without losing critical information. Similarly, in customer service, these strategies enable handling extensive customer interactions in real-time, improving both response quality and customer satisfaction.
Large Language Models (LLMs) such as ChatGPT represent a significant breakthrough in artificial intelligence. These models process and generate human-like text by learning from vast datasets of existing text. By understanding context and nuances in language, LLMs can engage in conversations, answer queries, and even create content that is often indistinguishable from that written by humans.
In Natural Language Processing (NLP), LLMs have transformed how machines understand and interact with human language. Their ability to analyze, interpret, and predict text data has applications across numerous industries, from automating customer service interactions to aiding in complex decision-making processes.
Challenges in Natural Language Processing with Large Documents
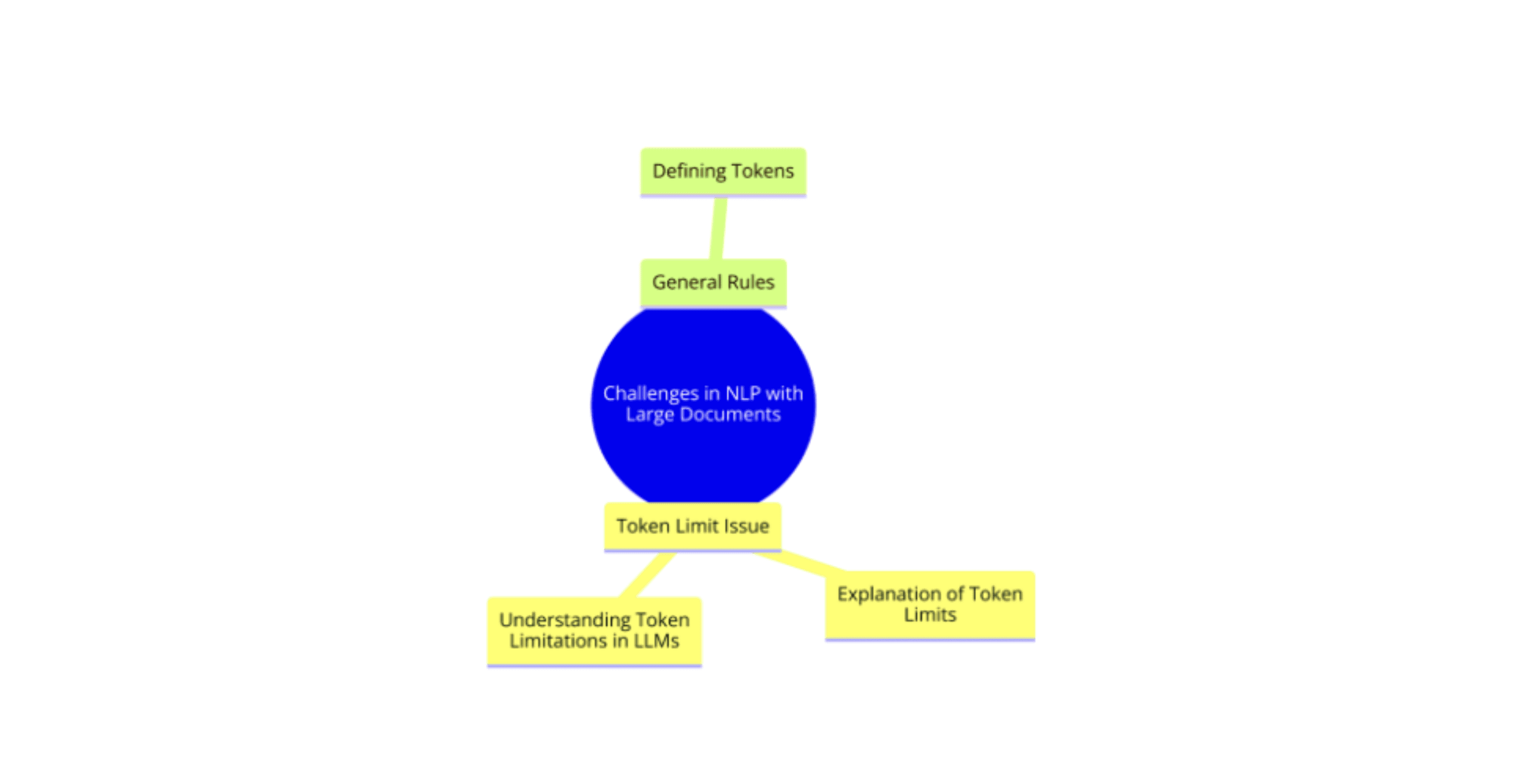
Despite their advanced capabilities, LLMs need help with processing large documents. These challenges primarily arise from the inherent limitations in handling lengthy texts due to the input data size or the computational demands required to process them effectively.
Token Limit Issue Explained
One particular challenge is the token limit issue. In NLP, texts are broken down into tokens (which can be words or parts of words), and LLMs like ChatGPT have a maximum number of tokens they can process in one go. This limit often restricts the model's ability to handle longer documents, affecting its effectiveness in contexts requiring detailed document analysis.
Understanding Token Limitations in LLMs
A "token" is a language model's basic text unit in language processing. Depending on the model's design, a token can be a word, part of a word (like a syllable), or even punctuation. Tokens are crucial because they are input data for language models to analyze and generate text. The more effectively a model can break down and understand these tokens, the better it can perform tasks involving language comprehension and generation.
General Rules for Defining Tokens
Tokens are typically defined using tokenization, where text is split into manageable pieces. The rules for tokenization can vary depending on the language, the task's specifics, or the language model's design. For instance, some models treat words as tokens, while others include spaces and punctuation.
Tokenization by Example and Methods
For example, the sentence "Hello, world!" could be tokenized into ["Hello", ",", "world", "!"] in a word-based approach. In contrast, using a tool like the Keras Tokenizer, which is popular in machine learning applications, might involve converting each token into numerical values representing their position in a learned index for processing efficiency.
from keras.preprocessing.text import Tokenizer
# Example of setting up a Keras Tokenizer
tokenizer = Tokenizer()
text = ["Hello, world!"]
tokenizer.fit_on_texts(text)
sequence = tokenizer.texts_to_sequences(text)
print(sequence)
Strategies to Overcome Token Limitations

Truncation with Example of Truncating Text in Python
One straightforward approach to manage token limitations is truncation, where the text exceeding the token limit is simply cut off. While this method is easy to implement, it risks losing important information that might be at the end of the text. Here’s how you might truncate text in Python:
def truncate_text(text, max_length):
# Split the text into tokens
tokens = text.split()
# Truncate the tokens to the maximum length allowed
if len(tokens) > max_length:
tokens = tokens[:max_length]
return ' '.join(tokens)
# Example usage
sample_text = "This is an example text that might be too long for certain processing limits."
truncated_text = truncate_text(sample_text, 10) # Limit to 10 tokens
print(truncated_text)
Chunk Processing Including Processing Text in Smaller Chunks
To ensure no critical information is missed, the text can be divided into smaller chunks that fit within the token limit. This method involves processing each chunk separately and combining the results, if necessary. This approach is beneficial for tasks like sentiment analysis or when the overall context does not need to be continuous.
Summarization Techniques to Reduce Token Count
Another effective strategy is to use summarization techniques to condense the text without losing critical information. Automated summarization tools can reduce the length of the text while retaining key points, thereby decreasing the number of tokens.
Remove Redundant Terms with an Example of Stop Word Removal Using NLTK
Removing redundant terms, such as common stop words, can also help reduce the token count. Here's an example using the Natural Language Toolkit (NLTK) to remove stop words:
import nltk
from nltk.corpus import stopwords
nltk.download('stopwords')
def remove_stop_words(text):
stop_words = set(stopwords.words('english'))
tokens = text.split()
filtered_tokens = [word for word in tokens if not word in stop_words]
return ' '.join(filtered_tokens)
# Example usage
text_with_stopwords = "This is an example where the stop words will be removed from the text."
cleaned_text = remove_stop_words(text_with_stopwords)
print(cleaned_text)
Fine-tuning Language Models
Fine-tuning language models on specific domains or shorter texts can also help them perform better within the constraints of token limits, adapting their capabilities to more focused content types.
Document Chunking with Description and Example Code for Splitting
Document chunking involves breaking a document into smaller, more manageable pieces. Here's a simple way to chunk text based on a specified number of tokens:
def chunk_text(text, chunk_size):
tokens = text.split()
for i in range(0, len(tokens), chunk_size):
yield ' '.join(tokens[i:i+chunk_size])
# Example usage
full_text = "Here is some longer text that needs to be split into smaller chunks for processing."
chunks = list(chunk_text(full_text, 10)) # Each chunk will have 10 tokens
print(chunks)
Parallel Processing with Explanation and Sample Code for Concurrent Processing
Parallel processing can handle multiple chunks concurrently, speeding up the overall processing time. This approach utilizes various CPU or GPU resources to process different text sections simultaneously.
Model Distribution Strategy with Example for Assigning Models
Distributing the workload across multiple model instances can also help manage significant texts more effectively. This might involve setting up a server cluster where different models handle document chunks.
Response Aggregation Mechanism with Sample Code for Aggregating Results
Finally, aggregating responses from multiple chunks or models is crucial to form a cohesive output. This can be done through various techniques, depending on the task:
def aggregate_responses(responses):
# Simple aggregation example: concatenate responses
return ' '.join(responses)
# Example usage
responses = ["This is part one of the response,", "and this is part two of the response."]
final_response = aggregate_responses(responses)
print(final_response)
These strategies provide a comprehensive approach to utilizing LLMs despite token limitations, ensuring that even extensive documents can be processed accurately and efficiently. Are you ready to explore how these strategies are practically applied to address token and rate limit issues?
Addressing Token and Rate Limit Issues
Now, let's explore how effectively managing token and rate limits not only resolve specific technical issues but also enhances the overall efficiency and capability of Large Language Models (LLMs) in handling extensive data.
Overview of Benefits Relating to Token Limit Issue
Addressing token limitations is crucial for ensuring that LLMs can handle extensive documents or datasets without sacrificing performance or accuracy. By implementing strategies like chunking, summarization, and parallel processing, developers can maximize the utility of LLMs in scenarios where the volume of data would otherwise be prohibitive.
Summary of Advantages Concerning Rate Limit Issue
Similar strategies can also help manage rate limits, which restrict the frequency of data processing to prevent overload and ensure equitable resource distribution among users. Developers can avoid hitting these rate limits by optimizing how and when data is sent for processing, thus maintaining steady workflow efficiency.
How Strategies Optimize Processing Time
These strategies collectively optimize processing time by ensuring that each piece of data is handled most efficiently. For example:
Chunk Processing: Divides the workload into manageable parts, allowing for faster individual processing and more effective use of resources.
Parallel Processing: Utilizes multiple processors simultaneously, significantly reducing the time to handle large volumes of data.
Response Aggregation: By efficiently combining results from various processes, response aggregation minimizes the overhead and latency of handling large datasets.
Here’s how a combined strategy might look in practice:
# Example Python code for parallel processing and aggregation of results
from concurrent.futures import ThreadPoolExecutor
def process_chunk(chunk):
# Simulate processing (e.g., running a model prediction)
return f"Processed {chunk}"
def main(text, chunk_size):
chunks = list(chunk_text(text, chunk_size)) # Assuming chunk_text is defined
results = []
with ThreadPoolExecutor(max_workers=5) as executor:
future_to_chunk = {executor.submit(process_chunk, chunk): chunk for chunk in chunks}
for future in concurrent.futures.as_completed(future_to_chunk):
chunk = future_to_chunk[future]
try:
result = future.result()
except Exception as exc:
print(f'{chunk} generated an exception: {exc}')
else:
results.append(result)
return aggregate_responses(results) # Assuming aggregate_responses is defined
# Example usage
large_text = "Here is some very long text that needs to be split and processed in parallel to avoid token and rate limits."
print(main(large_text, 10))
This example demonstrates how to process text in parallel, reducing overall processing time and efficiently handling larger volumes than typically allowed by token and rate limits.
By effectively addressing token and rate limit issues, organizations can enhance the scalability and responsiveness of their LLM applications, ensuring they remain robust across a range of demanding scenarios.
Finally, as the field of AI continues to evolve, exploring low-code solutions that incorporate these strategies can significantly reduce the barrier to entry for deploying advanced AI technologies. Low-code platforms that support modular, scalable approaches to token management will be particularly valuable in democratizing AI, making powerful tools like LLMs accessible to a broader range of users and industries.
In conclusion, while token limitations present significant challenges, the strategies and technologies developed to overcome them not only solve practical issues but also drive forward the capabilities of AI in processing natural language at scale. As we refine these approaches and technologies, the scope of what's possible with LLMs will undoubtedly expand, offering exciting prospects for the future of AI and machine learning.
Conclusion
Understanding token limitations in LLMs is crucial for anyone developing or deploying natural language processing applications at scale. If not properly managed, these limitations can significantly restrict the effectiveness of LLMs, especially when dealing with large volumes of text. By employing strategic approaches to managing these limitations, developers can unlock the full potential of LLMs, making them more versatile and capable in various complex scenarios.
The strategies discussed—ranging from text truncation and chunk processing to advanced techniques like summarization and parallel processing—provide robust solutions to the challenges posed by token limits. These approaches ensure that large documents are processed efficiently and enhance the overall performance of LLMs by allowing them to handle more data without compromising speed or accuracy.
Real-world Impact: In practical applications, these strategies have proven highly effective. For instance, in legal and medical fields where document length often exceeds standard token limits, chunking, and summarization allow for thorough analysis without losing critical information. Similarly, in customer service, these strategies enable handling extensive customer interactions in real-time, improving both response quality and customer satisfaction.
Large Language Models (LLMs) such as ChatGPT represent a significant breakthrough in artificial intelligence. These models process and generate human-like text by learning from vast datasets of existing text. By understanding context and nuances in language, LLMs can engage in conversations, answer queries, and even create content that is often indistinguishable from that written by humans.
In Natural Language Processing (NLP), LLMs have transformed how machines understand and interact with human language. Their ability to analyze, interpret, and predict text data has applications across numerous industries, from automating customer service interactions to aiding in complex decision-making processes.
Challenges in Natural Language Processing with Large Documents
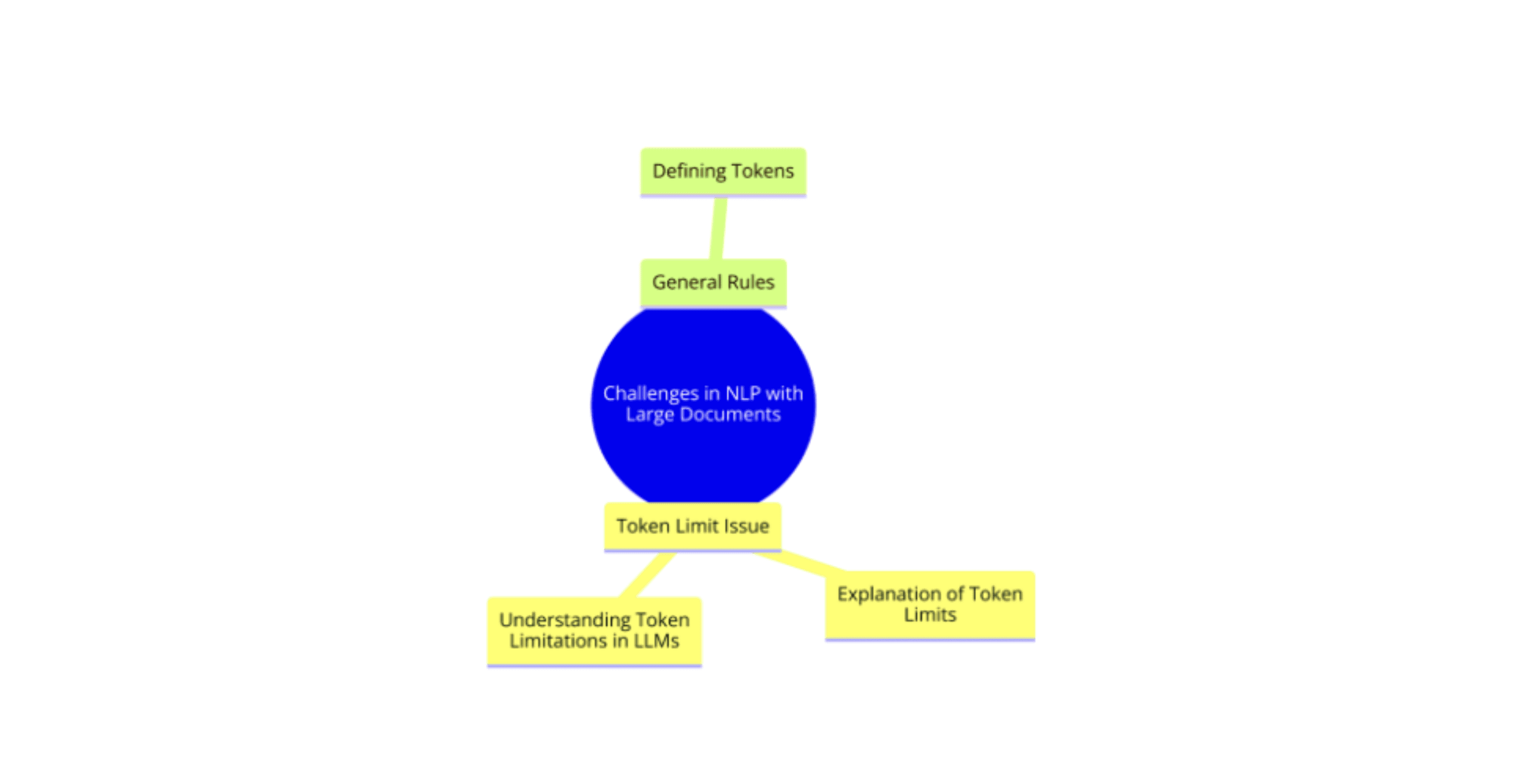
Despite their advanced capabilities, LLMs need help with processing large documents. These challenges primarily arise from the inherent limitations in handling lengthy texts due to the input data size or the computational demands required to process them effectively.
Token Limit Issue Explained
One particular challenge is the token limit issue. In NLP, texts are broken down into tokens (which can be words or parts of words), and LLMs like ChatGPT have a maximum number of tokens they can process in one go. This limit often restricts the model's ability to handle longer documents, affecting its effectiveness in contexts requiring detailed document analysis.
Understanding Token Limitations in LLMs
A "token" is a language model's basic text unit in language processing. Depending on the model's design, a token can be a word, part of a word (like a syllable), or even punctuation. Tokens are crucial because they are input data for language models to analyze and generate text. The more effectively a model can break down and understand these tokens, the better it can perform tasks involving language comprehension and generation.
General Rules for Defining Tokens
Tokens are typically defined using tokenization, where text is split into manageable pieces. The rules for tokenization can vary depending on the language, the task's specifics, or the language model's design. For instance, some models treat words as tokens, while others include spaces and punctuation.
Tokenization by Example and Methods
For example, the sentence "Hello, world!" could be tokenized into ["Hello", ",", "world", "!"] in a word-based approach. In contrast, using a tool like the Keras Tokenizer, which is popular in machine learning applications, might involve converting each token into numerical values representing their position in a learned index for processing efficiency.
from keras.preprocessing.text import Tokenizer
# Example of setting up a Keras Tokenizer
tokenizer = Tokenizer()
text = ["Hello, world!"]
tokenizer.fit_on_texts(text)
sequence = tokenizer.texts_to_sequences(text)
print(sequence)
Strategies to Overcome Token Limitations

Truncation with Example of Truncating Text in Python
One straightforward approach to manage token limitations is truncation, where the text exceeding the token limit is simply cut off. While this method is easy to implement, it risks losing important information that might be at the end of the text. Here’s how you might truncate text in Python:
def truncate_text(text, max_length):
# Split the text into tokens
tokens = text.split()
# Truncate the tokens to the maximum length allowed
if len(tokens) > max_length:
tokens = tokens[:max_length]
return ' '.join(tokens)
# Example usage
sample_text = "This is an example text that might be too long for certain processing limits."
truncated_text = truncate_text(sample_text, 10) # Limit to 10 tokens
print(truncated_text)
Chunk Processing Including Processing Text in Smaller Chunks
To ensure no critical information is missed, the text can be divided into smaller chunks that fit within the token limit. This method involves processing each chunk separately and combining the results, if necessary. This approach is beneficial for tasks like sentiment analysis or when the overall context does not need to be continuous.
Summarization Techniques to Reduce Token Count
Another effective strategy is to use summarization techniques to condense the text without losing critical information. Automated summarization tools can reduce the length of the text while retaining key points, thereby decreasing the number of tokens.
Remove Redundant Terms with an Example of Stop Word Removal Using NLTK
Removing redundant terms, such as common stop words, can also help reduce the token count. Here's an example using the Natural Language Toolkit (NLTK) to remove stop words:
import nltk
from nltk.corpus import stopwords
nltk.download('stopwords')
def remove_stop_words(text):
stop_words = set(stopwords.words('english'))
tokens = text.split()
filtered_tokens = [word for word in tokens if not word in stop_words]
return ' '.join(filtered_tokens)
# Example usage
text_with_stopwords = "This is an example where the stop words will be removed from the text."
cleaned_text = remove_stop_words(text_with_stopwords)
print(cleaned_text)
Fine-tuning Language Models
Fine-tuning language models on specific domains or shorter texts can also help them perform better within the constraints of token limits, adapting their capabilities to more focused content types.
Document Chunking with Description and Example Code for Splitting
Document chunking involves breaking a document into smaller, more manageable pieces. Here's a simple way to chunk text based on a specified number of tokens:
def chunk_text(text, chunk_size):
tokens = text.split()
for i in range(0, len(tokens), chunk_size):
yield ' '.join(tokens[i:i+chunk_size])
# Example usage
full_text = "Here is some longer text that needs to be split into smaller chunks for processing."
chunks = list(chunk_text(full_text, 10)) # Each chunk will have 10 tokens
print(chunks)
Parallel Processing with Explanation and Sample Code for Concurrent Processing
Parallel processing can handle multiple chunks concurrently, speeding up the overall processing time. This approach utilizes various CPU or GPU resources to process different text sections simultaneously.
Model Distribution Strategy with Example for Assigning Models
Distributing the workload across multiple model instances can also help manage significant texts more effectively. This might involve setting up a server cluster where different models handle document chunks.
Response Aggregation Mechanism with Sample Code for Aggregating Results
Finally, aggregating responses from multiple chunks or models is crucial to form a cohesive output. This can be done through various techniques, depending on the task:
def aggregate_responses(responses):
# Simple aggregation example: concatenate responses
return ' '.join(responses)
# Example usage
responses = ["This is part one of the response,", "and this is part two of the response."]
final_response = aggregate_responses(responses)
print(final_response)
These strategies provide a comprehensive approach to utilizing LLMs despite token limitations, ensuring that even extensive documents can be processed accurately and efficiently. Are you ready to explore how these strategies are practically applied to address token and rate limit issues?
Addressing Token and Rate Limit Issues
Now, let's explore how effectively managing token and rate limits not only resolve specific technical issues but also enhances the overall efficiency and capability of Large Language Models (LLMs) in handling extensive data.
Overview of Benefits Relating to Token Limit Issue
Addressing token limitations is crucial for ensuring that LLMs can handle extensive documents or datasets without sacrificing performance or accuracy. By implementing strategies like chunking, summarization, and parallel processing, developers can maximize the utility of LLMs in scenarios where the volume of data would otherwise be prohibitive.
Summary of Advantages Concerning Rate Limit Issue
Similar strategies can also help manage rate limits, which restrict the frequency of data processing to prevent overload and ensure equitable resource distribution among users. Developers can avoid hitting these rate limits by optimizing how and when data is sent for processing, thus maintaining steady workflow efficiency.
How Strategies Optimize Processing Time
These strategies collectively optimize processing time by ensuring that each piece of data is handled most efficiently. For example:
Chunk Processing: Divides the workload into manageable parts, allowing for faster individual processing and more effective use of resources.
Parallel Processing: Utilizes multiple processors simultaneously, significantly reducing the time to handle large volumes of data.
Response Aggregation: By efficiently combining results from various processes, response aggregation minimizes the overhead and latency of handling large datasets.
Here’s how a combined strategy might look in practice:
# Example Python code for parallel processing and aggregation of results
from concurrent.futures import ThreadPoolExecutor
def process_chunk(chunk):
# Simulate processing (e.g., running a model prediction)
return f"Processed {chunk}"
def main(text, chunk_size):
chunks = list(chunk_text(text, chunk_size)) # Assuming chunk_text is defined
results = []
with ThreadPoolExecutor(max_workers=5) as executor:
future_to_chunk = {executor.submit(process_chunk, chunk): chunk for chunk in chunks}
for future in concurrent.futures.as_completed(future_to_chunk):
chunk = future_to_chunk[future]
try:
result = future.result()
except Exception as exc:
print(f'{chunk} generated an exception: {exc}')
else:
results.append(result)
return aggregate_responses(results) # Assuming aggregate_responses is defined
# Example usage
large_text = "Here is some very long text that needs to be split and processed in parallel to avoid token and rate limits."
print(main(large_text, 10))
This example demonstrates how to process text in parallel, reducing overall processing time and efficiently handling larger volumes than typically allowed by token and rate limits.
By effectively addressing token and rate limit issues, organizations can enhance the scalability and responsiveness of their LLM applications, ensuring they remain robust across a range of demanding scenarios.
Finally, as the field of AI continues to evolve, exploring low-code solutions that incorporate these strategies can significantly reduce the barrier to entry for deploying advanced AI technologies. Low-code platforms that support modular, scalable approaches to token management will be particularly valuable in democratizing AI, making powerful tools like LLMs accessible to a broader range of users and industries.
In conclusion, while token limitations present significant challenges, the strategies and technologies developed to overcome them not only solve practical issues but also drive forward the capabilities of AI in processing natural language at scale. As we refine these approaches and technologies, the scope of what's possible with LLMs will undoubtedly expand, offering exciting prospects for the future of AI and machine learning.
Conclusion
Understanding token limitations in LLMs is crucial for anyone developing or deploying natural language processing applications at scale. If not properly managed, these limitations can significantly restrict the effectiveness of LLMs, especially when dealing with large volumes of text. By employing strategic approaches to managing these limitations, developers can unlock the full potential of LLMs, making them more versatile and capable in various complex scenarios.
The strategies discussed—ranging from text truncation and chunk processing to advanced techniques like summarization and parallel processing—provide robust solutions to the challenges posed by token limits. These approaches ensure that large documents are processed efficiently and enhance the overall performance of LLMs by allowing them to handle more data without compromising speed or accuracy.
Real-world Impact: In practical applications, these strategies have proven highly effective. For instance, in legal and medical fields where document length often exceeds standard token limits, chunking, and summarization allow for thorough analysis without losing critical information. Similarly, in customer service, these strategies enable handling extensive customer interactions in real-time, improving both response quality and customer satisfaction.
Large Language Models (LLMs) such as ChatGPT represent a significant breakthrough in artificial intelligence. These models process and generate human-like text by learning from vast datasets of existing text. By understanding context and nuances in language, LLMs can engage in conversations, answer queries, and even create content that is often indistinguishable from that written by humans.
In Natural Language Processing (NLP), LLMs have transformed how machines understand and interact with human language. Their ability to analyze, interpret, and predict text data has applications across numerous industries, from automating customer service interactions to aiding in complex decision-making processes.
Challenges in Natural Language Processing with Large Documents
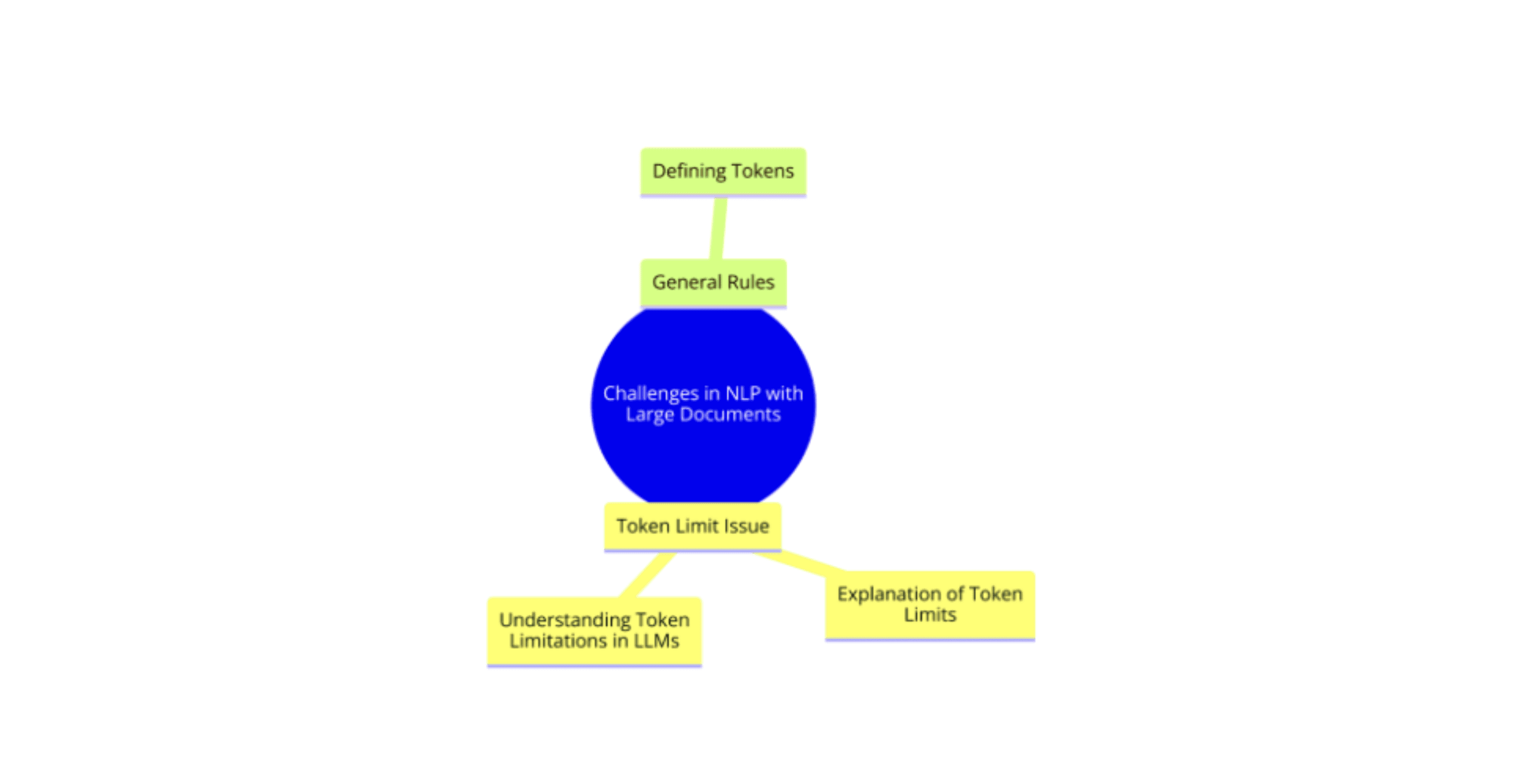
Despite their advanced capabilities, LLMs need help with processing large documents. These challenges primarily arise from the inherent limitations in handling lengthy texts due to the input data size or the computational demands required to process them effectively.
Token Limit Issue Explained
One particular challenge is the token limit issue. In NLP, texts are broken down into tokens (which can be words or parts of words), and LLMs like ChatGPT have a maximum number of tokens they can process in one go. This limit often restricts the model's ability to handle longer documents, affecting its effectiveness in contexts requiring detailed document analysis.
Understanding Token Limitations in LLMs
A "token" is a language model's basic text unit in language processing. Depending on the model's design, a token can be a word, part of a word (like a syllable), or even punctuation. Tokens are crucial because they are input data for language models to analyze and generate text. The more effectively a model can break down and understand these tokens, the better it can perform tasks involving language comprehension and generation.
General Rules for Defining Tokens
Tokens are typically defined using tokenization, where text is split into manageable pieces. The rules for tokenization can vary depending on the language, the task's specifics, or the language model's design. For instance, some models treat words as tokens, while others include spaces and punctuation.
Tokenization by Example and Methods
For example, the sentence "Hello, world!" could be tokenized into ["Hello", ",", "world", "!"] in a word-based approach. In contrast, using a tool like the Keras Tokenizer, which is popular in machine learning applications, might involve converting each token into numerical values representing their position in a learned index for processing efficiency.
from keras.preprocessing.text import Tokenizer
# Example of setting up a Keras Tokenizer
tokenizer = Tokenizer()
text = ["Hello, world!"]
tokenizer.fit_on_texts(text)
sequence = tokenizer.texts_to_sequences(text)
print(sequence)
Strategies to Overcome Token Limitations

Truncation with Example of Truncating Text in Python
One straightforward approach to manage token limitations is truncation, where the text exceeding the token limit is simply cut off. While this method is easy to implement, it risks losing important information that might be at the end of the text. Here’s how you might truncate text in Python:
def truncate_text(text, max_length):
# Split the text into tokens
tokens = text.split()
# Truncate the tokens to the maximum length allowed
if len(tokens) > max_length:
tokens = tokens[:max_length]
return ' '.join(tokens)
# Example usage
sample_text = "This is an example text that might be too long for certain processing limits."
truncated_text = truncate_text(sample_text, 10) # Limit to 10 tokens
print(truncated_text)
Chunk Processing Including Processing Text in Smaller Chunks
To ensure no critical information is missed, the text can be divided into smaller chunks that fit within the token limit. This method involves processing each chunk separately and combining the results, if necessary. This approach is beneficial for tasks like sentiment analysis or when the overall context does not need to be continuous.
Summarization Techniques to Reduce Token Count
Another effective strategy is to use summarization techniques to condense the text without losing critical information. Automated summarization tools can reduce the length of the text while retaining key points, thereby decreasing the number of tokens.
Remove Redundant Terms with an Example of Stop Word Removal Using NLTK
Removing redundant terms, such as common stop words, can also help reduce the token count. Here's an example using the Natural Language Toolkit (NLTK) to remove stop words:
import nltk
from nltk.corpus import stopwords
nltk.download('stopwords')
def remove_stop_words(text):
stop_words = set(stopwords.words('english'))
tokens = text.split()
filtered_tokens = [word for word in tokens if not word in stop_words]
return ' '.join(filtered_tokens)
# Example usage
text_with_stopwords = "This is an example where the stop words will be removed from the text."
cleaned_text = remove_stop_words(text_with_stopwords)
print(cleaned_text)
Fine-tuning Language Models
Fine-tuning language models on specific domains or shorter texts can also help them perform better within the constraints of token limits, adapting their capabilities to more focused content types.
Document Chunking with Description and Example Code for Splitting
Document chunking involves breaking a document into smaller, more manageable pieces. Here's a simple way to chunk text based on a specified number of tokens:
def chunk_text(text, chunk_size):
tokens = text.split()
for i in range(0, len(tokens), chunk_size):
yield ' '.join(tokens[i:i+chunk_size])
# Example usage
full_text = "Here is some longer text that needs to be split into smaller chunks for processing."
chunks = list(chunk_text(full_text, 10)) # Each chunk will have 10 tokens
print(chunks)
Parallel Processing with Explanation and Sample Code for Concurrent Processing
Parallel processing can handle multiple chunks concurrently, speeding up the overall processing time. This approach utilizes various CPU or GPU resources to process different text sections simultaneously.
Model Distribution Strategy with Example for Assigning Models
Distributing the workload across multiple model instances can also help manage significant texts more effectively. This might involve setting up a server cluster where different models handle document chunks.
Response Aggregation Mechanism with Sample Code for Aggregating Results
Finally, aggregating responses from multiple chunks or models is crucial to form a cohesive output. This can be done through various techniques, depending on the task:
def aggregate_responses(responses):
# Simple aggregation example: concatenate responses
return ' '.join(responses)
# Example usage
responses = ["This is part one of the response,", "and this is part two of the response."]
final_response = aggregate_responses(responses)
print(final_response)
These strategies provide a comprehensive approach to utilizing LLMs despite token limitations, ensuring that even extensive documents can be processed accurately and efficiently. Are you ready to explore how these strategies are practically applied to address token and rate limit issues?
Addressing Token and Rate Limit Issues
Now, let's explore how effectively managing token and rate limits not only resolve specific technical issues but also enhances the overall efficiency and capability of Large Language Models (LLMs) in handling extensive data.
Overview of Benefits Relating to Token Limit Issue
Addressing token limitations is crucial for ensuring that LLMs can handle extensive documents or datasets without sacrificing performance or accuracy. By implementing strategies like chunking, summarization, and parallel processing, developers can maximize the utility of LLMs in scenarios where the volume of data would otherwise be prohibitive.
Summary of Advantages Concerning Rate Limit Issue
Similar strategies can also help manage rate limits, which restrict the frequency of data processing to prevent overload and ensure equitable resource distribution among users. Developers can avoid hitting these rate limits by optimizing how and when data is sent for processing, thus maintaining steady workflow efficiency.
How Strategies Optimize Processing Time
These strategies collectively optimize processing time by ensuring that each piece of data is handled most efficiently. For example:
Chunk Processing: Divides the workload into manageable parts, allowing for faster individual processing and more effective use of resources.
Parallel Processing: Utilizes multiple processors simultaneously, significantly reducing the time to handle large volumes of data.
Response Aggregation: By efficiently combining results from various processes, response aggregation minimizes the overhead and latency of handling large datasets.
Here’s how a combined strategy might look in practice:
# Example Python code for parallel processing and aggregation of results
from concurrent.futures import ThreadPoolExecutor
def process_chunk(chunk):
# Simulate processing (e.g., running a model prediction)
return f"Processed {chunk}"
def main(text, chunk_size):
chunks = list(chunk_text(text, chunk_size)) # Assuming chunk_text is defined
results = []
with ThreadPoolExecutor(max_workers=5) as executor:
future_to_chunk = {executor.submit(process_chunk, chunk): chunk for chunk in chunks}
for future in concurrent.futures.as_completed(future_to_chunk):
chunk = future_to_chunk[future]
try:
result = future.result()
except Exception as exc:
print(f'{chunk} generated an exception: {exc}')
else:
results.append(result)
return aggregate_responses(results) # Assuming aggregate_responses is defined
# Example usage
large_text = "Here is some very long text that needs to be split and processed in parallel to avoid token and rate limits."
print(main(large_text, 10))
This example demonstrates how to process text in parallel, reducing overall processing time and efficiently handling larger volumes than typically allowed by token and rate limits.
By effectively addressing token and rate limit issues, organizations can enhance the scalability and responsiveness of their LLM applications, ensuring they remain robust across a range of demanding scenarios.
Finally, as the field of AI continues to evolve, exploring low-code solutions that incorporate these strategies can significantly reduce the barrier to entry for deploying advanced AI technologies. Low-code platforms that support modular, scalable approaches to token management will be particularly valuable in democratizing AI, making powerful tools like LLMs accessible to a broader range of users and industries.
In conclusion, while token limitations present significant challenges, the strategies and technologies developed to overcome them not only solve practical issues but also drive forward the capabilities of AI in processing natural language at scale. As we refine these approaches and technologies, the scope of what's possible with LLMs will undoubtedly expand, offering exciting prospects for the future of AI and machine learning.
Conclusion
Understanding token limitations in LLMs is crucial for anyone developing or deploying natural language processing applications at scale. If not properly managed, these limitations can significantly restrict the effectiveness of LLMs, especially when dealing with large volumes of text. By employing strategic approaches to managing these limitations, developers can unlock the full potential of LLMs, making them more versatile and capable in various complex scenarios.
The strategies discussed—ranging from text truncation and chunk processing to advanced techniques like summarization and parallel processing—provide robust solutions to the challenges posed by token limits. These approaches ensure that large documents are processed efficiently and enhance the overall performance of LLMs by allowing them to handle more data without compromising speed or accuracy.
Real-world Impact: In practical applications, these strategies have proven highly effective. For instance, in legal and medical fields where document length often exceeds standard token limits, chunking, and summarization allow for thorough analysis without losing critical information. Similarly, in customer service, these strategies enable handling extensive customer interactions in real-time, improving both response quality and customer satisfaction.
Large Language Models (LLMs) such as ChatGPT represent a significant breakthrough in artificial intelligence. These models process and generate human-like text by learning from vast datasets of existing text. By understanding context and nuances in language, LLMs can engage in conversations, answer queries, and even create content that is often indistinguishable from that written by humans.
In Natural Language Processing (NLP), LLMs have transformed how machines understand and interact with human language. Their ability to analyze, interpret, and predict text data has applications across numerous industries, from automating customer service interactions to aiding in complex decision-making processes.
Challenges in Natural Language Processing with Large Documents
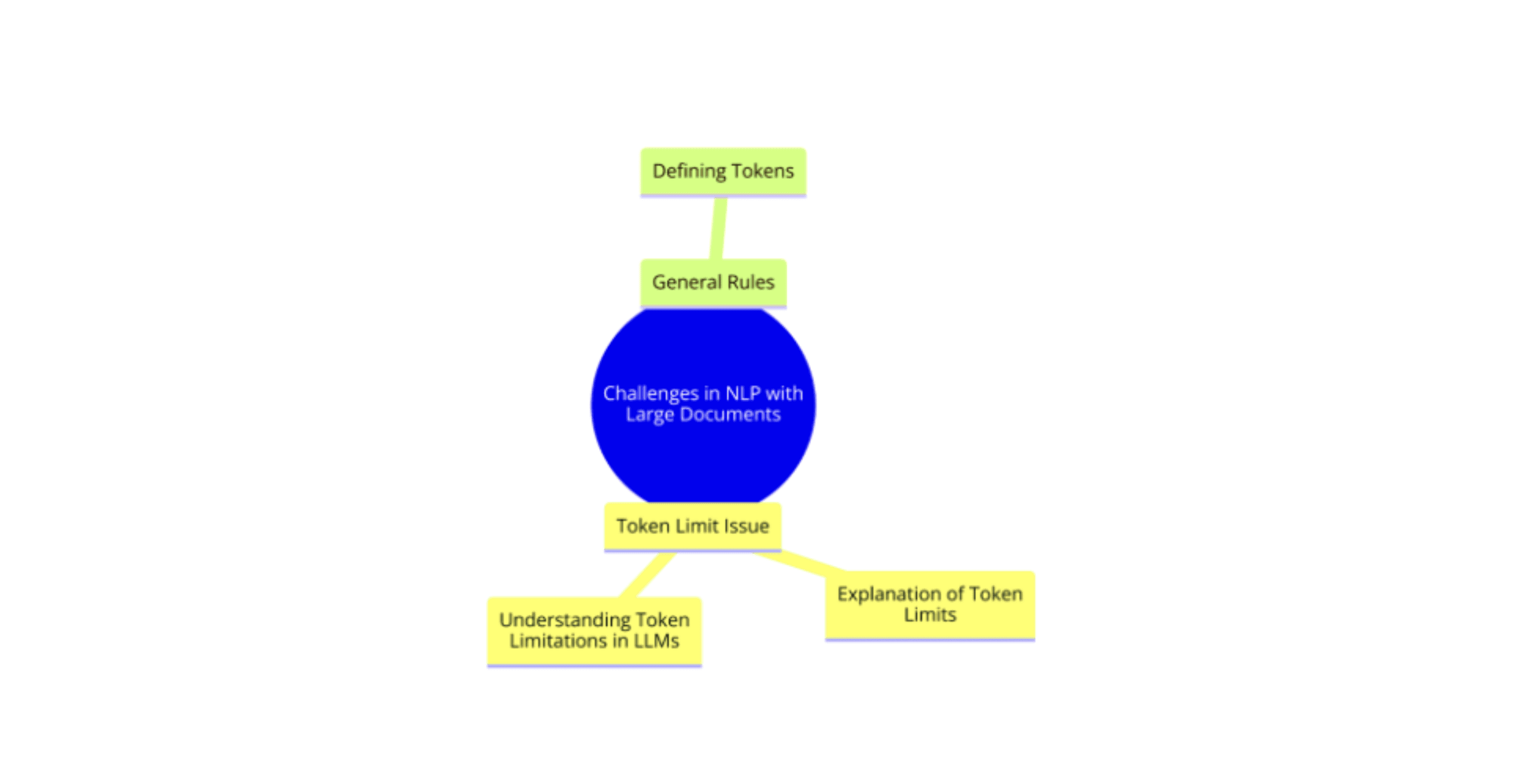
Despite their advanced capabilities, LLMs need help with processing large documents. These challenges primarily arise from the inherent limitations in handling lengthy texts due to the input data size or the computational demands required to process them effectively.
Token Limit Issue Explained
One particular challenge is the token limit issue. In NLP, texts are broken down into tokens (which can be words or parts of words), and LLMs like ChatGPT have a maximum number of tokens they can process in one go. This limit often restricts the model's ability to handle longer documents, affecting its effectiveness in contexts requiring detailed document analysis.
Understanding Token Limitations in LLMs
A "token" is a language model's basic text unit in language processing. Depending on the model's design, a token can be a word, part of a word (like a syllable), or even punctuation. Tokens are crucial because they are input data for language models to analyze and generate text. The more effectively a model can break down and understand these tokens, the better it can perform tasks involving language comprehension and generation.
General Rules for Defining Tokens
Tokens are typically defined using tokenization, where text is split into manageable pieces. The rules for tokenization can vary depending on the language, the task's specifics, or the language model's design. For instance, some models treat words as tokens, while others include spaces and punctuation.
Tokenization by Example and Methods
For example, the sentence "Hello, world!" could be tokenized into ["Hello", ",", "world", "!"] in a word-based approach. In contrast, using a tool like the Keras Tokenizer, which is popular in machine learning applications, might involve converting each token into numerical values representing their position in a learned index for processing efficiency.
from keras.preprocessing.text import Tokenizer
# Example of setting up a Keras Tokenizer
tokenizer = Tokenizer()
text = ["Hello, world!"]
tokenizer.fit_on_texts(text)
sequence = tokenizer.texts_to_sequences(text)
print(sequence)
Strategies to Overcome Token Limitations

Truncation with Example of Truncating Text in Python
One straightforward approach to manage token limitations is truncation, where the text exceeding the token limit is simply cut off. While this method is easy to implement, it risks losing important information that might be at the end of the text. Here’s how you might truncate text in Python:
def truncate_text(text, max_length):
# Split the text into tokens
tokens = text.split()
# Truncate the tokens to the maximum length allowed
if len(tokens) > max_length:
tokens = tokens[:max_length]
return ' '.join(tokens)
# Example usage
sample_text = "This is an example text that might be too long for certain processing limits."
truncated_text = truncate_text(sample_text, 10) # Limit to 10 tokens
print(truncated_text)
Chunk Processing Including Processing Text in Smaller Chunks
To ensure no critical information is missed, the text can be divided into smaller chunks that fit within the token limit. This method involves processing each chunk separately and combining the results, if necessary. This approach is beneficial for tasks like sentiment analysis or when the overall context does not need to be continuous.
Summarization Techniques to Reduce Token Count
Another effective strategy is to use summarization techniques to condense the text without losing critical information. Automated summarization tools can reduce the length of the text while retaining key points, thereby decreasing the number of tokens.
Remove Redundant Terms with an Example of Stop Word Removal Using NLTK
Removing redundant terms, such as common stop words, can also help reduce the token count. Here's an example using the Natural Language Toolkit (NLTK) to remove stop words:
import nltk
from nltk.corpus import stopwords
nltk.download('stopwords')
def remove_stop_words(text):
stop_words = set(stopwords.words('english'))
tokens = text.split()
filtered_tokens = [word for word in tokens if not word in stop_words]
return ' '.join(filtered_tokens)
# Example usage
text_with_stopwords = "This is an example where the stop words will be removed from the text."
cleaned_text = remove_stop_words(text_with_stopwords)
print(cleaned_text)
Fine-tuning Language Models
Fine-tuning language models on specific domains or shorter texts can also help them perform better within the constraints of token limits, adapting their capabilities to more focused content types.
Document Chunking with Description and Example Code for Splitting
Document chunking involves breaking a document into smaller, more manageable pieces. Here's a simple way to chunk text based on a specified number of tokens:
def chunk_text(text, chunk_size):
tokens = text.split()
for i in range(0, len(tokens), chunk_size):
yield ' '.join(tokens[i:i+chunk_size])
# Example usage
full_text = "Here is some longer text that needs to be split into smaller chunks for processing."
chunks = list(chunk_text(full_text, 10)) # Each chunk will have 10 tokens
print(chunks)
Parallel Processing with Explanation and Sample Code for Concurrent Processing
Parallel processing can handle multiple chunks concurrently, speeding up the overall processing time. This approach utilizes various CPU or GPU resources to process different text sections simultaneously.
Model Distribution Strategy with Example for Assigning Models
Distributing the workload across multiple model instances can also help manage significant texts more effectively. This might involve setting up a server cluster where different models handle document chunks.
Response Aggregation Mechanism with Sample Code for Aggregating Results
Finally, aggregating responses from multiple chunks or models is crucial to form a cohesive output. This can be done through various techniques, depending on the task:
def aggregate_responses(responses):
# Simple aggregation example: concatenate responses
return ' '.join(responses)
# Example usage
responses = ["This is part one of the response,", "and this is part two of the response."]
final_response = aggregate_responses(responses)
print(final_response)
These strategies provide a comprehensive approach to utilizing LLMs despite token limitations, ensuring that even extensive documents can be processed accurately and efficiently. Are you ready to explore how these strategies are practically applied to address token and rate limit issues?
Addressing Token and Rate Limit Issues
Now, let's explore how effectively managing token and rate limits not only resolve specific technical issues but also enhances the overall efficiency and capability of Large Language Models (LLMs) in handling extensive data.
Overview of Benefits Relating to Token Limit Issue
Addressing token limitations is crucial for ensuring that LLMs can handle extensive documents or datasets without sacrificing performance or accuracy. By implementing strategies like chunking, summarization, and parallel processing, developers can maximize the utility of LLMs in scenarios where the volume of data would otherwise be prohibitive.
Summary of Advantages Concerning Rate Limit Issue
Similar strategies can also help manage rate limits, which restrict the frequency of data processing to prevent overload and ensure equitable resource distribution among users. Developers can avoid hitting these rate limits by optimizing how and when data is sent for processing, thus maintaining steady workflow efficiency.
How Strategies Optimize Processing Time
These strategies collectively optimize processing time by ensuring that each piece of data is handled most efficiently. For example:
Chunk Processing: Divides the workload into manageable parts, allowing for faster individual processing and more effective use of resources.
Parallel Processing: Utilizes multiple processors simultaneously, significantly reducing the time to handle large volumes of data.
Response Aggregation: By efficiently combining results from various processes, response aggregation minimizes the overhead and latency of handling large datasets.
Here’s how a combined strategy might look in practice:
# Example Python code for parallel processing and aggregation of results
from concurrent.futures import ThreadPoolExecutor
def process_chunk(chunk):
# Simulate processing (e.g., running a model prediction)
return f"Processed {chunk}"
def main(text, chunk_size):
chunks = list(chunk_text(text, chunk_size)) # Assuming chunk_text is defined
results = []
with ThreadPoolExecutor(max_workers=5) as executor:
future_to_chunk = {executor.submit(process_chunk, chunk): chunk for chunk in chunks}
for future in concurrent.futures.as_completed(future_to_chunk):
chunk = future_to_chunk[future]
try:
result = future.result()
except Exception as exc:
print(f'{chunk} generated an exception: {exc}')
else:
results.append(result)
return aggregate_responses(results) # Assuming aggregate_responses is defined
# Example usage
large_text = "Here is some very long text that needs to be split and processed in parallel to avoid token and rate limits."
print(main(large_text, 10))
This example demonstrates how to process text in parallel, reducing overall processing time and efficiently handling larger volumes than typically allowed by token and rate limits.
By effectively addressing token and rate limit issues, organizations can enhance the scalability and responsiveness of their LLM applications, ensuring they remain robust across a range of demanding scenarios.
Finally, as the field of AI continues to evolve, exploring low-code solutions that incorporate these strategies can significantly reduce the barrier to entry for deploying advanced AI technologies. Low-code platforms that support modular, scalable approaches to token management will be particularly valuable in democratizing AI, making powerful tools like LLMs accessible to a broader range of users and industries.
In conclusion, while token limitations present significant challenges, the strategies and technologies developed to overcome them not only solve practical issues but also drive forward the capabilities of AI in processing natural language at scale. As we refine these approaches and technologies, the scope of what's possible with LLMs will undoubtedly expand, offering exciting prospects for the future of AI and machine learning.
Conclusion
Understanding token limitations in LLMs is crucial for anyone developing or deploying natural language processing applications at scale. If not properly managed, these limitations can significantly restrict the effectiveness of LLMs, especially when dealing with large volumes of text. By employing strategic approaches to managing these limitations, developers can unlock the full potential of LLMs, making them more versatile and capable in various complex scenarios.
The strategies discussed—ranging from text truncation and chunk processing to advanced techniques like summarization and parallel processing—provide robust solutions to the challenges posed by token limits. These approaches ensure that large documents are processed efficiently and enhance the overall performance of LLMs by allowing them to handle more data without compromising speed or accuracy.
Real-world Impact: In practical applications, these strategies have proven highly effective. For instance, in legal and medical fields where document length often exceeds standard token limits, chunking, and summarization allow for thorough analysis without losing critical information. Similarly, in customer service, these strategies enable handling extensive customer interactions in real-time, improving both response quality and customer satisfaction.